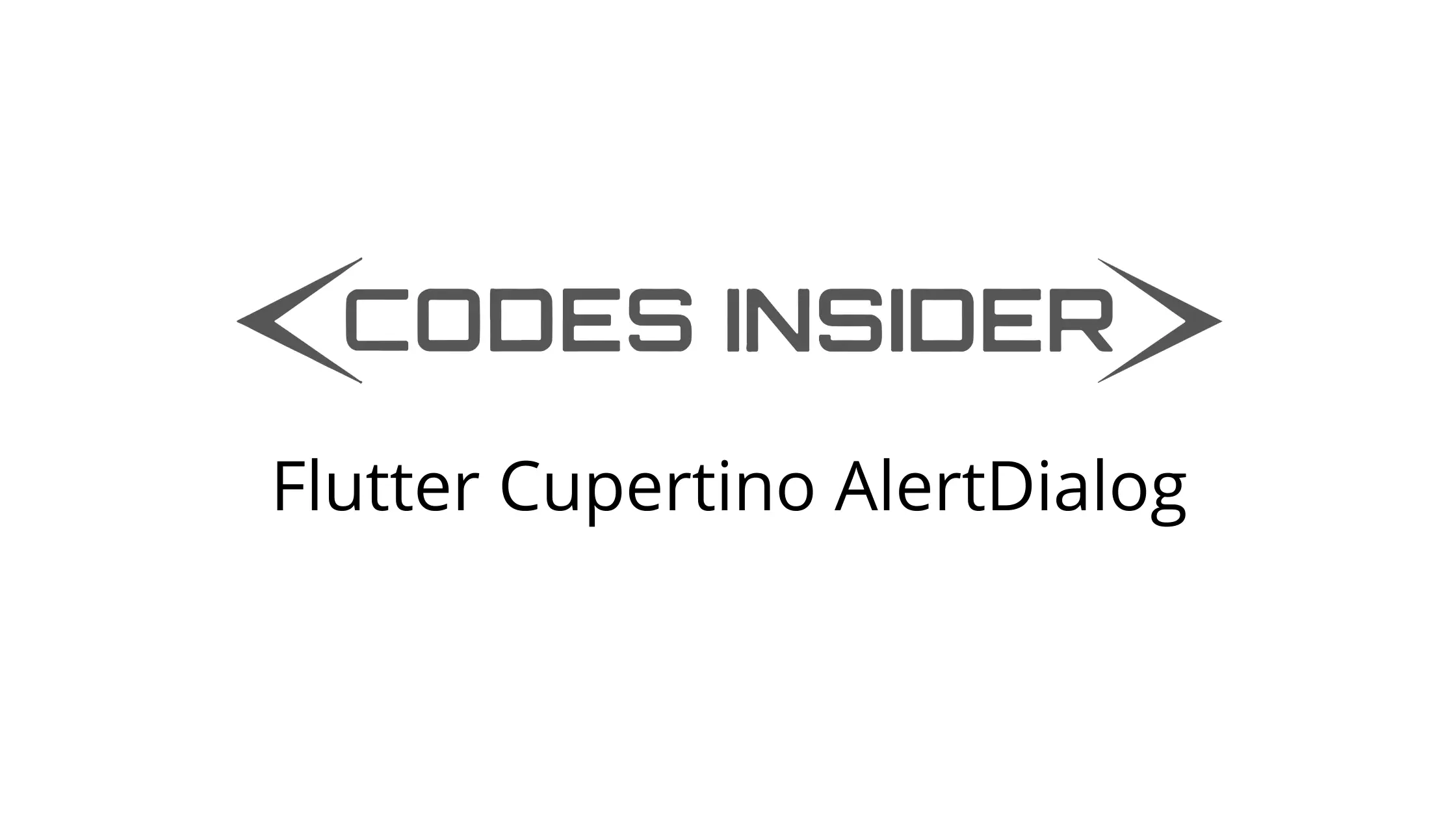
Flutter Cupertino Alert Dialog
Cupertino Alert dialog is a widget in flutter used to display ios style alert dialog. We will use it to take confirmation from the user while performing an action. For example, while deleting a file it will ask for confirmation whether to delete or cancel the operation. Alert dialog has three properties title, content, and actions. The title is displayed at the top followed by content and actions. Though these properties are optional we have to use them as these are basic things of a dialog.
Don’t know what Cupertino is? it is nothing but a set of flutter widgets that follow the ios design pattern. These widgets are designed to implement ios features in flutter apps built for the ios platform.
In this tutorial, we will learn how to use cupertino alert dialog in flutter with example. We will also learn how to customize the widget using different properties.
To create alert dialog for android platform consider using flutter alertdialog which follows material design.
How To Create Cupertino Alert Dialog In Flutter?
To create a cupertino alert dialog in flutter we have to call the constructor of CupertinoAlertDialog class and provide required properties. Since there are no required properties for cupertino alert dialog we can use our desired ones. Generally, we will use title and actions which make the alert dialog complete. We will use Text widget for Title and content. For actions we will use CupertinoDialogAction widget.
Cupertion Alert Dialog Constructor:
CupertinoAlertDialog(
{Key? key,
Widget? title,
Widget? content,
List<Widget> actions,
ScrollController? scrollController,
ScrollController? actionScrollController,
Duration insetAnimationDuration,
Curve insetAnimationCurve}
)
Generally, we have to show alert dialogs when some event is triggered like button click, etc. We can’t display them directly like other flutter widgets. We have to use showCupertinoDialog function to display a cupertino alert dialog. This function takes CupertinoAlertdialog as child and displays is when we call it.
ShowCupertinoDialog Constructor:
showCupertinoDialog<T>(
{required BuildContext context,
required WidgetBuilder builder,
String? barrierLabel,
bool useRootNavigator = true,
bool barrierDismissible = false,
RouteSettings? routeSettings}
)
Flutter Cupertino Alert Dialog Properties
The properties of cupertino alert dialog are:
- title
- content
- actions
- scrollController
- actionScrollController
- insetAnimationDuration
- insetAnimationCurve
title
To change or add title to the cupertino alert dialog we will use title property. It takes text widget as value.
CupertinoAlertDialog(
title: Text("Delete File"),
);
Output:
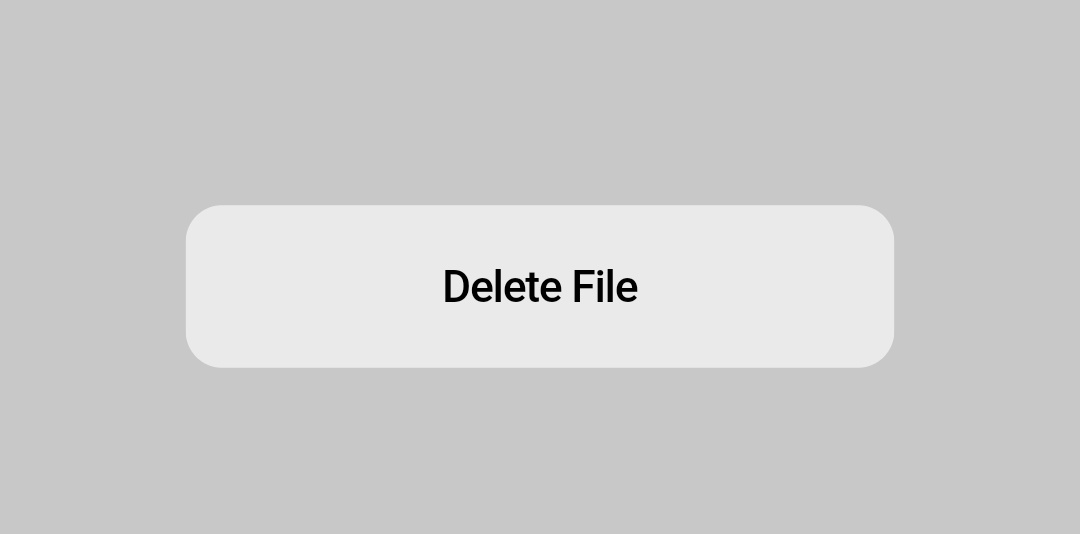
content
To add a message to the user on cupertino alert dialog we will use content property. It also takes a text widget as value.
CupertinoAlertDialog(
title: Text("Delete File"),
content: Text("Are you sure you want to delete the file?"),
);
Output:
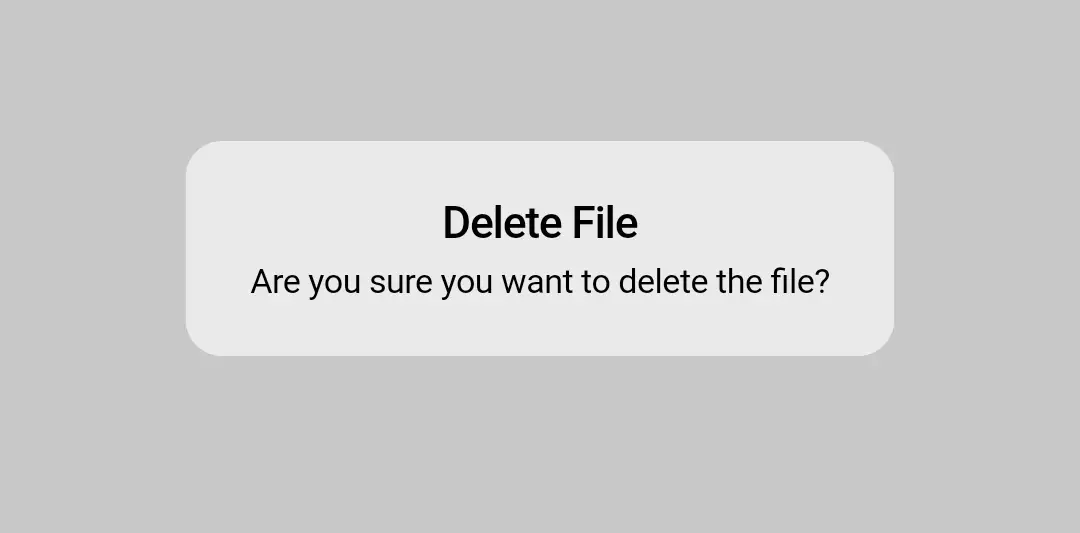
actions
To add actions to the cupertino alert dialog we will use content property. Generally, we will use CupertinoDialogAction widgets for actions.
CupertinoAlertDialog(
title: Text("Delete File"),
content: Text("Are you sure you want to delete the file?"),
actions: [
CupertinoDialogAction(
child: Text("YES"),
onPressed: ()
{
Navigator.of(context).pop();
}
),
CupertinoDialogAction(
child: Text("NO"),
onPressed: (){
Navigator.of(context).pop();
}
)
],
);
Output:
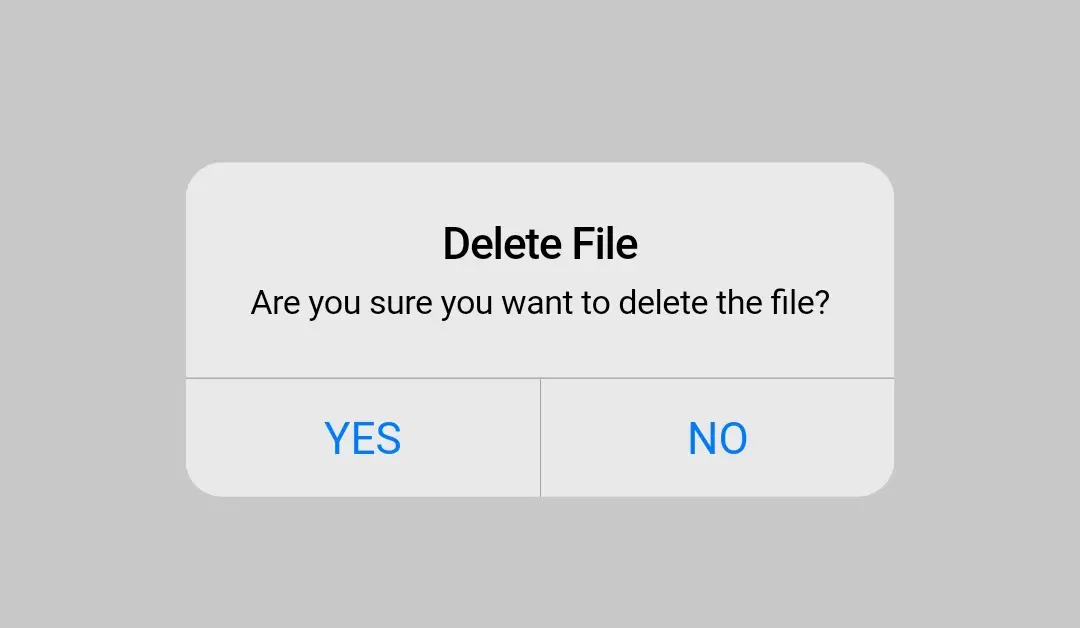
If you want to dismiss the dialog even if the user did’nt select an action set the barrierDismissible property of showCupertionDialog to true. By default this value will be false.
showCupertinoDialog(
context:context,
builder:(){
CupertinoAlertDialog();
}
barrierDiamissible: true,
)
Flutter Cupertino Alert Dialog Example
Lets create an example which demonstrates the use of cupertino alert dialog. In this example we will display a button clicking on which will dispay a cupertino alert dialog. The dialog will ask the user to confirm deletion of a file.
First we will display a button which will trigger our alert dialog.
ElevatedButton(
child:Text("Delete File"),
onPressed: ()
{
},
)
Now, lets create a function showDialog() inside which we will call the showCupertinoDialog by providing cupertinoAlertDialog as child.
void showDialog()
{
showCupertinoDialog(
context: context,
builder: (context) {
return CupertinoAlertDialog(
title: Text("Delete File"),
content: Text("Are you sure you want to delete the file?"),
actions: [
CupertinoDialogAction(
child: Text("YES"),
onPressed: ()
{
Navigator.of(context).pop();
}
),
CupertinoDialogAction(
child: Text("NO"),
onPressed: (){
Navigator.of(context).pop();
}
,
)
],
);
},
);
}
Now lets call the function inside onPressed() callback of the button created in the beginnig.
ElevatedButton(
child:Text("Delete File"),
onPressed: ()
{
showDialog();
},
)
Complete Code:
Lets sum up all the code snippets above to complete our cupertino alert dialog example.
import 'dart:async';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
double value = 0;
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: Text("Flutter Cupertino Alert Dialog"),
),
body:Container(
margin: EdgeInsets.all(40),
alignment: Alignment.topCenter,
child: ElevatedButton(
child:Text("Show Dialog"),
onPressed: ()
{
showDialog();
},
)
),
);
}
void showDialog()
{
showCupertinoDialog(
context: context,
builder: (context) {
return CupertinoAlertDialog(
title: Text("Delete File"),
content: Text("Are you sure you want to delete the file?"),
actions: [
CupertinoDialogAction(
child: Text("YES"),
onPressed: ()
{
Navigator.of(context).pop();
}
),
CupertinoDialogAction(
child: Text("NO"),
onPressed: (){
Navigator.of(context).pop();
}
,
)
],
);
},
);
}
}
Output:
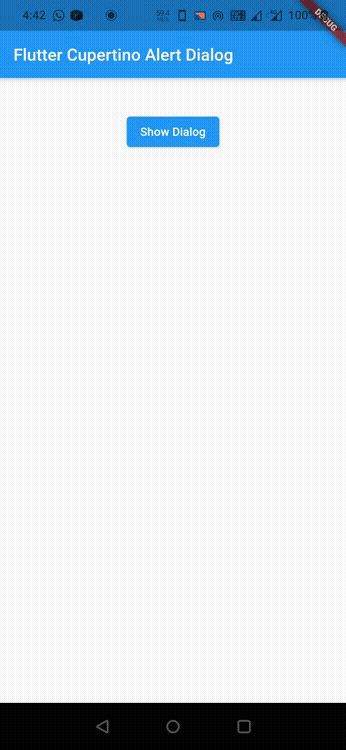
That brings an end to the tutorial about how to create and use Cupertino Alert Dialog widget in flutter with example. I hope you understand the tutorial. Let’s catch up with some other widget in the next post. Have a great day!!
Do share, subscribe, and like my Facebook page if you find this post helpful. Thank you!!
Reference: Flutter Official Documentation.

Leave a Reply