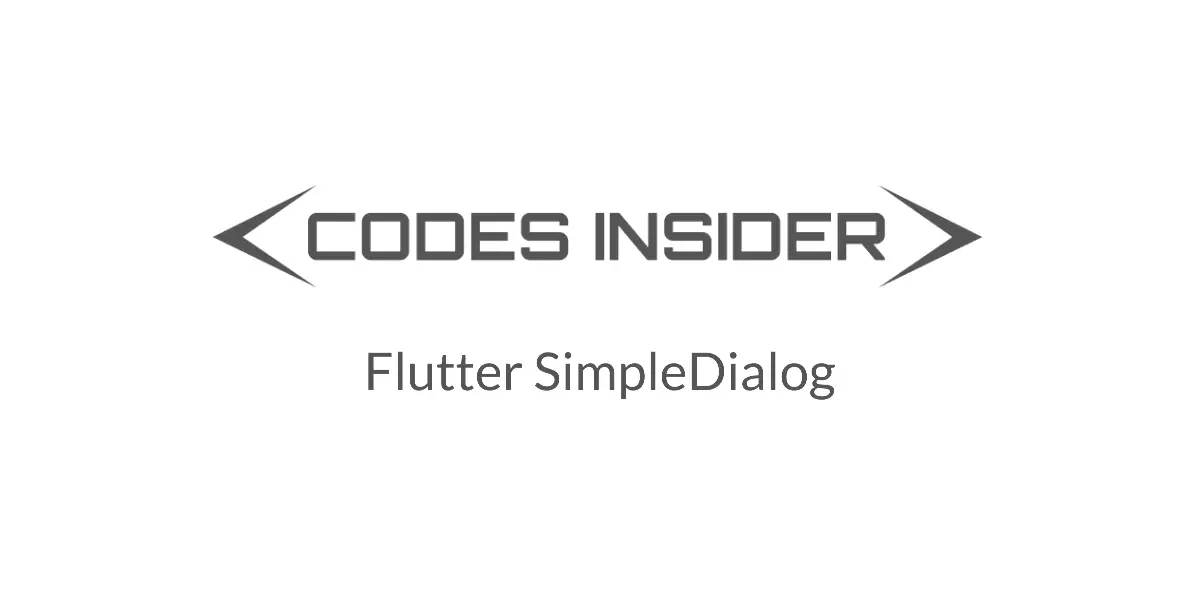
Flutter SimpleDialog
SimpleDialog is a material widget in flutter that lets the user choose an option from a set of options. A simple dialog has a title property that is optional. We can use simple dialogs in situations where the user has to pick a single item from a list of items. For example, while making payments we will be displayed with a list of payment options where we have to choose one payment option. We will create the options using the SimpleDialogOption widget.
This tutorial is about how to use SimpleDialog widget in flutter. We will also customize its style with different properties and see an example.
If you just want to display a message to the user instead of providing options consider using AlertDialog.
Constructor :
SimpleDialog({
Key key,
Widget title,
EdgeInsetsGeometry titlePadding,
TextStyle titleTextStyle,
List<Widget> children,
EdgeInsetsGeometry contentPadding,
Color backgroundColor,
double elevation,
String semanticLabel,
EdgeInsets insetPadding,
Clip clipBehavior,
ShapeBorder shape
})
Creating SimpleDialog In Flutter
We can create a simpledialog in flutter by using its constructor. To display the simple dialog we have to use showDialog() method. We will return simpledialog as the child widget to showDialog, which displays the dialog.
Let’s create a simple dialog and display it. First, we have to design the UI and a function where we will call the showDialog method that displays the alert dialog.
Designing The UI
We will design the UI where we will display a Button widget and a text widget. Clicking on the button will call the showDialog method that will show the Simple dialog. When the user chooses an option we will display the selected option in the text widget.
var _selected ="";
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter SimpleDialog"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
_displayDialog(context);
},
child: Text("Show Dialog"),
),
Text(_selected)
],
),
),
);
}
Designing UI Of SimpleDialog
We will now design a simple dialog where we will have a title and two options. The title is a text widget and the options are simpleDialogOption widgets.
SimpleDialog(
title: Text('Choose food'),
children:[
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Pizza"); },
child: const Text('Pizza'),
),
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Burger");
},
child: const Text('Burger'),
),
],
elevation: 10,
//backgroundColor: Colors.green,
),
Creating the showDialog() method
Now let’s create a method _displayDialog() where we call the showDialog() function which will display SimpleDialog widget with a title, and two options. when the user chooses an option we are assigning it to a variable ( _selected ) and updating the text view inside the setState().
_displayDialog(BuildContext context) async {
_selected = await showDialog(
context: context,
builder: (BuildContext context) {
return Expanded(
child: SimpleDialog(
title: Text('Choose food'),
children:[
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Pizza"); },
child: const Text('Pizza'),
),
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Burger");
},
child: const Text('Burger'),
),
],
elevation: 10,
//backgroundColor: Colors.green,
),
);
},
);
setState(() {
_selected = _selected;
});
}
If you don’t want the user to dismiss the dialog without selecting an option, set barrierDismissible property of showDialog method to false.
showDialog(
context: context,
barrierDismissible: false,
builder: (BuildContext context) {
return Expanded(
child: SimpleDialog(
title: Text('Choose food'),
children:[
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Pizza"); },
child: const Text('Pizza'),
),
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Burger");
},
child: const Text('Burger'),
),
],
elevation: 10,
backgroundColor: Colors.yellowAccent,
shape: StadiumBorder(),
),
);
},
);
Flutter SimleDialog Example Complete Code
Below is the complete code example for creating a simple dialog in flutter and displaying it.
import 'dart:io';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter SimpleDialog',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
var _selected ="";
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter SimpleDialog"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
_displayDialog(context);
},
child: Text("Show Dialog"),
),
Text(_selected)
],
),
),
);
}
_displayDialog(BuildContext context) async {
_selected = await showDialog(
context: context,
builder: (BuildContext context) {
return Expanded(
child: SimpleDialog(
title: Text('Choose food'),
children:[
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Pizza"); },
child: const Text('Pizza'),
),
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Burger");
},
child: const Text('Burger'),
),
],
elevation: 10,
//backgroundColor: Colors.green,
),
);
},
);
if(_selected != null)
{
setState(() {
_selected = _selected;
});
}
}
}
Output:
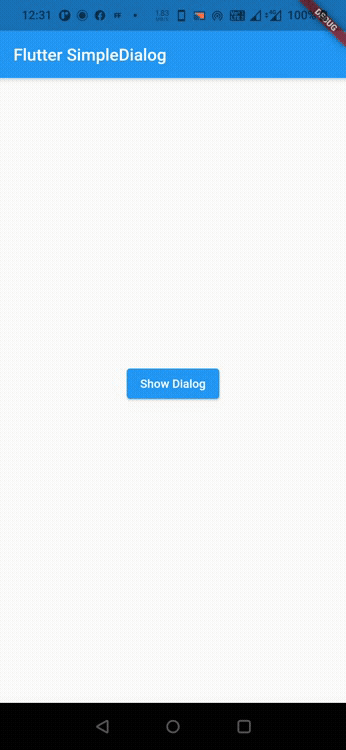
How to change background color and elevation of simpledialog
SimpleDialog(
title: Text('Choose food'),
children:[
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Pizza"); },
child: const Text('Pizza'),
),
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Burger");
},
child: const Text('Burger'),
),
],
elevation: 10,
backgroundColor: Colors.yellowAccent,
),
Output:
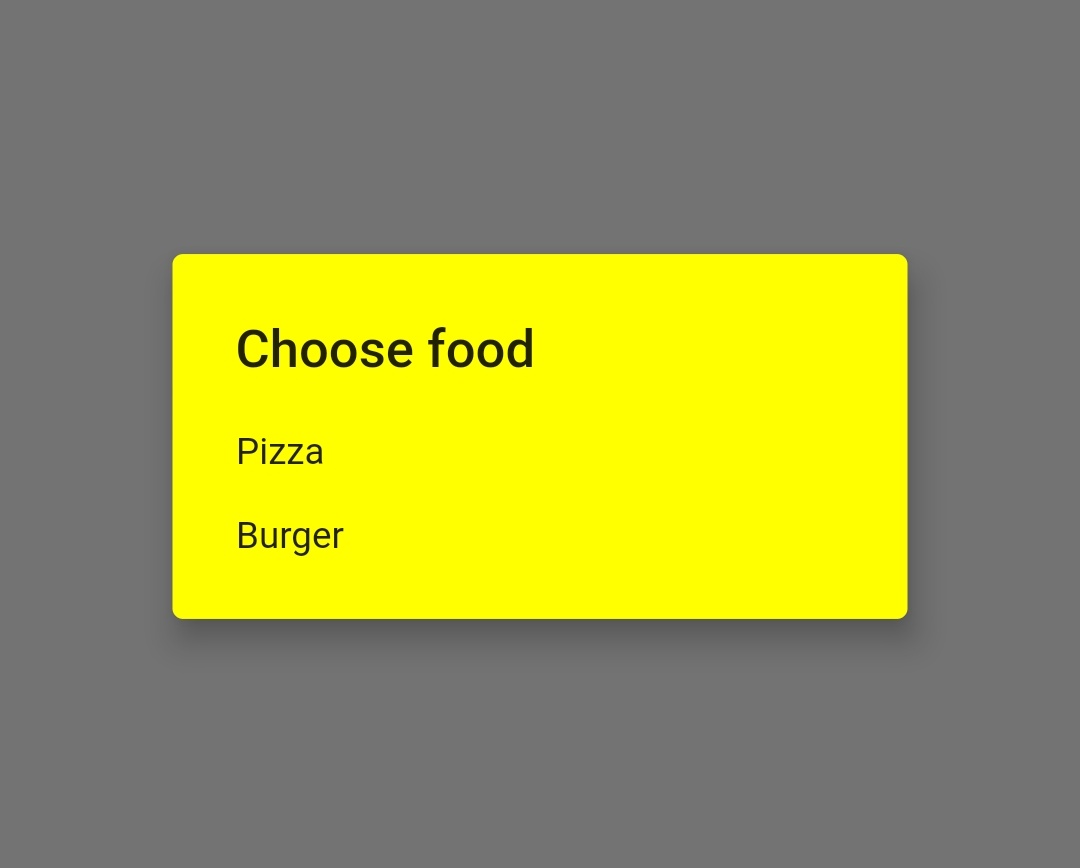
How to change shape of simpledialog
In the below example I’ve used stadiumborder for the shape of simpledialog. If you want to try other shapes just refer shaping RaisedButton section of flutter RaisedButton widget Example.
SimpleDialog(
title: Text('Choose food'),
children:[
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Pizza"); },
child: const Text('Pizza'),
),
SimpleDialogOption(
onPressed: () {
Navigator.pop(context, "Burger");
},
child: const Text('Burger'),
),
],
elevation: 10,
backgroundColor: Colors.yellowAccent,
shape: StadiumBorder(),
),
Output:
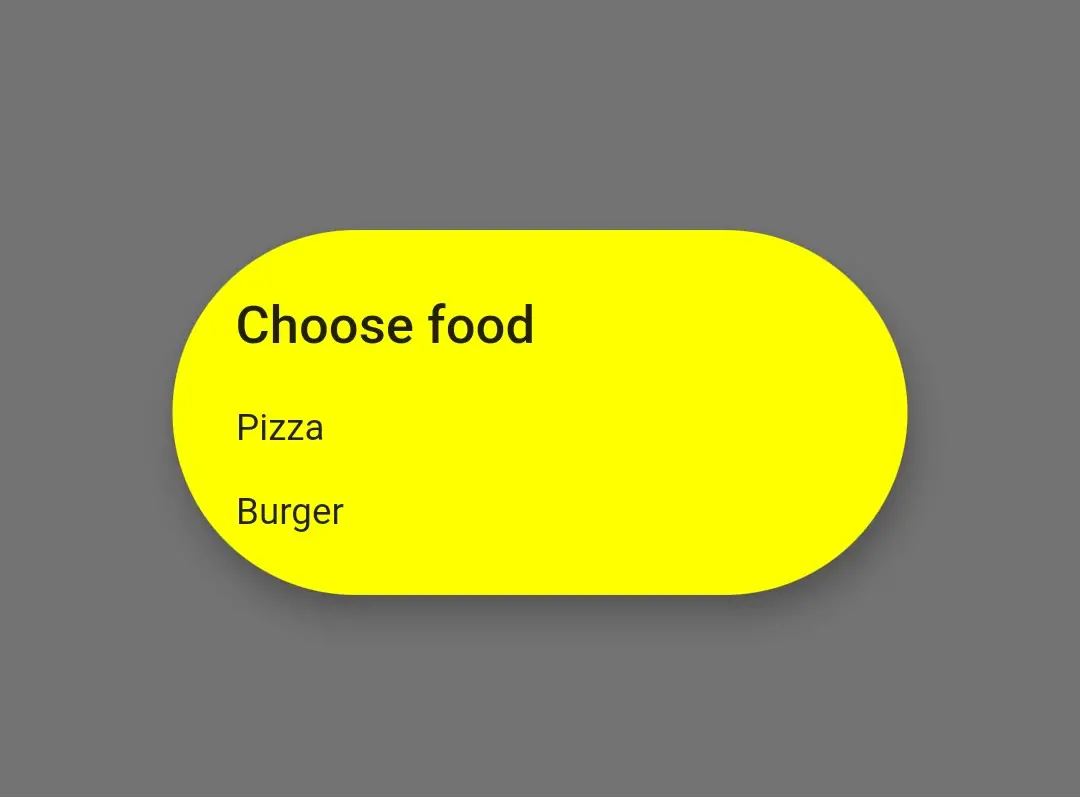
That brings an end to the tutorial about how to use the SimpleDialog widget in flutter with example. I hope you understand how to create and display simpledialog in flutter. Let’s catch up with some other widget in the next post. Have a great day!!
Do share, subscribe, and like my Facebook page if you find this post helpful. Thank you!!
Reference: Flutter Official Documentation.

Leave a Reply