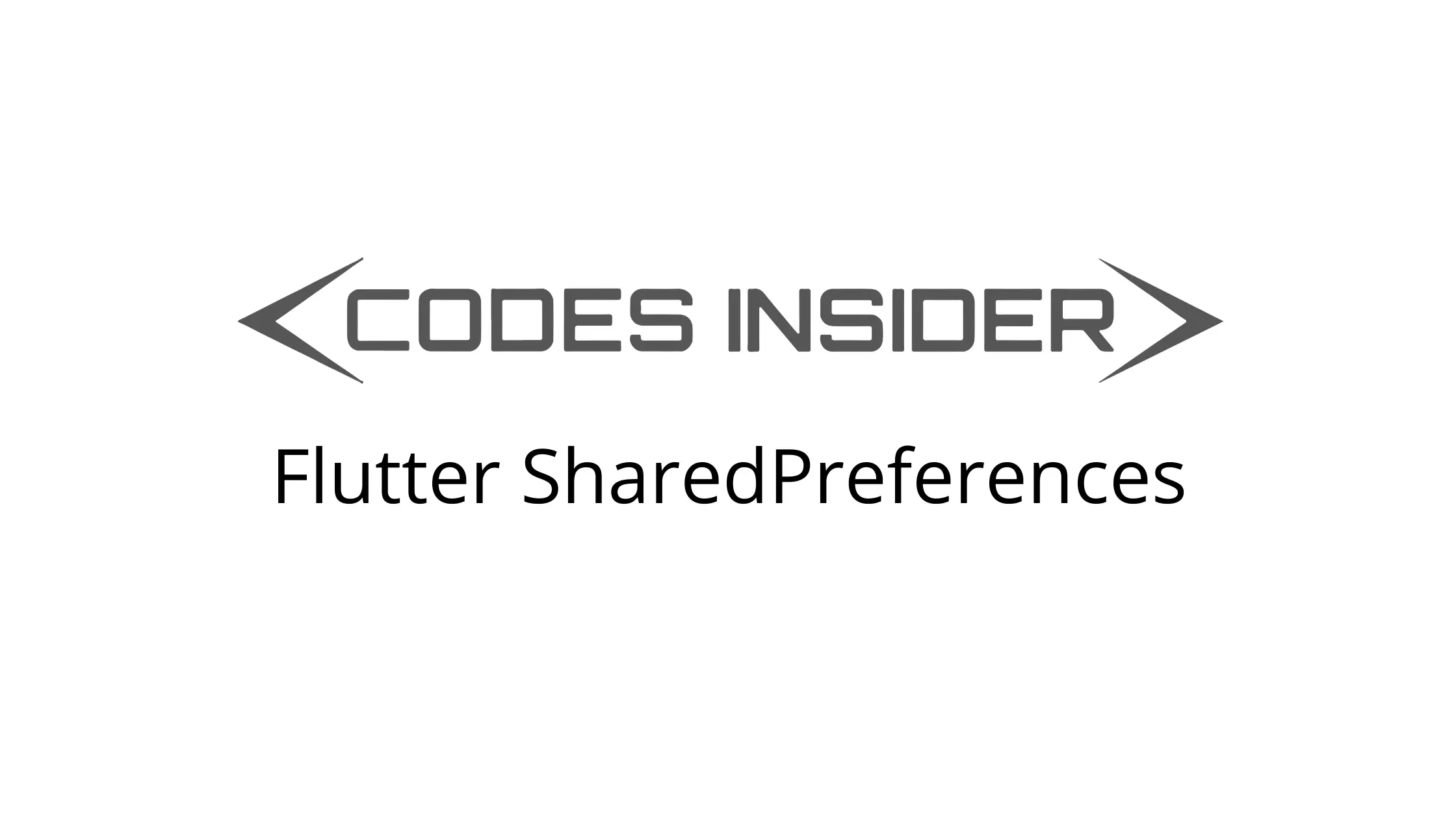
SharedPreferences in flutter are used to store data locally in key-value pairs in android and ios apps. We might have to save data locally while developing applications. Data like login credentials or a flag etc. Sharedpreferences comes in handy in such situations. For example, we have to save login credentials or a flag locally when the user logs in for the first time. This data will help the user access his account without logging in every time the app launches.
Flutter provides a plugin shared_preferences to implement sharedpreferences. It uses NSUserDefaults on ios and sharedpreferences in android. It provides persistent storage to save simple data. In this tutorial, we will learn how to implement and use sharedpreferences in flutter with example. We will also learn how to save, retrieve, and delete data locally using sharedpreferences.
Note: Don’t use sharedpreferences to save complex data. It is useful to save simple data.
To implement and use sharedpreferences in flutter follow the steps below:
- Create a new flutter project.
- Add shared_preferences plugin or dependency to the project.
- Import the shared prferences package :- import ‘package:shared_preferences/sharedpreferences.dart’;
- Implement the code as per your requirement like saving, retreiving, deleting, etc.
Let’s add shared_preferences plugin or dependency to pubspec.yaml file present in the root folder of our project.
dependencies:
shared_preferences: ^2.0.8
Import the package to use its functionality in our project.
import 'package:shared_preferences/shared_preferences.dart';
We are done adding the dependency and importing the package. Now we will see how to save, retrieve and delete data in sharedpreferences.
To save data in sharedpreferences in flutter declare an object of SharedPreferences class. Initialize the object and call the setter methods to save data. Using sharedpreferences we can save five types of values int, string, double, bool, and stringList. So we have five setter methods for saving these five types of data. We have to provide each setter method with a key and a value. The key must be a string for all the methods. The values will change based on the method.
The setter methods of sharedpreferences class are:
- setString()
- setInt()
- setDouble()
- setBool()
- setStringList()
First, declare the object.
SharedPreferences prefs;
Initialize the object.
getSharedPreferences () async
{
prefs = await SharedPreferences.getInstance();
}
Now we will use the above object to call the setter methods and save data.
To save string value in sharedpreferences call the setString() method and provide the key and value.
saveStringValue () async
{
prefs = await SharedPreferences.getInstance();
prefs.setString("username", "naresh");
}
The above code snippet will add naresh as a value to the key username.
To save int value in sharedpreferences we will use setInt() method. Call the method and provide the key and value.
saveIntValue () async
{
prefs = await SharedPreferences.getInstance();
prefs.setInt("status", 1);
}
The above code snippet will add 1 as a value to the key status.
Call the setter method setDouble() and provide key and value to save a double value in sharedpreferences.
saveDoubleValue () async
{
prefs = await SharedPreferences.getInstance();
prefs.setDouble("balance", 20.5);
}
The above code snippet will add 20.5 as a value to the key balance.
To save the boolean value in sharedpreferences call the setBool() method and provide the key and value.
saveBoolValue () async
{
prefs = await SharedPreferences.getInstance();
prefs.setBool("loggedIn", true);
}
The above code snippet will add true as a value to the key loggedIn.
To save a string list value in sharedpreferences call the setStringList() method and provide the key and value.
saveStringListValue () async
{
prefs = await SharedPreferences.getInstance();
prefs.setStringList("foodList", ["pizza", "burger", "sandwich"]);
}
The above code snippet will add list( [“pizza”, “burger”, “sandwich”] ) as a value to the key foodList.
To retrieve or read data from sharedpreferences in flutter we have to use getter methods of sharedpreferences class. There are five getter methods in sharedpreferences class. We have to provide the getter method with only the key which is a string. The method will return the value of that corresponding key.
The getter methods of sharedpreferences class are:
- getSring()
- getInt()
- getDouble()
- getBool()
- getStringList()
While retrieving or reading data from shared preferences we might get null as a value. If the value is not present for the provided key or if we provide a wrong key it will return null. It will throw an error. We can solve this problem by using ‘if null‘ (??). If the previous value is null it will return the value provided next to it. Let’s see the implementation below.
prefs = await SharedPreferences.getInstance();
int users = prefs.getInt("count") ?? 0;
If prefs.getInt(“count”) returns a non null value it will be assigned to users variable. If it returns null as a value then ‘ 0 ‘ will be assigned to users variable. The prefs.getInt(“count”) will return null if no value is found for the key ‘count’ or if the key itself doesn’t exist.
To retrieve or read string value from sharedpreferences call getString() method and provide the key.
retrieveStringValue () async
{
prefs = await SharedPreferences.getInstance();
String value = prefs.getString("username");
print(value);
}
Output: naresh
To retrieve or read int value from sharedpreferences call getInt() method and provide the key.
retrieveIntValue () async
{
prefs = await SharedPreferences.getInstance();
int value = prefs.getInt("status");
print(value);
}
Output: 1
To retrieve or read double value from sharedpreferences call getDouble() method and provide the key.
retrieveDoubleValue () async
{
prefs = await SharedPreferences.getInstance();
double value = prefs.getDouble("balance");
print(value);
}
Output: 20.5
To retrieve or read boolean value from sharedpreferences call getBool() method and provide the key.
retrieveBooleanValue () async
{
prefs = await SharedPreferences.getInstance();
bool value = prefs.getBool("loggedIn");
print(value);
}
Output: true
To retrieve or read string list value from sharedpreferences call getStringList() method and provide the key.
retrieveStringListValue () async
{
prefs = await SharedPreferences.getInstance();
List<String> foodList = prefs.getStringList("foodList");
print(value);
}
Output: [“pizza”, “burger”, “sandwich”]
To delete or remove data from sharedpreferences in flutter we have to use remove() method. We have to provide only the key to delete the record (both the key and the value corresponding to that key). This method can delete all four types of data by simply taking the key.
deleteValue () async
{
prefs = await SharedPreferences.getInstance();
prefs.remove("username");
}
To check if a key or record is present in shared preferences we have to use containsKey() method. This methods takes key as value and checks if the key is present in sharedpreferences. If the key is present it will return true else it will return false.
checkKey () async
{
prefs = await SharedPreferences.getInstance();
bool hasKey = prefs.containsKey("username");
print(hasKey);
}
Output: true
checkKey () async
{
prefs = await SharedPreferences.getInstance();
bool hasKey = prefs.containsKey("counter");
print(hasKey);
}
Output: false
Lets create an example where we will implement sharedpreferences in flutter. In this example we will display a textfield and a text widget. Below the text widget we will display three buttons save, retrieve and delete. We will save retrieve and delete the data entered in the textfield using sharedpreferences. When we press the save button we will save the date entered in the text field. When we press retrieve button we will retrieve the data from shared preferences and display it in the text widget. Delete button will clear the data in sharedpreferences.
First, let’s declare the variable for holding the text entered in the textfield and textediting controller. Also declare an object for SharedPreferences.
SharedPreferences prefs;
TextEditingController controller = new TextEditingController();
String name="";
Now implement the code for displaying the textfield, text widget and three buttons. Call the respective functions inside onPressed callback of each button.
body:Container(
margin: EdgeInsets.all(20),
child: Column(
children: [
TextField(
controller: controller,
decoration: InputDecoration(
border: OutlineInputBorder(),
),
),
SizedBox(
height:30,
),
Text(name, style: TextStyle(fontSize: 20),),
SizedBox(
height:30,
),
ElevatedButton(
child:Text("Save"),
onPressed: ()
{
save();
},
),
ElevatedButton(
child:Text("retrieve"),
onPressed: ()
{
retrieve();
},
),
ElevatedButton(
child:Text("Delete"),
onPressed: ()
{
delete();
},
)
],
)
),
Create three functions save, retrieve and delete.
save() async
{
prefs = await SharedPreferences.getInstance();
prefs.setString("username", controller.text.toString());
}
retrieve() async{
prefs = await SharedPreferences.getInstance();
name = prefs.getString("username");
setState(() {
});
}
delete() async
{
prefs = await SharedPreferences.getInstance();
prefs.remove("username");
name = "";
setState(() {
});
}
Complete Code
Lets sum up the above code snippets to complete our sharedpreferences example in flutter. Create a new flutter project and replace the code in main.dart file with the code below.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:shared_preferences/shared_preferences.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
SharedPreferences prefs;
TextEditingController controller = new TextEditingController();
String name="";
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: Text("Flutter SharedPreferences"),
),
body:Container(
margin: EdgeInsets.all(20),
child: Column(
children: [
TextField(
controller: controller,
decoration: InputDecoration(
border: OutlineInputBorder(),
),
),
SizedBox(
height:30,
),
Text(name, style: TextStyle(fontSize: 20),),
SizedBox(
height:30,
),
ElevatedButton(
child:Text("Save"),
onPressed: ()
{
save();
},
),
ElevatedButton(
child:Text("retrieve"),
onPressed: ()
{
retrieve();
},
),
ElevatedButton(
child:Text("Delete"),
onPressed: ()
{
delete();
},
)
],
)
),
);
}
save() async
{
prefs = await SharedPreferences.getInstance();
prefs.setString("username", controller.text.toString());
}
retrieve() async{
prefs = await SharedPreferences.getInstance();
name = prefs.getString("username");
setState(() {
});
}
delete() async
{
prefs = await SharedPreferences.getInstance();
prefs.remove("username");
name = "";
setState(() {
});
}
}
Since the data is saved locally in sharedpreferences it will not be deleted even if we killl the app. If we relaunch the app we can retrieve the data again.
Output:
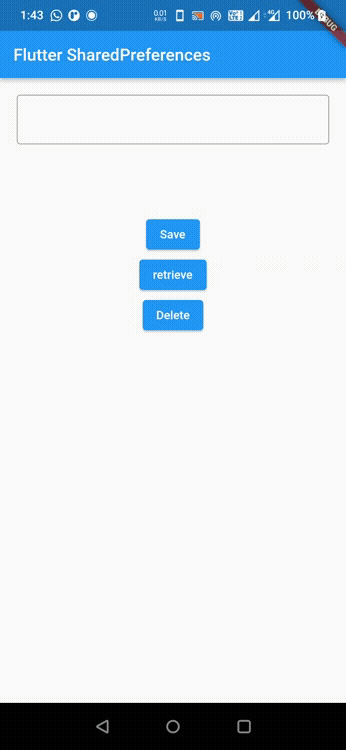
Thats all about the tutorial on how to implement & use sharedpreferences in flutter. We have also seen an example where we have used shared_preferences plugin to save and retrieve data. Let’s catch up with some other widgets in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official Documentation.

Great content! Keep up the good work!
Thank you.
your example is very super , its very use full bro..thank
Thank you bro
Thank u so much, it helps me alot to this article.
Thank you