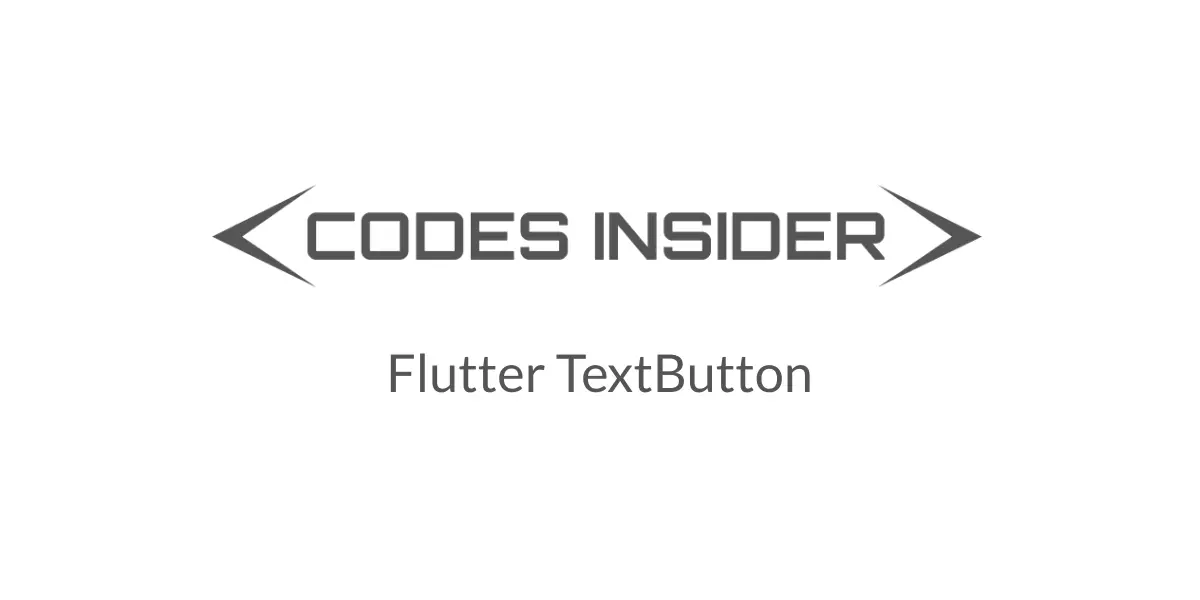
In flutter, we will use TextButton widget to display a simple button. It is the replaced version of FlatButton widget as the FlatButton widget will be deprecated soon. It is one of the most used widgets in flutter. In this example tutorial, we will learn how to use a TextButton widget in flutter and its properties in detail.
Note: To use TextButton widget in flutter use flutter SDK version 1.22.0 or above. Also, update your flutter plugin.
Flutter TextButton
A textbutton is nothing but a simple button in flutter with no elevation and background by default. We can perform some action inside the onPressed callback when a user clicks on it. It also has a onLongPressed callback to listen when the user press the button for a long period of time. Generally textbuttons should be used in dialogs and cards. Don’t use text button in places where it would mix with the content as it has no border. We can apply a background color instead to differentiate it with the content.
Types of Flutter TextButton
Flutter offers two types of text buttons
- TextButton
- TextButton.icon
Both TextButton and TextButton.icon have the same properties. The only difference between TextButton and TextButton.icon is, TextButton requires a child which is a widget, and TextButton.icon requires an icon and label.
TextButton
A text button contains a child widget and onPressed and onLongPress callback to listen user click/tap. The child is required which means we can’t use the TextButton without providing a value to the child property. Setting onPressed to null or not using onPressed property will disable the button.
Constructor:
TextButton(
{Key key,
@required VoidCallback onPressed,
VoidCallback onLongPress,
ButtonStyle style,
FocusNode focusNode,
bool autofocus: false,
Clip clipBehavior: Clip.none,
@required Widget child
}
)
Flutter TextButton Example
TextButton(
child: Text("Button"),
onPressed:() {
},
)
Output:
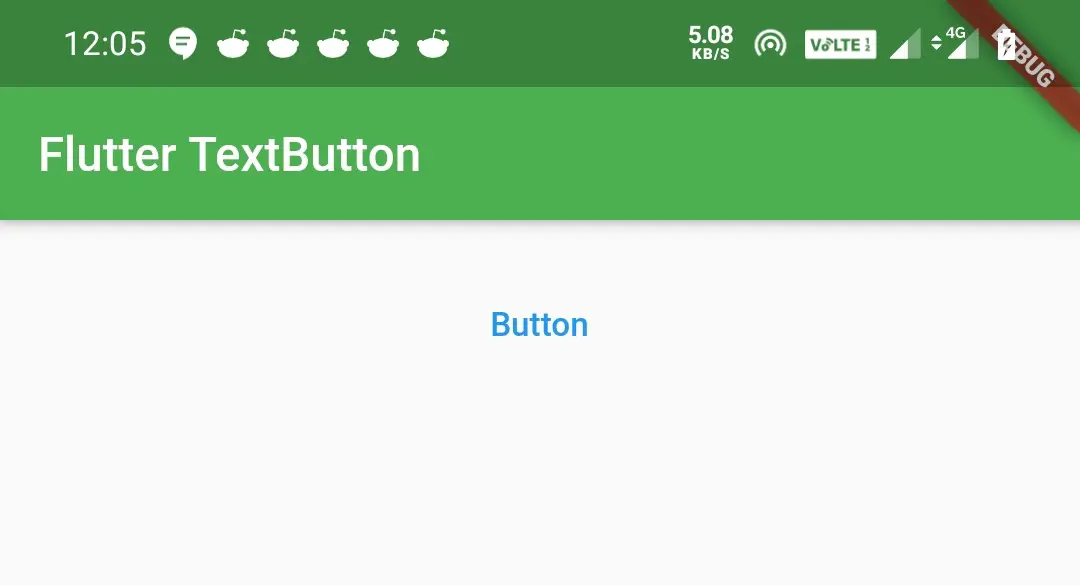
TextButton.icon
It contains icon, label widgets and an onPressed and onLongPress callback. The icon and label properties are required which means we can’t use the TextButton.icon without providing values to icon and label properties. Setting onPressed to null or not using onPressed property will disable the button.
Constructor:
TextButton.icon(
{Key key,
@required VoidCallback onPressed,
VoidCallback onLongPress,
ButtonStyle style,
FocusNode focusNode,
bool autofocus,
Clip clipBehavior,
@required Widget icon,
@required Widget label
}
)
Basic TextButton.icon code
TextButton.icon(
icon: Icon(Icons.save),
label: Text("SAVE"),
onPressed:() {
},
)
Output:
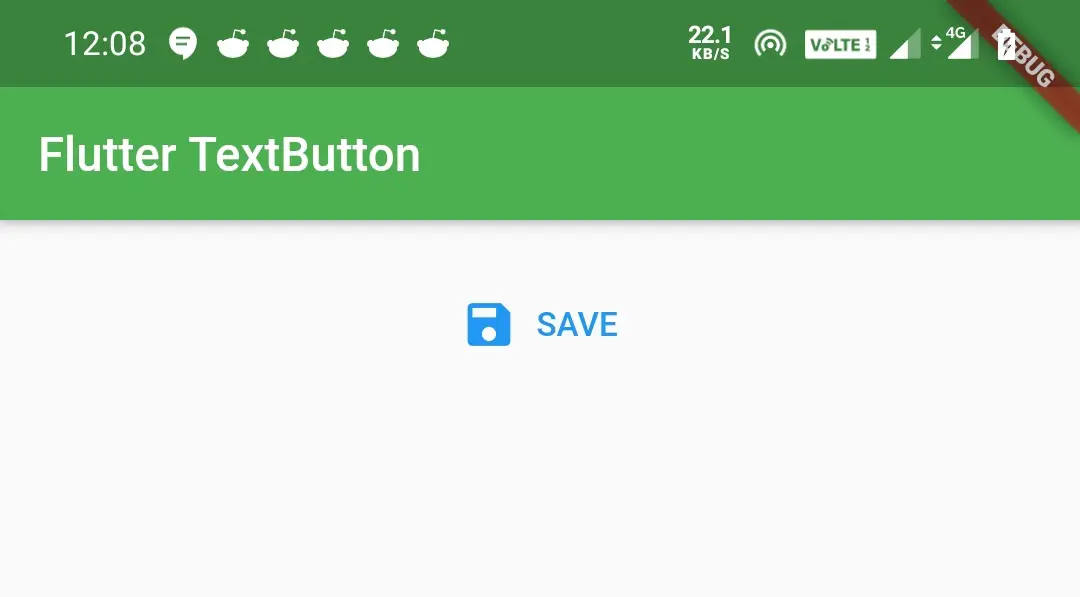
Styling Flutter TextButton
We can style the TextButton widget by changing colors, backgroundColor, etc. Let’s see the properties that help us to style the text button. To style the text button we have to use the style property which takes ButtonStyle() or styleFrom(). The second one is convenient for implementation.
Using ButtonStyle() to change foregroundColor.
TextButton(
style: ButtonStyle(
backgroundColor: MaterialStateProperty.all<Color>(Colors.green),
),
)
Using styleFrom() to change foregroundColor.
TextButton(
style: TextButton.styleFrom(
primary: Colors.green),
)
If we observe the above two cases the code of styleFrom() is simple compared to ButtonStyle(). So I will be using styleFrom() in the coming examples. You can use your desired one.
The styleFrom() has many properties. Lets see the important ones in detail.
- primary
- backgroundColor
- onSurface
- side
- elevation
- minimumSize
- shadowColor
- shape
- padding
- textStyle
Flutter TextButton foregroundColor(primary)
We will use the primary(foregroundColor) property to apply color to text and icon of the TextButton.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.green,
),
onPressed:() {
},
)
Output:
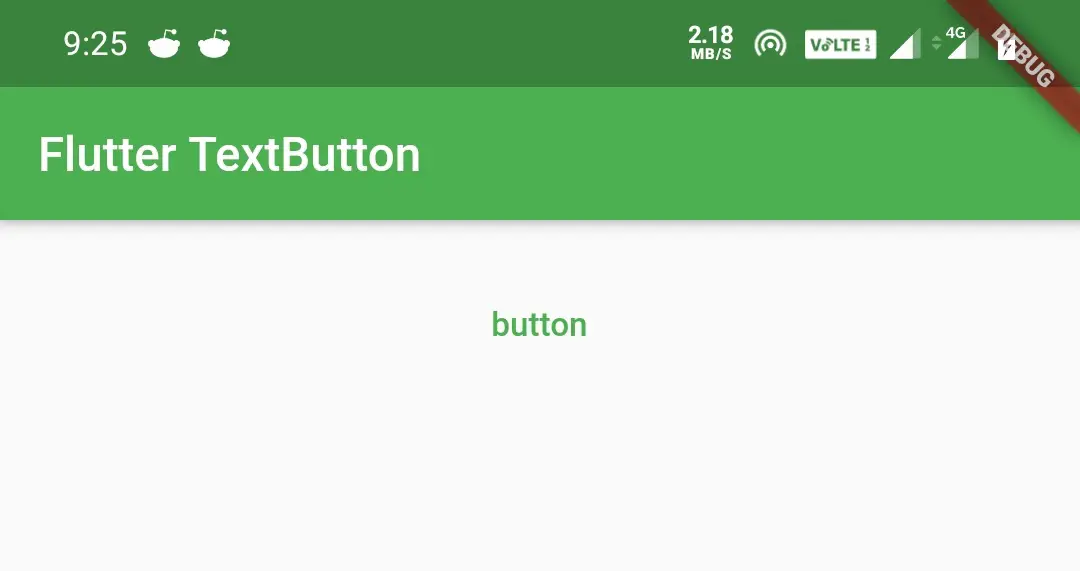
Flutter TextButton backgroundColor
We will use backgroundColor property to apply background color to the TextButton.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
),
onPressed:() {
},
)
Output:
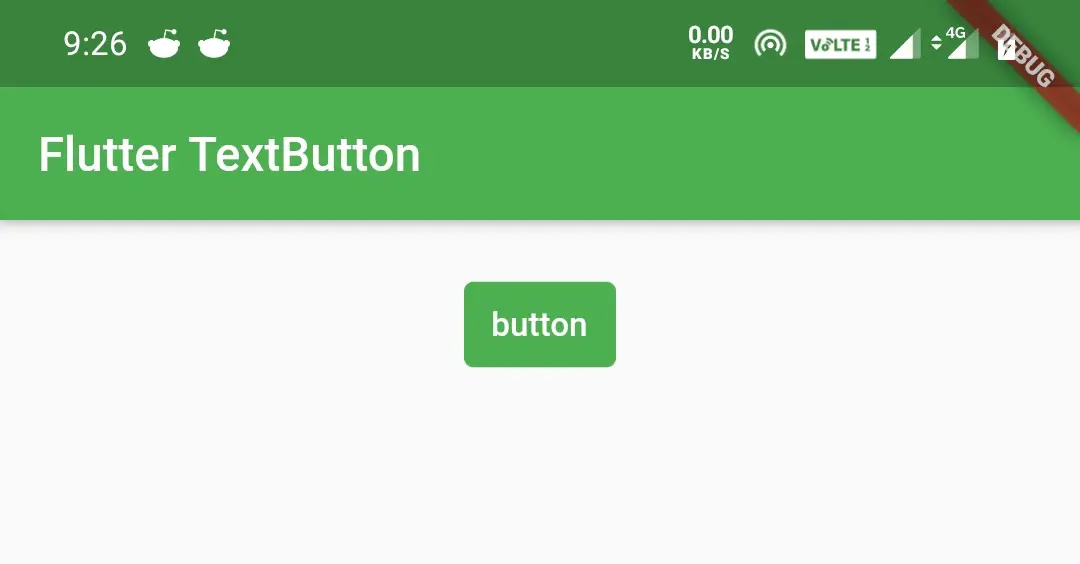
Flutter TextButton disabledColor(onSurface)
We will use onSurface (disabledColor) property to define the color of the TextButton text we want to display when the button is disabled. I’m disabling the button by setting the onPressed to null, to display the output.
TextButton(
child: Text("Button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
onSurface: Colors.black,
),
onPressed:null,
)
Output:
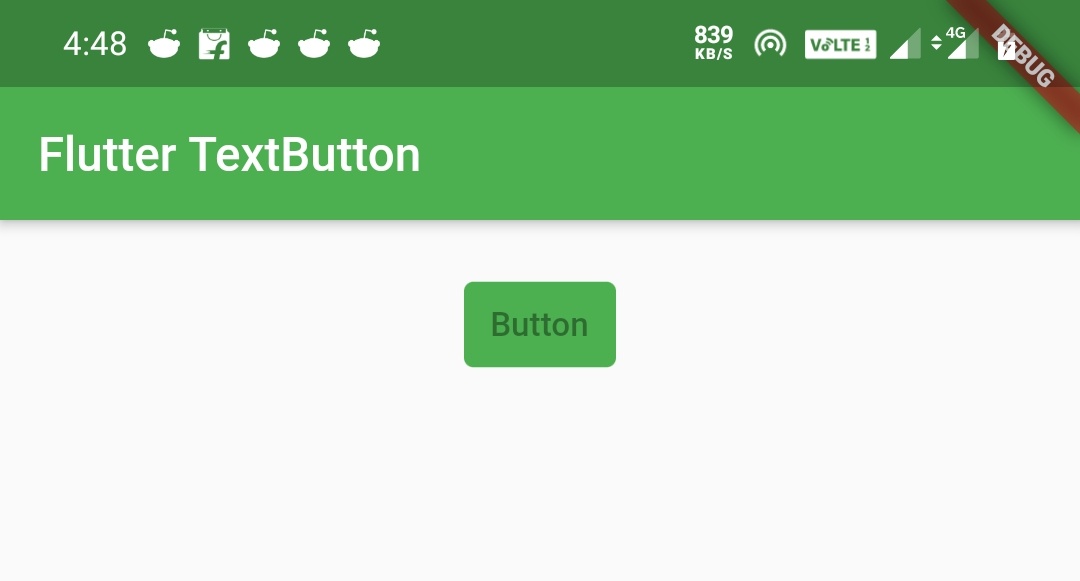
Flutter TextButton overlayColor
We will use overlayColor property to display a color when the TextButton is pressed (down). It will start filling the button when it is in pressed (down)state and disappears when the button is released. For this purpose i’m using ButtonStyle() as styleFrom() don’t have overlayColor property.
TextButton(
child: Text("button"),
style: ButtonStyle(
foregroundColor: MaterialStateProperty.all<Color>(Colors.white),
backgroundColor: MaterialStateProperty.all<Color>(Colors.green),
),
overlayColor: MaterialStateProperty.all<Color>(Colors.deepOrange),
onPressed:() {
},
)
Flutter TextButton side
We will use the side property if we want to apply a border to the TextButton. We can define the border color, width and style.
TextButton(
child: Text("button"),
style:TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1)
),
onPressed:() {
},
)
Output:
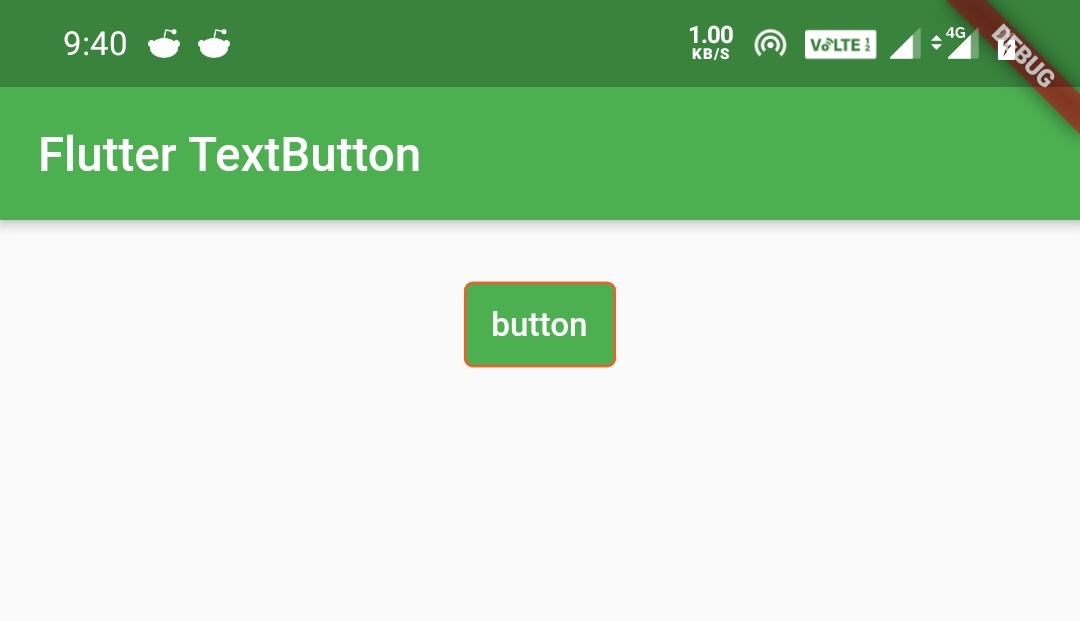
Flutter TextButton elevation
We will use the elevation property to apply elevation to the TextButton. Elevation makes a button look like as it is lifted upward from the background/surface on which the button is placed.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
),
onPressed:() {
},
)
Output:
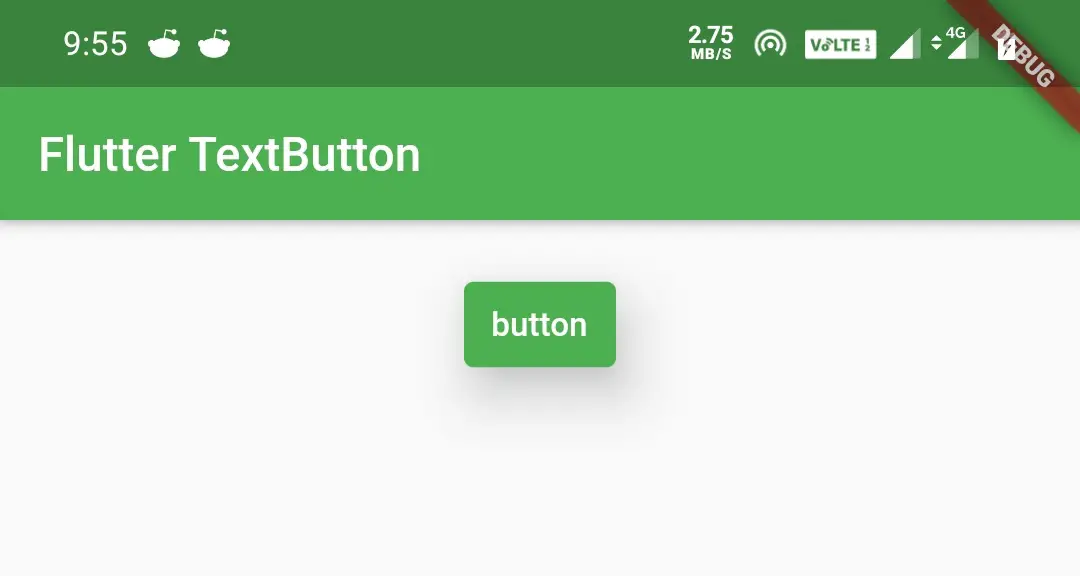
Flutter TextButton minimumSize
We will use minimumSize property to define the minimum size we want the TextButton to be display.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
),
onPressed:() {
},
)
Output:
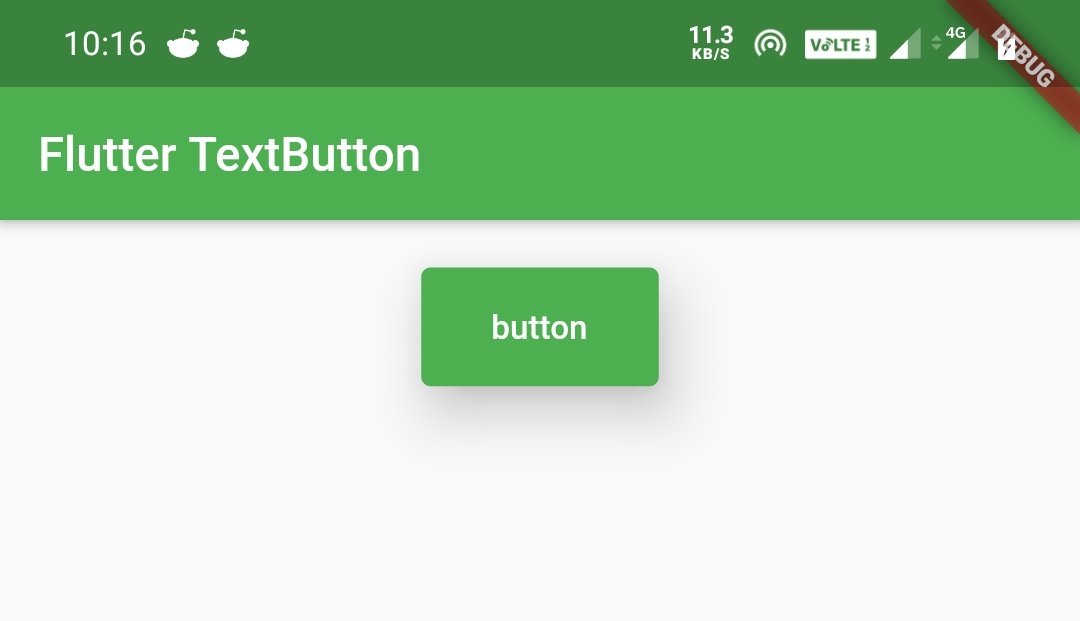
Flutter TextButton shadowColor
We will use shadowColor property to change the color of the shadow that appears when the TextButton is elevated.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
),
onPressed:() {
},
)
Output:
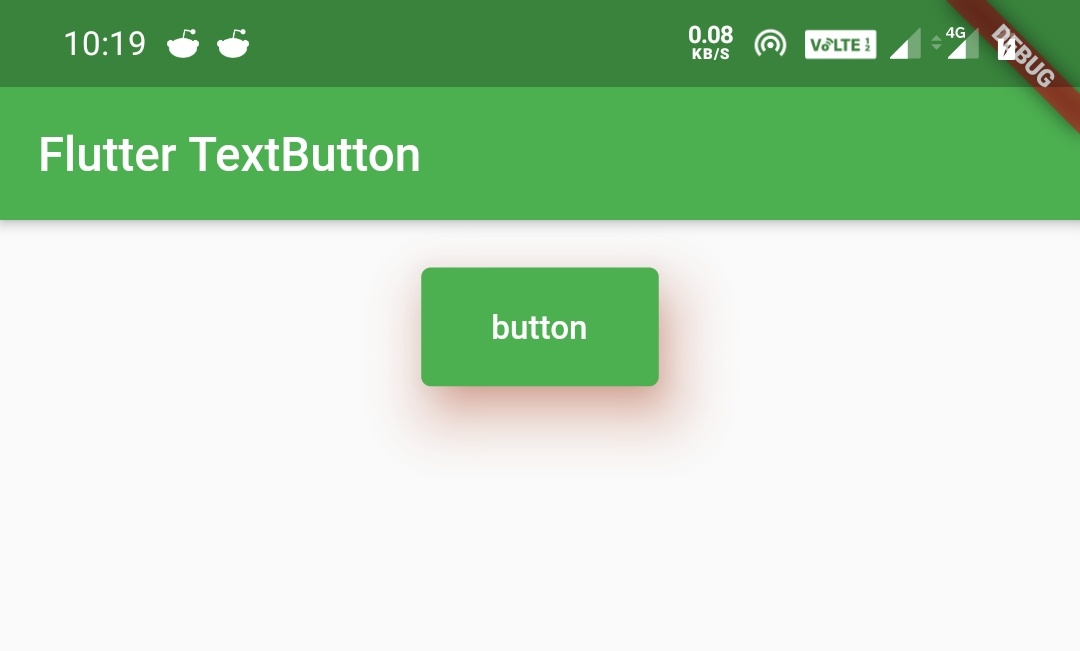
Flutter TextButton shape
By using shape property we can apply different shapes to the TextButton. We can apply shapes like rectangular , rounded rectangle, circular, etc,.
I’m explaining two shapes as we can’t discuss all the concepts in one article. So try the rest for yourself. To know more about different shapes refer shaping RaisedButton section of flutter RaisedButton widget Example.
RoundedRectangleBorder()
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(30)),
),
onPressed:() {
},
)
Output:
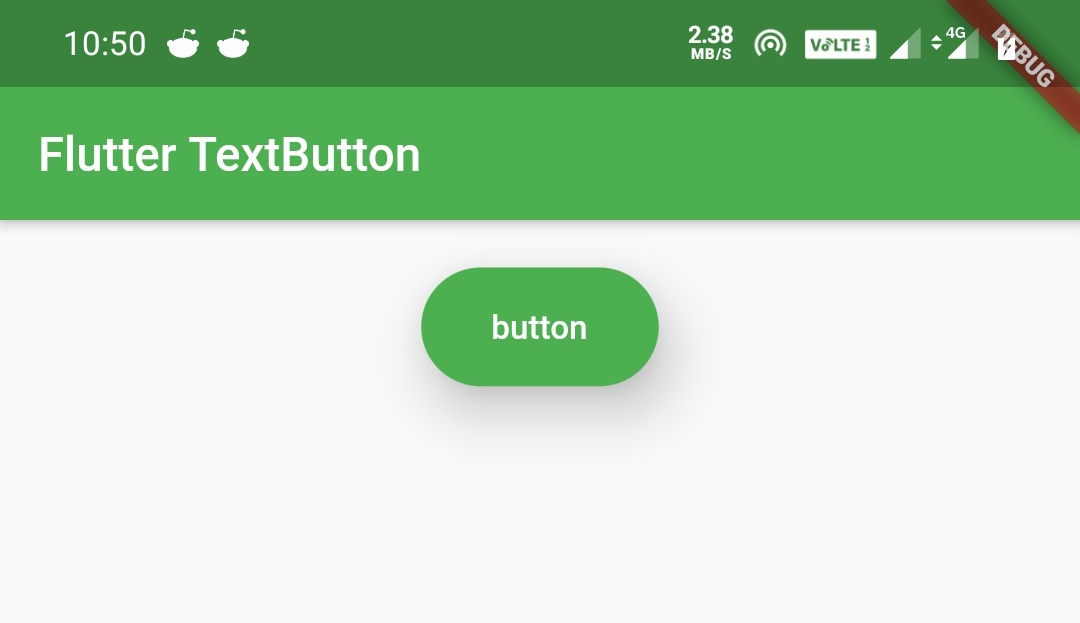
CircleBorder()
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
shape: CircleBorder(),
),
onPressed:() {
},
)
Output:
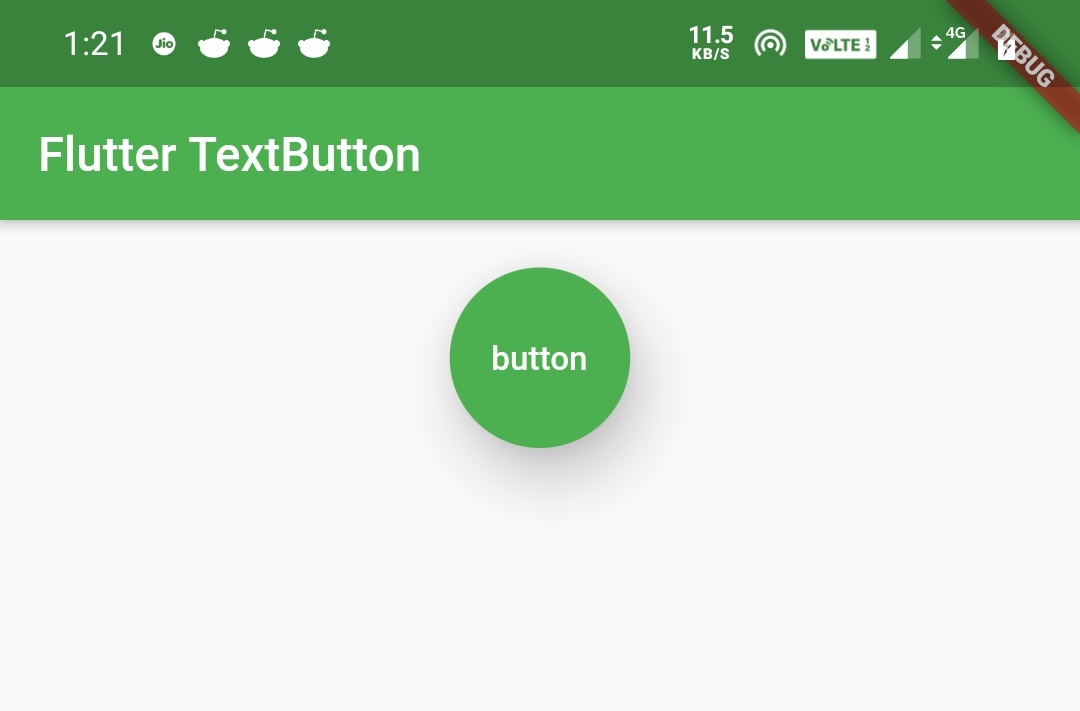
Flutter TextButton padding
The padding property provides padding to the TextButton,which means internal spacing. It takes EdgeInsetsGeometry which has three methods all, only and symmetric.
EdgeInsets.only()
We can specify up to four values, which means we can define our desired sides and leave the sides we don’t want to define padding for.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
padding: EdgeInsets.only(
left: 60,
right: 60,
top: 15,
bottom: 15
)
),
onPressed:() {
},
)
Output:
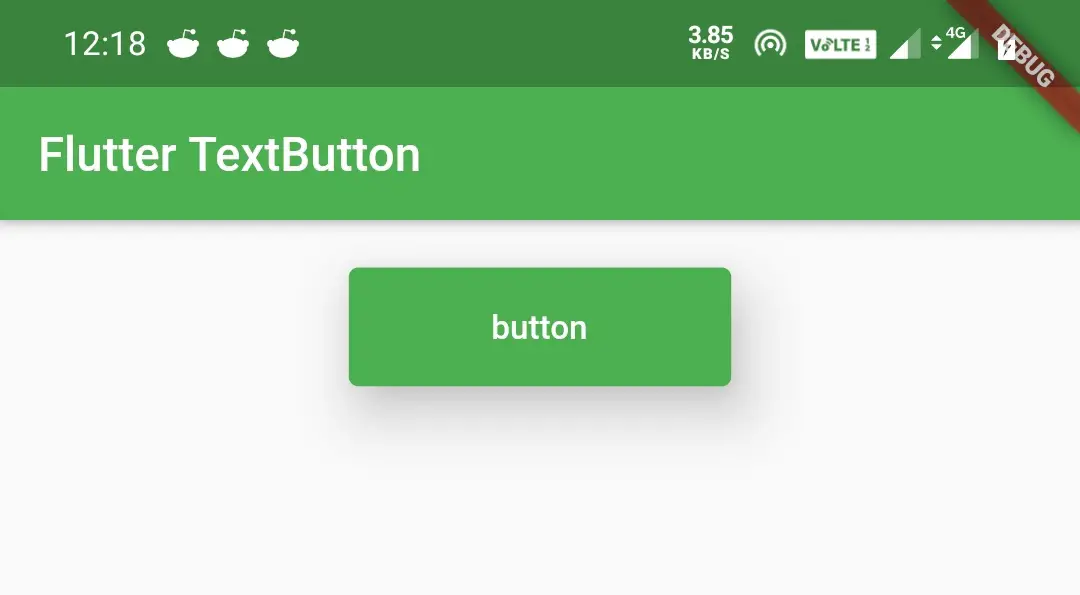
EdgeInsets.all()
It accepts one value which will be applied to all the sides.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
padding: EdgeInsets.all(30)
),
onPressed:() {
},
)
Output:
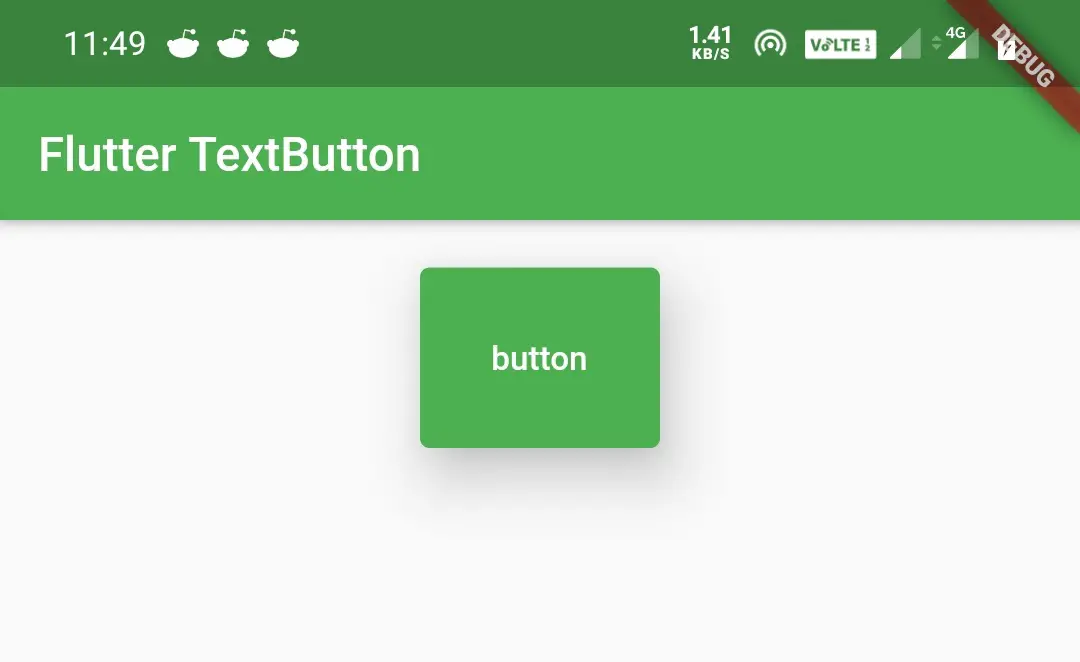
EdgeInsets.symmetric()
This accepts horizontal and vertical values.You can provide only horizontal, only vertical or both.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
padding: EdgeInsets.symmetric(
vertical: 20,
horizontal: 50
)
),
onPressed:() {
},
)
Output:
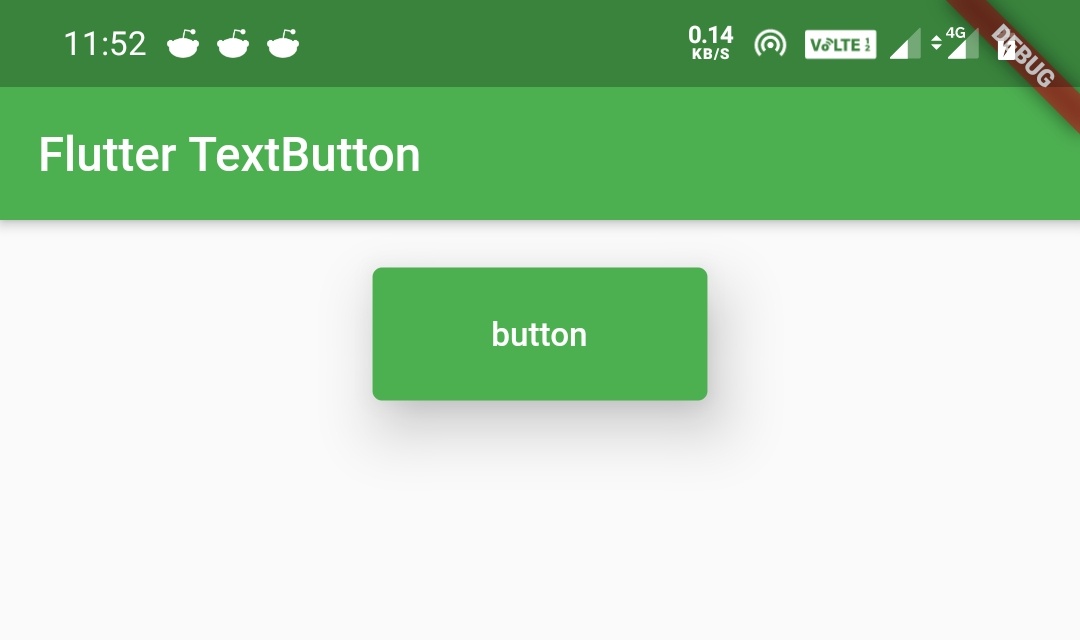
Flutter TextButton textStyle
The textStyle property is used to apply different properties like fontStyle, fontSize etc. to the TextButton text. It accepts TextStyle class as value.
We can’t discuss all the properties of TextStyle class in this article so i’m showing a single example with fontStyle and fontSize. Try the rest for yourself. To know about all the properties of the TextStyle class refer flutter text widget tutorial.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
side: BorderSide(color: Colors.deepOrange, width: 1),
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
shape: CircleBorder(),
padding: EdgeInsets.symmetric(
vertical: 20,
horizontal: 50
),
textStyle: TextStyle(
fontSize: 20,
fontStyle: FontStyle.italic
)
),
onPressed:() {
},
)
Output:
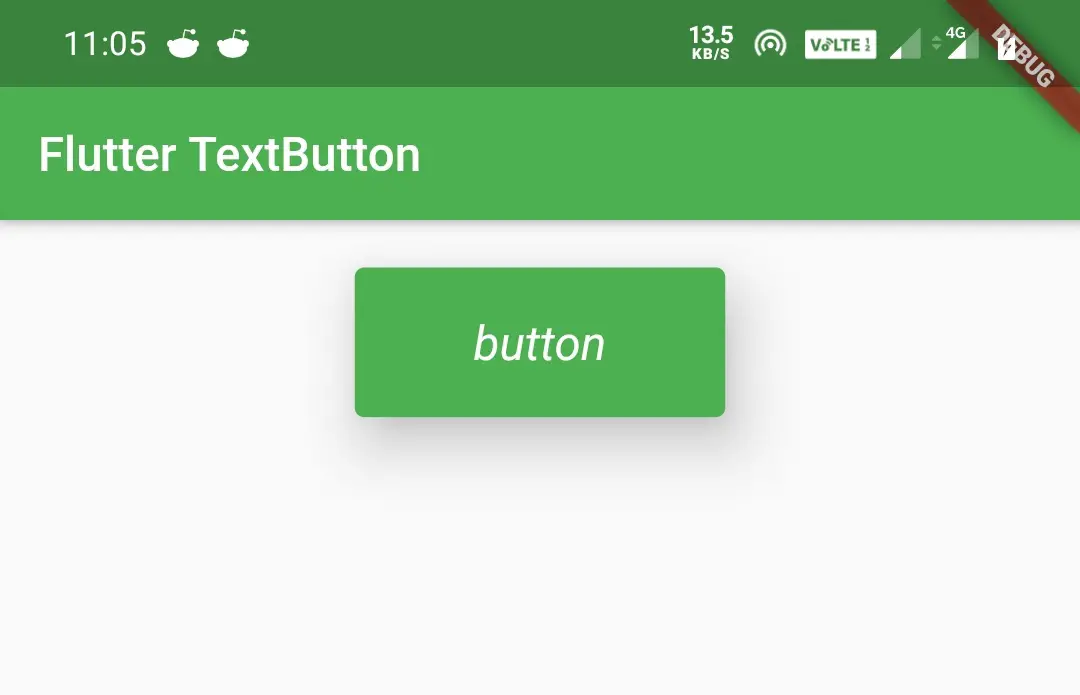
Comparing ButtonStyle and styleFrom
Let’s see how the code will look like if we use ButtonStyle() instead of styleFrom() with all the properties.
Using ButtonStyle() for styling the TextButton.
TextButton(
child: Text("button"),
style:ButtonStyle(
backgroundColor: MaterialStateProperty.all<Color>(Colors.green),
foregroundColor: MaterialStateProperty.all<Color>(Colors.white),
elevation: MaterialStateProperty.all<double>(20),
minimumSize: MaterialStateProperty.all<Size>(Size(100, 50)),
shadowColor: MaterialStateProperty.all<Color>(Colors.red),
shape: MaterialStateProperty.all<CircleBorder>(
CircleBorder()
),
padding: MaterialStateProperty.all<EdgeInsetsGeometry>(
EdgeInsets.all(30)
),
textStyle: MaterialStateProperty.all<TextStyle>(
TextStyle(
fontSize: 20,
fontStyle: FontStyle.italic
)
)
),
onPressed:() {
},
)
Using styleFrom() for styling the TextButton.
TextButton(
child: Text("button"),
style: TextButton.styleFrom(
primary: Colors.white,
backgroundColor: Colors.green,
elevation: 20,
minimumSize: Size(100, 50),
shadowColor: Colors.red,
shape: CircleBorder(),
padding: EdgeInsets.all(30),
textStyle: TextStyle(
fontSize: 20,
fontStyle: FontStyle.italic
)
),
onPressed:() {
},
)
If we observe the above code snippets, the output will be same for both, but we can see that the styleFrom() is clear and convenient than ButtonStyle(). So its better to use styleFrom() instead of ButtonStyle().
That’s all about flutter TextButton example. Let’s catch up with some other flutter widget in the next article. Have a great day!!
Do share, subscribe and like my facebook page, if you find this post helpful. Thank you!!
Reference: Flutter Official Documentation.

Leave a Reply