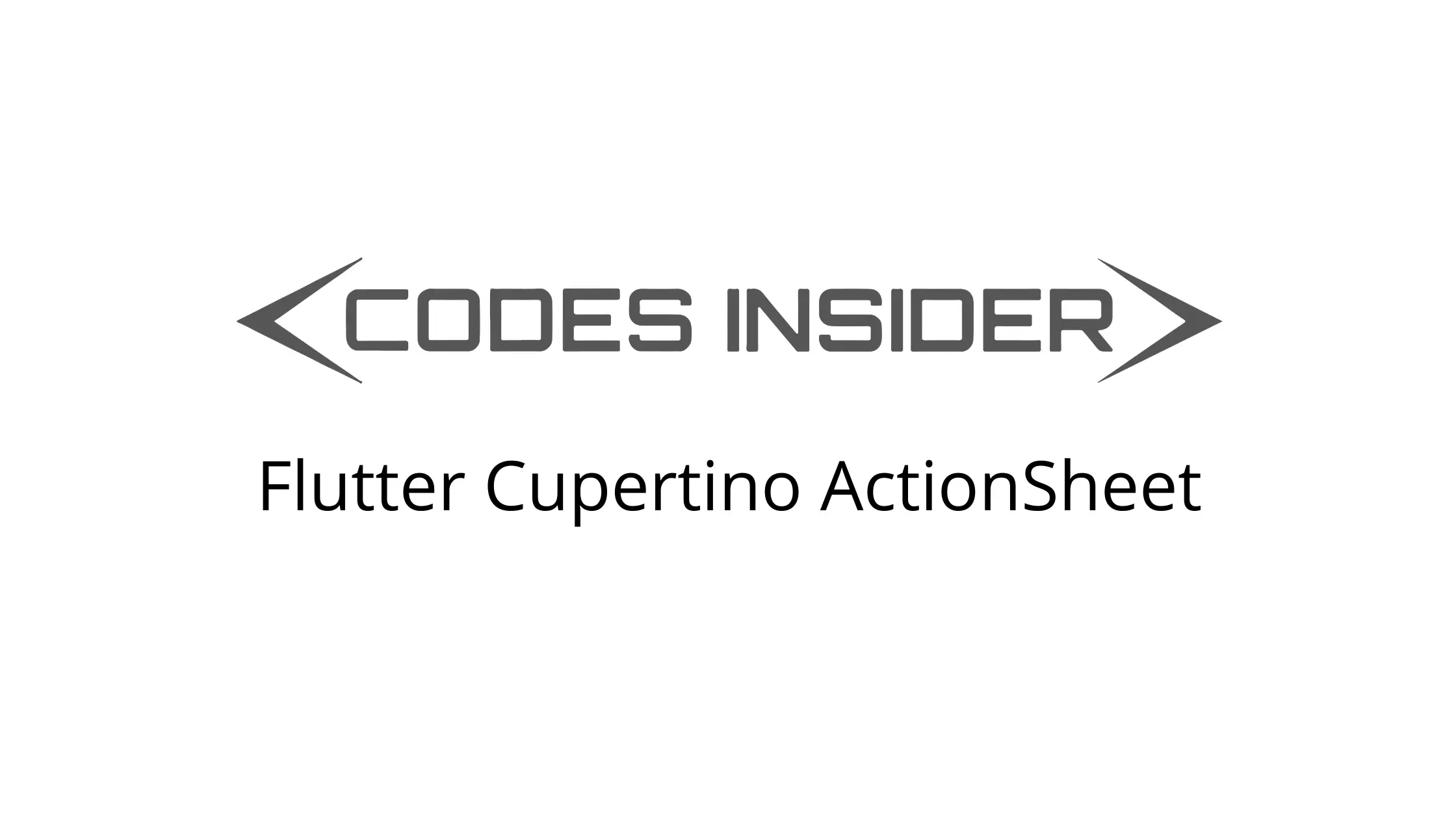
Flutter Cupertino Action Sheet
Cupertino action sheet in flutter is a widget used to display ios style action sheet. It is kind of an alert that slides in from the bottom of the screen. It provides the user with two or more options to choose from. Generally, a cupertino action sheet will have a title, message, and actions. We can also provide a cancel button to dismiss the action sheet.
Don’t know what Cupertino is? it is nothing but a set of flutter widgets that follow the ios design pattern. These widgets are designed to implement ios features in flutter apps built for the ios platform.
In this tutorial, we will learn how to use cupertino action sheet in flutter with example. We will also learn how to customize the widget using different properties.
Also read: Flutter Bottom Sheet Example.
How To Create Cupertino ActionSheet In Flutter ?
To create a cupertino action sheet in flutter we have to call the constructor of the CupertinoActionSheet Class & provide the required arguments. The constructor has no required properties. But to make the cupertino action sheet complete we have to provide the title, message, and actions. Generally, we will use text widgets for title & message properties. The actions will be CupertionActionSheetAction widgets.
Action sheets are not intended to display continuously like other widgets. We have to show them when an event is triggered, like a button click, etc. Since we can’t display an action sheet directly we have to use showCupertinoModalPopup function. This function takes Cupertino ActionSheet as a child and displays it when we call it.
Flutter Cupertino ActionSheet Constructor:
CupertinoActionSheet({
Key? key,
Widget? title,
Widget? message,
List<Widget>? actions,
ScrollController? messageScrollController,
ScrollController? actionScrollController,
Widget? cancelButton
})
Flutter showCupertinoModalPopup Constructor:
showCupertinoModalPopup<T>(
{required BuildContext context,
required WidgetBuilder builder,
ImageFilter? filter,
Color barrierColor = kCupertinoModalBarrierColor,
bool barrierDismissible = true,
bool useRootNavigator = true,
bool? semanticsDismissible,
RouteSettings? routeSettings}
)
Basic implementation of cupertino action sheet
CupertinoActionSheet(
title: Text('Select An Option'),
actions: <Widget>[
CupertinoActionSheetAction(
),
CupertinoActionSheetAction(
),
...
],
),
Flutter Cupertino Action Sheet Properties
The properties of flutter cupertino action sheet are:
- title
- message
- actions
- cancelButton
- messageScrollController
- actionScrollController
title
Use title property to show a title related to the options to the users. It is optional but the action sheet will be incomplete without a title. It takes a widget as value. Generally, a text widget as the title will be text.
showCupertinoModalPopup(
context: context,
builder: (BuildContext context) =>
CupertinoActionSheet(
title: Text('Select An Option'),
actions: <Widget>[
CupertinoActionSheetAction(
child: Text('One'),
onPressed: () {
Navigator.pop(context, 'One');
},
),
CupertinoActionSheetAction(
child: Text('Two'),
onPressed: () {
Navigator.pop(context, 'Two');
},
)
],
),
);
Output:
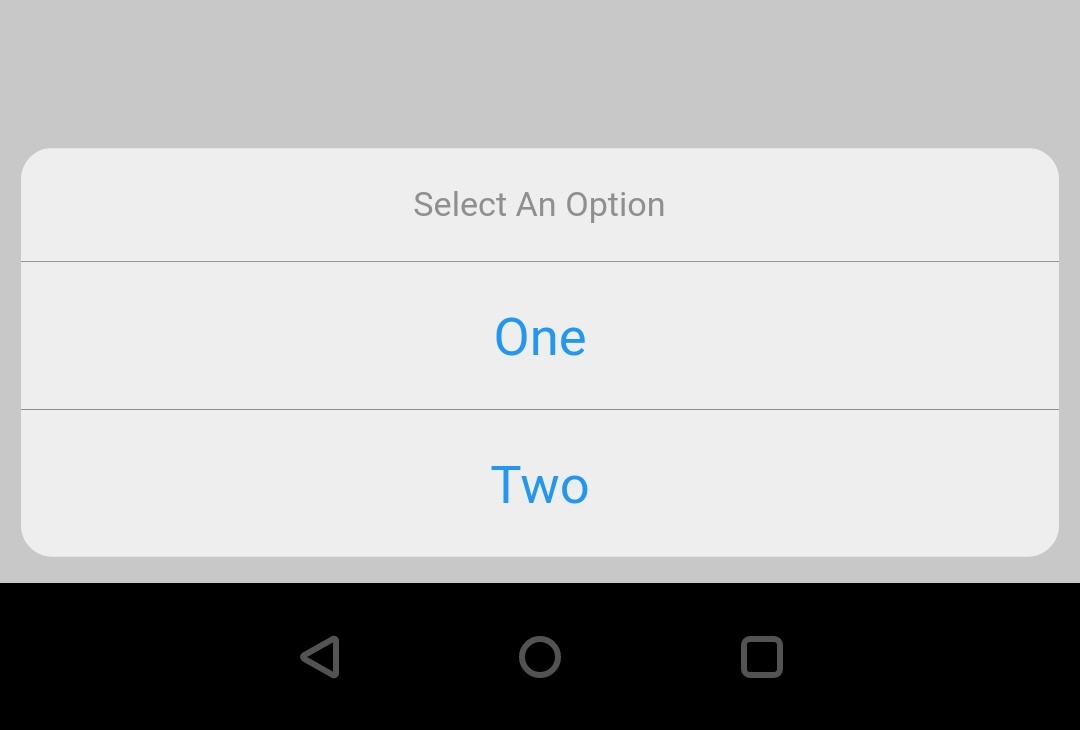
message
We will use this property to display a mesage to the user. Message will appear below the title. This property accepts a widget as value. Generally a text widget.
CupertinoActionSheet(
title: const Text('Select An Option'),
message: Text("This is message"),
actions: <Widget>[
CupertinoActionSheetAction(
child: Text('One'),
onPressed: () {
Navigator.pop(context, 'One');
},
),
CupertinoActionSheetAction(
child: Text('Two'),
onPressed: () {
Navigator.pop(context, 'Two');
},
)
],
),
Output:
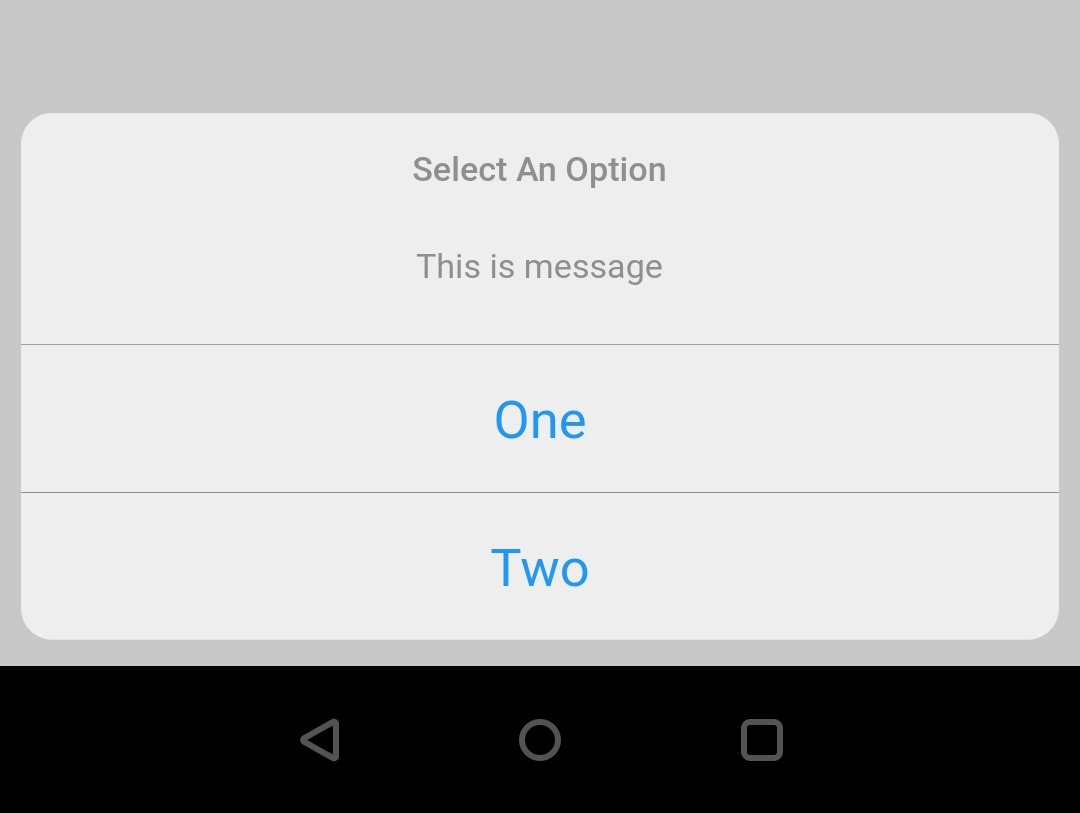
actions
The actions property takes a list of widgets as value. Generally we will use CupertinoActionSheetAction widget as children for the actions. Actions will appear below the message.
CupertinoActionSheet(
title: const Text('Select An Option'),
message: Text("This is message"),
actions: <Widget>[
CupertinoActionSheetAction(
child: Text('One'),
onPressed: () {
Navigator.pop(context, 'One');
},
),
CupertinoActionSheetAction(
child: Text('Two'),
onPressed: () {
Navigator.pop(context, 'Two');
},
)
],
),
cancelButton
We will use this property to display a cancel button which enables the user to dismiss the action sheet.
CupertinoActionSheet(
title: const Text('Select An Option'),
message: Text("This is message"),
actions: <Widget>[
CupertinoActionSheetAction(
child: const Text('One'),
onPressed: () {
Navigator.pop(context, 'One');
},
),
CupertinoActionSheetAction(
child: const Text('Two'),
onPressed: () {
Navigator.pop(context, 'Two');
},
)
],
cancelButton: CupertinoActionSheetAction(
child: const Text('Cancel'),
onPressed: () {
Navigator.pop(context, 'Cancel');
},
)
),
Output:
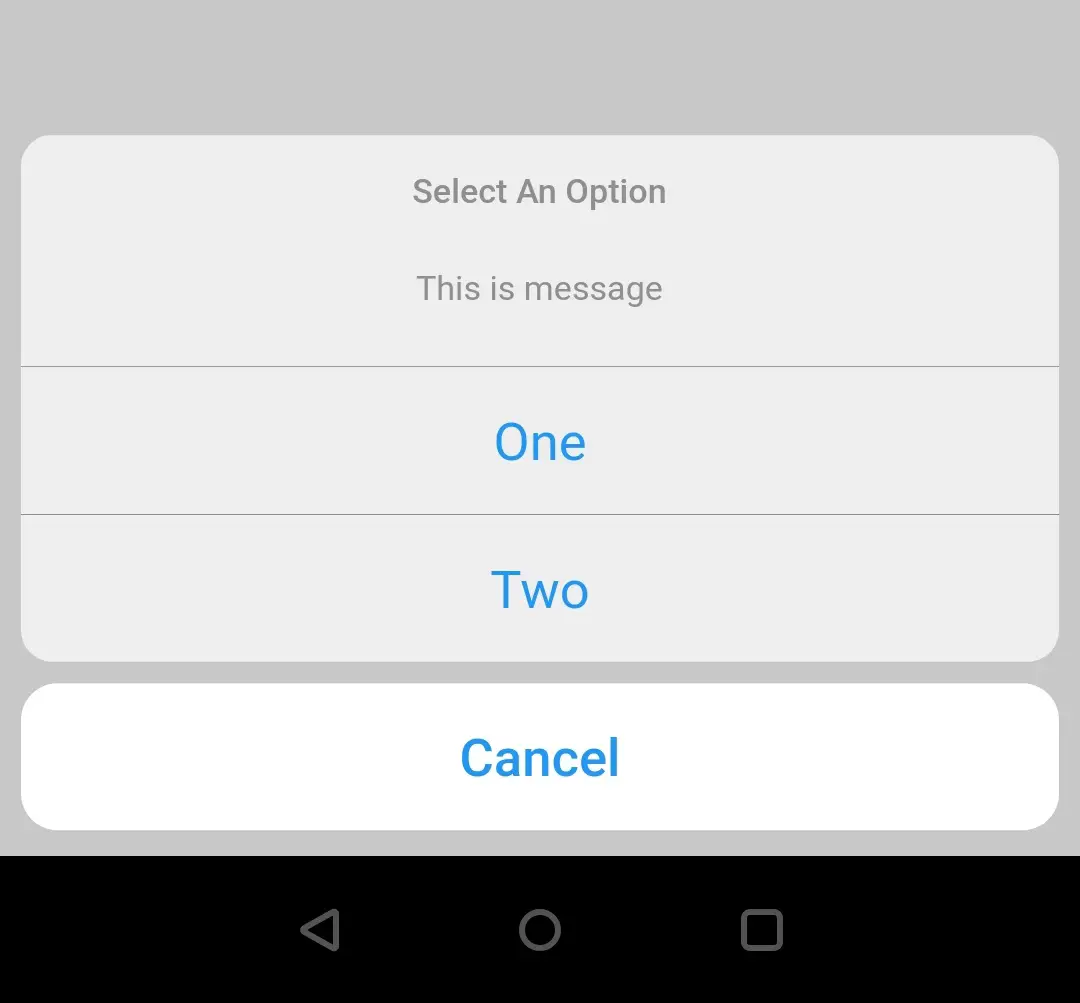
Flutter Cupertino Action Sheet Example
Lets create an example which demonstrates the use of cupertino action sheet in flutter. In this example we will display a delete button. Clicking this button will display a cupertino action sheet with two options delete for me, delete for everyone & cancel button like in whatsapp. Create a new flutter project and replace the code in main.dart file with the code below. The below code will display a button which will trigger the action sheet.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: Text("Flutter Cupertino Action Sheet"),
),
body: Container(
margin: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child: ElevatedButton(
child: Text("Delete"),
onPressed: () {
},
),
),
);
}
Now that we have created the button let’s create a function where we will call the showCupertinoModalPopup that will display the action sheet.
showActionsheet()
{
showCupertinoModalPopup(
context: context,
builder: (BuildContext context) =>
CupertinoActionSheet(
title: Text('Choose An Option'),
actions: <Widget>[
CupertinoActionSheetAction(
child: const Text('Delete For Me'),
onPressed: () {
Navigator.pop(context, 'Delete For Me');
},
),
CupertinoActionSheetAction(
child: const Text('Delete For Everyone'),
isDestructiveAction: true,
onPressed: () {
Navigator.pop(context, 'Delete For Everyone');
},
)
],
cancelButton: CupertinoActionSheetAction(
child: const Text('Cancel'),
onPressed: () {
Navigator.pop(context, 'Cancel');
},
)
),
);
}
If you don’t want the action sheet to dismiss when the user clicks outside the action sheet set barrierDismissible property of showCupertinoModalPopup function to true.
showCupertinoModalPopup(
context: context,
builder: (BuildContext context) =>
CupertinoActionSheet(
title: Text('Choose An Option'),
actions: <Widget>[
CupertinoActionSheetAction(
child: const Text('Delete For Me'),
onPressed: () {
Navigator.pop(context, 'Delete For Me');
},
),
CupertinoActionSheetAction(
child: const Text('Delete For Everyone'),
isDestructiveAction: true,
onPressed: () {
Navigator.pop(context, 'Delete For Everyone');
},
)
],
cancelButton: CupertinoActionSheetAction(
child: const Text('Cancel'),
onPressed: () {
Navigator.pop(context, 'Cancel');
},
),
),
barrierDismissible: true,
);
Now, let’s call the above function inside the onClick callback of the delete button we created at first.
body: Container(
margin: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child: ElevatedButton(
child: Text("Delete"),
onPressed: () {
showActionsheet();
},
),
),
Lets sum up all the code snippets above to complete the cupertino action sheet example. Create a new flutter project and replace the code of main.dart file with the code below and run the project to see the output.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter/services.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: Text("Flutter Cupertino Action Sheet"),
),
body: Container(
margin: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child: ElevatedButton(
child: Text("Delete"),
onPressed: () {
showActionsheet();
},
),
),
);
}
showActionsheet()
{
showCupertinoModalPopup(
context: context,
builder: (BuildContext context) =>
CupertinoActionSheet(
title: Text('Choose An Option'),
actions: <Widget>[
CupertinoActionSheetAction(
child: const Text('Delete For Me'),
onPressed: () {
Navigator.pop(context, 'Delete For Me');
},
),
CupertinoActionSheetAction(
child: const Text('Delete For Everyone'),
isDestructiveAction: true,
onPressed: () {
Navigator.pop(context, 'Delete For Everyone');
},
)
],
cancelButton: CupertinoActionSheetAction(
child: const Text('Cancel'),
onPressed: () {
Navigator.pop(context, 'Cancel');
},
),
),
barrierDismissible: true,
);
}
}
Output:
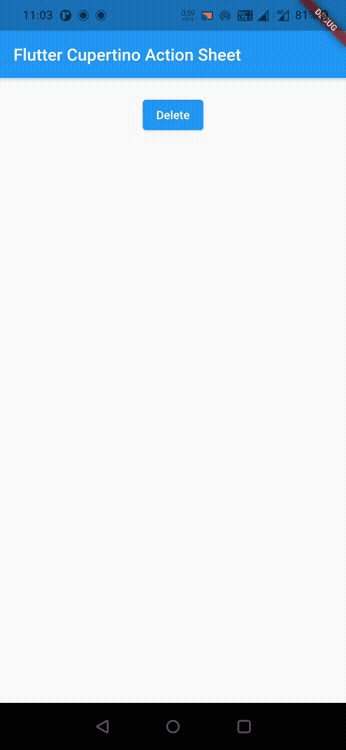
That’s all about the tutorial on how to use cupertino action sheet widget in flutter. We have also seen an example where we implemented the same. Let’s catch up with some other widget in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official Documentation.

Leave a Reply