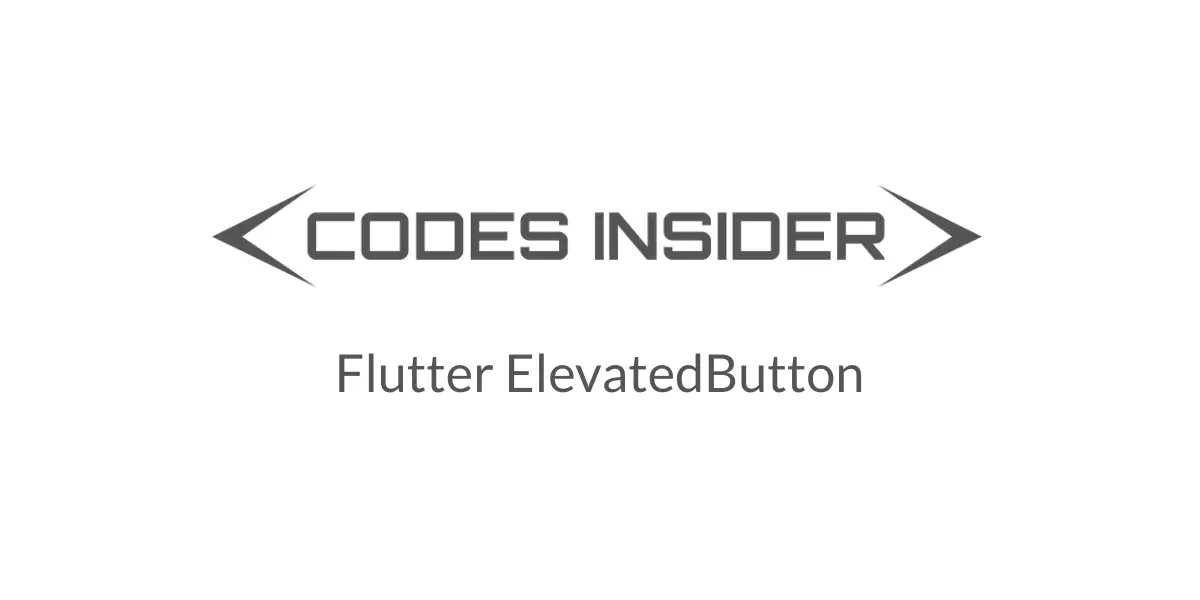
In flutter, we will use ElevatedButton widget to display a simple button. It is the replaced version of RaisedButton widget as the RaisedButton widget will deprecate soon. It is one of the most used widgets in flutter. In this example tutorial, we will learn how to use an ElevatedButton widget in flutter and its properties in detail.
Note: Elevated Button widget will be available in flutter 1.22.0 or above. Also update your flutter plugin.
Flutter ElevatedButton
An elevatedbutton is a material widget in flutter which is elevated by default. When we press the elevated button its elevation will increase. We can also display an elevated button with an icon and label using ElevatedButton.icon. It is advised to use the elevated button on flat layouts. Don’t use elevated buttons on dialogs or cards since they are already elevated. The elevated button has onPressed callback which is invoked when the user taps the button.
Types of Flutter ElevatedButton
Flutter offers two types of Elevated buttons.
- ElevatedButton
- ElevatedButton.icon
Both ElevatedButton and ElevatedButton.icon have the same properties. The only difference is, ElevatedButton requires a child, and ElevatedButton.icon requires an icon and label.
ElevatedButton
ElevatedButton contains a child widget and onPressed and onLongPress callback to listen to user click/tap. The child is required which means we can’t use the button without providing a value to the child property. Setting onPressed to null or not using onPressed property will disable the button.
Constructor:
ElevatedButton(
{Key key,
@required VoidCallback onPressed,
VoidCallback onLongPress,
ButtonStyle style,
FocusNode focusNode,
bool autofocus: false,
Clip clipBehavior: Clip.none,
@required Widget child
}
)
Flutter ElevatedButton Example
ElevatedButton(
child: Text("Button"),
onPressed:() {
},
)
Output:
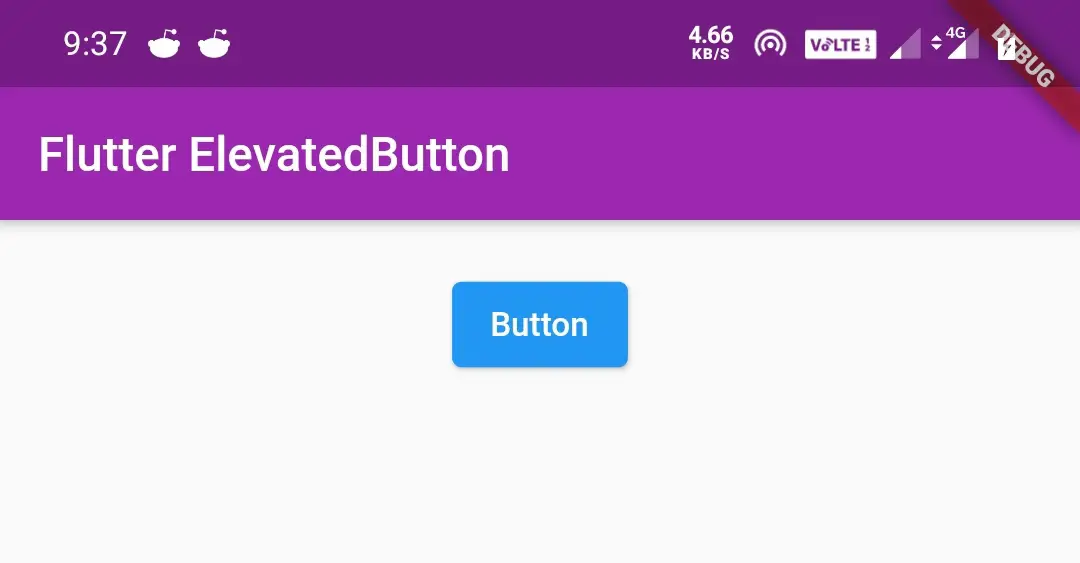
ElevatedButton.icon
It contains icon, label widgets, and an onPressed and onLongPress callback. The icon and label properties are required which means we can’t use ElevatedButton.icon without providing values to icon and label properties. Setting onPressed to null or not using onPressed property will disable the button.
Constructor:
ElevatedButton.icon(
{Key key,
@required VoidCallback onPressed,
VoidCallback onLongPress,
ButtonStyle style,
FocusNode focusNode,
bool autofocus,
Clip clipBehavior,
@required Widget icon,
@required Widget label
}
)
Basic ElevatedButton.icon code
ElevatedButton.icon(
icon: Icon(Icons.save),
label: Text("Save"),
onPressed:() {
},
)
Output:
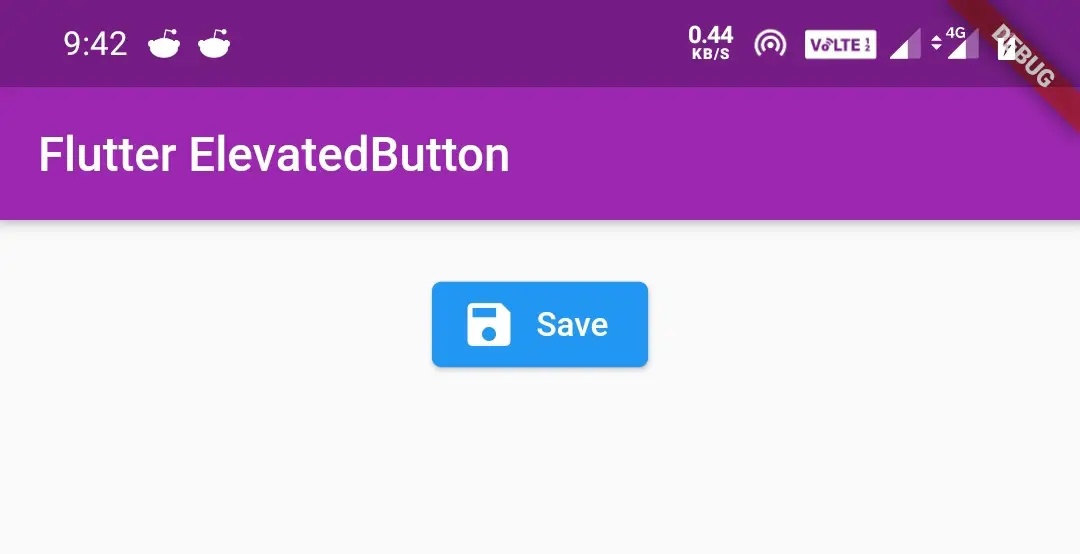
Styling Flutter ElevatedButton
We can style the ElevatedButton widget by changing colors, backgroundColor, etc. To style the ElevatedButton we have to use style property which takes ButtonStyle() or styleFrom().
The static styleFrom method is a convenient way to create an ElevatedButton ButtonStyle from simple values rather than ButtonStyle(). Let’s see an example.
Using ButtonStyle() for changing foregroundColor.
ElevatedButton(
style: ButtonStyle(
backgroundColor: MaterialStateProperty.all<Color>(Colors.green),
),
)
Using styleFrom() for changing foregroundColor.
ElevatedButton(
child: Text("button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.green
),
If we observe the above two cases the code of styleFrom() is simple compared to ButtonStyle(). So I will be using styleFrom() in the coming examples.
The styleFrom() has many properties. Let’s see the important ones in detail.
- onPrimary
- primary
- onSurface
- side
- elevation
- minimumSize
- shadowColor
- shape
- padding
- textStyle
Flutter ElevatedButton foregroundColor(onPrimary)
We will use the onPrimary (foregroundColor) property to apply color to text and icon of the ElevatedButton.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
),
onPressed:() {
},
)
Output:
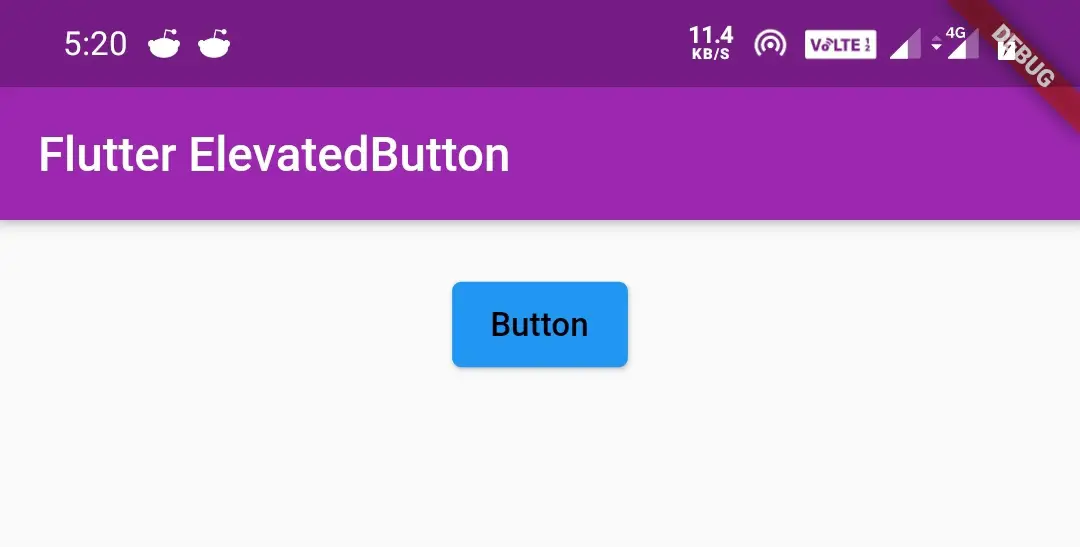
Flutter ElevatedButton backgroundColor(primary)
We will use the primary (backgroundColor) property to apply background color to the ElevatedButton.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
),
onPressed:() {
},
)
Output:
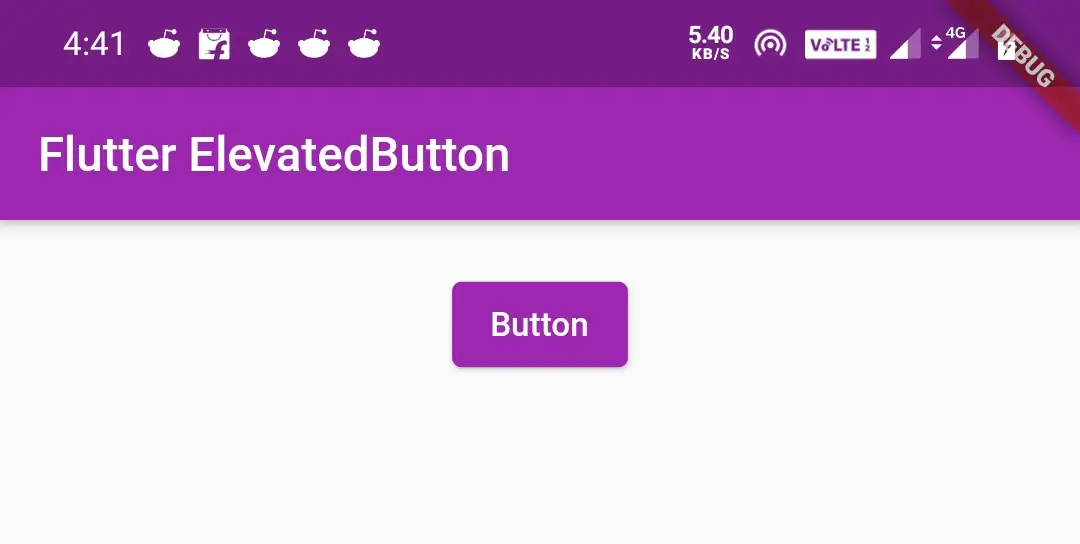
Flutter ElevatedButton disabledColor(onSurface)
We will use onSurface (disabledColor) property to define the color of the ElevatedButton text and background we want to display when the button is disabled. I’m disabling the button by setting the onPressed to null, to display the output.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
),
onPressed:null,
)
Output:
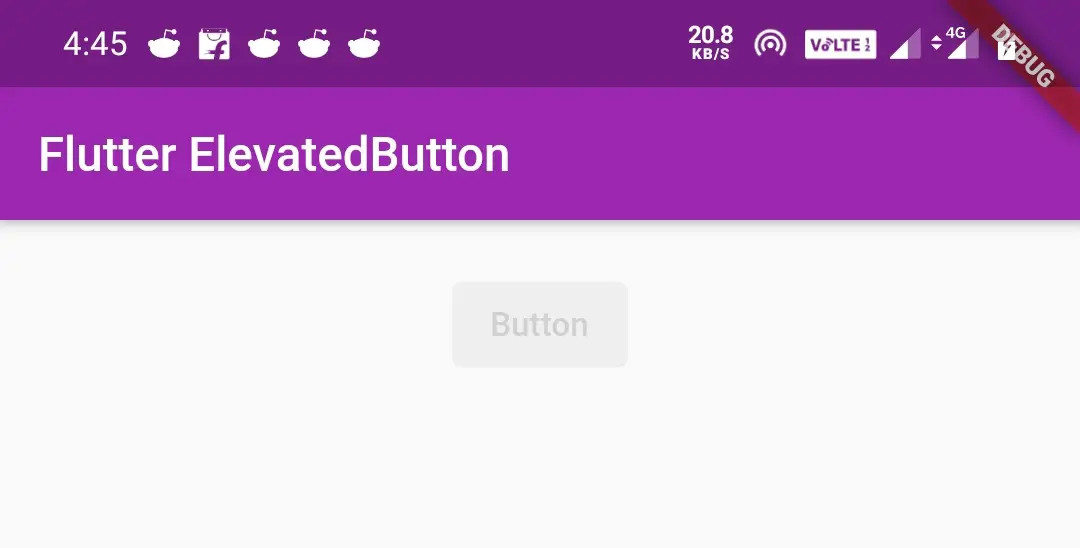
Flutter ElevatedButton overlayColor
Use overlayColor property to display color when we press (down) the ElevatedButton. It will start filling the button when we press (down) it and disappears when we release the button. For this purpose I’m using ButtonStyle(), as styleFrom() don’t have overlayColor property.
ElevatedButton(
child: Text("Button"),
style: ButtonStyle(
backgroundColor: MaterialStateProperty.all<Color>(Colors.purple),
foregroundColor: MaterialStateProperty.all<Color>(Colors.white),
overlayColor: MaterialStateProperty.all<Color>(Colors.grey),
),
onPressed:() {
},
)
Flutter ElevatedButton side
We will use the side property if we want to apply a border to the ElevatedButton. We can define the border color, width, and style.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
),
onPressed:() {
},
)
Output:
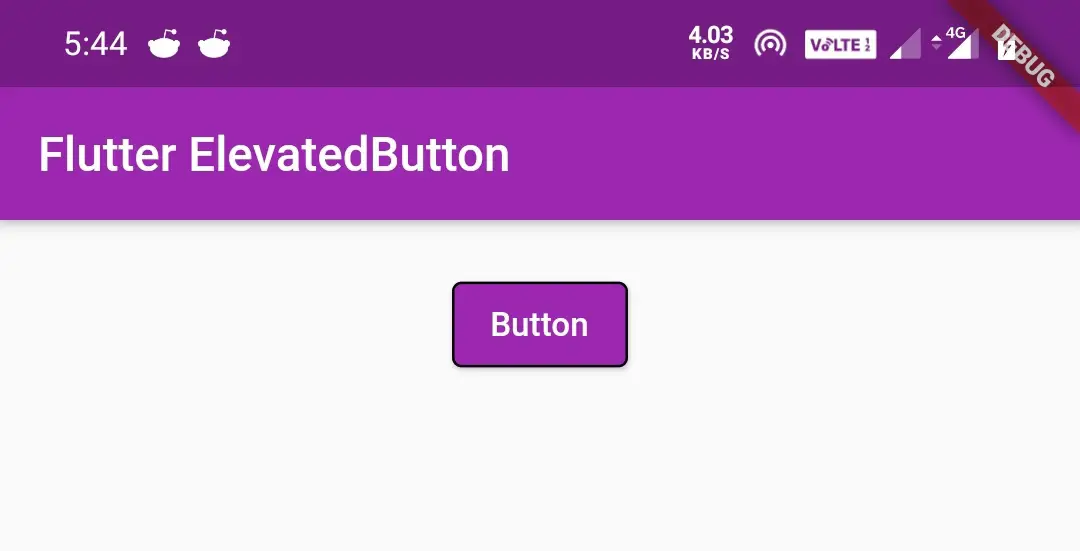
Flutter ElevatedButton elevation
We will use the elevation property to apply elevation to the ElevatedButton. Elevation makes a button look like as it is lifted upward from the background/surface on which the button is placed.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
),
onPressed:() {
},
)
Output:
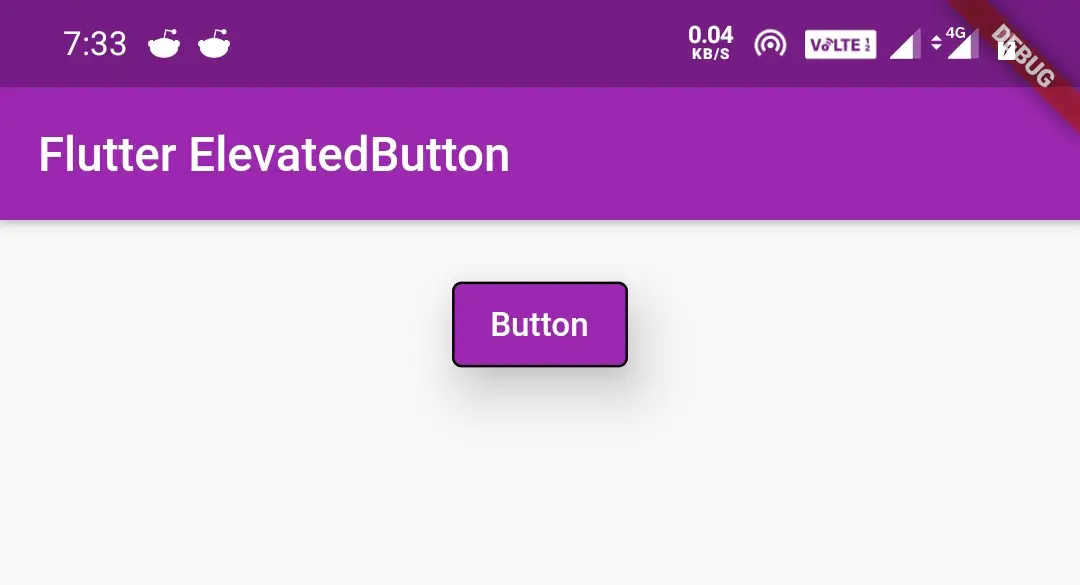
Flutter ElevatedButton minimumSize
We will use minimumSize property to define the minimum size we want the ElevatedButton to be displayed.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
),
onPressed:() {
},
)
Output:
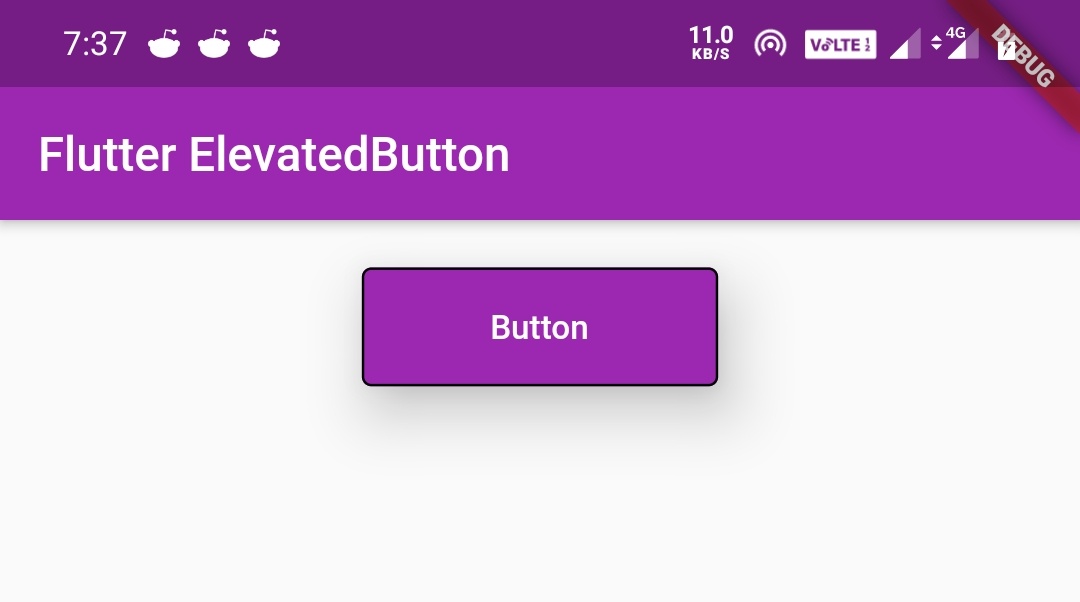
Flutter ElevatedButton shadowColor
We will use shadowColor Property to change the color of the shadow that appears when the ElevatedButton is elevated.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
),
onPressed:() {
},
)
Output:
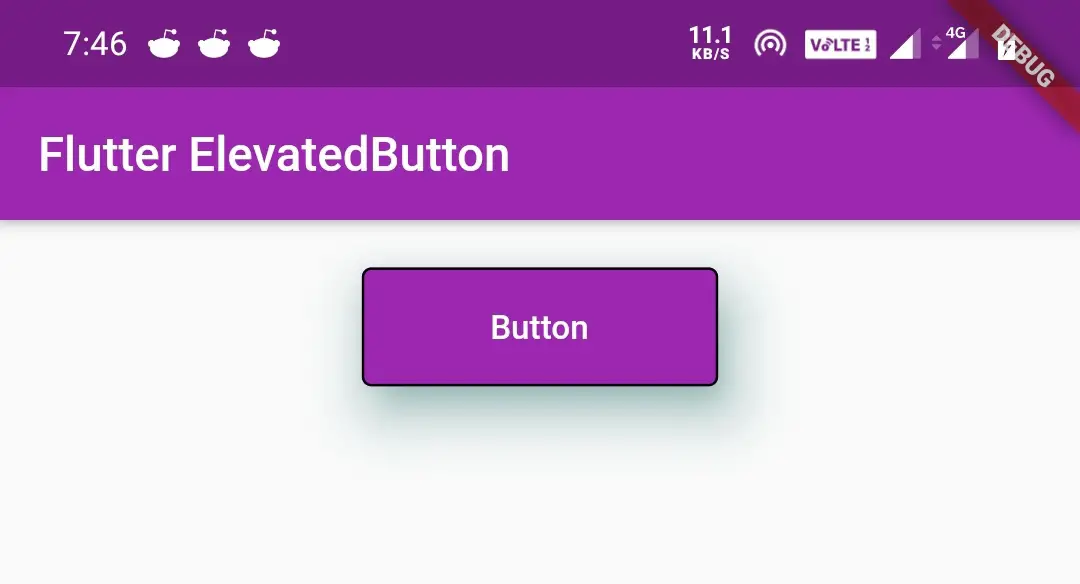
Flutter ElevatedButton shape
By using the shape property we can apply different shapes to the ElevatedButton. We can apply shapes like rectangular, rounded rectangle, circular, etc.
I’m explaining two shapes as we can’t discuss all the concepts in one article. So try the rest for yourself. To know more about different shapes refer shaping RaisedButton section of flutter RaisedButton widget Example.
RoundedRectangleBorder()
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(30)),
),
onPressed:() {
},
)
Output:
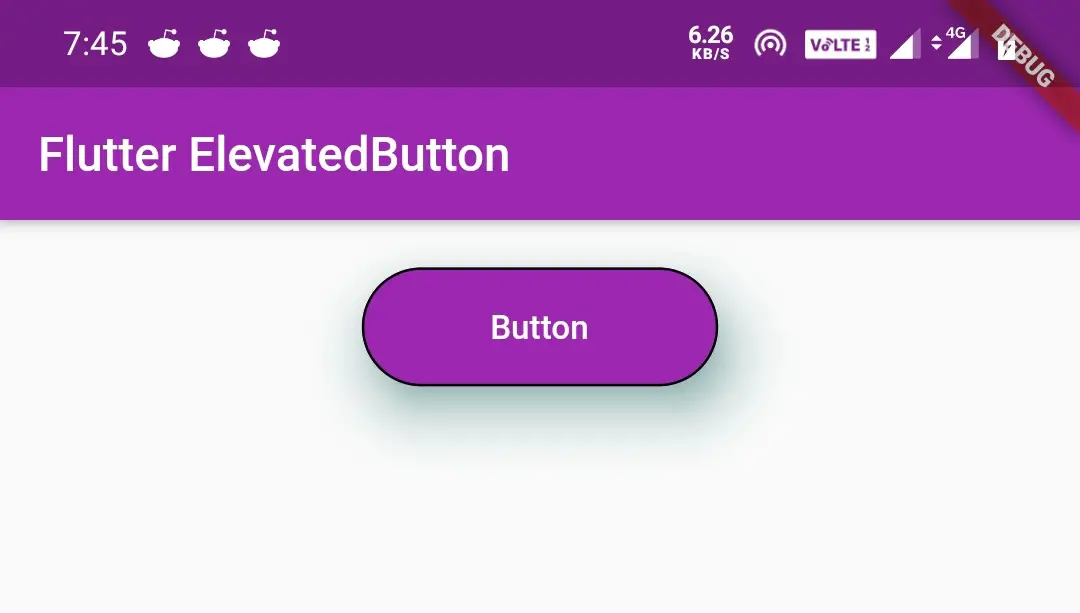
BeveledRectangleBorder()
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
shape: BeveledRectangleBorder(
side: BorderSide(
color: Colors.green,
width: 2
),
borderRadius: BorderRadius.circular(15)
),
),
onPressed:() {
},
)
Output:
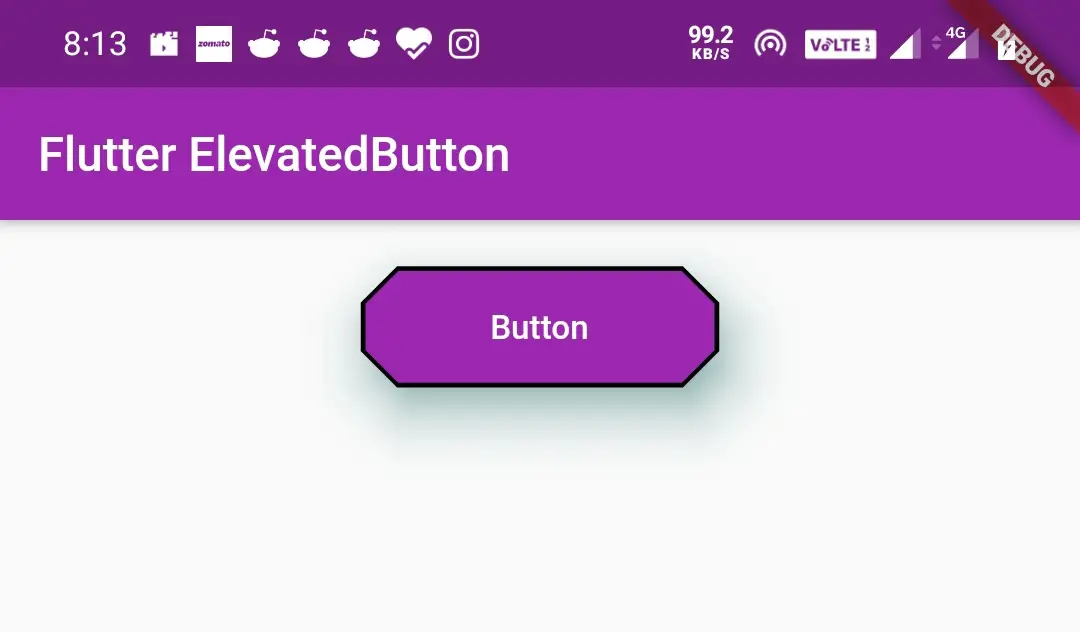
Flutter ElevatedButton padding
The padding property provides padding to the ElevatedButton, which means internal spacing. It takes EdgeInsetsGeometry which has three methods all, only and symmetric.
EdgeInsets.only()
We can specify up to four values, which means we can define our desired sides and leave the sides we don’t want to define padding for.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
padding: EdgeInsets.only(
left: 80,
right: 80,
top: 15,
bottom: 15
)
),
onPressed:() {
},
)
Output:
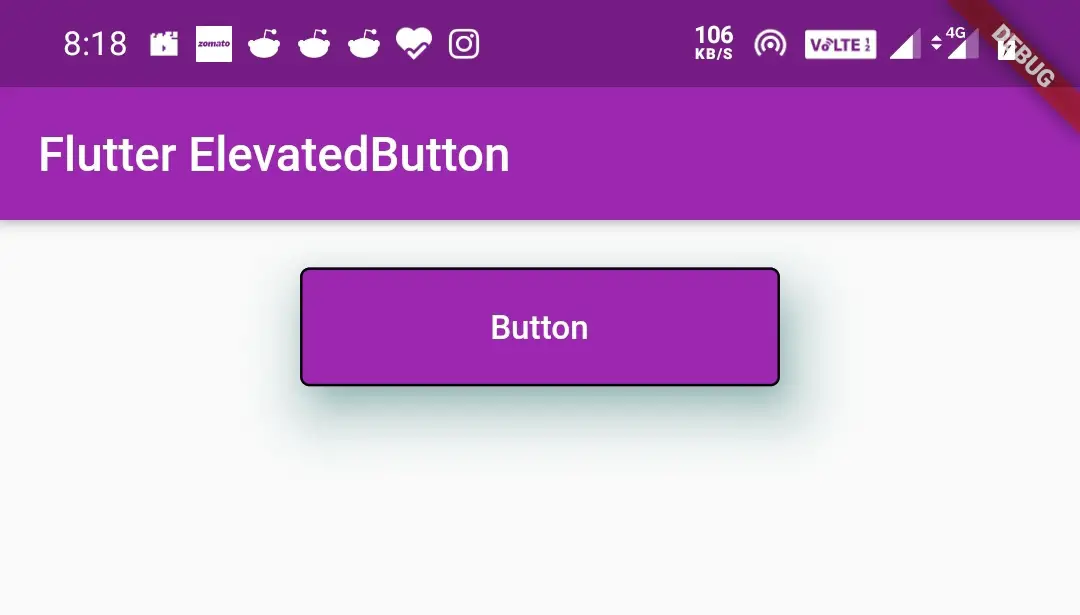
EdgeInsets.all()
It accepts one value which will be applied to all the sides.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
padding: EdgeInsets.all(30)
),
onPressed:() {
},
)
Output:
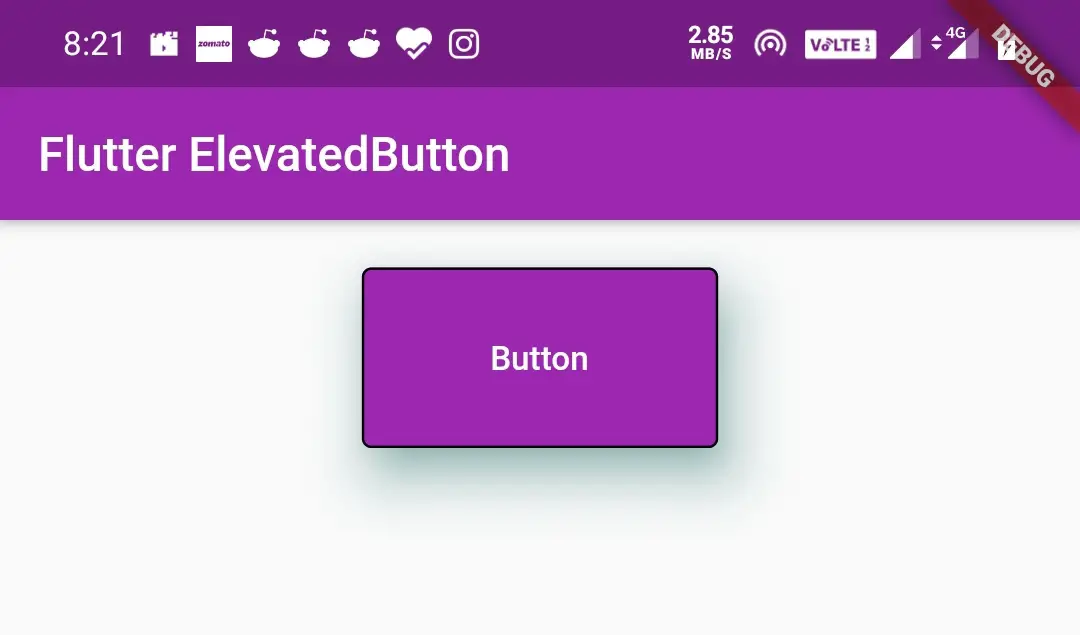
EdgeInsets.symmetric()
This accepts horizontal and vertical values. You can provide only horizontal, only vertical, or both.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
padding: EdgeInsets.symmetric(
vertical: 20,
horizontal: 120
)
),
onPressed:() {
},
)
Output:
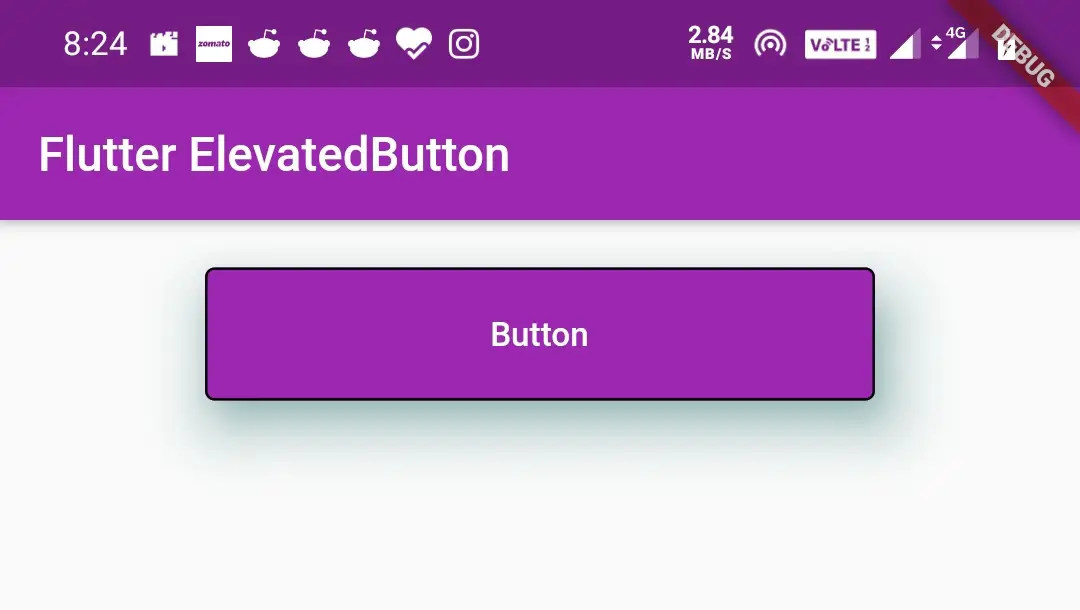
Flutter ElevatedButton textStyle
We will use textStyle property to apply different styles like fontStyle, fontSize etc. to the ElevatedButton text. It accepts TextStyle class as the value.
We can’t discuss all the properties of TextStyle class in this article. I’m showing a single example with fontStyle, fontSize, and fontFamily . Try the rest for yourself. To know about all the properties of the TextStyle class refer flutter text widget tutorial.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
onSurface: Colors.grey,
side: BorderSide(color: Colors.black, width: 1),
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
padding: EdgeInsets.symmetric(
vertical: 20,
horizontal: 60
),
textStyle: TextStyle(
fontSize: 20,
fontStyle: FontStyle.italic,
fontFamily: "alex",
)
),
onPressed:() {
},
)
Output:
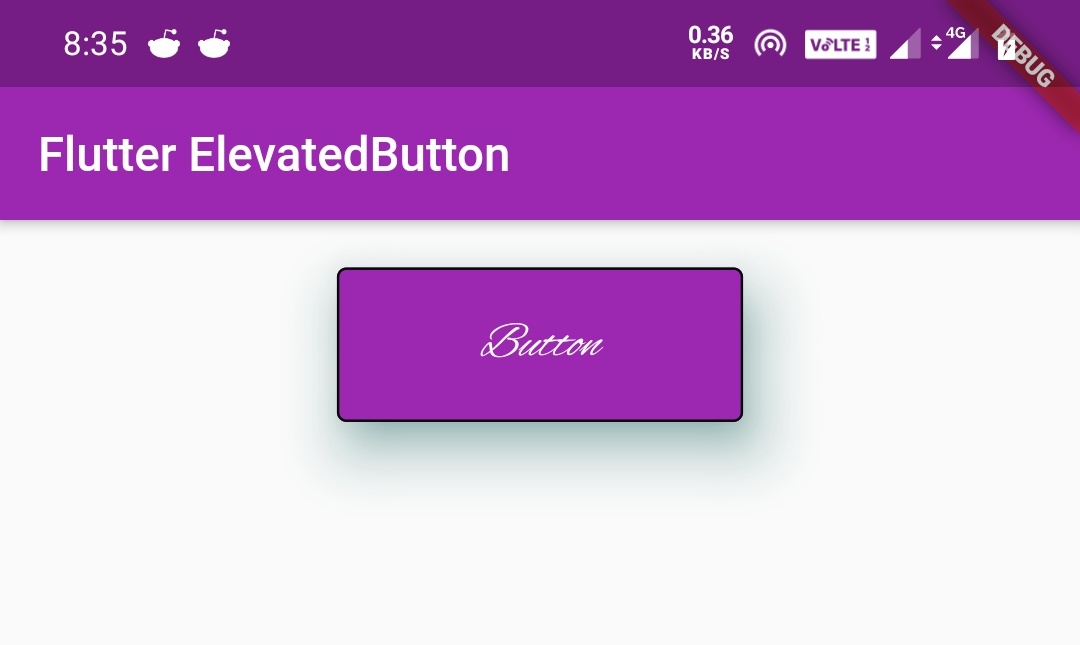
Comparing ButtonStyle and styleFrom
Let’s see how the code will look like if we use ButtonStyle() instead of styleFrom() with all the properties.
Using ButtonStyle() for styling the button.
ElevatedButton(
child: Text("button"),
style:ButtonStyle(
backgroundColor: MaterialStateProperty.all<Color>(Colors.purple),
foregroundColor: MaterialStateProperty.all<Color>(Colors.white),
elevation: MaterialStateProperty.all<double>(20),
minimumSize: MaterialStateProperty.all<Size>(Size(150, 50)),
shadowColor: MaterialStateProperty.all<Color>(Colors.teal),
shape: MaterialStateProperty.all<CircleBorder>(
CircleBorder()
),
padding: MaterialStateProperty.all<EdgeInsetsGeometry>(
EdgeInsets.all(30)
),
textStyle: MaterialStateProperty.all<TextStyle>(
TextStyle(
fontSize: 20,
fontStyle: FontStyle.italic,
fontFamily: "alex"
)
)
),
onPressed:() {
},
)
Using styleFrom() for styling the button.
ElevatedButton(
child: Text("Button"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.purple,
elevation: 20,
minimumSize: Size(150,50),
shadowColor: Colors.teal,
padding: EdgeInsets.symmetric(
vertical: 20,
horizontal: 60
),
textStyle: TextStyle(
fontSize: 20,
fontStyle: FontStyle.italic,
fontFamily: "alex",
)
),
onPressed:() {
},
)
If we observe the above code snippets, the output will be the same for both. We can see that the styleFrom() is clear and convenient than ButtonStyle(). So its better to use styleFrom() instead of ButtonStyle().
That’s all about flutter ElevatedButton example. Let’s catch up with some other flutter widget in the next article. Have a great day!!
Do share, subscribe, and like my Facebook page, if you find this post helpful. Thank you!!
Reference:Flutter Official Documentation.

Leave a Reply