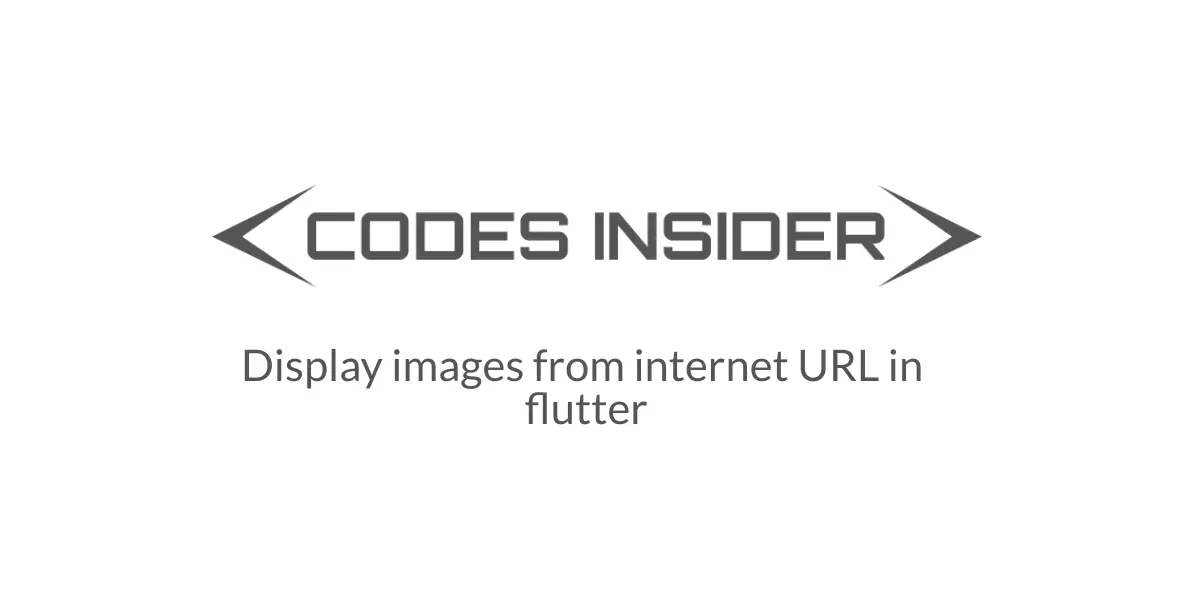
In this tutorial, we will learn how to display image from the internet URL in the flutter app. To display network images, flutter has a built-in method Image.network().
Displaying images in apps has become a basic feature nowadays. We have already seen how to add images in flutter app, in our previous article. In this article, we will display an image from the internet in our app using the Image.network() method.
Displaing image from internet url in flutter
Simply displaying image
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Flutter Demo Home Page"),
),
body: Container(
width: double.infinity,
child: Image.network('https://picsum.photos/250?image=9')
), //
),
);
}
}
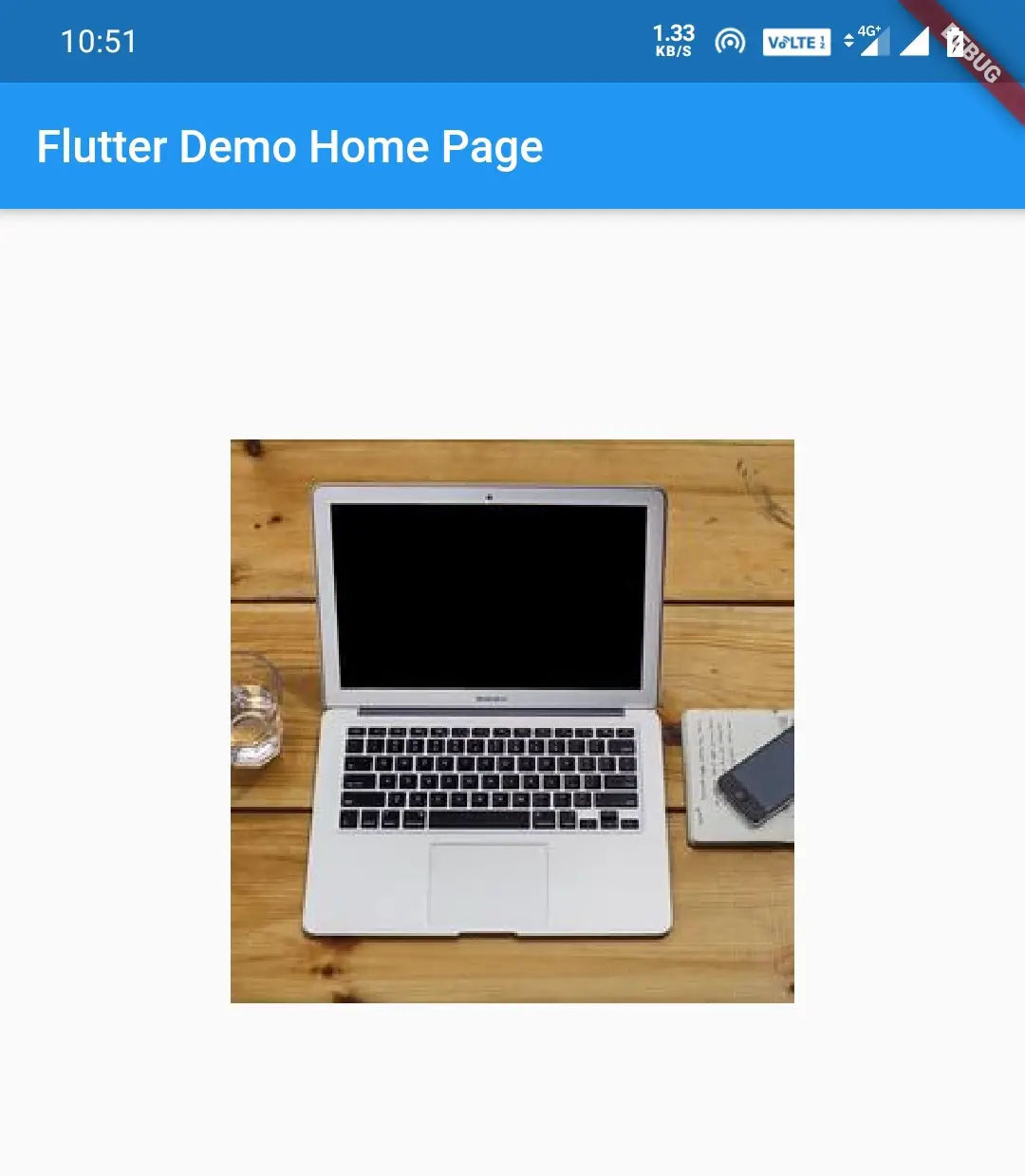
List of arguments for Image.network()
Flutter Image.network() method supports multiple arguments, so let’s see them below.
- semanticLabel: Semantic description of the image.
- excludeFromSemantics: Boolean value which indicates whether to exclude the image from semantics.
- scale: To define the amount of scaling.
- width: To define the width of the image.
- height: To define the height of the image.
- color: Blends each image pixel with the defined color, depending on the colorBlendMode.
- colorBlendMode: How the color is blended with the image.
- fit: How to fit the image in the allocated space.
- alignment: How to align the image.
- repeat: To paint the area not covered by the image.
- centerSlice: The center slice for a nine patch image.
- matchTextDirection: Whether to pain the image in the direction of text.
- gaplessPlayBack: Boolean value whether to show old image when the image provider changes.
- filterQuality: Quality of image filter.
width and height
Let’s define width and height for the image.
Image.network('https://picsum.photos/250?image=9', width:100, height:100)
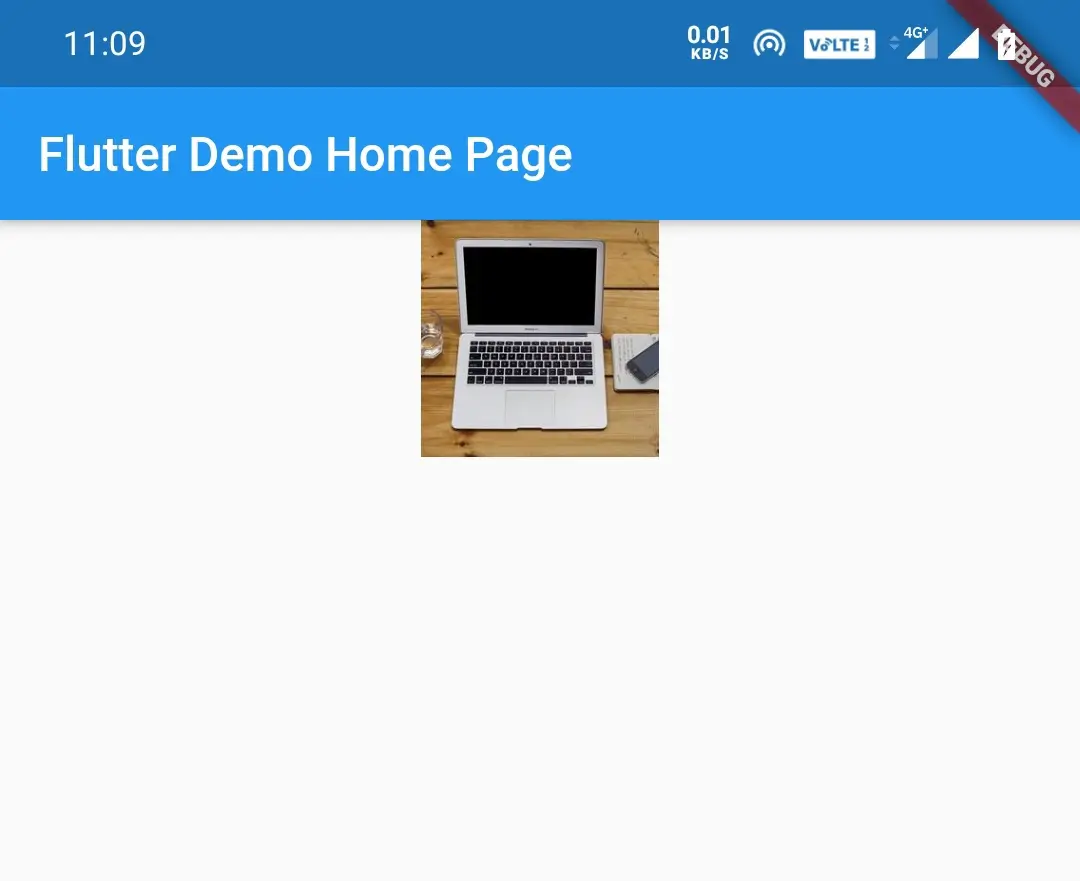
Fit
The fit argument has multiple properties, here I’m using fit attribute with the cover property. Try the rest for yourself to know the difference.
Image.network('https://picsum.photos/250?image=9' ,fit: BoxFit.cover)
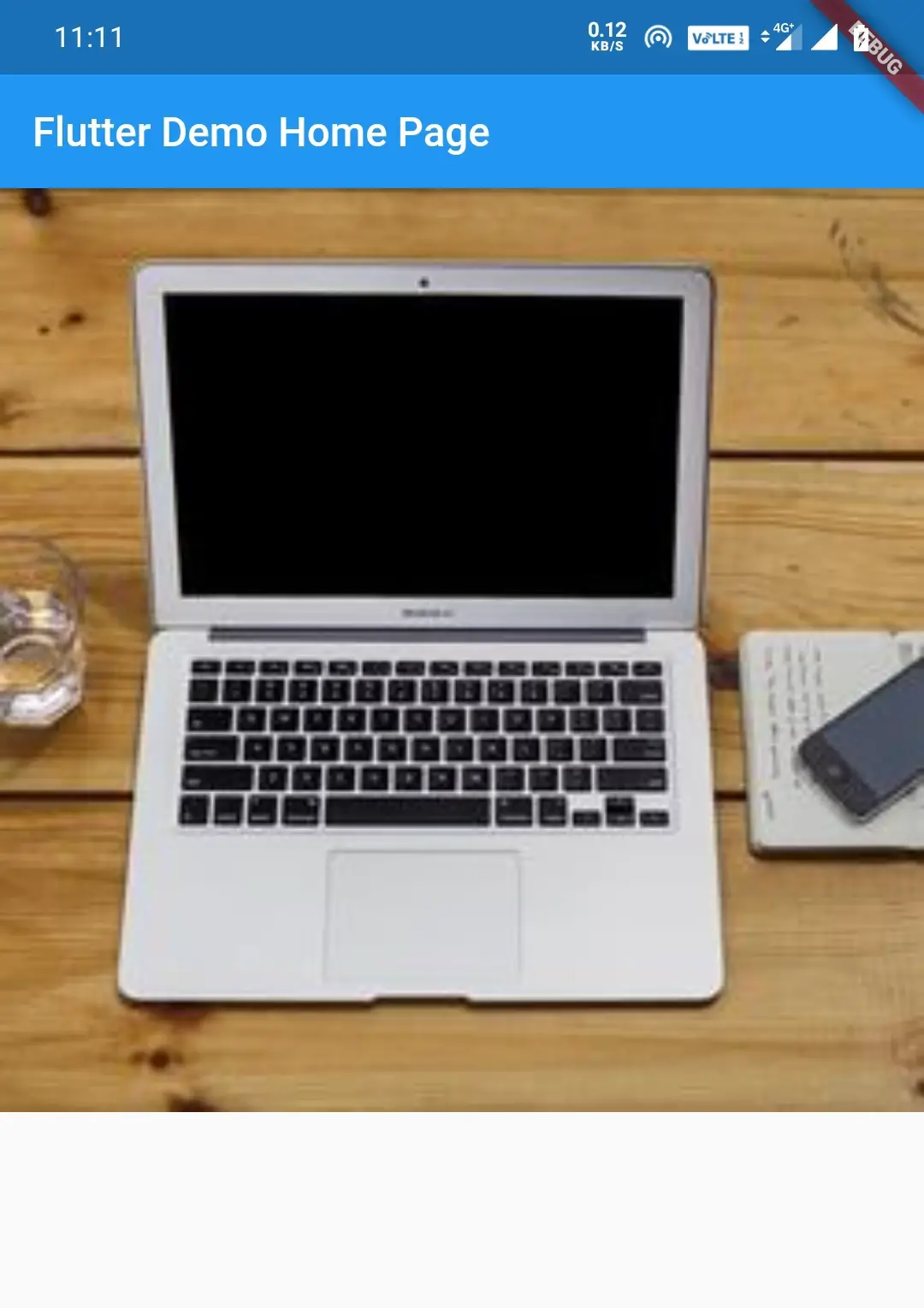
Check out the rest of the arguments and their properties of Image.network() method to know them in detail.
That’s all about how to display image from internet URL in flutter app. Let’s catch up with some other cool flutter tutorials in the next articles.
Do like and share if you find this post helpful. Thank you!!
Reference: Flutter Official Documentation.

Leave a Reply