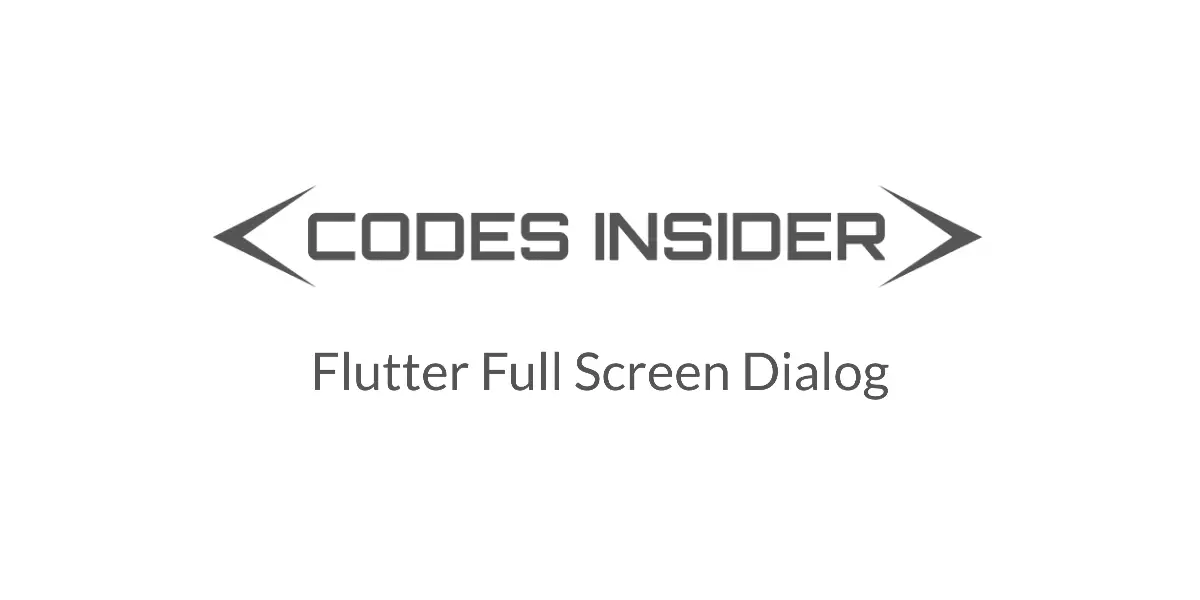
In this tutorial, you will learn how to use full screen dialog in flutter with example. We will also customize its style with different properties.
Flutter Full Screen Dialog
Full screen dialog in flutter is nothing but a general dialog occupying the entire screen. Generally, we will use full screen dialog in situations like editing content. For example, if we want to edit a user profile we can use it. In flutter, there is no specific widget available for displaying a full screen dialog. So we can’t display it using showDialog method, since it only accepts an object of type dialog.
If you want to display normal dialog consider using flutter simpleDialog.
Creating Full screen Dialog
To create a full screen dialog in flutter we have two ways
- Using showGeneralDialog()
- Using MaterialPageRoute
Using showGeneralDialog()
We can create and display a full screen dialog in flutter using showGeneralDialog function. First, let’s see the function.
showGeneralDialog function:
Future<T?> showGeneralDialog<T extends Object?>(
{required BuildContext context,
required RoutePageBuilder pageBuilder,
bool barrierDismissible = false,
String? barrierLabel,
Color barrierColor = const Color(2147483648),
Duration transitionDuration = const Duration(milliseconds: 200),
RouteTransitionsBuilder? transitionBuilder,
bool useRootNavigator = true,
RouteSettings? routeSettings}
)
Let’s see the important properties of the showGeneralDialog function.
- pageBuilder
- barrierDismissible
- barrierColor
- transitionDuration
- transitionBuilder
Full Screen Dialog Example Complete Code Using ShowGeneralDialog
Now let’s design the UI where we will display a button clicking on which will display the full screen dialog. The dialog will have a text widget and a single button which will dismiss the dialog when the user clicks it. Let’s create a function _displayDialog where we will have the showGenerelDialog function. When the user clicks the button we will call the _displayDialog method that will call the showGenerealDialog function which displays the full screen dialog.
import 'dart:io';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter Full Screen Dialog"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
_displayDialog(context);
},
child: Text("Show Dialog"),
),
],
),
),
);
}
_displayDialog(BuildContext context) {
showGeneralDialog(
context: context,
barrierDismissible: false,
transitionDuration: Duration(milliseconds: 2000),
transitionBuilder: (context, animation, secondaryAnimation, child) {
return FadeTransition(
opacity: animation,
child: ScaleTransition(
scale: animation,
child: child,
),
);
},
pageBuilder: (context, animation, secondaryAnimation) {
return SafeArea(
child: Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
padding: EdgeInsets.all(20),
color: Colors.white,
child: Center(
child:Column(
mainAxisSize: MainAxisSize.min,
children:<Widget> [
Text('Hai This Is Full Screen Dialog', style: TextStyle(color: Colors.red, fontSize: 20.0),),
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("DISMISS",
style: TextStyle(color: Colors.white),
),
)
],
),
),
),
);
},
);
}
}
Output:
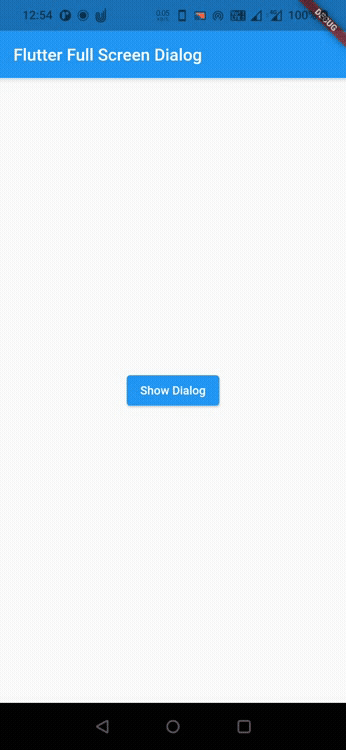
If you want to display a transparent background just use the below property for showGeneralDialog function and remove the color property of the container in the above code.
barrierColor: Colors.black.withOpacity(0.9)
Output:
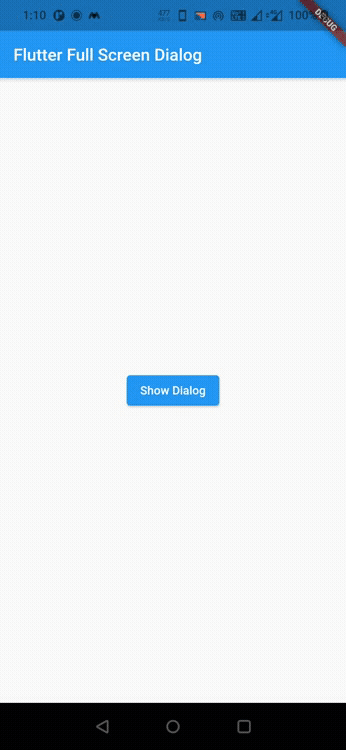
Using MaterialPageRoute
This is another way of creating and displaying a full screen dialog. In this method, we will use the materialPageRoute class. Let’s see the constructor of the materialPageRoute class.
Constructor:
MaterialPageRoute({
required WidgetBuilder builder,
RouteSettings? settings,
bool maintainState,
bool fullscreenDialog = false
})
The materialPageRoute has two important properties
- builder: It should return an object of type widget so we will design a custom full screen dialog and return it.
- fullscreenDialog: This property is used to notify the navigator whether the page is a new page or a full screen dialog. Setting this property to true will display a close icon (‘X’) on the appBar, while setting to false will display a back arrow icon(‘<-‘).
Full Screen Dialog Example Complete Code Using MaterialPageRoute
The UI will be the same but the full screen dialog UI will change in this example. We will return a scaffold widget as a full screen dialog to the builder property of the materialPageRoute. Also, we will set the fullscreenDialog property to true which will display the ‘X’ icon on the app bar.
We will display the dialog using Navigator like below
Navigator.of(context).push()
Let’s create a function _openDialog() where we will put all our code inside this function and lets make this function async and receive the user chosen result into a variable. In this function, we are returning the scaffold widget as full screen dialog to materialPageRoute and pushing it using Navigator’s push() method.
_openDialog function:
void _openDialog() async {
_selected = await Navigator.of(context).push(new MaterialPageRoute<String>(
builder: (BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: const Text('Full Screen Dialog'),
actions: [
new ElevatedButton(
onPressed: () {
Navigator.of(context).pop("hai");
},
child: new Text('ADD',
style: TextStyle(color: Colors.white),
)),
],
),
body: Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
padding: EdgeInsets.all(20),
color: Colors.white,
child: Column(
children: [
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('Full Screen',
style: TextStyle(color: Colors.white),
),
)
],
),
),
);
},
fullscreenDialog: true
));
if(_selected != null)
setState(() {
_selected = _selected;
});
}
Complete Code Example:
import 'dart:io';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
//import 'widgets/add_entry_dialog.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
var _selected ="";
var _test = "Full Screen";
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter Full Screen Dialog"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
_openDialog();
},
child: Text("Show Dialog"),
),
Text(_selected)
],
),
),
);
}
void _openDialog() async {
_selected = await Navigator.of(context).push(new MaterialPageRoute<String>(
builder: (BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: const Text('Full Screen Dialog'),
actions: [
new ElevatedButton(
onPressed: () {
Navigator.of(context).pop("hai");
},
child: new Text('ADD',
style: TextStyle(color: Colors.white),
)),
],
),
body: Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
padding: EdgeInsets.all(20),
color: Colors.white,
child: Column(
children: [
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('Full Screen',
style: TextStyle(color: Colors.white),
),
)
],
),
),
);
},
fullscreenDialog: true
));
if(_selected != null)
setState(() {
_selected = _selected;
});
}
}
Output:
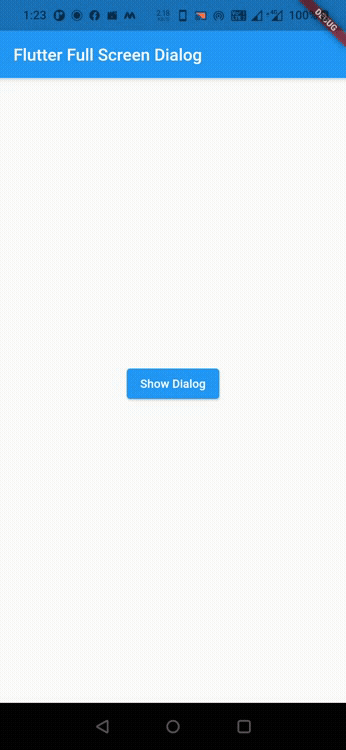
That’s all about the tutorial on how to use full screen dialog in flutter with example. I hope you understand how to create and display full screen dialog in flutter. Let’s catch up with some other widget in the next post. Have a great day!!
Do share, subscribe, and like my Facebook page if you find this post helpful. Thank you!!

Leave a Reply