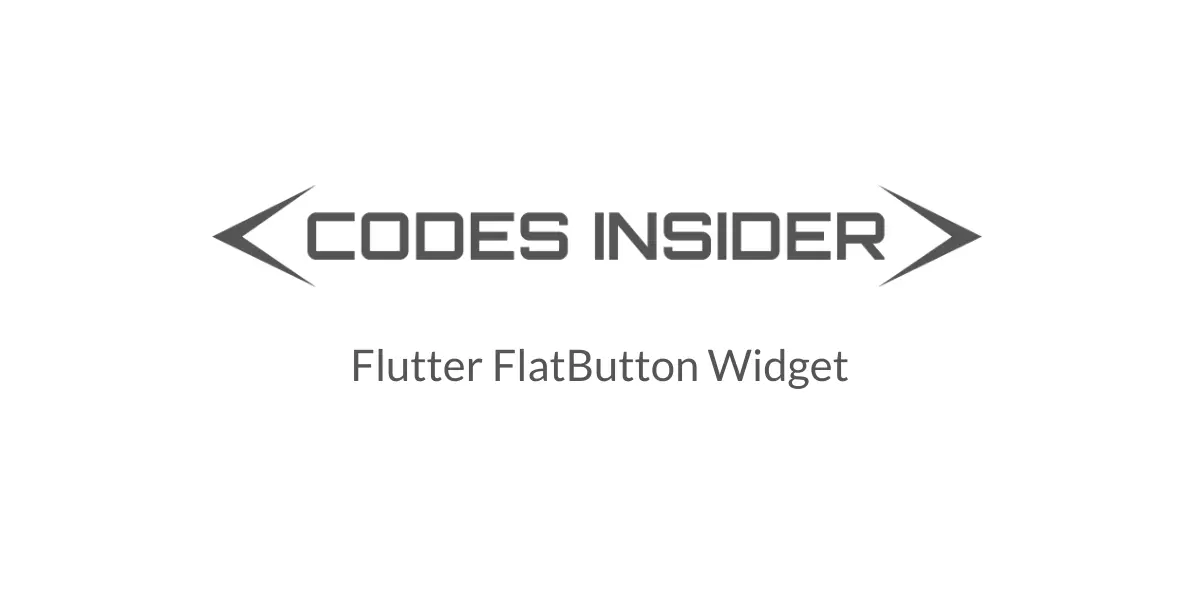
Flutter FlatButton Widget
The FlatButton widget in flutter is used to display a simple button. It is one of the most used widgets in flutter. The button when pressed will perform some user-defined action. Flat button is just a text button because it has no style and border. It has no elevation, button color, and text color like an elevated button. The text color is black with no background by default. If you want to use other buttons consider reading different types of buttons in flutter.
In this tutorial, you will learn how to create and use flatbutton widget in flutter with example. We will also customize the button with different properties.
Basic FlatButton
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
onPressed: () {
},
)
)
Output:
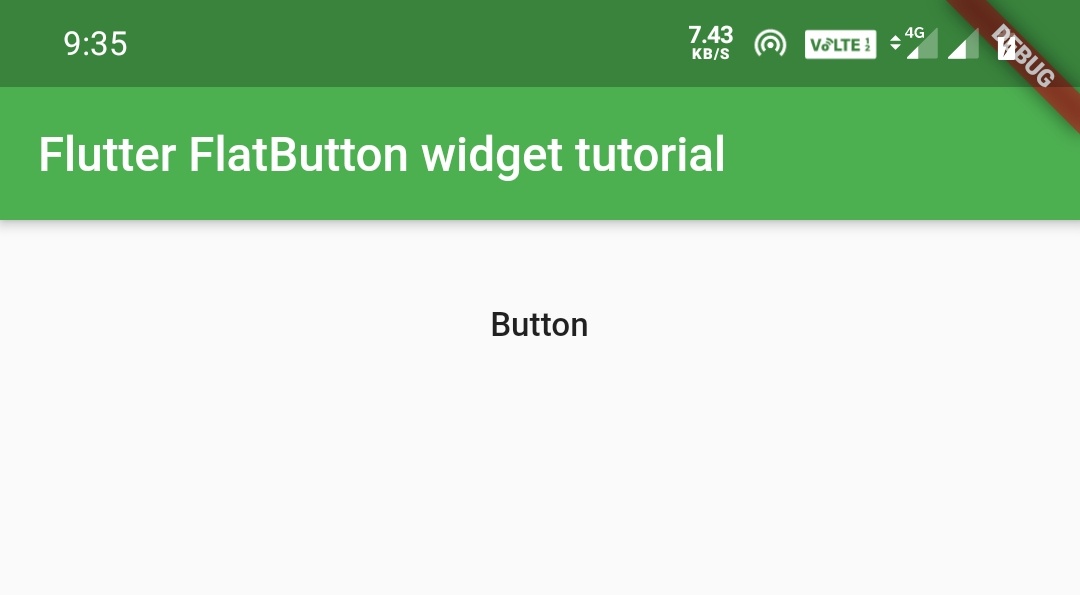
FlatButton Widget Properties
Flat button has many properties which help in styling the button. By using those properties we can change colors, background, fontsize etc,.Let’s see the constructor of FlatButton.
FlatButton({
Key key,
@required VoidCallback onPressed,
VoidCallback onLongPress,
ValueChanged<bool> onHighlightChanged,
MouseCursor mouseCursor,
ButtonTextTheme textTheme,
Color textColor,
Color disabledTextColor,
Color color,
Color disabledColor,
Color focusColor,
Color hoverColor,
Color highlightColor,
Color splashColor,
Brightness colorBrightness,
EdgeInsetsGeometry padding,
VisualDensity visualDensity,
ShapeBorder shape,
Clip clipBehavior: Clip.none,
FocusNode focusNode,
bool autofocus: false,
MaterialTapTargetSize materialTapTargetSize,
@required Widget child
})
Handling Callbacks of FlatButton
FlatButton – onPressed
When the user taps the button this gets called. It is a VoidCallback method which means no arguments and return values. We need to pass a method with no arguments. The button will be disabled if we don’t provide onPressed: property or if we set onPressed: to null.
Eg: void testFunction();
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
onPressed: () {
//your code
},
)
)
or we can use a function name inside the anonymous method of onPressed callback.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
onPressed: () {
//your method name without paranthesis
eg: testFunction;
},
)
)
FlatButton – onLongPress
This callback is invoked when the user long press the button.It works similar to onPressed.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
onLongPress: () {
//you can implement code here or use a function name without parnthesis similar to onPressed.
},
)
)
FlatButton – onHighlightChanged
It gets called when the button is pressed/down or released/up. It will return true when the button is pressed and false when released.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
onHighlightChanged: (value) {
print(value);
},
onPressed: () {
},
)
)
Styling the FlatButton widget
FlatButton – textTheme
To change text color based on theme we will use textTheme property. This property takes ButtonTextTheme which has three constants like normal, accent and primary.
ButtonTextTheme.primary
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
textTheme: ButtonTextTheme.primary,
onPressed: () {
},
)
)
Output:
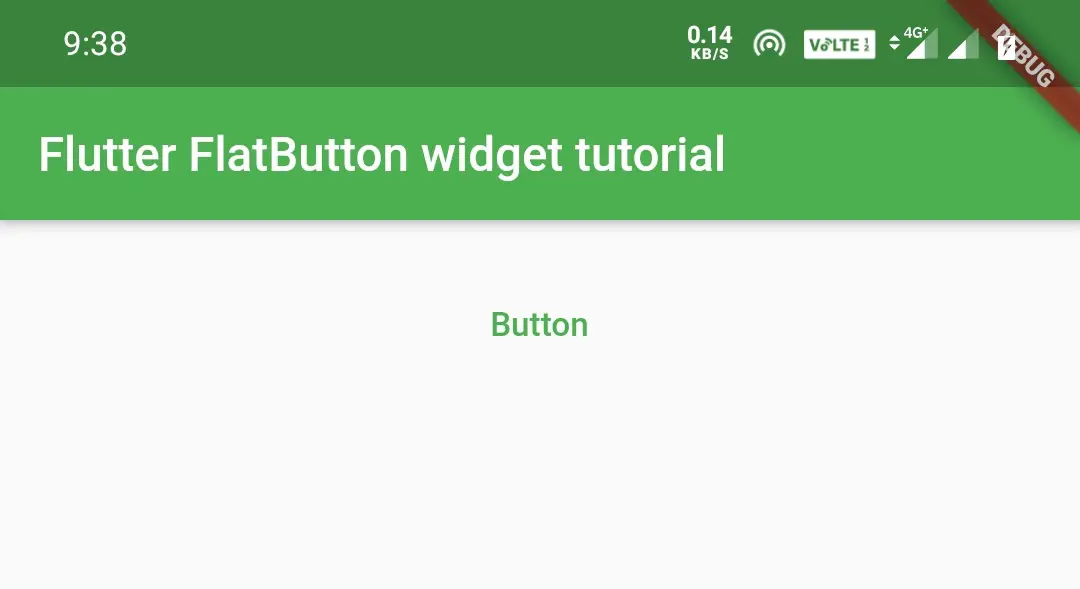
FlatButton – textColor
The textColor property is used to change the color of button text.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
textColor: Colors.deepOrange,
onPressed: () {
},
)
)
Output:
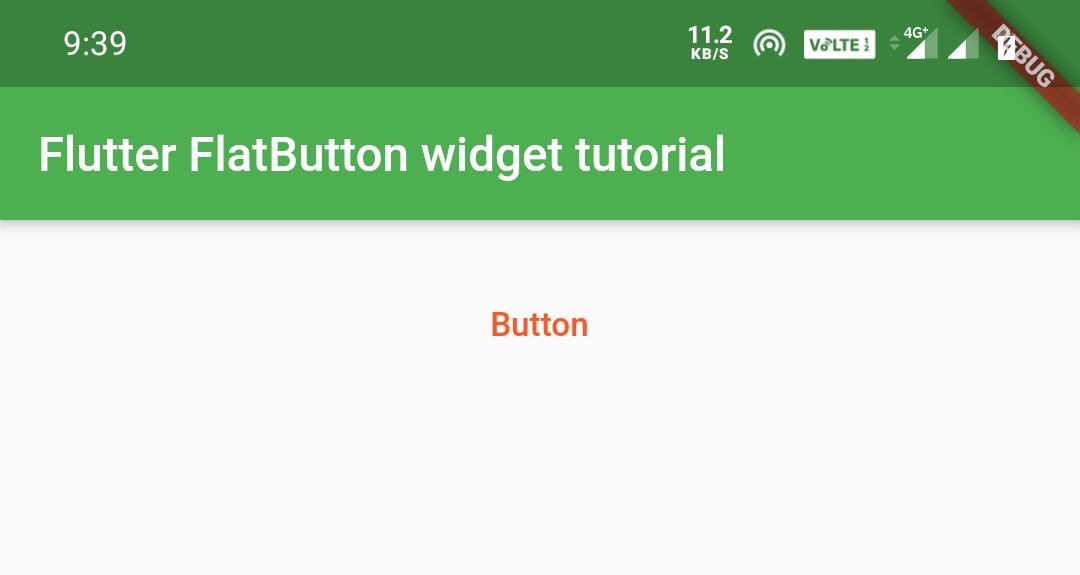
FlatButton – disabledTextColor
The disabledTextColor property is used to set text color to be displayed, when the button is disabled. In this example i am setting onPressed to null so that we can see the output.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
disabledTextColor: Colors.orangeAccent,
textColor: Colors.deepOrange,
onPressed: null,
)
)
Output:
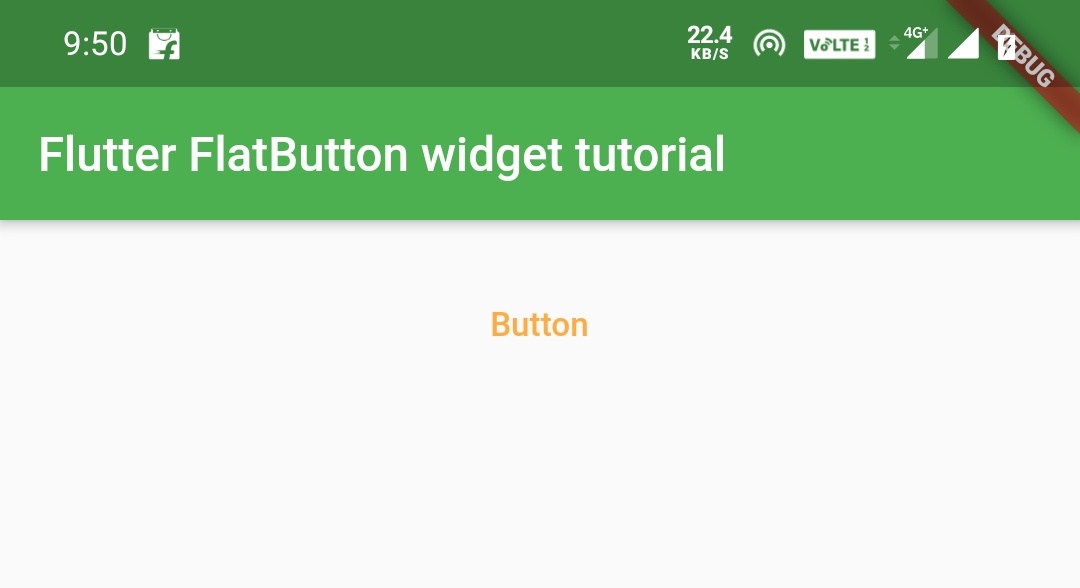
FlatButton – color
This property is used to set background color for the button.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
textColor: Colors.white,
color:Colors.green,
onPressed: (){},
)
)
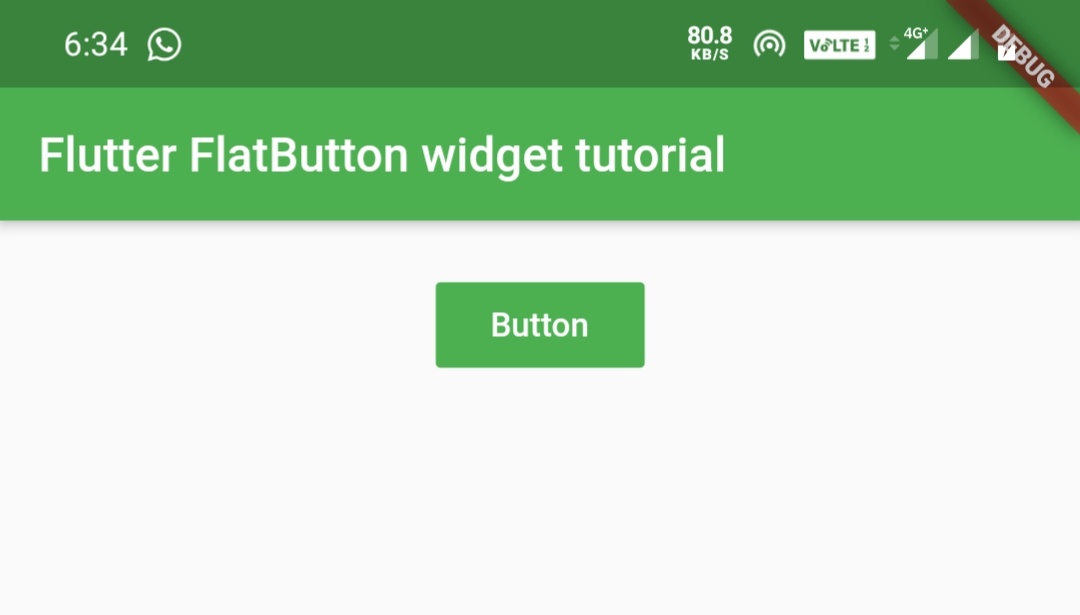
FlatButton – highlightColor
When the user taps the button, highlightColor will be displayed over the entire button and fades out when the user releases the button.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
highlightColor: Colors.green,
textColor: Colors.deepOrange,
onPressed: (){},
)
)
FlatButton – splashColor
When the user taps the button the splashColor starts filling the button slowly. It fades out when the user releases the button.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
splashColor: Colors.green,
textColor: Colors.deepOrange,
onPressed: (){},
)
)
FlatButton – colorBrightness
The colorBrightness property increases the readability of button text. This property takes Brightness which has two constants light and dark. Its better to use Brightness.dark for dark backgrounds and Brightness.light for light background.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
color: Colors.black,
colorBrightness: Brightness.dark,
textColor: Colors.white,
onPressed: (){},
)
)
FlatButton – Padding
The padding property provides padding to the button,which means internal spacing. It takes EdgeInsets which has three methods all, only and symmetric.
EdgeInsets.all()
It takes one value and sets that amount of padding for all the sides.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
color: Colors.black,
padding: EdgeInsets.all(20),
colorBrightness: Brightness.dark,
textColor: Colors.white,
onPressed: (){},
)
)
Output:
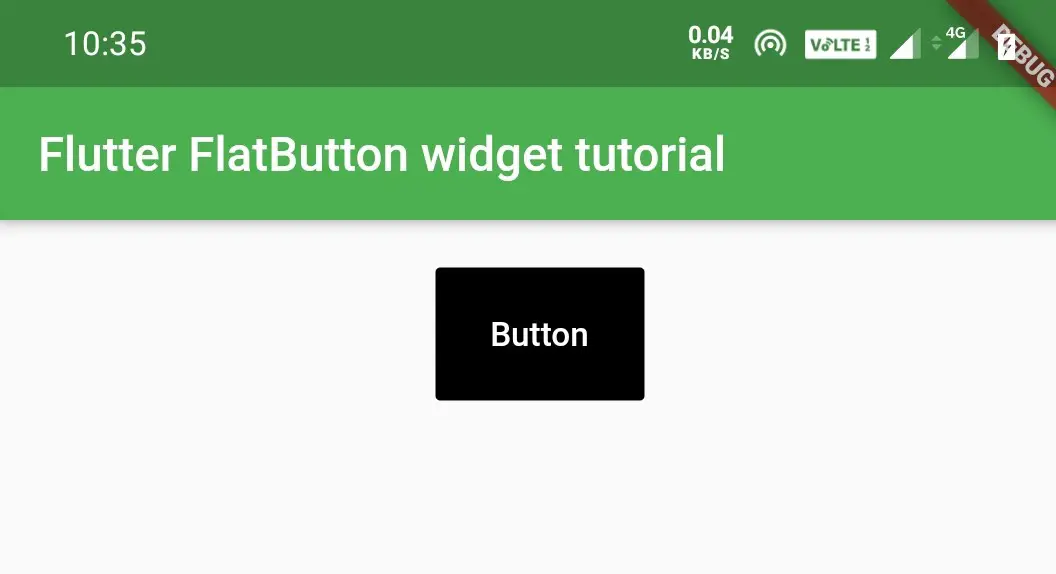
EdgeInsets.only()
You can specify up to 4 sides, which means you can define any number of sides you want below four.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
color: Colors.black,
padding: EdgeInsets.only(
left: 20,
right: 20,
top: 30
),
colorBrightness: Brightness.dark,
textColor: Colors.white,
onPressed: (){},
)
)
Output:
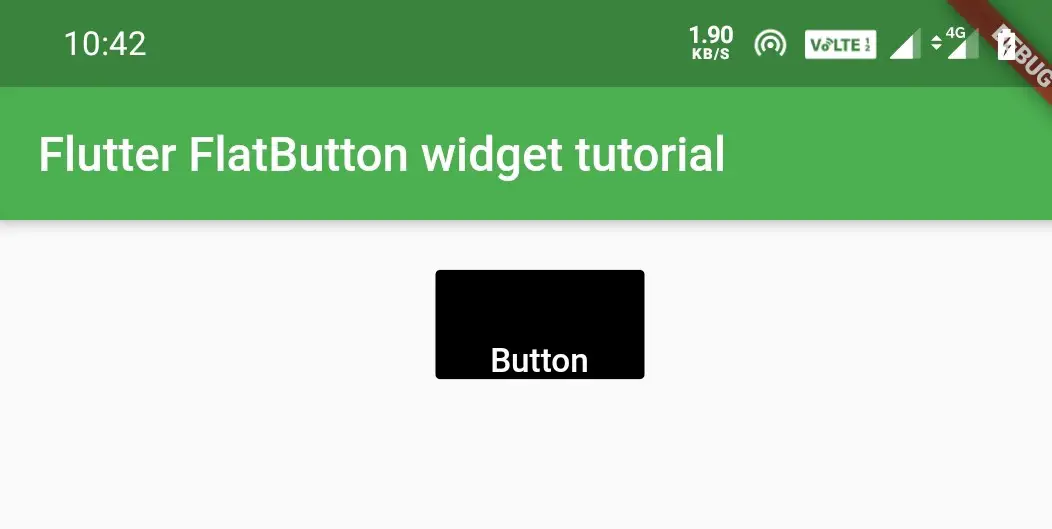
EdgeInsets.symmetric()
This accepts horizontal and vertical values.You can provide only horizontal, only vertical or both.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
color: Colors.black,
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 10
),
colorBrightness: Brightness.dark,
textColor: Colors.white,
onPressed: (){},
)
)
Output:
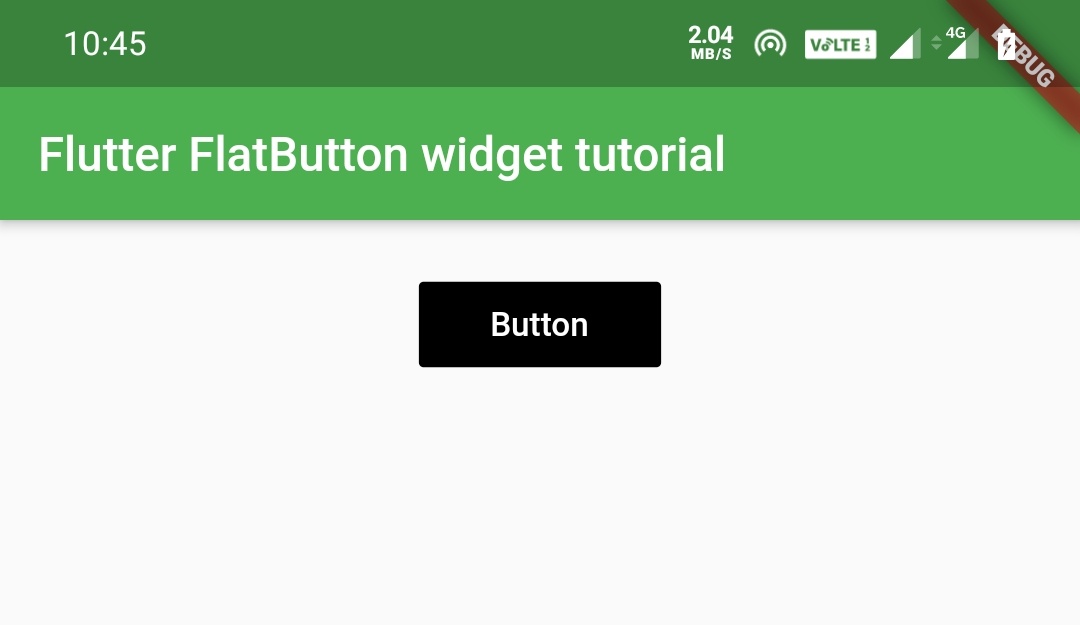
Shaping FlatButton
FlatButton – shape
By using shape property we can apply different shapes to the button. We can apply shapes like rectangular , rounded rectangle, circular, etc,.
FlatButton – Rectangular Border
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 10
),
shape:Border.all(width: 2,color: Colors.green),
textColor: Colors.green,
onPressed: (){},
)
)
Output:
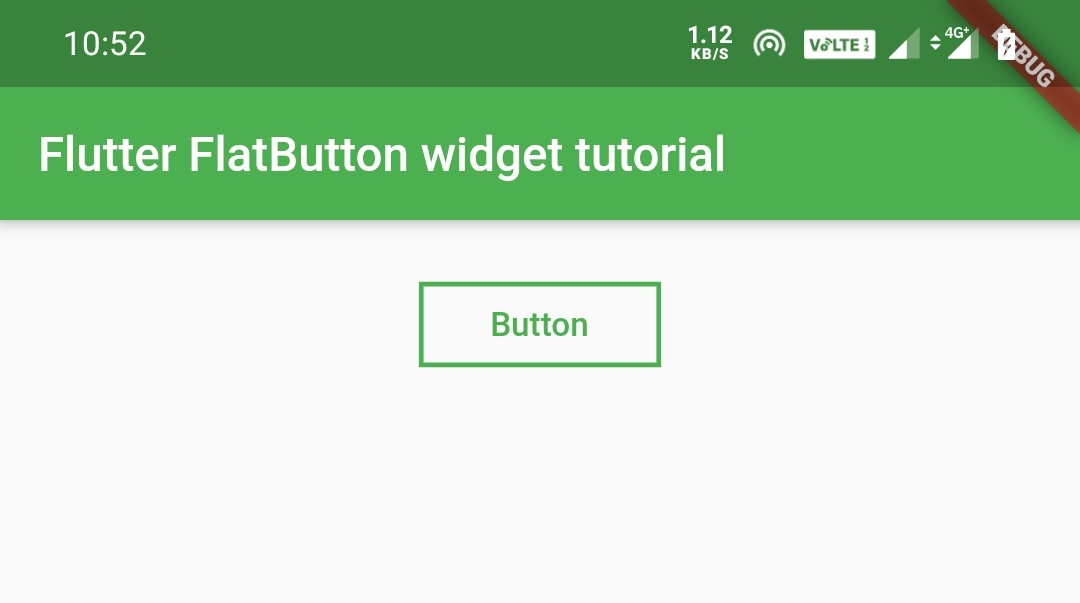
FlatButton – OutlineInputBorder
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 10
),
shape: OutlineInputBorder(),
textColor: Colors.black,
onPressed: (){},
)
)
Output:
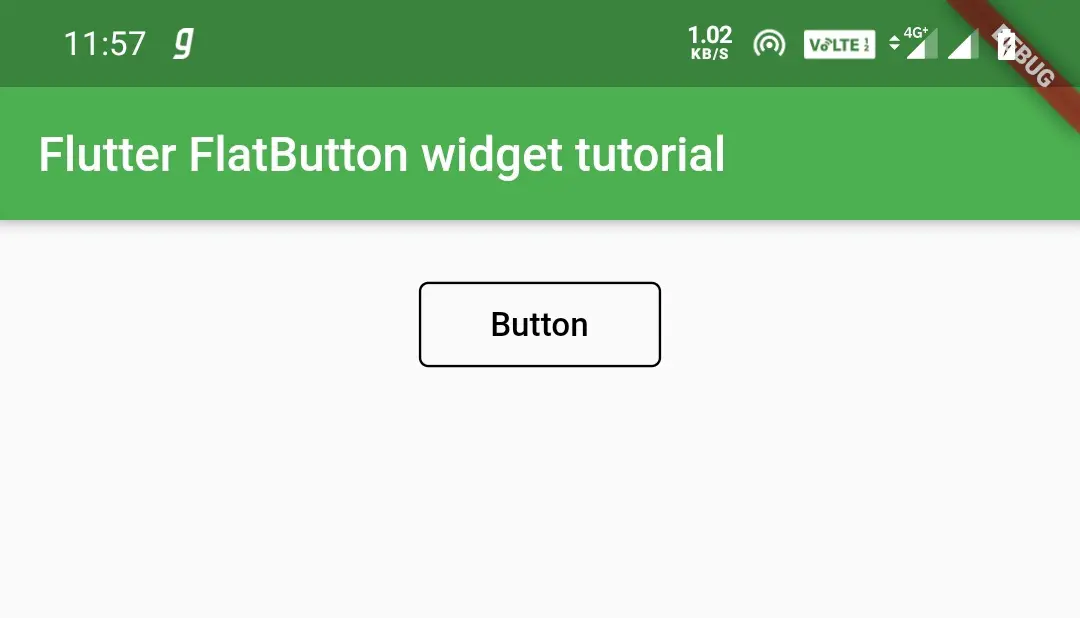
Using borderSide for styling border.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 10
),
shape: OutlineInputBorder(
borderSide: BorderSide(
color: Colors.green,
width: 3,
style: BorderStyle.solid
)
),
textColor: Colors.green,
onPressed: (){},
)
)
Output:
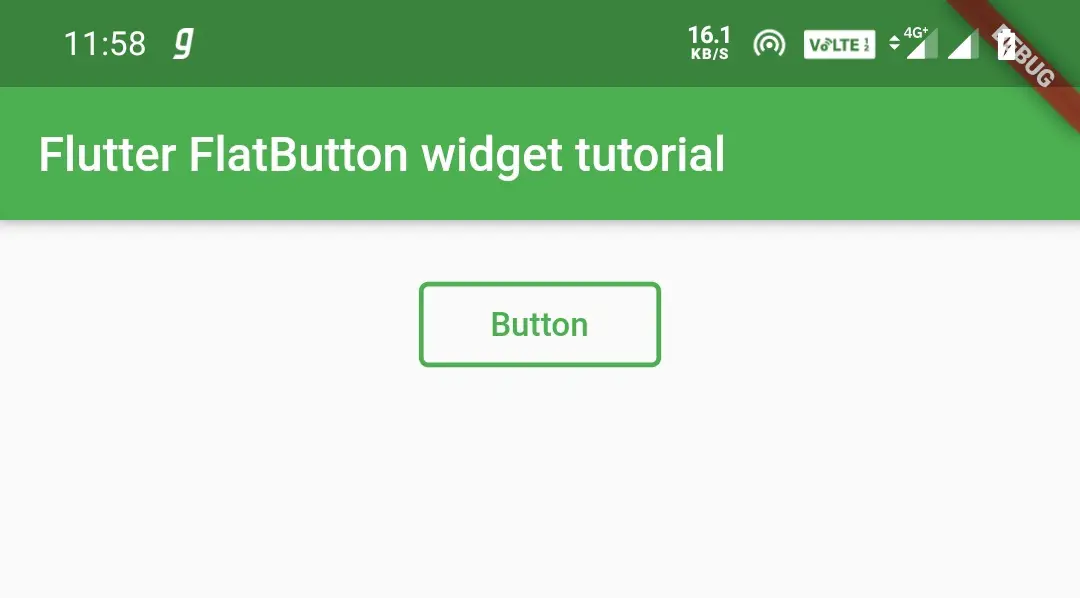
FlatButton – UnderlineInputBorder
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 10
),
shape: UnderlineInputBorder(
borderSide: BorderSide(
color: Colors.green,
width: 3,
style: BorderStyle.solid
)
),
textColor: Colors.green,
color: Colors.black,
onPressed: (){},
)
)
Output:
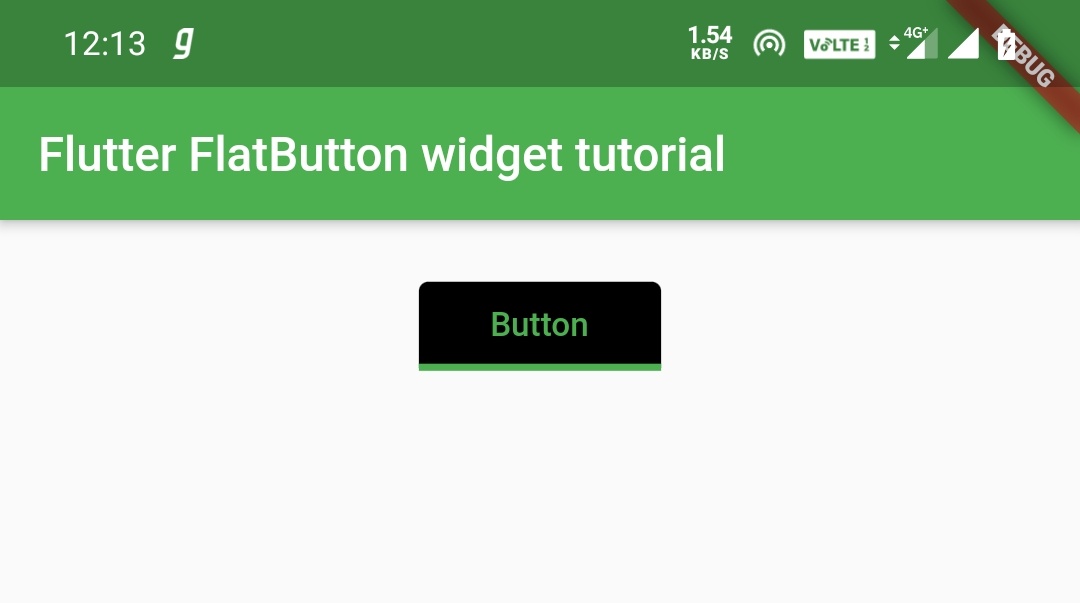
We can remove the arguments for UnderlineInputBorder. If we remove the arguments, it simply displays black border by default.
FlatButton – StadiumBorder
It directly forms the shape with rounded edges without providing any BorderRadius.
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 10
),
shape: StadiumBorder(
side: BorderSide(
color: Colors.green,
width: 2
)
),
textColor: Colors.green,
onPressed: (){},
)
)
Output:
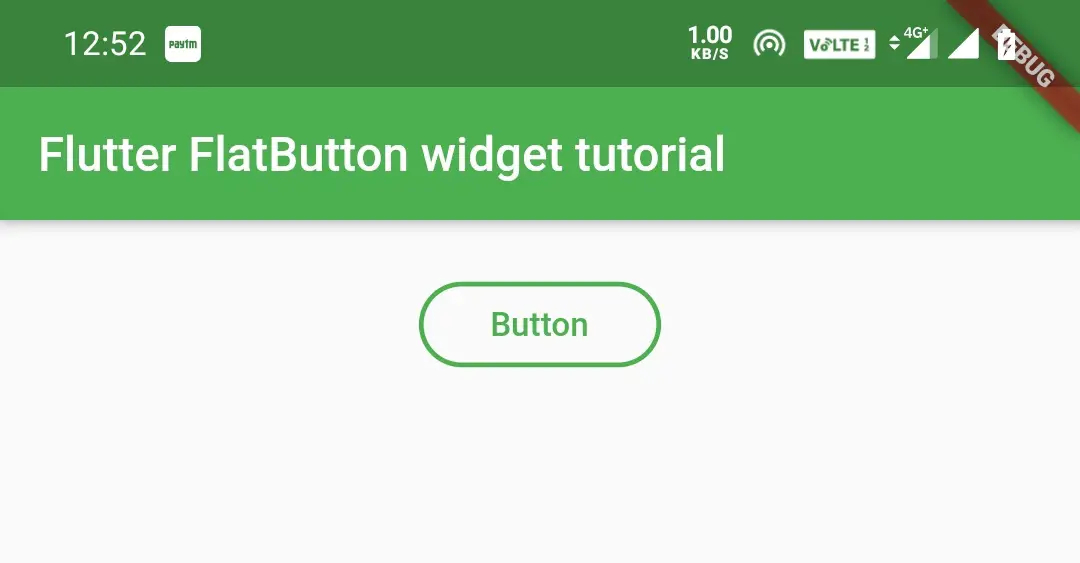
FlatButton – RoundedRectangleBorder
It looks similar to StadiumBorder, but we have to provide border radius to get rounded edges.
FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 10
),
shape: RoundedRectangleBorder(
side: BorderSide(
color: Colors.green,
width: 2
),
borderRadius: BorderRadius.circular(20)
),
textColor: Colors.green,
onPressed: (){},
)
)
Output:
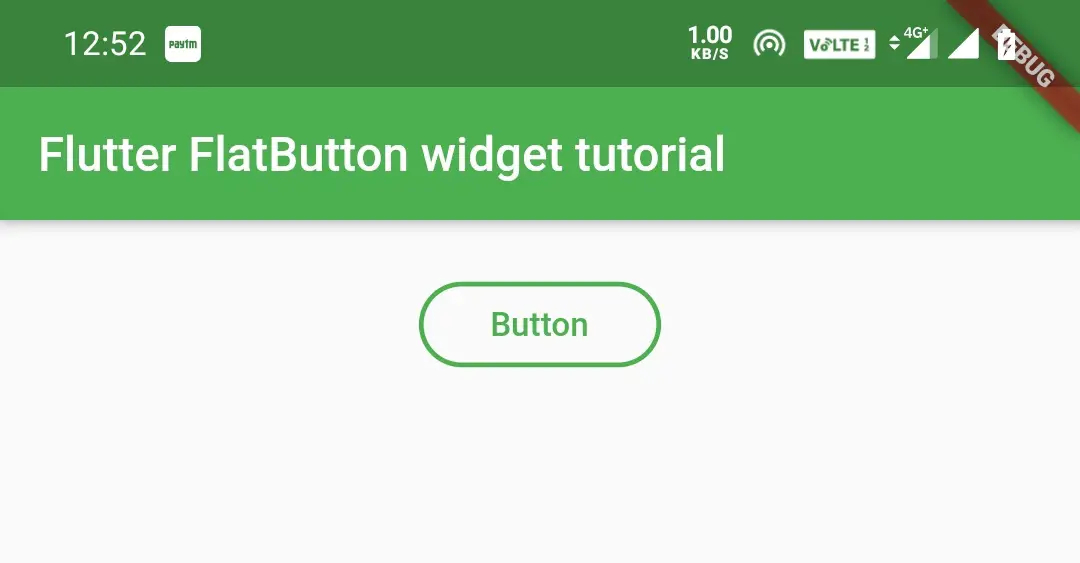
FlatButton – CircleBorder
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 30
),
shape: CircleBorder(
side: BorderSide(
color: Colors.green,
width: 2
)
),
textColor: Colors.green,
onPressed: (){},
)
)
Output:
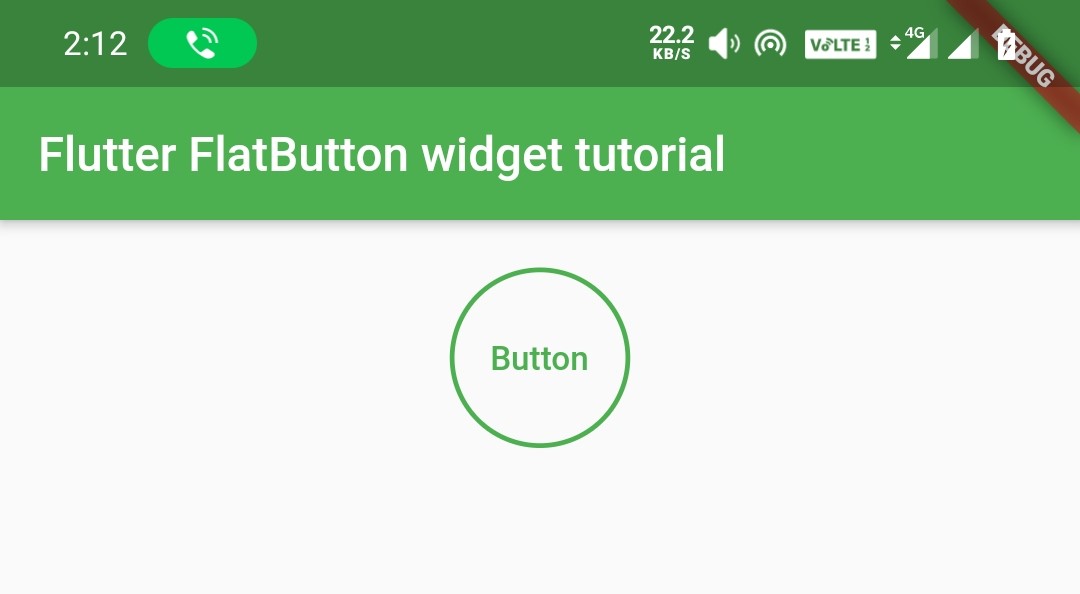
FlatButton – BeveledRectangleBorder
Container(
width:double.infinity ,
padding: EdgeInsets.all(20),
alignment: Alignment.topCenter,
child:FlatButton(
child:Text("Button"),
padding: EdgeInsets.symmetric(
horizontal: 30,
vertical: 20
),
shape: BeveledRectangleBorder(
side: BorderSide(
color: Colors.green,
width: 2
),
borderRadius: BorderRadius.circular(15)
),
textColor: Colors.green,
onPressed: (){},
)
)
Output:
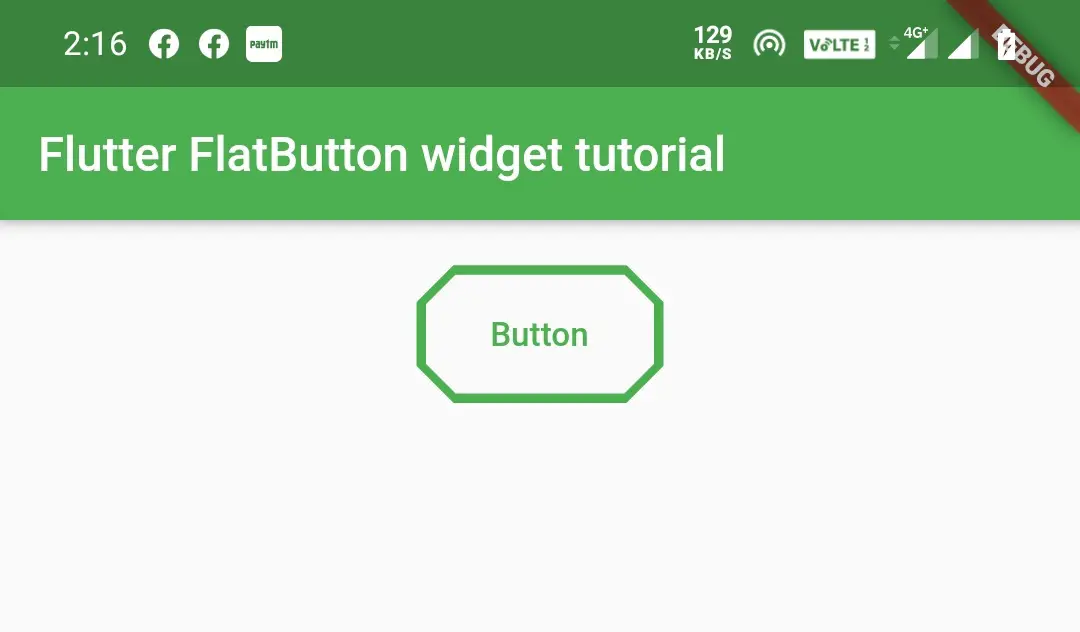
That all about flutter flatbutton widget tutorial.I’ll see you again with some other flutter tutorial.Have a great day!!
Do like and share if you find this post helpful.Thank you!!
Reference: Flutter Official Documentation.

Leave a Reply