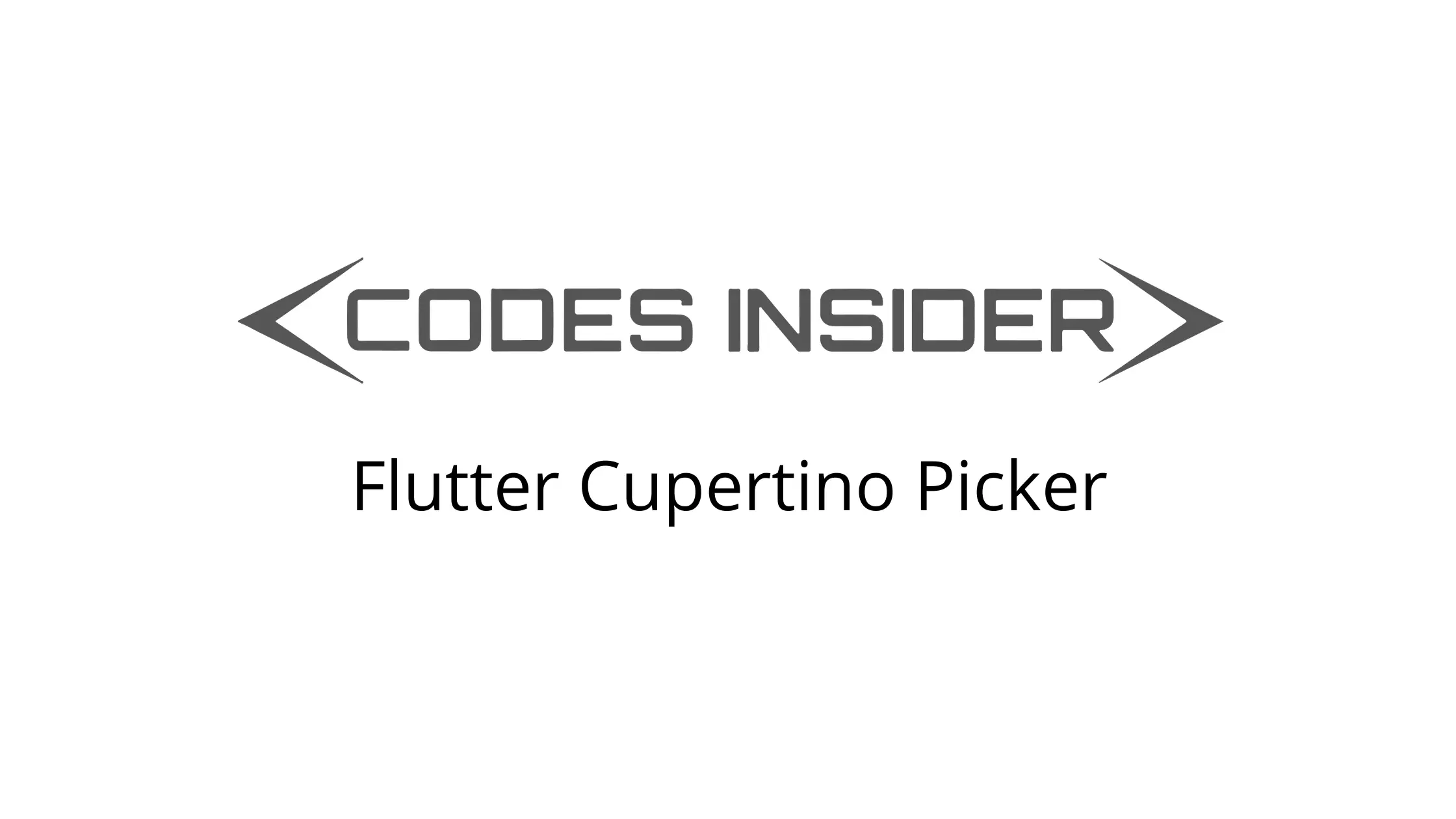
Flutter Cupertino Picker
Cupertino Picker in flutter is nothing but an ios like (style) picker similar to a dropdown menu. CupertinoPicker widget lets us create a dropdown menu in ios style. It displays a list and lets the user scroll to select an item from that list. Each time the user selects an item the callback function of onSelectedItemChanged property will invoke. We can get the value selected by the user inside this function. To display a cupertino picker we have to use it as a child of showCupertinoModalPopup or showCupertinoDialog function.
Don’t know what Cupertino is? it is nothing but a set of flutter widgets that follow the ios design pattern. These widgets are designed to implement ios features in flutter apps built for the ios platform.
In this tutorial, we will learn how to use cupertino picker in flutter with example. We will also learn how to customize the CupertinoPicker widget using different properties.
To implement a similar widget for a cupertino picker in android consider using dropdown menu in flutter.
How To Create Cupertino Picker In Flutter?
To create a cupertino picker in flutter we have to call the constructor of CupertinoPicker Class and provide the required properties. The cupertino picker has three required properties itemExtent, onSelectedItemChanged, and children. The itemExtent will accept a double as value. The onSelectedItemChanged property will accept a callback function as a value. This function will invoke every time the user selects an option. We can get the user selection inside this function. The children property will accept a list of widgets as value. Generally, we will use text widgets as children.
Generally, we have to display a cupertino picker when an event is triggered. For example on click of a button or any other widget. To display a picker we have to use showCupertinoModalPopup function or showCupertinoDialog function. We have to return the cupertino picker to the builder property of the above functions.
Flutter Cupertino Picker Constructor:
CupertinoPicker(
{Key? key,
double diameterRatio,
Color? backgroundColor,
double offAxisFraction,
bool useMagnifier,
double magnification,
FixedExtentScrollController? scrollController,
double squeeze,
required double itemExtent,
required ValueChanged<int>? onSelectedItemChanged,
required List<Widget> children,
Widget? selectionOverlay,
bool looping = false}
)
Flutter Cupertino Picker Properties
The properties of a cupertino picker are:
- diameterRatio
- backgroundColor
- offAxisFraction
- useMagnifier
- magnification
- scrollController
- squeeze
- itemExtent
- onSelectedItemChanged
- children
- selectionOverlay
- looping
children
This is a required property. It takes a list of widgets as value and displays them in the picker. Generally, we will use text widgets for this.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
)
Output:
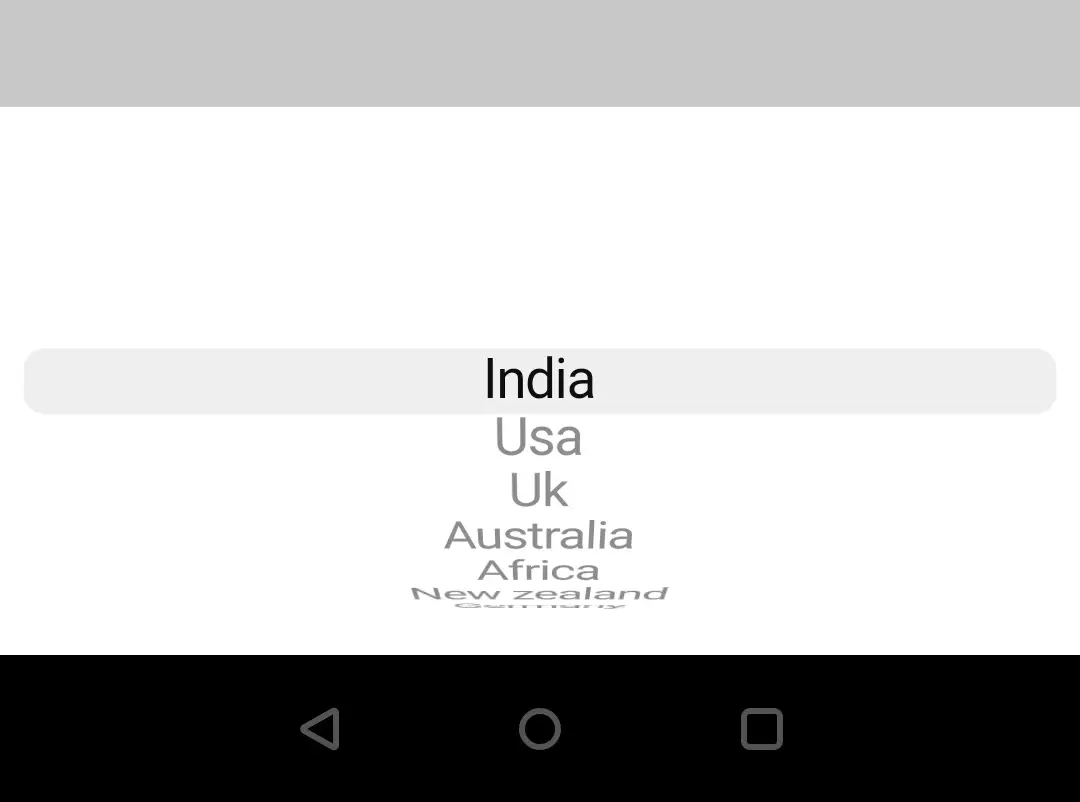
itemExtent
To increase or decrease hight for each item of the picker we will use this property. It takes double as value.
CupertinoPicker(
children: [
],
onSelectedItemChanged: (value){
....
},
itemExtent: 45,
)
Output:
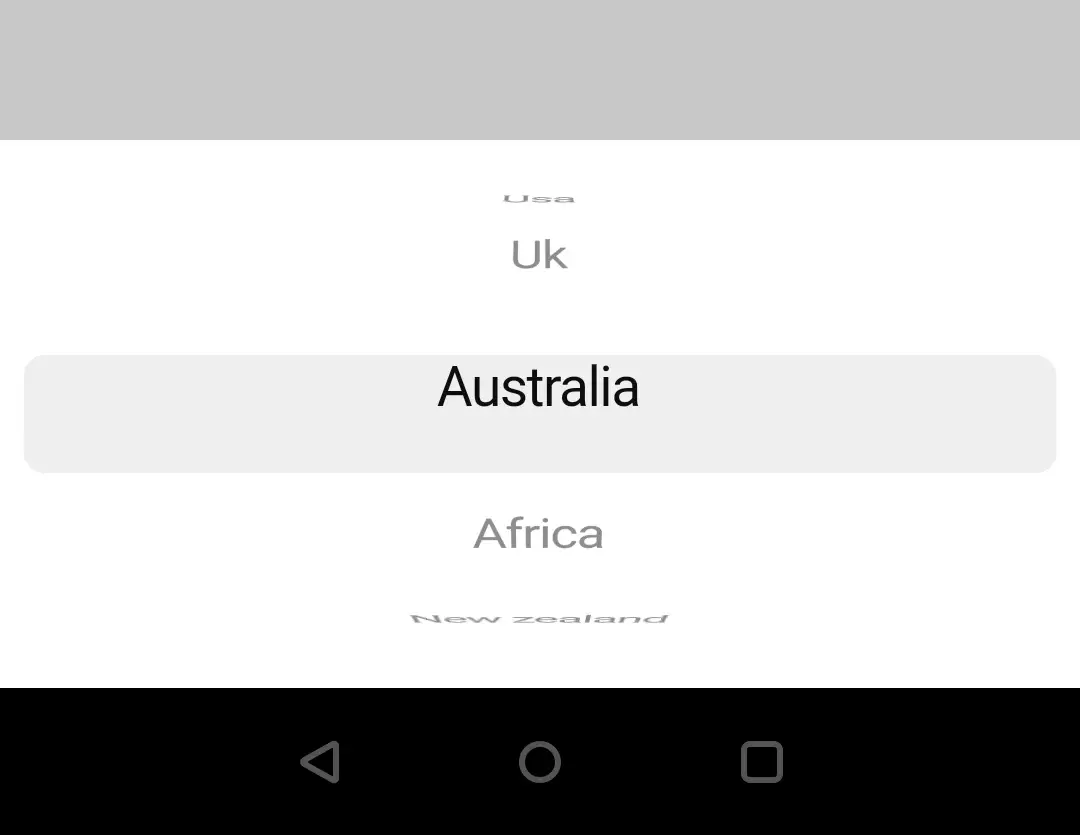
onSelectedItemChanged
This property takes a callback function which will invoke every time the user changes the selection. We will get the index of user selected item inside this function.
CupertinoPicker(
children:[]
onSelectedItemChanged: (value){
},
itemExtent: 45,
)
diameterRatio
We will use this property to define the ratio between the diameter of the cylinder that will scroll and the height of the picker. It takes double as value. By default, the value is 1.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
)
Output:
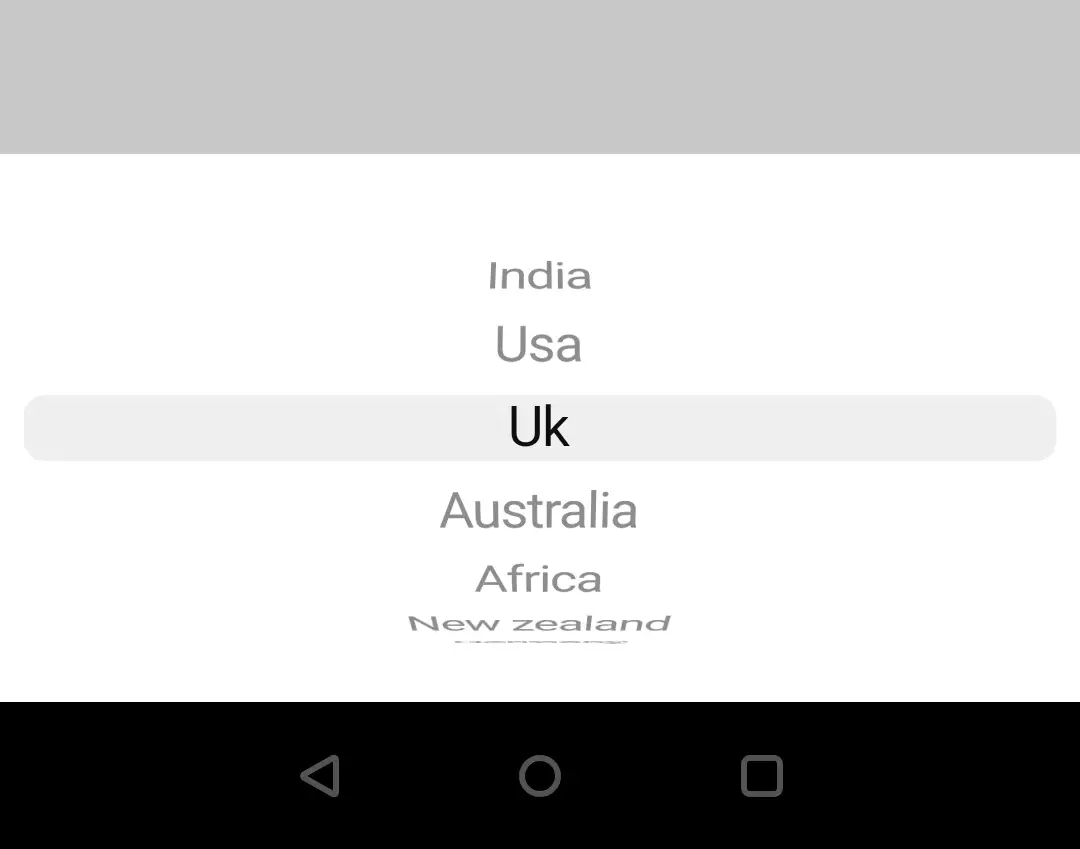
backgroundColor
We will use this property to add or change the background color of the picker. It takes CupertinoColors or Colors constant as value. If you want to use a custom color use a color class constructor.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
backgroundColor:CupertinoColors.activeGreen,
)
Output:
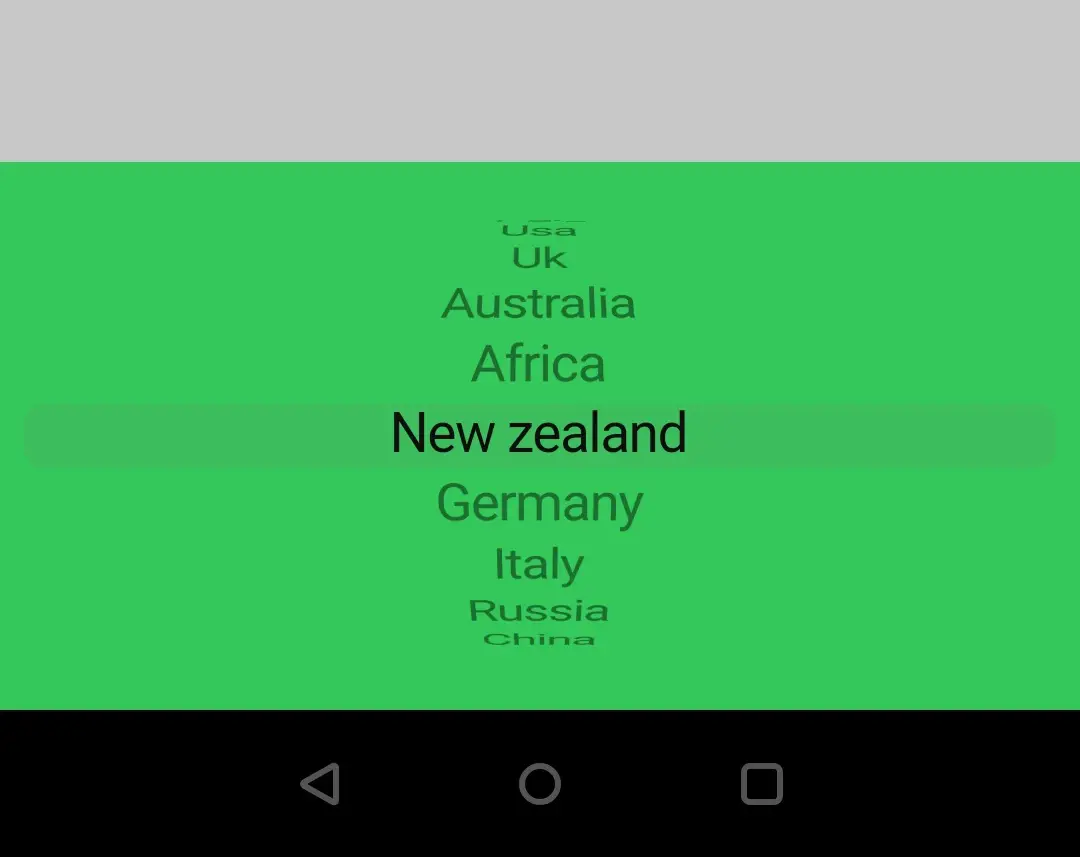
offAxisFraction
The horizontal off-center fraction of the width of the wheel. This creates a side view of a wheel that is scrolling vertically where the options will curve to one side. It takes double as value. By default, the value is 0.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
offAxisFraction: 0.5
)
Output:
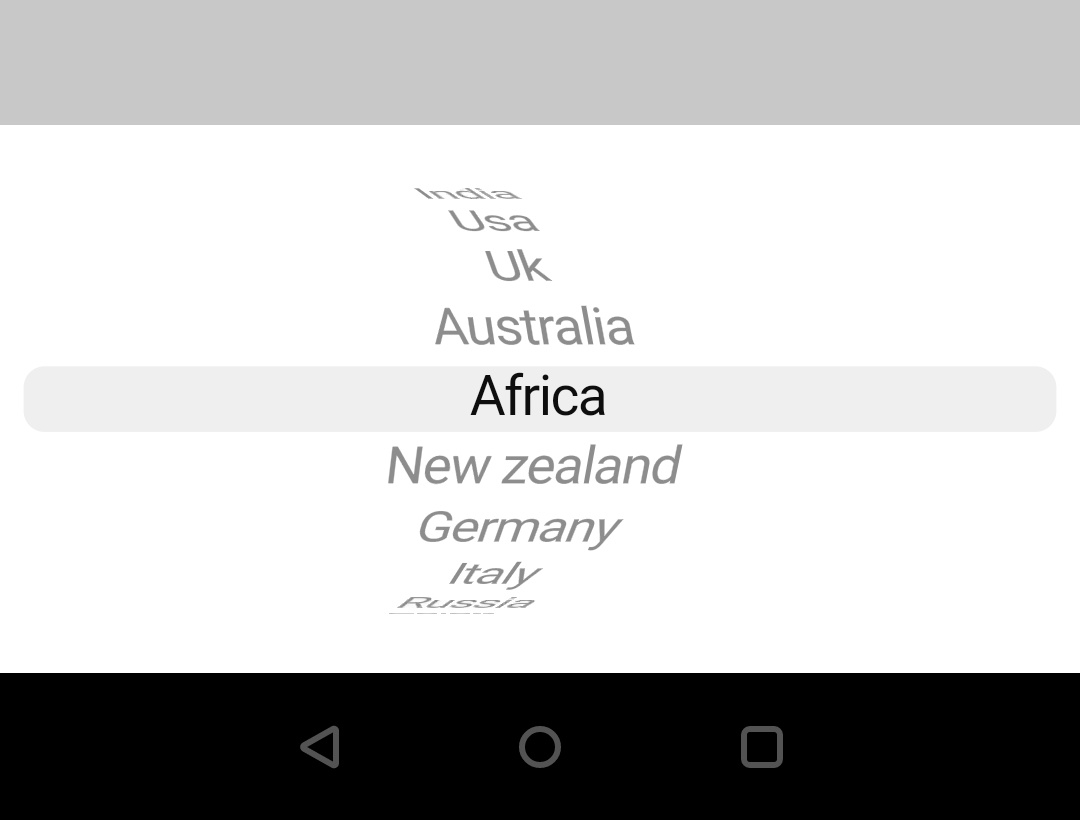
useMagnifier
It takes a boolean as a value. If we want to magnify the selection we have to set this property to true.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
useMagnifier: true,
)
magnification
We will use this property to define the amount of magnification we want to apply. It works only when the useMagnifier property is set to true. It takes double as value.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
useMagnifier: true,
magnification: 1.3,
)
Output:
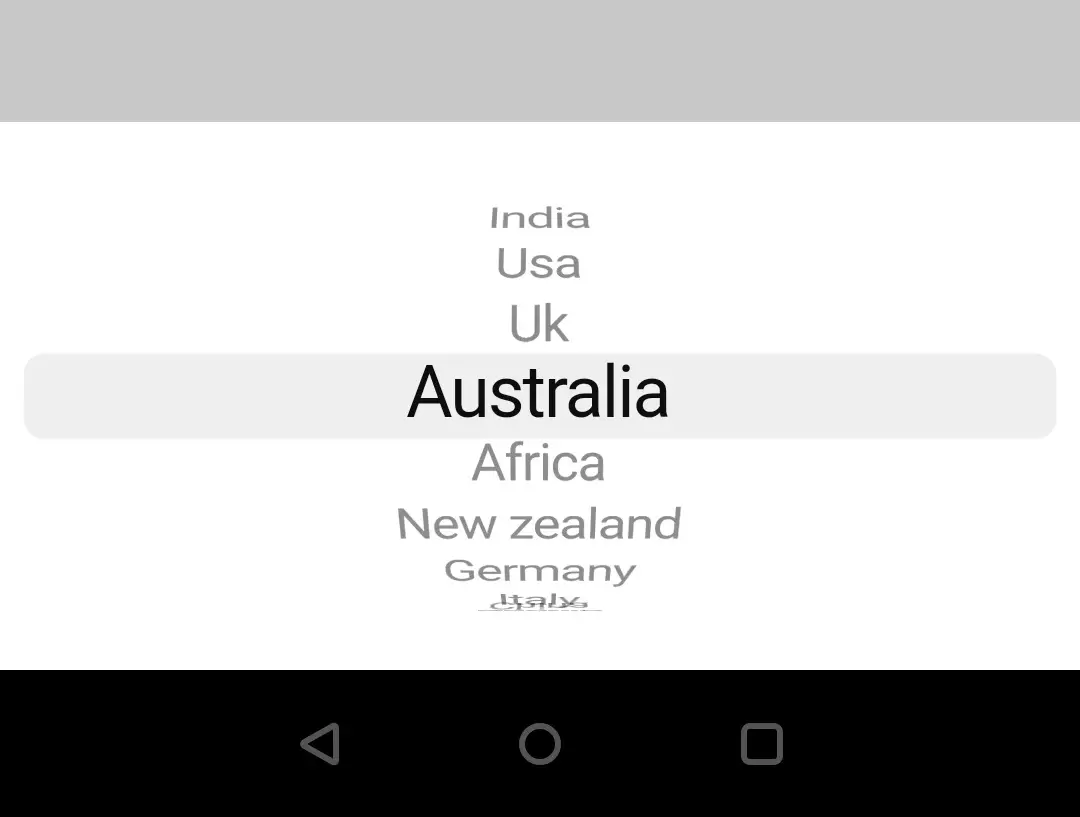
squeeze
We will use this property to display more children in the same viewport of the wheel by squeezing the children. If the height of the viewport of a wheel is 200px and itemExtent is 20px, 10 items would fit on a flat list whose size is also 200px. With a squeeze of 2, 20 items would be shown in the viewport of the wheel.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
useMagnifier: true,
magnification: 1.3,
squeeze: 2
)
Output:
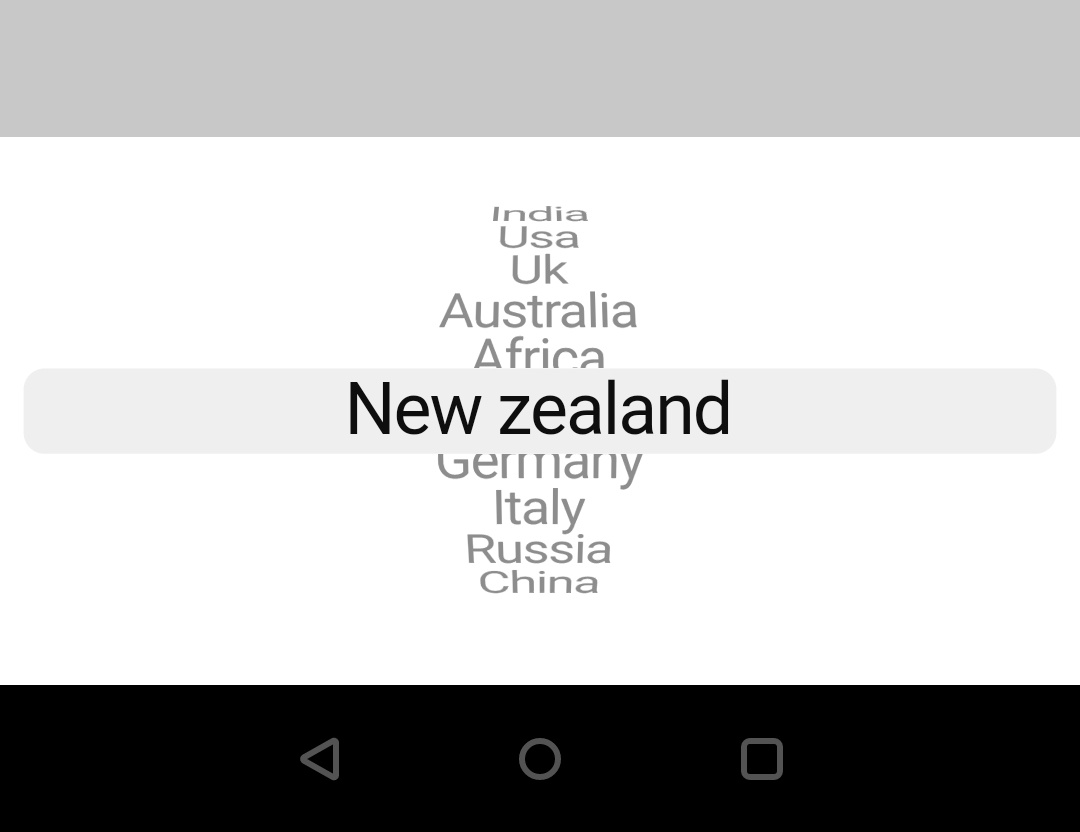
looping
It takes boolean as value. If we want to loop the items when the list reaches the end we have to set this property to true. By default it is false.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
useMagnifier: true,
magnification: 1.3,
looping: true,
)
Output:
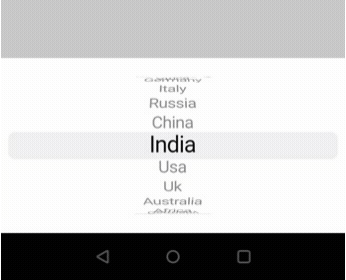
selectionOverlay
If we want to display an overlay for the selection we have to use this property.
CupertinoPicker(
children: [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
],
onSelectedItemChanged: (value){
},
itemExtent: 25,
diameterRatio:1,
useMagnifier: true,
magnification: 1.3,
looping: true,
selectionOverlay: CupertinoPickerDefaultSelectionOverlay(
background: CupertinoColors.tertiaryLabel,
),
)
Output:
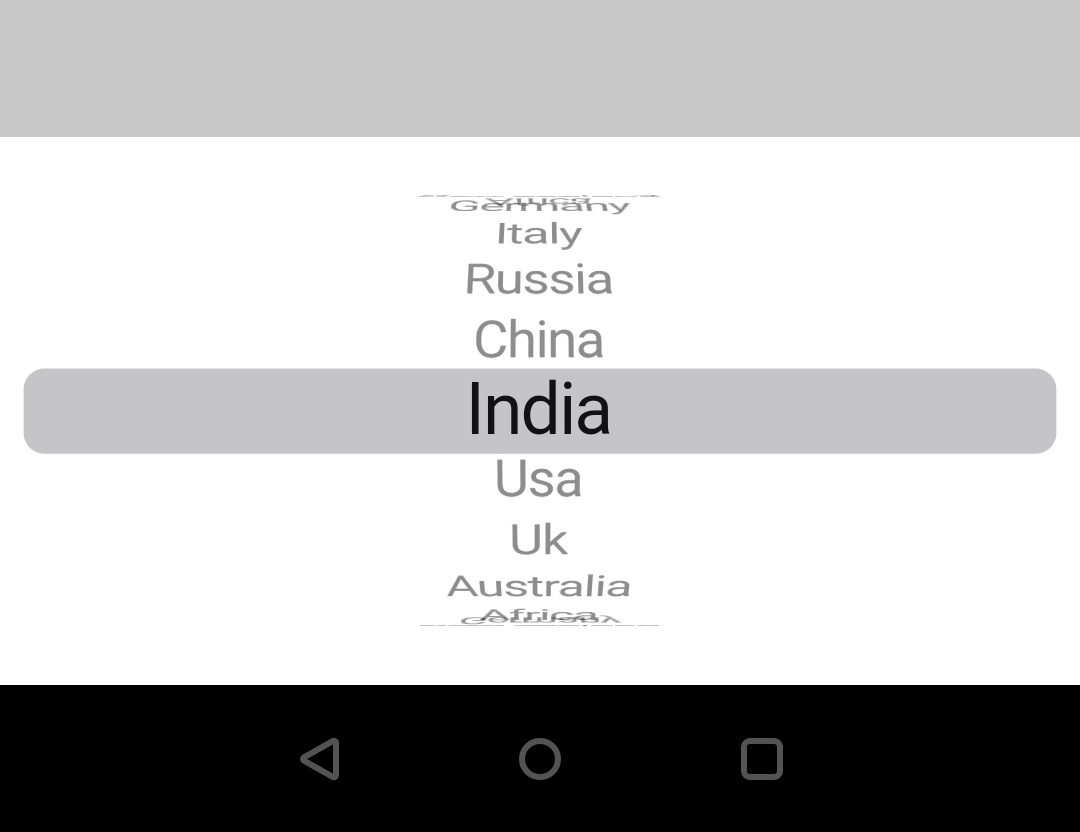
Flutter Cupertino Picker Example
Let’s create an example where we will implement a cupertino picker in flutter using the CupertinoPicker widget. In this example, we will display a button and a text widget. Clicking on the button will display the cupertino picker. When the user selects an item we will display it in the text widget.
First, let’s declare a variable for saving the user selection.
String selectedValue = "";
Now let’s create a List (countries) of type widget that we will use for children property. Inside this list, we will have text widgets as children.
List <Widget> countries = [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
];
Display the button and the text widget. We will provide the text widget with the variable (selectedValue) created at the beginning of the example. So for the first time, it will display nothing. When the user changes the selection it will get updated.
child:Container(
margin: EdgeInsets.all(40),
width: double.infinity,
height: MediaQuery.of(context).size.height*0.50,
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
ElevatedButton(
child: Text("Show Picker"),
onPressed: (){
showPicker();
},
),
Material(
child: Text(
selectedValue,
style: TextStyle(
fontSize: 18
),
),
),
]
)
),
Create a function (showPicker() ) inside which we will call showCupertinoModalPopup function that will display the picker. We will set the above list as children for the picker. To update the UI with the user selection we will get the index of selection inside the onSelectedItemChanged callback function. Using this index we will get the value from the list (countries) and update the text widget with that value.
void showPicker()
{ showCupertinoModalPopup(
context: context,
builder: (BuildContext builder) {
return Container(
height: MediaQuery.of(context).copyWith().size.height*0.25,
color: Colors.white,
child: CupertinoPicker(
children: countries,
onSelectedItemChanged: (value){
Text text = countries[value];
selectedValue = text.data.toString();
setState(() {
});
},
itemExtent: 25,
diameterRatio:1,
useMagnifier: true,
magnification: 1.3,
looping: true,
)
);
}
);
Complete Code
Let’s sum up all the code snippets to complete the cupertino picker example in flutter. Create a new flutter project and replace the code in main.dart file with the code below.
import 'dart:async';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
List <Widget> countries = [
Text("India"),
Text("Usa"),
Text("Uk"),
Text("Australia"),
Text("Africa"),
Text("New zealand"),
Text("Germany"),
Text("Italy"),
Text("Russia"),
Text("China"),
];
String selectedValue = "";
@override
Widget build(BuildContext context) {
return new CupertinoPageScaffold(
navigationBar:CupertinoNavigationBar(
leading: CupertinoNavigationBarBackButton(
onPressed: ()
{
},
color: CupertinoColors.label,
),
middle: Text("Profile"),
),
child:Container(
margin: EdgeInsets.all(40),
width: double.infinity,
height: MediaQuery.of(context).size.height*0.50,
//alignment: Alignment.topCenter,
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
ElevatedButton(
child: Text("Show DatePicker"),
onPressed: (){
showPicker();
},
),
Material(
child: Text(
selectedValue,
style: TextStyle(
fontSize: 18
),
),
),
]
)
),
);
}
void showPicker()
{ showCupertinoModalPopup(
context: context,
builder: (BuildContext builder) {
return Container(
height: MediaQuery.of(context).copyWith().size.height*0.25,
color: Colors.white,
child: CupertinoPicker(
children: countries,
onSelectedItemChanged: (value){
Text text = countries[value];
selectedValue = text.data.toString();
setState(() {
});
},
itemExtent: 25,
diameterRatio:1,
useMagnifier: true,
magnification: 1.3,
looping: true,
)
);
}
);
}
}
Output:
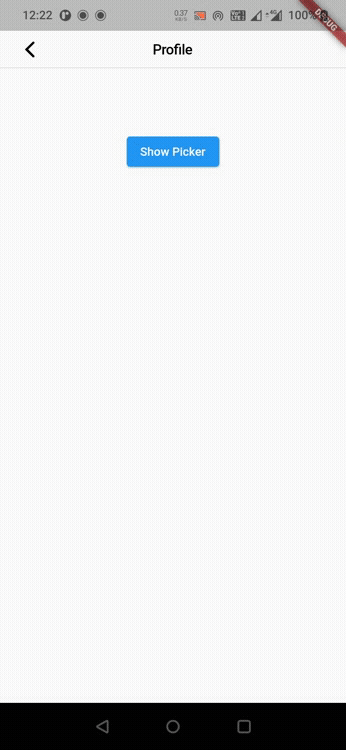
That brings an end to the tutorial on how to create & use cupertino picker in flutter. We have also seen an example where we have used the CupertinoPicker widget to display the picker. Let’s catch up with some other widget in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official Documentation.

Leave a Reply