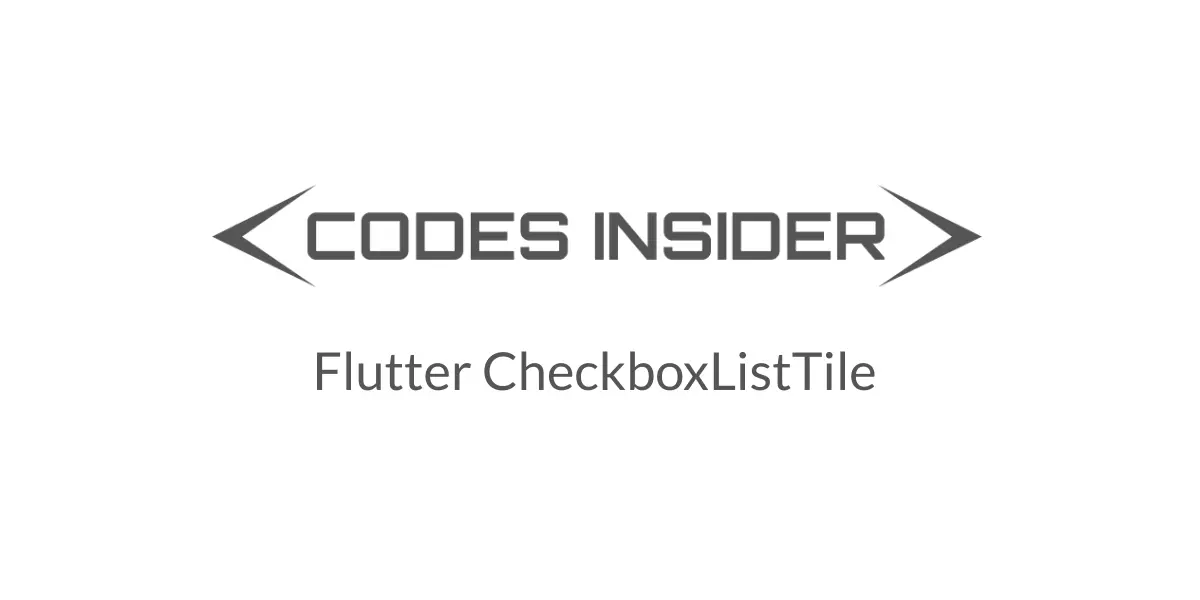
In flutter, CheckboxListTile is a ListTile with a Checkbox. We can also call it as a checkbox with a label. In this example tutorial, we will learn how to use a CheckboxListTile widget in flutter and its properties with examples.
Flutter CheckboxListTile
CheckboxListTile in flutter is nothing but a ListTile containing a checkbox by default. If we tap anywhere on the list tile it will toggle the state of the checkbox. Checkboxlisttile can be used as a child of listview. For example, If we want the users to choose a few or all options from a list of options we can use checkboxlisttile.
Most of the properties of the checkboxlisttile are similar to checkbox and listtile. So check those widgets for reference. If you want to select / unselect the tile when the checkbox is checked / unchecked provide the same value to value and selected properties which is boolean.
Constructor:
CheckboxListTile({
Key key,
@required bool value,
@required ValueChanged<bool> onChanged,
Color activeColor,
Color checkColor,
Widget title,
Widget subtitle,
bool isThreeLine: false,
bool dense,
Widget secondary,
bool selected: false,
ListTileControlAffinity controlAffinity: ListTileControlAffinity.platform,
bool autofocus: false,
EdgeInsetsGeometry contentPadding,
bool tristate: false
})
Flutter CheckboxListTile Example
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
void main() {
runApp(MyApp());
}
//void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyState createState() {
return _MyState();
}
}
class _MyState extends State<MyApp>
{
bool _value = false;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
backgroundColor: Colors.green[400],
title: Text("Flutter CheckboxListTile"),
),
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Checkbox"),
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
)
);
}
}
Output:
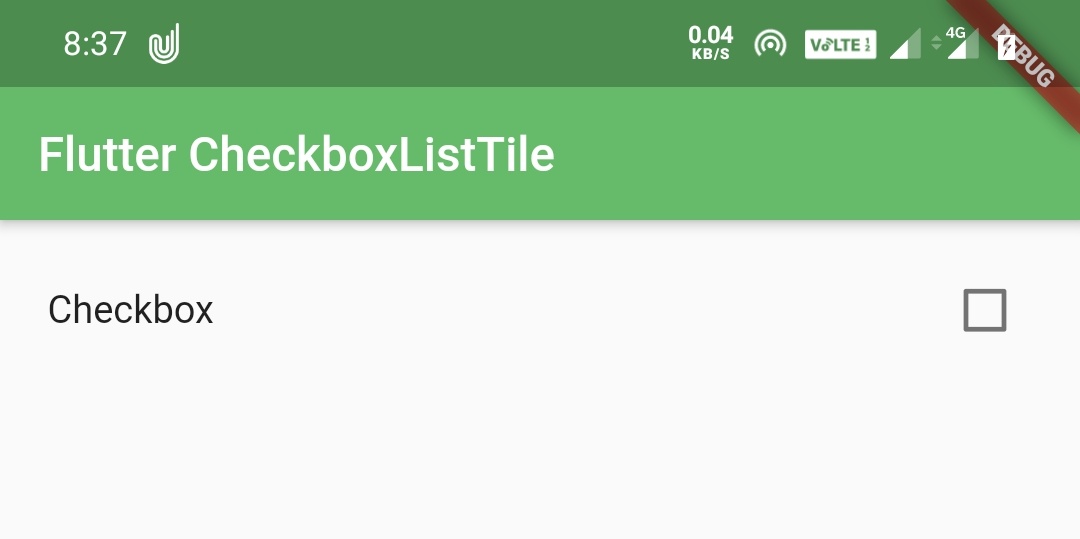
Flutter CheckboxListTile Properties
The different flutter CheckboxListTile properties are:
- value(required)
- onChanged(require)
- activeColor
- checkColor
- tristate
- title
- subtitle
- secondary
- selected
- controlAffinity
- contentPadding
As the value, onChanged, activeColor, checkColor and tristate properties of this widget are identical to the similarly-named properties on the Checkbox widget i’m not discussing them in this post.
tristate
We can use tristate property in CheckboxListTile but we cannot use same value(true/false) to both the value and selected properties. When tristate property is true the value property will accept three values(true, false, null) but the selected property will only accept two values(true, false). Passing same value to both will result in error when the value is null.
title
We will use title property to set a text or label to the checkbox. To show the users what the checkbox indicates, we will use this property.
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Checkbox"),
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
Output:
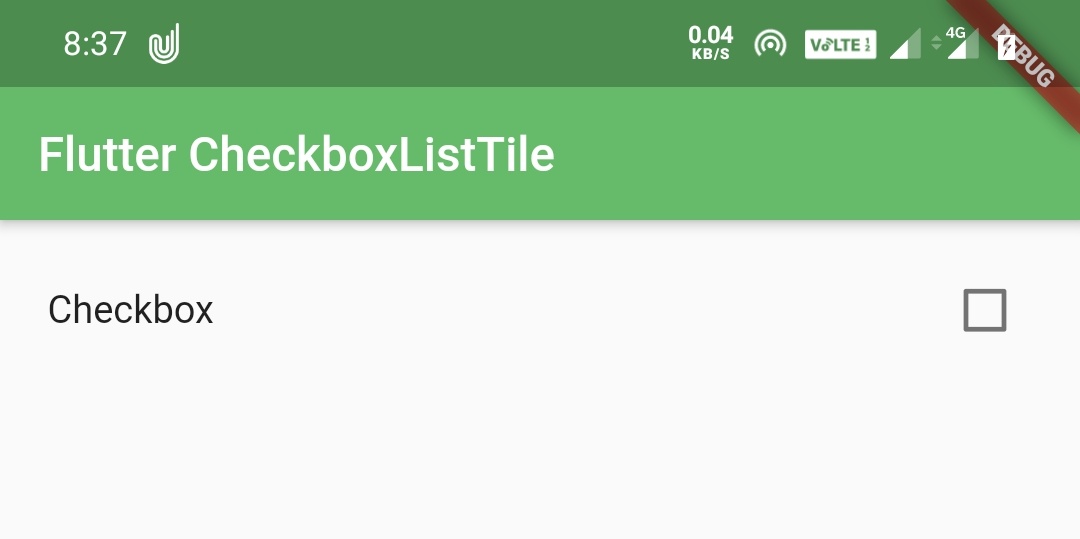
subtitle
We will use subtitle property to add or change subtitle to the checkbox title.
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Title"),
subtitle: Text("Subtitle"),
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
Output:
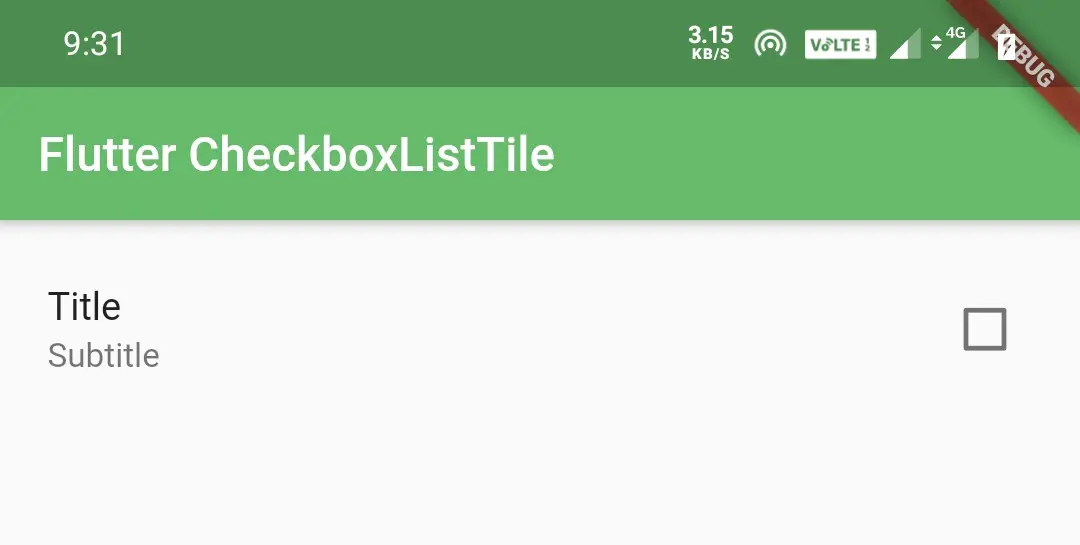
secondary
The secondary property can be any widget, generally we will use an icon as secondary widget. We can use Text or any other widget instead.
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Product"),
subtitle: Text("Description"),
secondary: Icon(Icons.ac_unit),
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
Output:
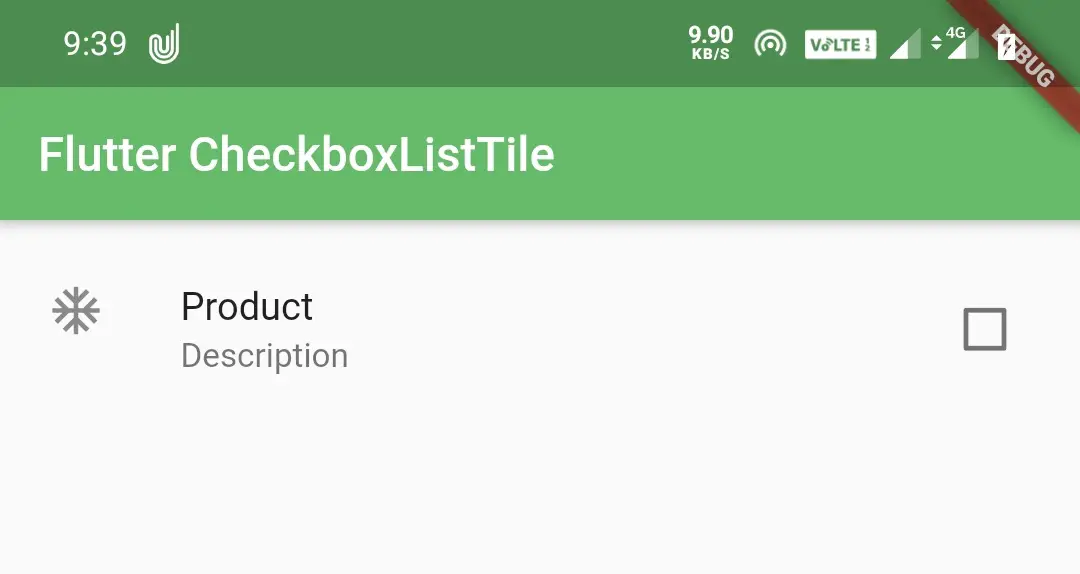
selected
We will use selected property to decide whether we want to render icon and text colors with the color of activeColor property. If there is no active color applied, it will use default color.
It takes a boolean value. If we set it to true it will render icon and text colors with the activecolor. If we set it to false there will be no change in text and icon colors. To make the ListTile appear selected when the checkbox is checked we have to pass same value(true or false) to the value and selected properties.
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Product"),
subtitle: Text("Description"),
secondary: Icon(Icons.ac_unit),
selected: _value,
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
Output:
When checkbox is checked
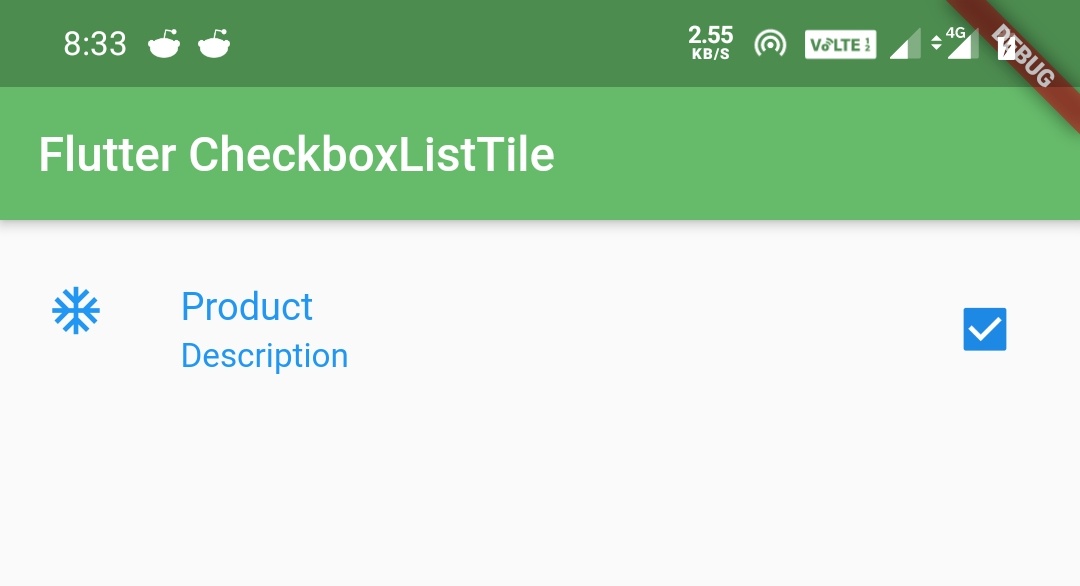
When unchecked
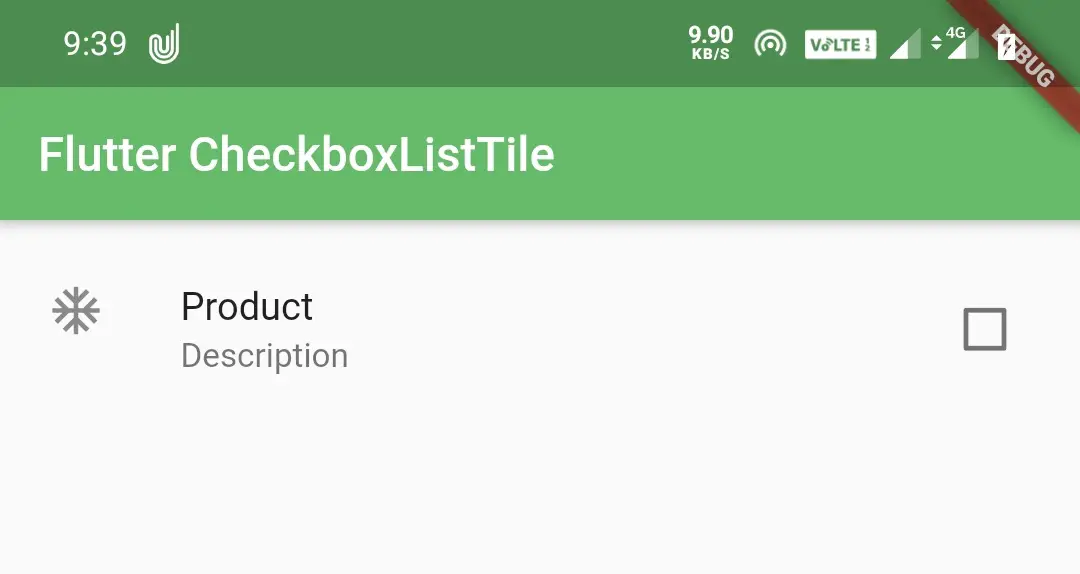
controlAffinity
We will use controlAffinity property to control the position of the checkbox relative to the text. It takes ListTileControlAffinity which has constants leading, trailing and platform.
ListTileControlAffinity.leading
Positions checkbox to left, text to center and secondary(generally an icon) to right.
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Product"),
subtitle: Text("Description"),
secondary: Icon(Icons.ac_unit),
selected: _value,
controlAffinity: ListTileControlAffinity.leading,
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
Output:
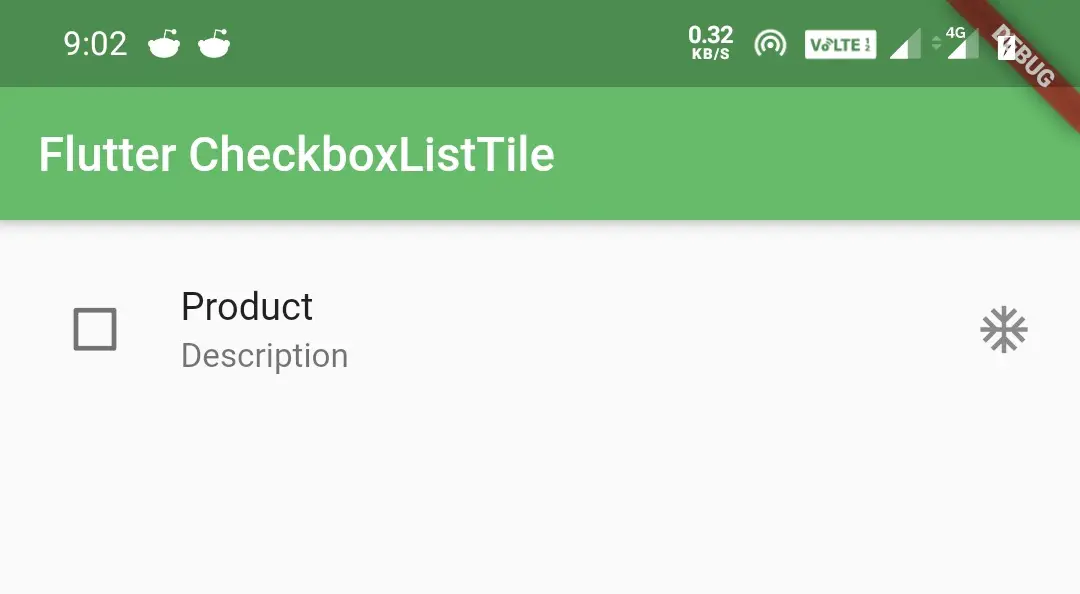
ListTileControlAffinity.trailing
Positions checkbox to right, text to center and secondary to left.
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Product"),
subtitle: Text("Description"),
secondary: Icon(Icons.ac_unit),
selected: _value,
controlAffinity: ListTileControlAffinity.trailing,
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
Output:
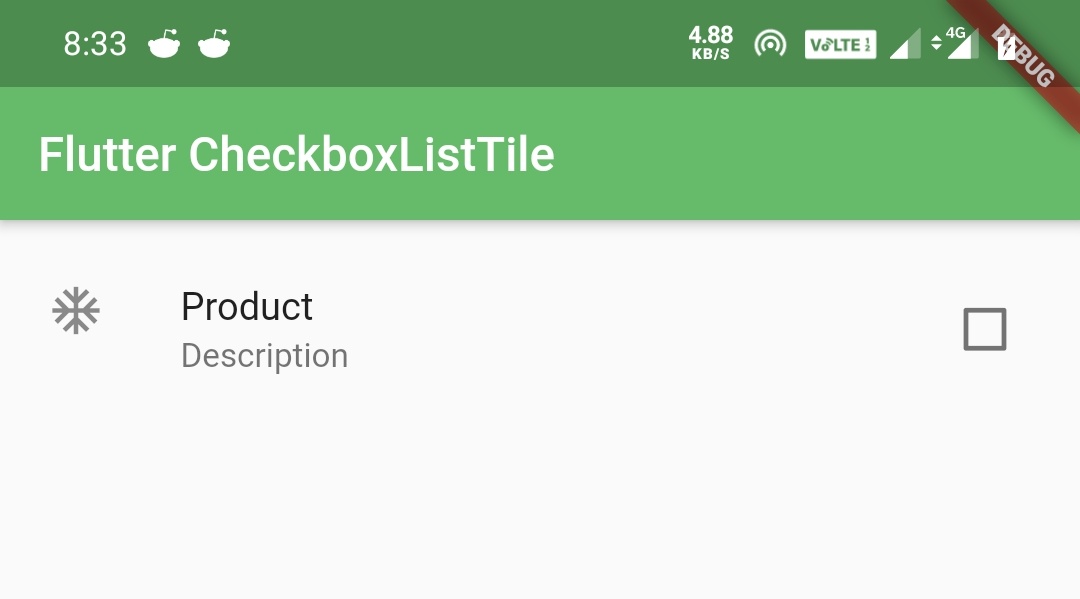
contentPadding
We will use contentPadding property to provide padding to the list tile content.
body:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Product"),
subtitle: Text("Description"),
secondary: Icon(Icons.ac_unit),
selected: _value,
controlAffinity: ListTileControlAffinity.trailing,
contentPadding: EdgeInsets.symmetric(
horizontal: 50,
vertical: 20
),
),
Output:
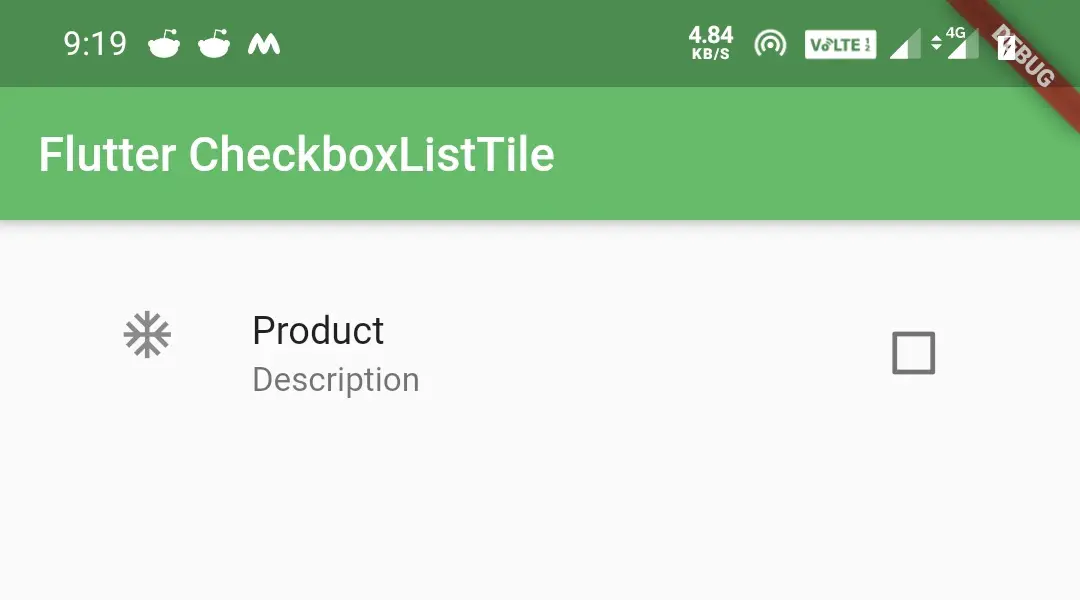
Changing CheckboxListTile border color when unchecked
To change the border color of the checkbox inside CheckboxListTile when it is unchecked just wrap the CheckboxListTile widget inside a Theme widget as a child and set ThemeData‘ s unselectedWidgetColor property to your desired color.
body:Theme(
data: ThemeData(
unselectedWidgetColor: Colors.red
),
child:CheckboxListTile(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
title: Text("Product"),
subtitle: Text("Description"),
secondary: Icon(Icons.ac_unit),
selected: _value,
controlAffinity: ListTileControlAffinity.trailing,
contentPadding: EdgeInsets.symmetric(
horizontal: 20,
vertical: 10
),
),
)
Output:
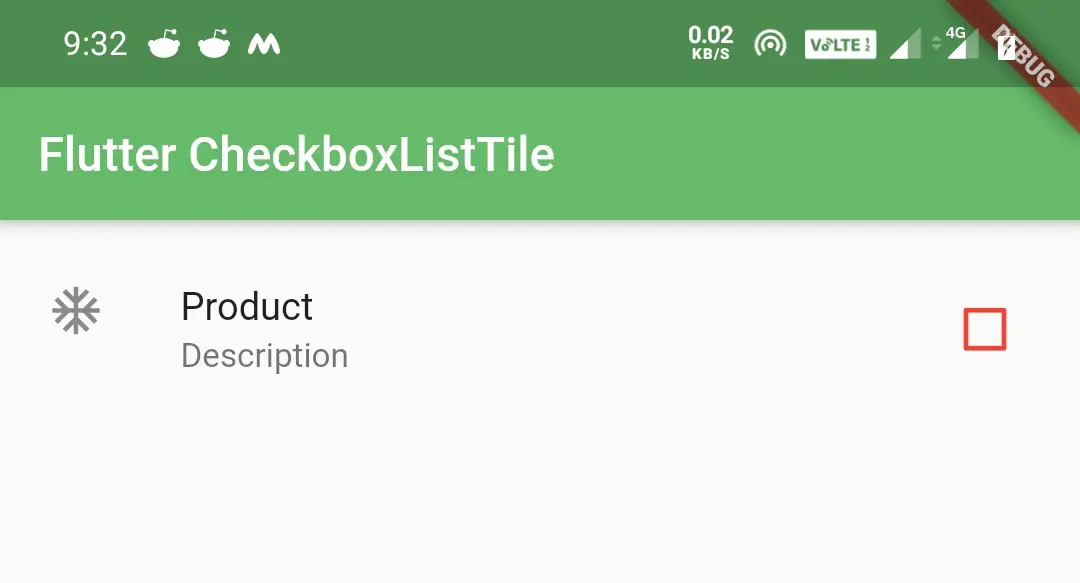
That brings an end to flutter CheckboxListTile example tutorial. Let’s catch up with some other flutter tutorial soon. Have a great day!!
Do share, subscribe and like my facebook page if you find this post helpful. Thank you!!
Reference: Flutter Official Documentation

Leave a Reply