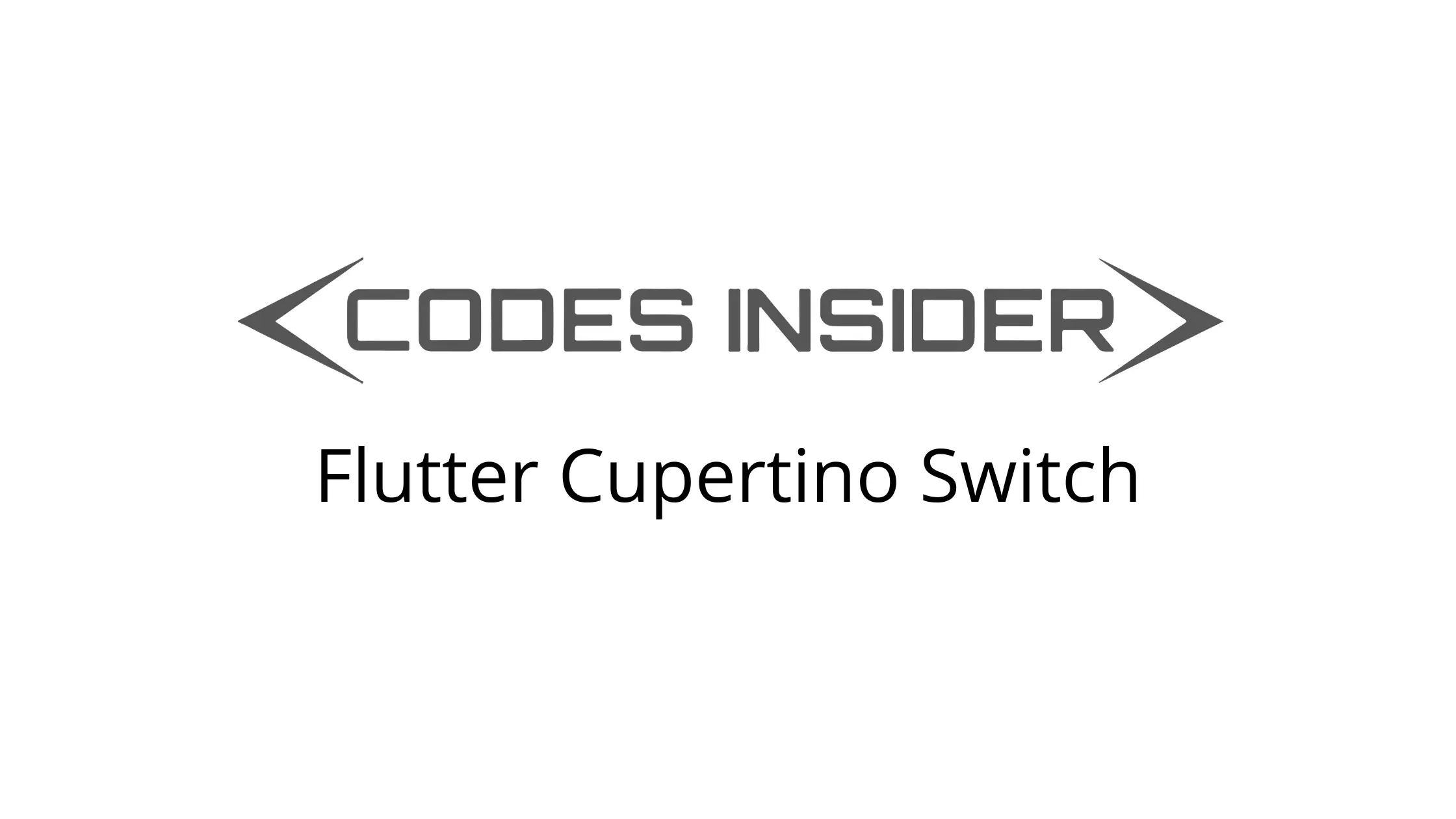
Flutter Cupertino Switch
Cupertino switch is nothing but ios style switch in flutter. CupertinoSwitch lets us add an ios style switch in our application. A switch is nothing but a button with two states ON and OFF. It is used to toggle between ON and OFF where true represents ON and false represents OFF. Whenever there is change in the state of the switch the onChanged callback will invoke. It will return the updated state which is true or false. So based on the value returned by the callback we have to update the UI and perform some acction. General use case of a switch is turning wifi or bluetooth on/off using switch in settings of mobile device.
Don’t know what Cupertino is? It is nothing but a set of flutter widgets that follow the ios design pattern. These widgets are designed to implement ios features in flutter apps built for the ios platform.
In this tutorial, we will learn how to use cupertino switch in flutter with example. We will also learn how to customize the style of CupertinoSwitch widget using different properties.
To implement switch in android platform using flutter refer switch in flutter.
How To Create Cupertino Switch In Flutter?
To create a cupetino switch in flutter we have to use CupertinoSwitch class. Call the constructor of the class and provide the required properties. The switch has two required properties value and onChanged. The value will indicate the state of the switch. The onChanged callback is used to update the value when the user changes the switch state.
Flutter Cupertino Switch Constructor:
CupertinoSwitch(
{Key? key,
required bool value,
required ValueChanged<bool>? onChanged,
Color? activeColor,
Color? trackColor,
Color? thumbColor,
DragStartBehavior dragStartBehavior}
)
Flutter Cupertino Switch Properties
The properties of a flutter cupertino switch are:
- value
- onChanged
- activeColor
- trackColor
- thumbColor
- dragStartBehavior
value
It takes boolean as value. This property indicates whether the switch is in ON state or OFF state. If we provide a static value for this property the UI will not be updated when the user turns the switch on/off. So we have to update this value dynamically when the user changes the state.
CupertinoSwitch(
value:true,
onChanged: (value){
},
),
Output:
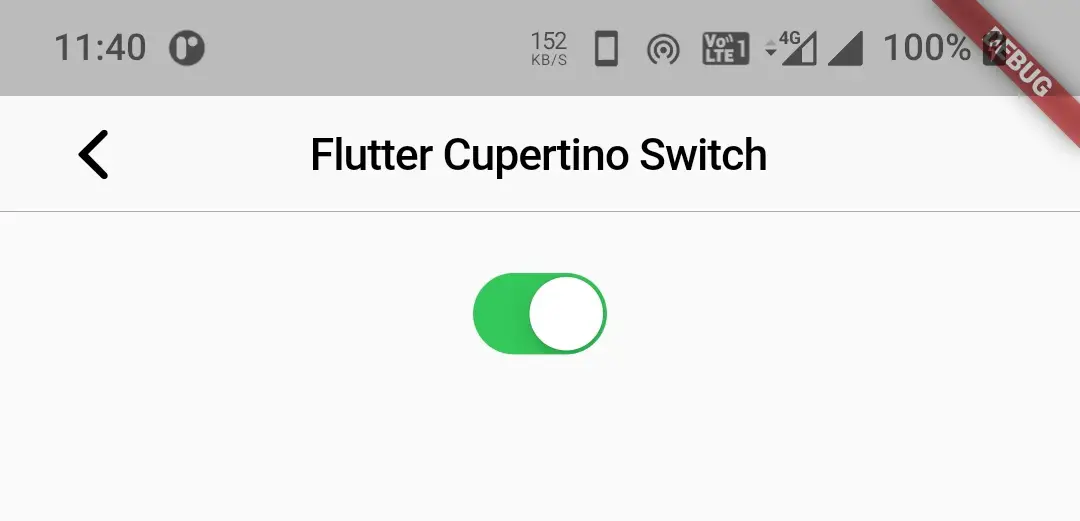
onChanged
It takes a callback function as value. This function will invoke every time the user turns the switch on/off. This function will return the updated value. We will assign the updated value to the value property using a global variable. To update the UI with the latest position we have to call setSatete() inside the onChanged function.
CupertinoSwitch(
value:state,
onChanged: (value){
state = value;
setState(() {
});
},
),
activeColor
We will use this property to change the color of the track when the switch is turned on. It will take Colors or CupertinoColors constant as value. By default it is green color.
CupertinoSwitch(
value:state,
onChanged: (value){
state = value;
setState(() {
});
},
activeColor: CupertinoColors.activeOrange,
),
Output:
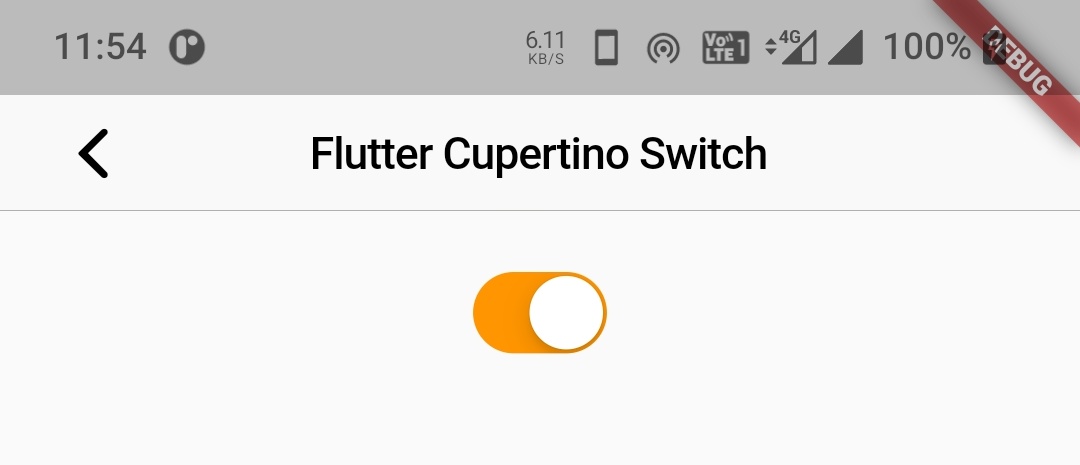
trackColor
We will use this property to change the track color. The track color will only appear when the switch is in OFF state. When it is in ON state the active color will appear.
CupertinoSwitch(
value:state,
onChanged: (value){
state = value;
setState(() {
});
},
activeColor: CupertinoColors.activeOrange,
trackColor: CupertinoColors.systemBlue,
),
Output:
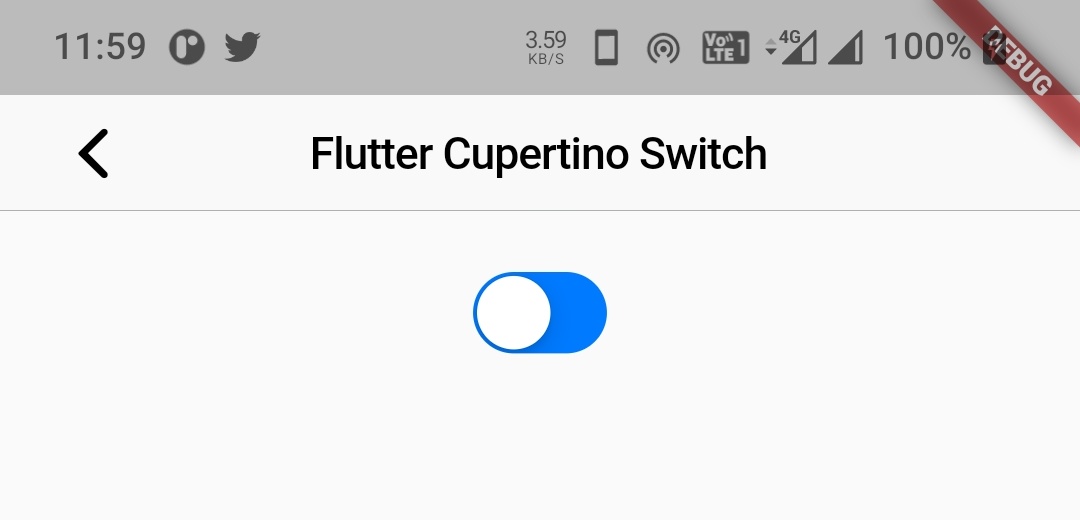
thumbColor
We will use this property to change the thumb color of the switch.
CupertinoSwitch(
value:state,
onChanged: (value){
state = value;
setState(() {
});
},
thumbColor: CupertinoColors.activeOrange,
),
Output:
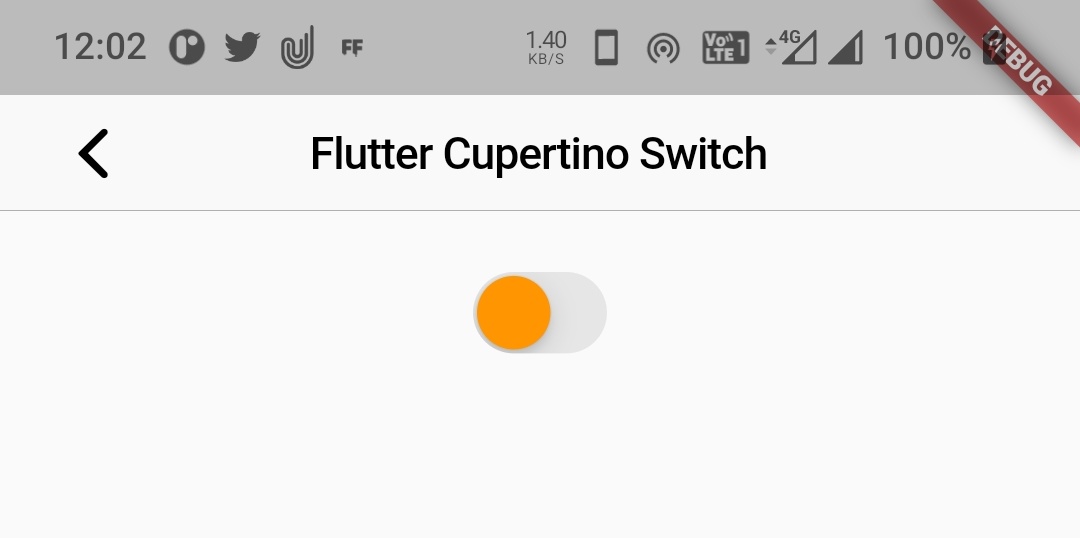
Flutter Cupertino Switch Example
Let’s create an example where we will implement cupertino switch in flutter using the CupertinoSwitch widget. In this example, we will display a settings menu with wifi option and a switch to enable or disable it. We will also display a message when the user turns the switch ON and OFF.
First, let’s declare a boolean variable to hold the state of the switch. It is false initially.
bool state = false;
Now we will implement the code for displaying the wifi option and switch to turn it on/off.
Row(
children: [
SizedBox(
width: MediaQuery.of(context).size.width*0.75,
child: Text("Wifi", style: TextStyle(fontWeight: FontWeight.bold),),
),
SizedBox(
width: MediaQuery.of(context).size.width*0.15,
child: CupertinoSwitch(
value:state,
onChanged: (value){
state = value;
setState(() {
});
},
),
),
],
),
Let’s add the code for displaying the text when the switch is turned on/off.
Text(state == true? "Wifi turned on": "Wifi turned off",
style:TextStyle(
fontWeight: FontWeight.bold,
color: state == true? CupertinoColors.activeGreen : CupertinoColors.destructiveRed
),
),
Complete Code:
Let’s sum up all the code snippets above to complete our cupertino switch example in flutter. Create a new flutter project and replace the code in main.dart file with the code below.
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
bool state = false;
@override
Widget build(BuildContext context) {
return new CupertinoPageScaffold(
navigationBar:CupertinoNavigationBar(
leading: CupertinoNavigationBarBackButton(
onPressed: ()
{ },
color: CupertinoColors.label,
),
middle: Text("Flutter Cupertino Switch")
),
child:Material(
child: Container(
margin: EdgeInsets.only(top:100, left: 20, right: 20),
width: double.infinity,
child:Column(
children: [
Container(
width: double.infinity,
child: Row(
children: [
SizedBox(
width: MediaQuery.of(context).size.width*0.75,
child: Text("Wifi", style: TextStyle(fontWeight: FontWeight.bold),),
),
SizedBox(
width: MediaQuery.of(context).size.width*0.15,
child: CupertinoSwitch(
value:state,
onChanged: (value){
state = value;
setState(() {
});
},
),
),
],
),
),
SizedBox(
height: 20,
),
Divider(
height: 1,
color: CupertinoColors.systemGrey5,
),
SizedBox(
height:30
),
Text(state == true? "Wifi turned on": "Wifi turned off",
style:TextStyle(
fontWeight: FontWeight.bold,
color: state == true? CupertinoColors.activeGreen : CupertinoColors.destructiveRed
),
),
],
)
),
),
);
}
}
Output:
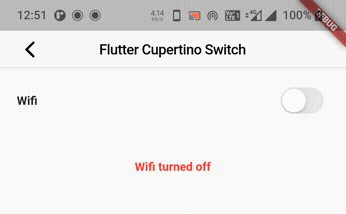
That brings an end to the tutorial on how to create & use cupertino switch in flutter. We have also seen an example where we have used the CupertinoSwitch widget and customized its style. Let’s catch up with some other widgets in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official Documentation.

Leave a Reply