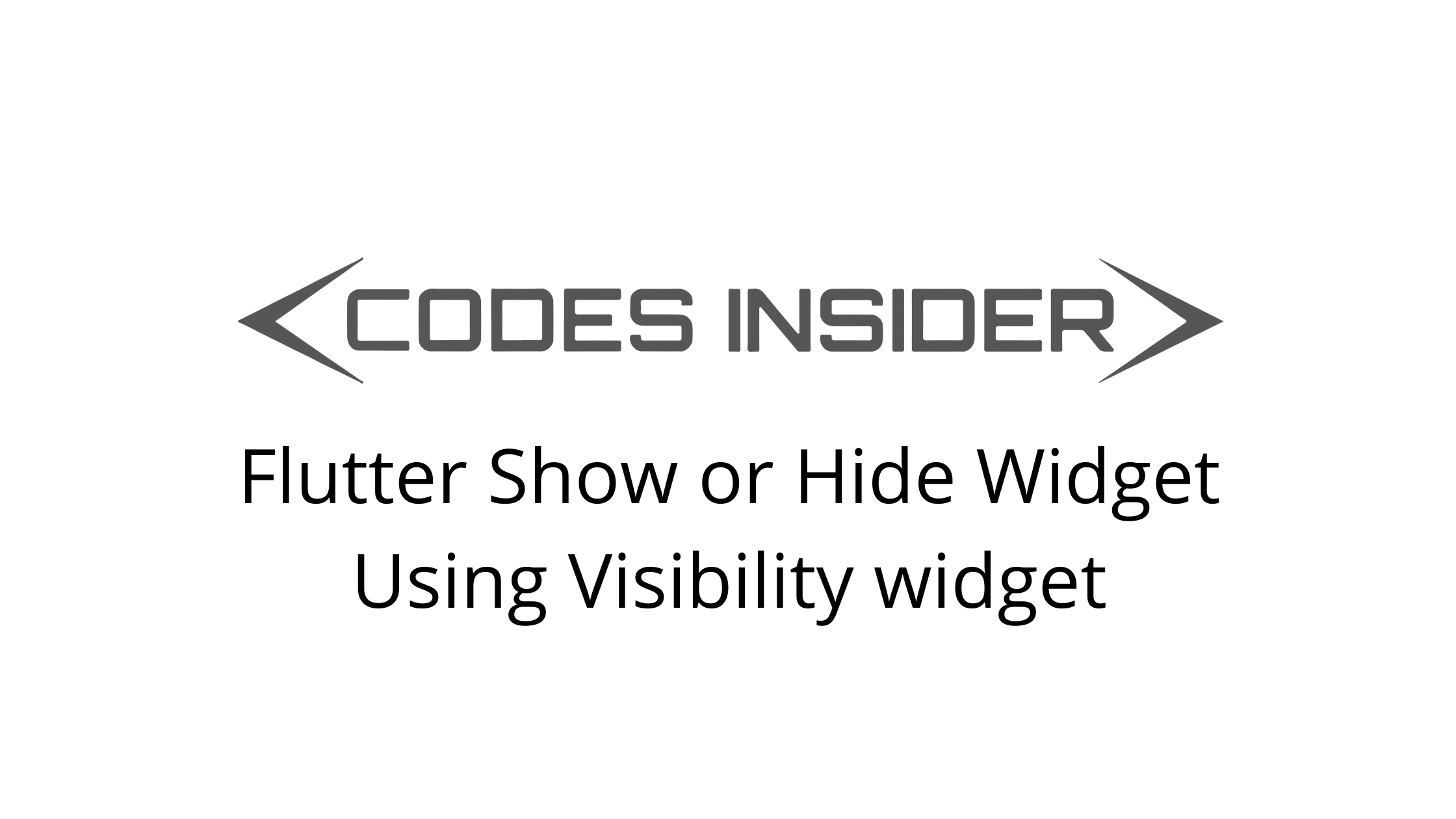
In flutter we can show or hide widgets using visibility widget. While designing mobile apps using flutter we might have to show or hide a widget based on some condition. For example, we will implement this kind of behavior while designing social networking apps. This feature comes in handy to control whether to show or hide the profile info.
Flutter Visibility Widget
Visibility is a widget that is useful to show or hide other widgets in flutter. We have to wrap the widget we want to show or hide inside the visibility widget as a child. This widget has a property called visible which controls the state (visible or invisible) of the child. Instead of hiding the child, we can also replace it with another widget. This widget gives us control over whether to maintain the space for the widget when it gets invisible.
In this tutorial, we will learn how to use a visibility widget to show or hide widgets in flutter with example. We will also learn how to customize the style of the Visibility widget using different properties.
How To Create Visibility Widget In Flutter ?
To create a visibility widget in flutter we have to use Visibility class. We have to call the constructor of the class and provide the required properties. The visibility widget has one required property child. The child can be any widget. We can use the remaining properties to customize the style and behavior based on our requirements.
Flutter Visibility Constructor:
Visibility(
{Key? key,
required Widget child,
Widget replacement,
bool visible,
bool maintainState,
bool maintainAnimation,
bool maintainSize,
bool maintainSemantics,
bool maintainInteractivity}
)
Flutter Visibility Widget Properties
The propertis of visibility widget are:
- child
- replacement
- visible
- maintainSize
- maintainInteractivity
- maintainState
- maintainAnimation
- maintainSemantics
child
The widget we want to show or hide will be the child. It can be any widget.
Visibility(
child:Image.asset("assets/images/burger.jpg")
)
Output:
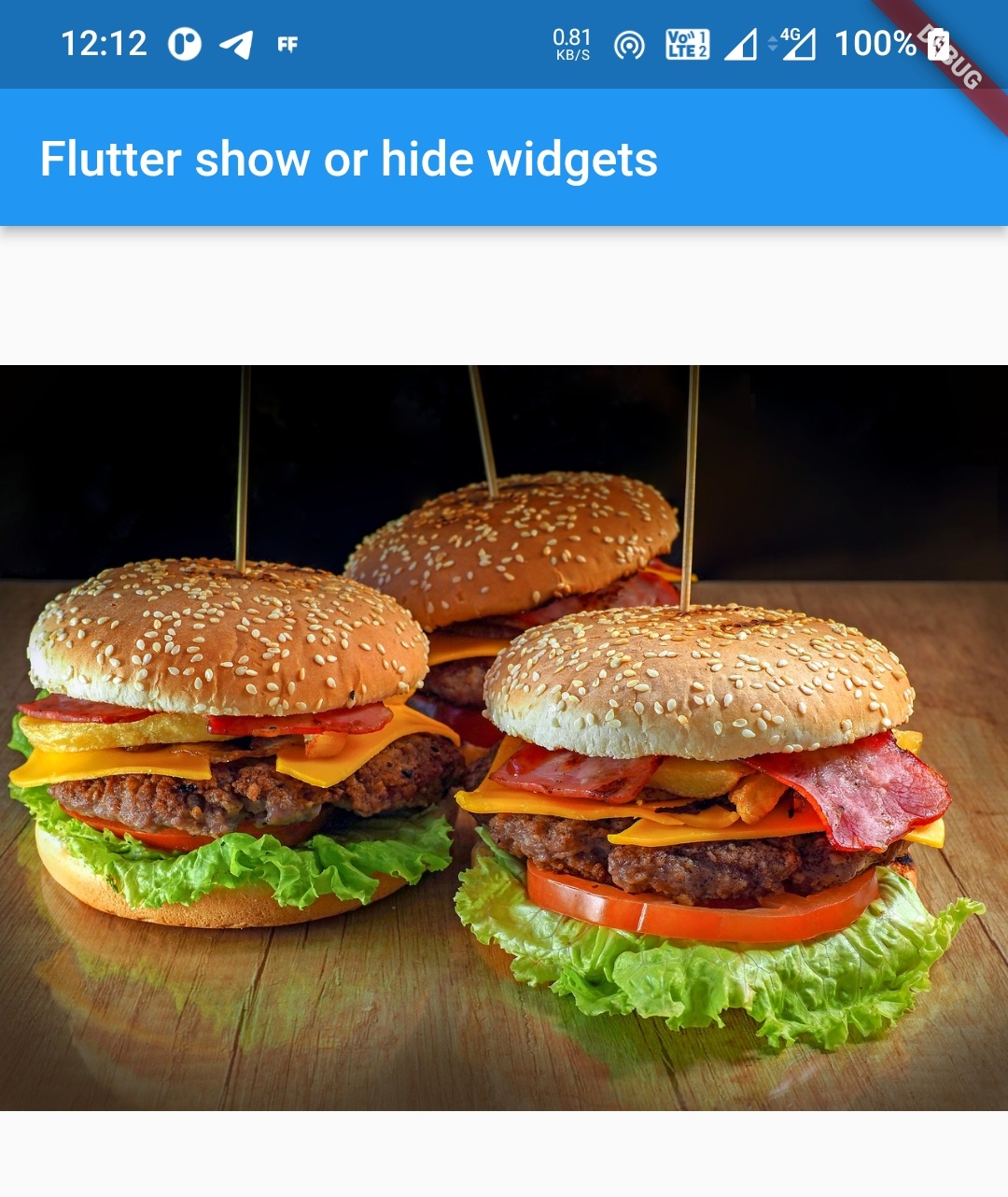
visible
This takes boolean as value. It controls whether to show or hide the child widget. By default the value is true.
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
)
Output:
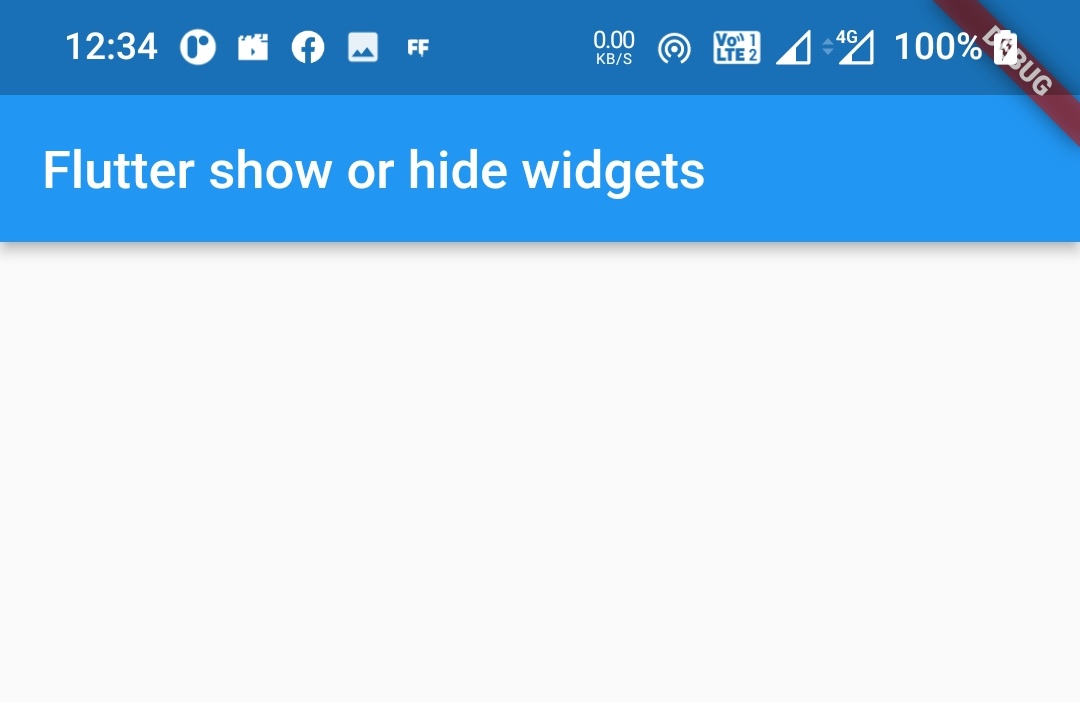
replacement
We will use this property to replace the child with another widget instead when visible is false. By default the value is false.
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
replacement: Image.asset("assets/images/pizza.jpg"),
),
Output:
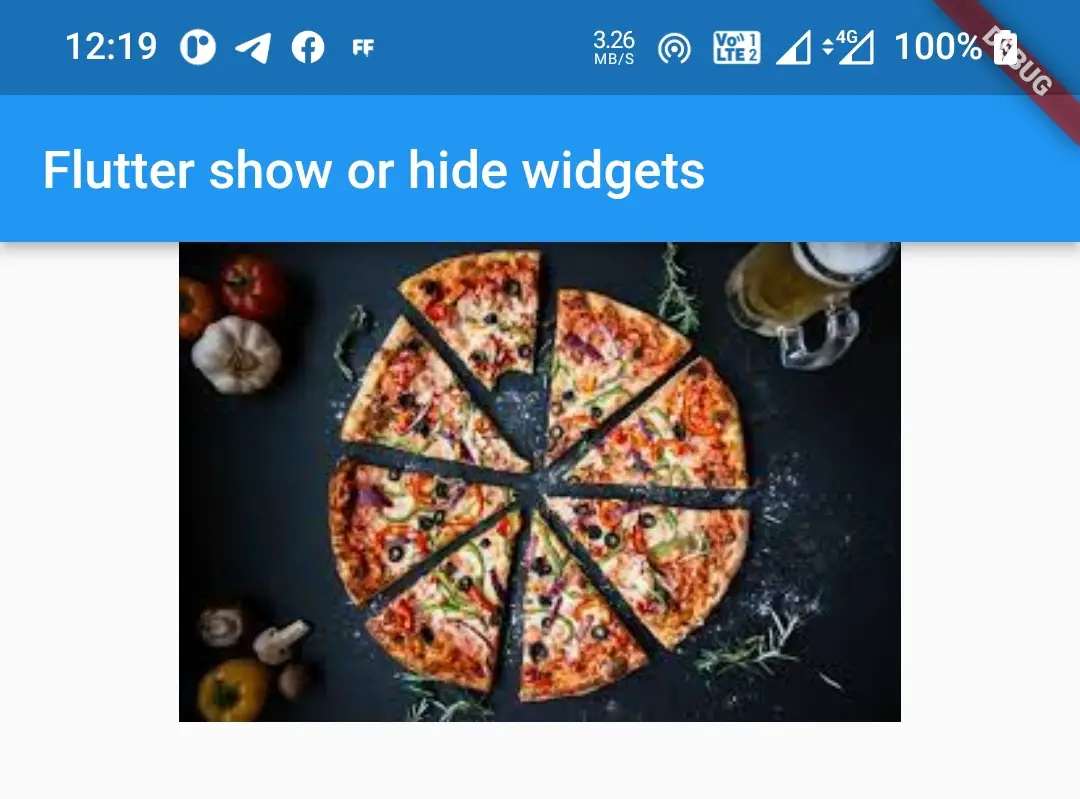
maintainState
We will use this property to control whether to maintain state objects of the child when it is hidden. It takes boolean as value. By default the value is false.
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
maintainState: true,
),
maintainAnimation
It takes boolean as value. We will use this property to control whether to maintain the animations of the child when it is hidden. By default the value is false.
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
maintainState: true,
maintainAnimation:tue,
),
maintainSize
We will use this property to control wheter or not to maintain the space after the child is hidden. It takes boolean as value. If we set this to true it will hide the widget but will maintain the space of that widget. If false it will not maintain the space for that widget. For this we have to set both maintainState and maintainAnimation properties to true. By default the value is false.
true
Column(
children: [
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
maintainSize: true,
maintainAnimation: true,
maintainState: true,
),
SizedBox(
height:20
),
Text("Example Text")
],
)
Output:
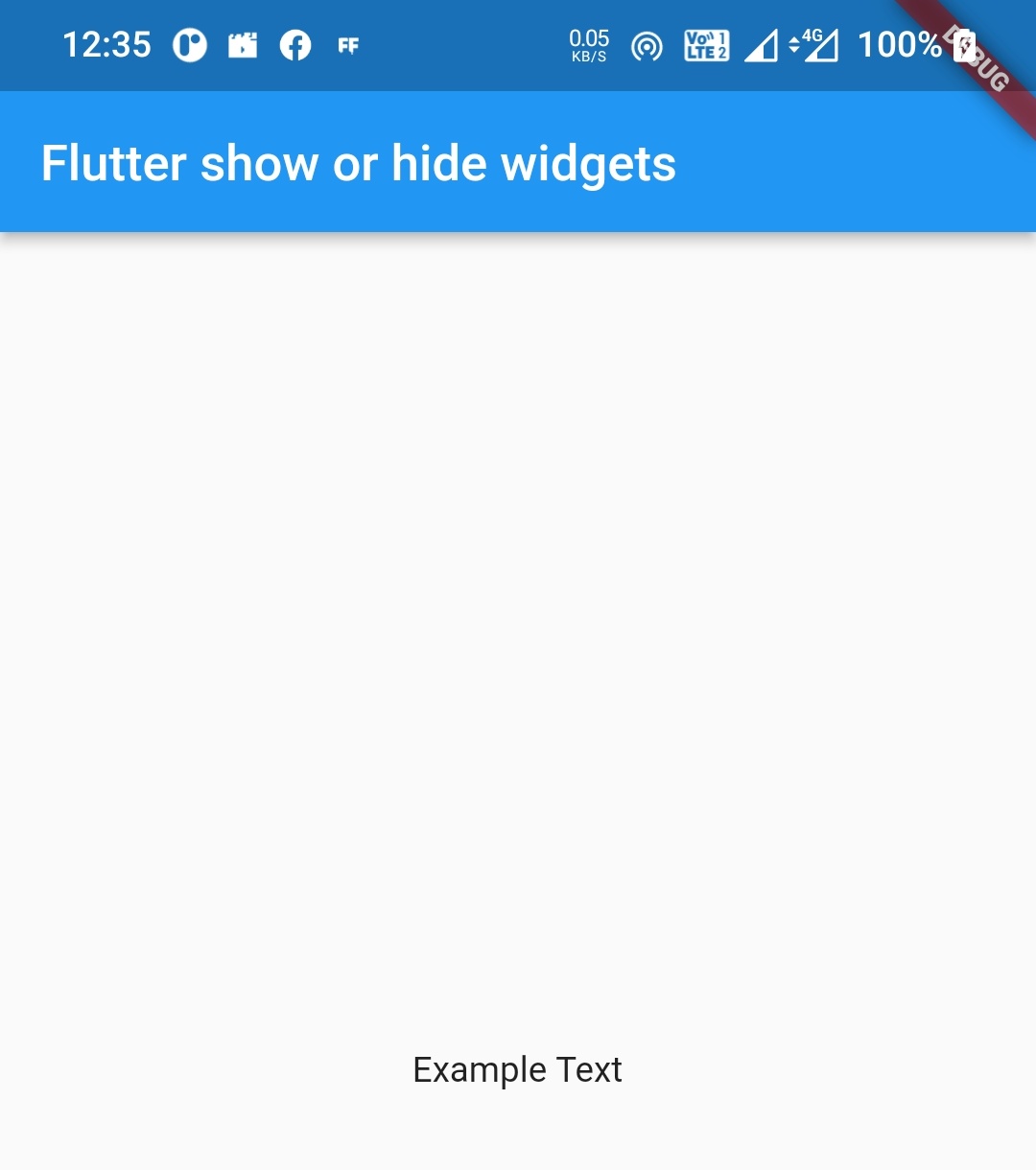
false
Column(
children: [
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
maintainSize: false,
maintainAnimation: true,
maintainState: true,
),
SizedBox(
height:20
),
Text("Example Text")
],
)
Output:
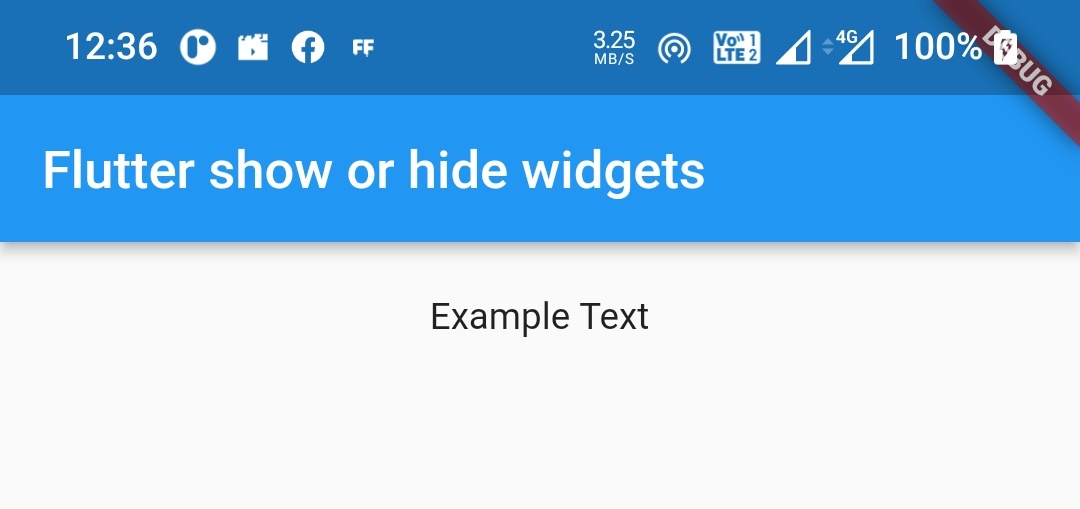
maintainInteractivity
We will use this property to control whether to maintain interactivity when the child is hidden. It takes boolean as value. If set to true the child will respond to touch event (if any) even when it is hidden. If false it will not respond to touch event. The maintainState, maintainAnimation, and maintainSize properties must be true for this property to work. By default the value is false.
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
maintainSize: true,
maintainAnimation: true,
maintainState: true,
maintainInteractivity: true
),
maintainSemantics
It takes boolean as value. We will use this property to control whether to maintain semantics of the child when it is hidden. We have to set maintainState, maintainAnimation, and maintainSize properties to true for this property to work. By default the value is false.
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: false,
maintainSize: true,
maintainAnimation: true,
maintainState: true,
maintainSemantics:true
),
Flutter Show or Hide Widget Example Using Visibility Widget
Let’s create an example where we will show or hide a widget using visibility widget in flutter. In this example, we will display a switch and an image. Toggling the switch will show and hide the image. For this purpose, we will need two boolean variables. One will hold the state of the switch and the other will hold the state of the child.
Declare the variables switchValue and isVisible.
bool switchValue = false;
bool isVisible = true;
Implement the code for displaying the switch and the visibility widget with an image as a child. Wrap the switch and the visibility widget inside a column widget. Provide switchValue variable to value property of the switch and isVisible variable to visible property of visibility widget.
body:Container(
margin: EdgeInsets.all(0),
width: double.infinity,
height: MediaQuery.of(context).size.height*0.50,
child: Column(
children: [
Switch(
value: switchValue,
onChanged: (value) {
},
),
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible:isVisible,
),
],
)
),
Inside onChanged callback of the switch widget update the values of switchValue and isVisible variables. Call setState to update the UI with the changes.
Switch(
value: switchValue,
onChanged: (value) {
switchValue = value;
isVisible = !isVisible;
setState(() {
});
},
),
Complete Code
Let’s complete our example about how to show or hide a widget using the visibility widget in flutter by summing up the code snippets above. Create a new flutter project and replace the code in main.dart file with the code below.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
bool switchValue = false;
bool isVisible = true;
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: Text("Flutter show or hide widgets"),
),
body:Container(
margin: EdgeInsets.all(0),
width: double.infinity,
height: MediaQuery.of(context).size.height*0.50,
child: Column(
children: [
Switch(
value: switchValue,
onChanged: (value) {
switchValue = value;
isVisible = !isVisible;
setState(() {
});
},
),
Visibility(
child:Image.asset("assets/images/burger.jpg"),
visible: isVisible,
),
],
)
),
);
}
}
Output:
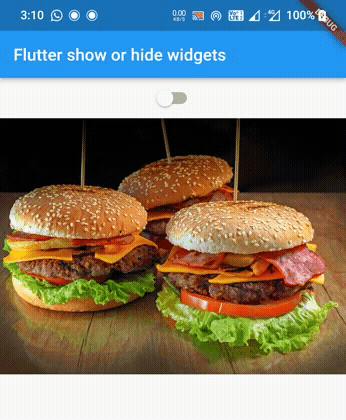
I hope you understand the tutorial on how to create & use Visibility widget to show or hide a widget in flutter. We have also seen an example where we have used the Visibility widget and customized its style. Let’s catch up with some other widgets in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official Documentation.

Leave a Reply