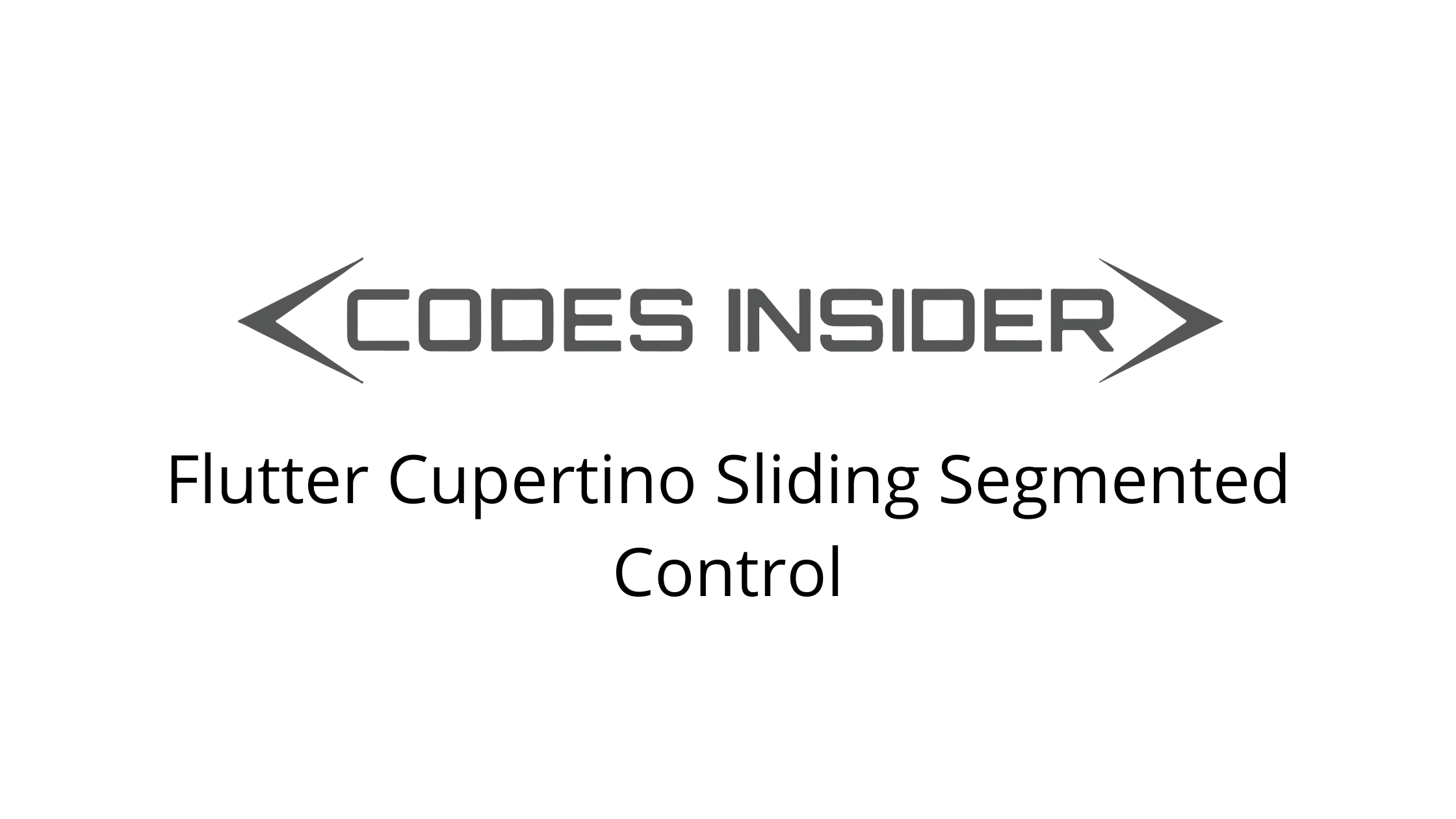
Flutter Cupertino Sliding Segmented Control
Cupertino sliding segmented control in flutter is nothing but ios style sliding segmented control. CupertinoSlidingSegmentedControl widget lets us create an ios-13 style sliding segmented control. It displays a horizontal list of options. It is similar to segmented control but with a thumb. We can select an option either by tapping on the option or by sliding the thumb to the desired option. These options are generated using map (key-value pairs <String, widget>). The keys should be similar for all the children of the map. We will use the keys to identify the selection by the user. The values can be any widget & don’t need to be similar. When we select one option we can’t select other options in the segmented control. It means we can’t select more than one option at a time.
Don’t know what Cupertino is? It is nothing but a set of flutter widgets that follow the ios design pattern. These widgets are designed to implement ios features in flutter apps built for the ios platform.
In this tutorial, we will learn how to use cupertino sliding segmented control in flutter with example. We will also learn how to customize the CupertinoSlidingSegmentedControl widget using different properties.
To implement similar behaviour for the android platform using flutter refer tabbar in flutter.
How To Create Cupertino Sliding Segmented Control In Flutter?
To create a cupertino sliding segmented control in flutter we have to use CupertinoSlidingSegmentedControl class. Call the constructor of the class and provide the required properties. The constructor has two required properties children & onValueChanged. The children should be of type map. The onValueChanged is a callback function that will return the key of the selected value.
Cupertino Sliding Segmented Control Constructor:
CupertinoSlidingSegmentedControl(
{Key? key,
required Map<T, Widget> children,
required ValueChanged<T?> onValueChanged,
T? groupValue,
Color thumbColor,
EdgeInsetsGeometry padding,
Color backgroundColor}
)
Flutter Cupertino Sliding Segmented Control Properties
The properties of a cupertino sliding segmented control are:
- children
- onValueChanged
- groupValue
- thumbColor
- padding
- backgroundColor
children
This property is used to provide children (options) to the sliding segmented control. It takes a map as value.
child: CupertinoSlidingSegmentedControl(
children: {
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
},
onValueChanged: (value)
{
},
),
Output:
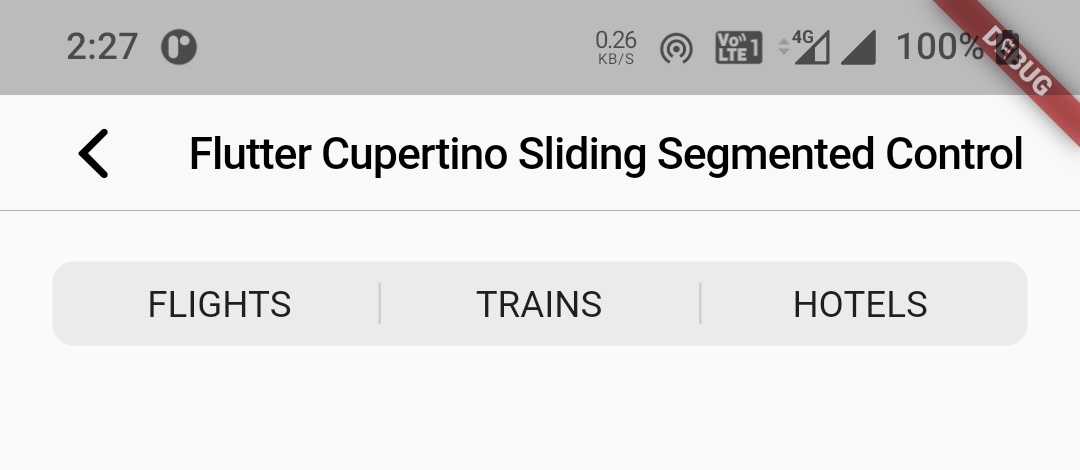
onValueChanged
It is a callback function that will invoke every time the user chooses an option. It returns the key of the selected option. We can use that value to update the UI.
child: CupertinoSlidingSegmentedControl(
children: {
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
},
onValueChanged: (value)
{
selectedValue = value;
setState(() {
});
},
),
groupValue
It indicates the option that is currently selected by the user using the value returned by onValueChanged callback. It should be either the key of the children map or null. If null it will hide the thumb as no option is selected. If it is key then the thumb will select the option of that key.
child: CupertinoSlidingSegmentedControl(
children: {
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
},
onValueChanged: (value)
{
selectedValue = value;
setState(() {
});
},
groupValue: selectedValue,
),
Output:
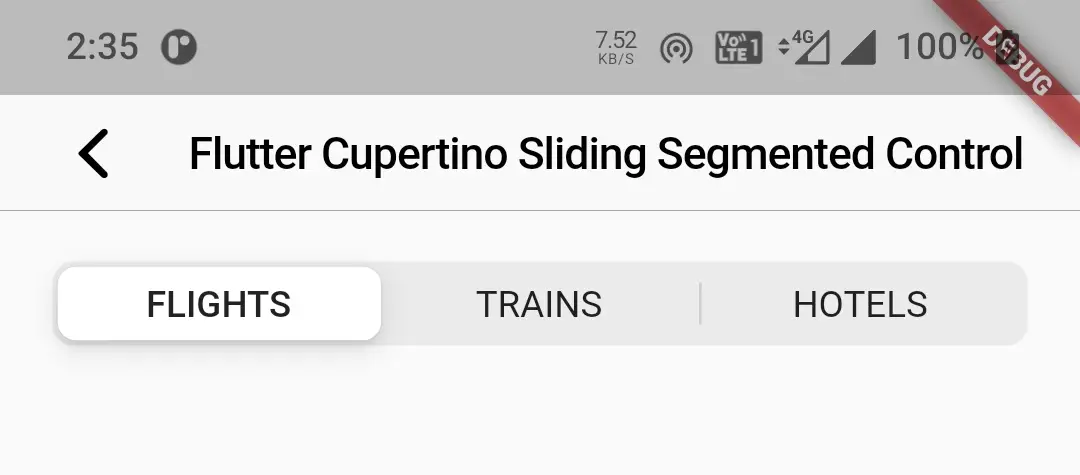
thumbColor
We will use this property to change the thumb color. It takes Colors or CupertinoColors class constant as value. To use custom color use Color class constructor.
child: CupertinoSlidingSegmentedControl(
children: {
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
},
onValueChanged: (value)
{
selectedValue = value;
setState(() {
});
},
groupValue: selectedValue,
thumbColor: CupertinoColors.activeGreen,
),
Output:
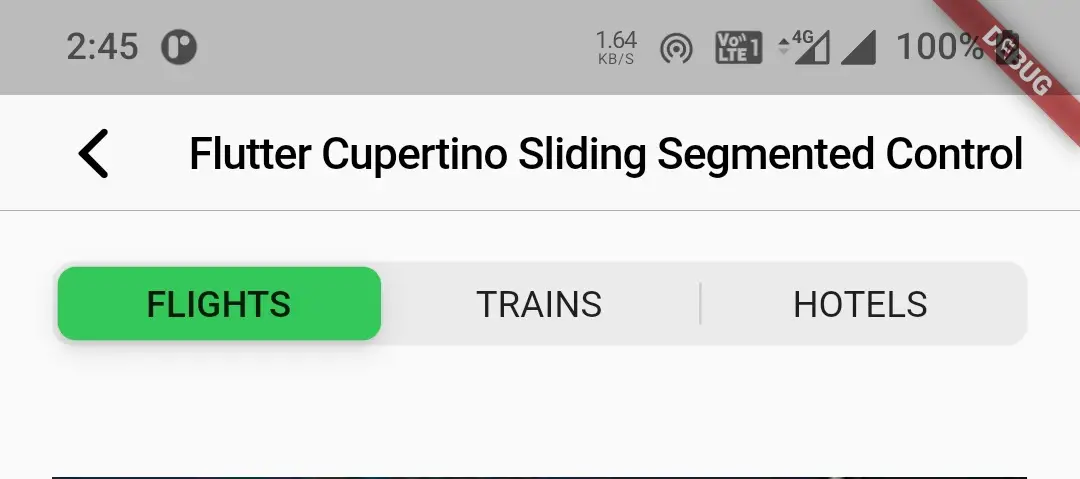
padding
We will use this property to apply padding to the sliding segmented control. It takes EdgeInsetsGeometry as value. By default it is EdgeInsets.symmetric(vertical: 2, horizontal: 3).
child: CupertinoSlidingSegmentedControl(
children: {
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
},
onValueChanged: (value)
{
selectedValue = value;
setState(() {
});
},
groupValue: selectedValue,
thumbColor: CupertinoColors.activeGreen,
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10)
),
Output:
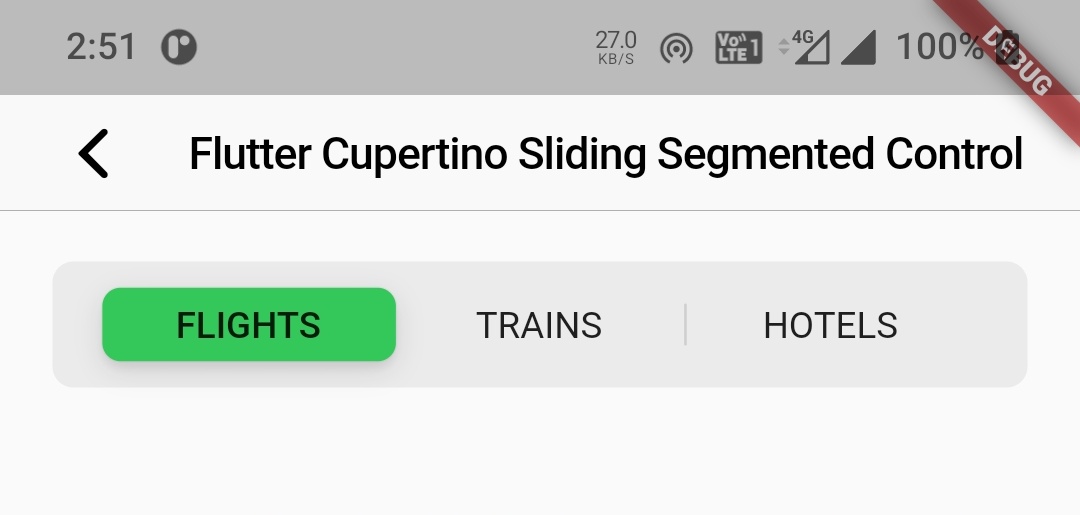
backgroundColor
To apply background color to the sliding segmented control we will use this property. It also takes CupertinoColors or Colors class constant as value.
child: CupertinoSlidingSegmentedControl(
children: {
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
},
onValueChanged: (value)
{
selectedValue = value;
setState(() {
});
},
groupValue: selectedValue,
thumbColor: CupertinoColors.activeGreen,
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10),
backgroundColor: CupertinoColors.white,
),
Output:
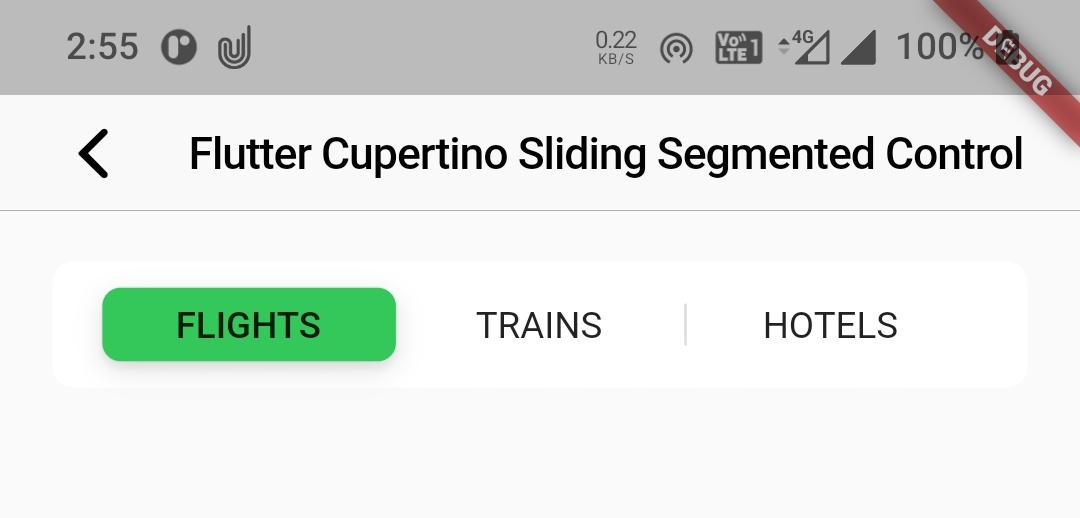
Flutter Cupertino Sliding Segmented Control Example
Let’s create an example where we will implement cupertino sliding segmented control in flutter using the CupertinoSlidingSegmentedControl widget. In this example, we will display a sliding segmented control with three options. When the user selects an option we will display the corresponding image. For this, we will need a map for children and a list for holding images.
Let’s declare and initialize the map for our children.
Map <int, Widget> children = <int, Widget>{
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
};
Now we will declare the List with the path to images. Please download three images for flight, train, and hotel. Name them as flight, train, and hotel. Add the images into assets folder. Refer how to add images to flutter app if you don’t know how to use images in a flutter.
List<String> images = ["assets/images/flight.jpg", "assets/images/train.jpg","assets/images/hotel.jpg"];
Let’s declare the variable that will hold the selected key.
int selectedValue = 0;
Let’s add the code for our cupertino sliding segmented control.
CupertinoSlidingSegmentedControl(
children: children,
onValueChanged: (value)
{
selectedValue = value;
setState(() {
});
},
groupValue: selectedValue,
thumbColor: CupertinoColors.activeGreen,
backgroundColor: CupertinoColors.white,
),
Now let’s declare the widget for displaying the image corresponding to the selected option.
Container(
height: MediaQuery.of(context).size.height*0.80,
width: double.infinity,
child: Image.asset(images[selectedValue],
fit: BoxFit.cover),
)
Complete Code:
Let’s sum up the code snippets above to complete cupertino sliding segmented control example. Create a new flutter project and replace the code in main.dart file with the code below.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
int selectedValue = 0;
Map <int, Widget> children = <int, Widget>{
0: Text("FLIGHTS"),
1: Text("TRAINS"),
2: Text("HOTELS")
};
List<String> images = ["assets/images/flight.jpg", "assets/images/train.jpg","assets/images/hotel.jpg"];
@override
Widget build(BuildContext context) {
return new CupertinoPageScaffold(
navigationBar:CupertinoNavigationBar(
leading: CupertinoNavigationBarBackButton(
onPressed: ()
{ },
color: CupertinoColors.label,
),
middle: Text("Flutter Cupertino Sliding Segmented Control")
),
child:Material(
child: Container(
margin: EdgeInsets.only(top:100, left: 20, right: 20),
//width: double.infinity,
child:Column(
children: [
Container(
width:double.infinity,
//height: MediaQuery.of(context).size.height*0.10,
child: CupertinoSlidingSegmentedControl(
children: children,
onValueChanged: (value)
{
selectedValue = value;
setState(() {
});
},
groupValue: selectedValue,
thumbColor: CupertinoColors.activeGreen,
backgroundColor: CupertinoColors.white,
),
),
SizedBox(
height:50,
),
Container(
height: MediaQuery.of(context).size.height*0.50,
width: double.infinity,
child: Image.asset(images[selectedValue],
fit: BoxFit.cover),
)
],
),
),
),
);
}
}
Output:
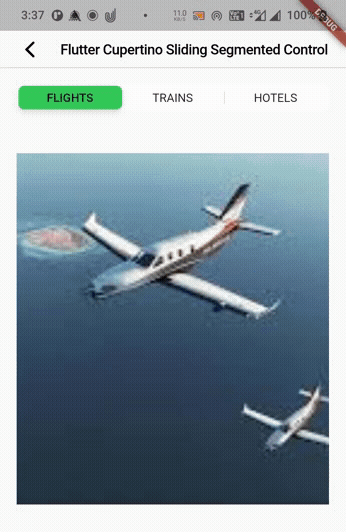
I hope you understand the tutorial on how to create & use cupertino sliding segmented control in flutter. We have also seen an example where we have used the CupertinoSlidingSegmentedControl widget and customized its style. Let’s catch up with some other widget in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official documentation.

Leave a Reply