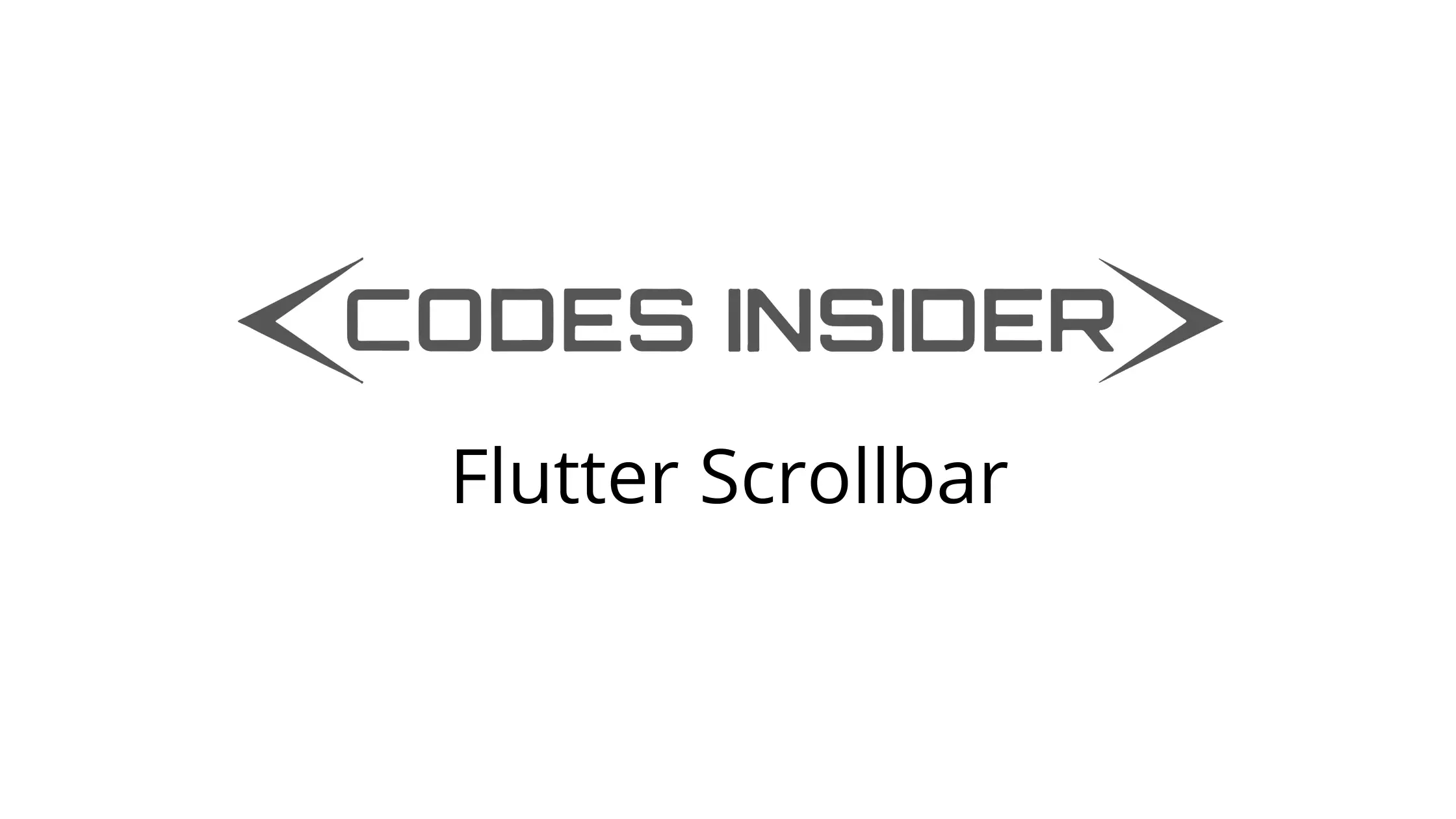
Flutter Scrollbar
Scrollbar is a material widget in flutter that indicates to the user how lengthy the view is. It has a thumb that indicates how long the user has scrolled either from top or bottom. To display a scrollbar for a scrollview we have to add the scrollview as a child to scrollbar widget. The thumb will fade in and out while we scroll the scrollview associated with the scrollbar. To make the thumb visible all the time we have to set isAlwaysShown property to true. We can scroll to our desired position by dragging the thumb or by tapping on the track of the scrollbar.
In this tutorial, we will learn how to use scrollbar in flutter with example. We will also learn how to customize the Scrollbar widget using different properties.
To implement a scrollbar in ios platform using flutter refer cupertino scrollbar in flutter.
How To Create Scrollbar In Flutter ?
To create a scrollbar in flutter we have to use Scrollbar class. Call the constructor of Scrollbar class and provide the required properties. It has one required property child. It takes a widget as a child. Generally, we will use scrollable widgets like a listview, gridview, etc as child.
Flutter Scrollbar Constructor:
Scrollbar(
{Key? key,
required Widget child,
ScrollController? controller,
bool? isAlwaysShown,
bool? showTrackOnHover,
double? hoverThickness,
double? thickness,
Radius? radius,
ScrollNotificationPredicate? notificationPredicate,
bool? interactive,
ScrollbarOrientation? scrollbarOrientation}
)
Flutter Scrollbar Properties
The properties of a flutter scrollbar are:
- child
- controller
- isAlwaysShown
- showTrackOnHover
- hoverThickness
- thickness
- radius
- notificationPredicate
- interactive
- scrollbarOrientation
child
The child will be the widget to which we will show the scrollbar for. Generally, we will use scrollable widgets like listview or gridview as child. We can also add a widget with content that can’t be displayed on the available screen space as the child.
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
)
Output:
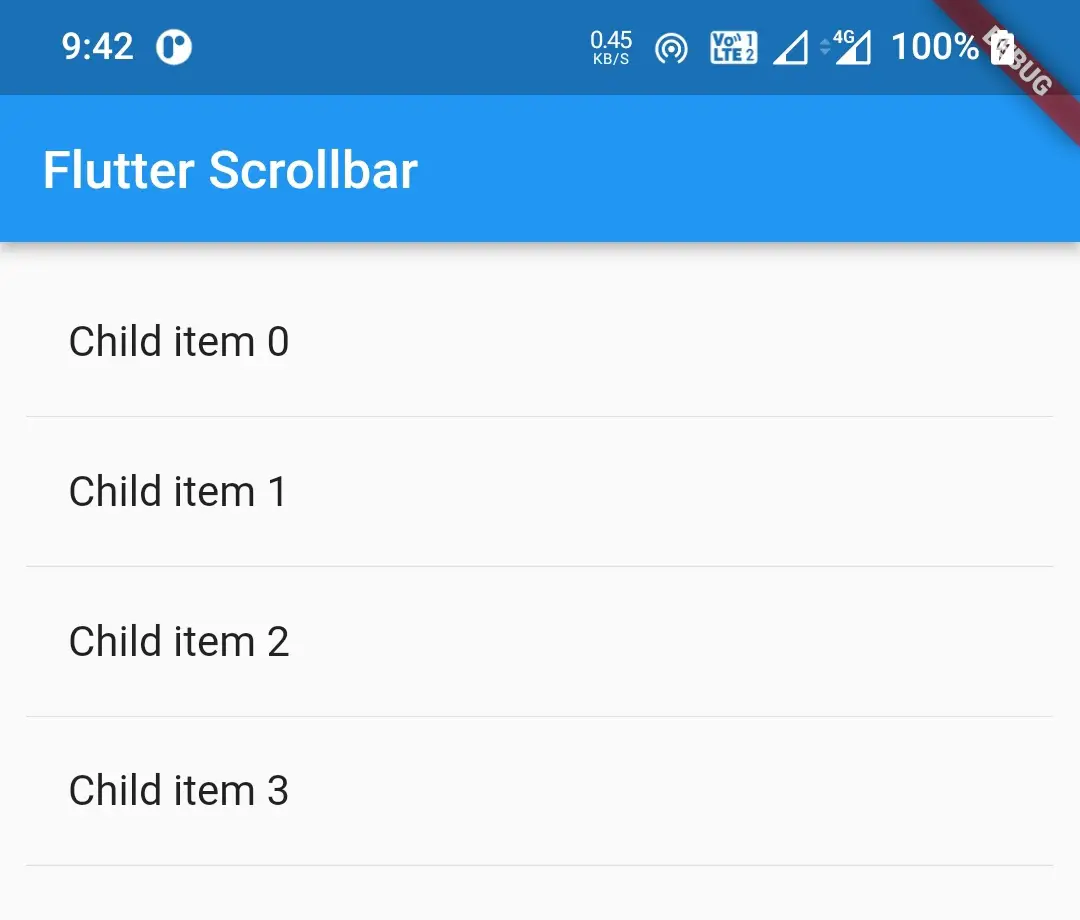
isAlwaysShown
It takes boolean as value. The scrollbar thumb fades in and out when the user scrolls the child. To make the scrollbar visible all the time set this property to true.
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
isAlwaysShown: true,
)
Output:
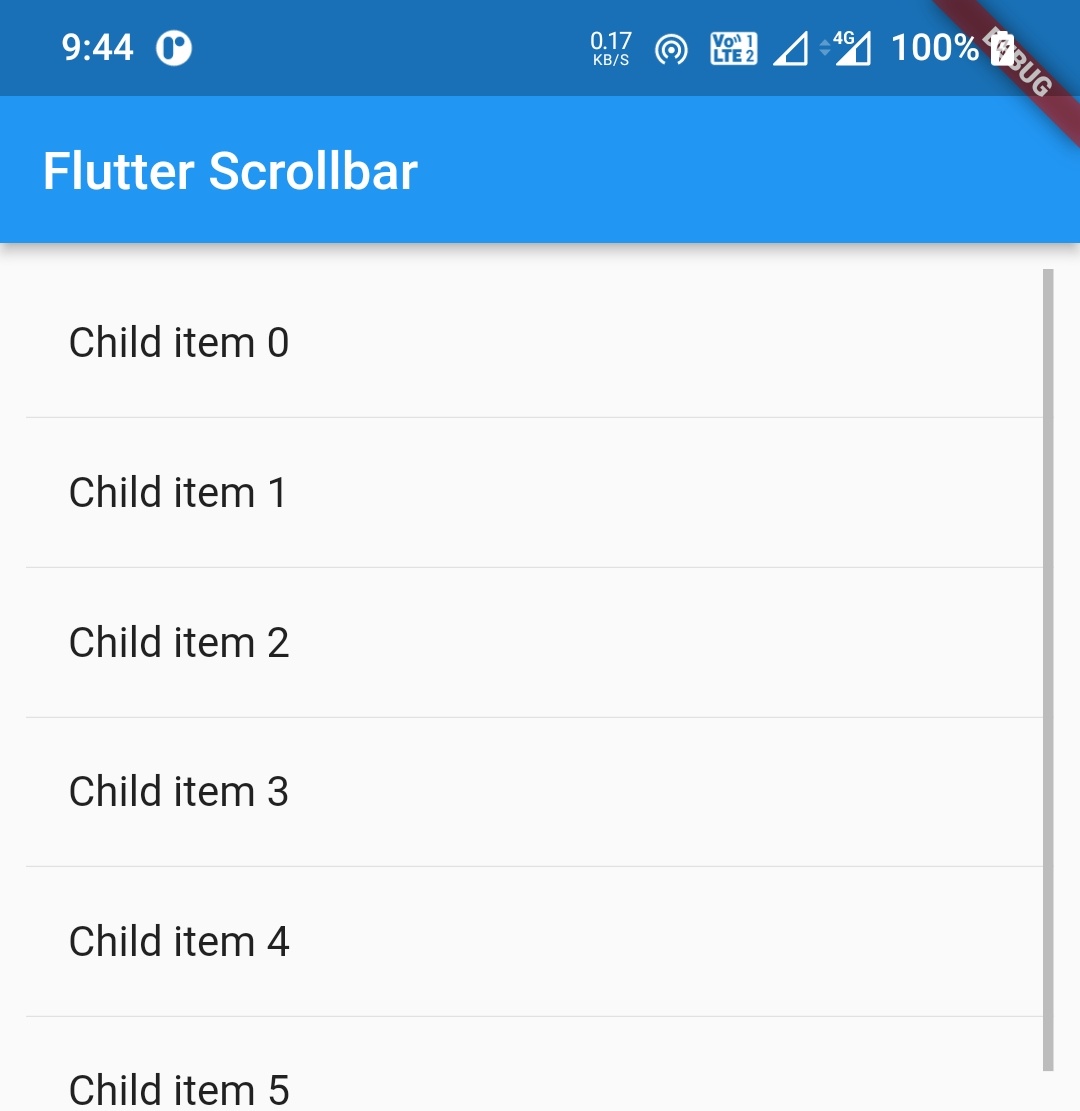
showTrackOnHover
We will use this property to control whether to show the track on hover. It takes boolean as value. By default it is false
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
isAlwaysShown: true,
showTrackOnHover: true
)
hoverThickness
We will use this property to change the thickness when hovered. It takes double as value. For this property to work we have to use showTrackOnHover property. The default thickness is 12 pixels.
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
isAlwaysShown: true,
showTrackOnHover: true,
hoverThickness: 40,
)
thickness
To change the thickness of the scrollbar thumb we will use this property. It takes double as value. By default, it is 4.0 pixels.
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
isAlwaysShown: true,
showTrackOnHover: true,
thickness: 15,
)
Output:
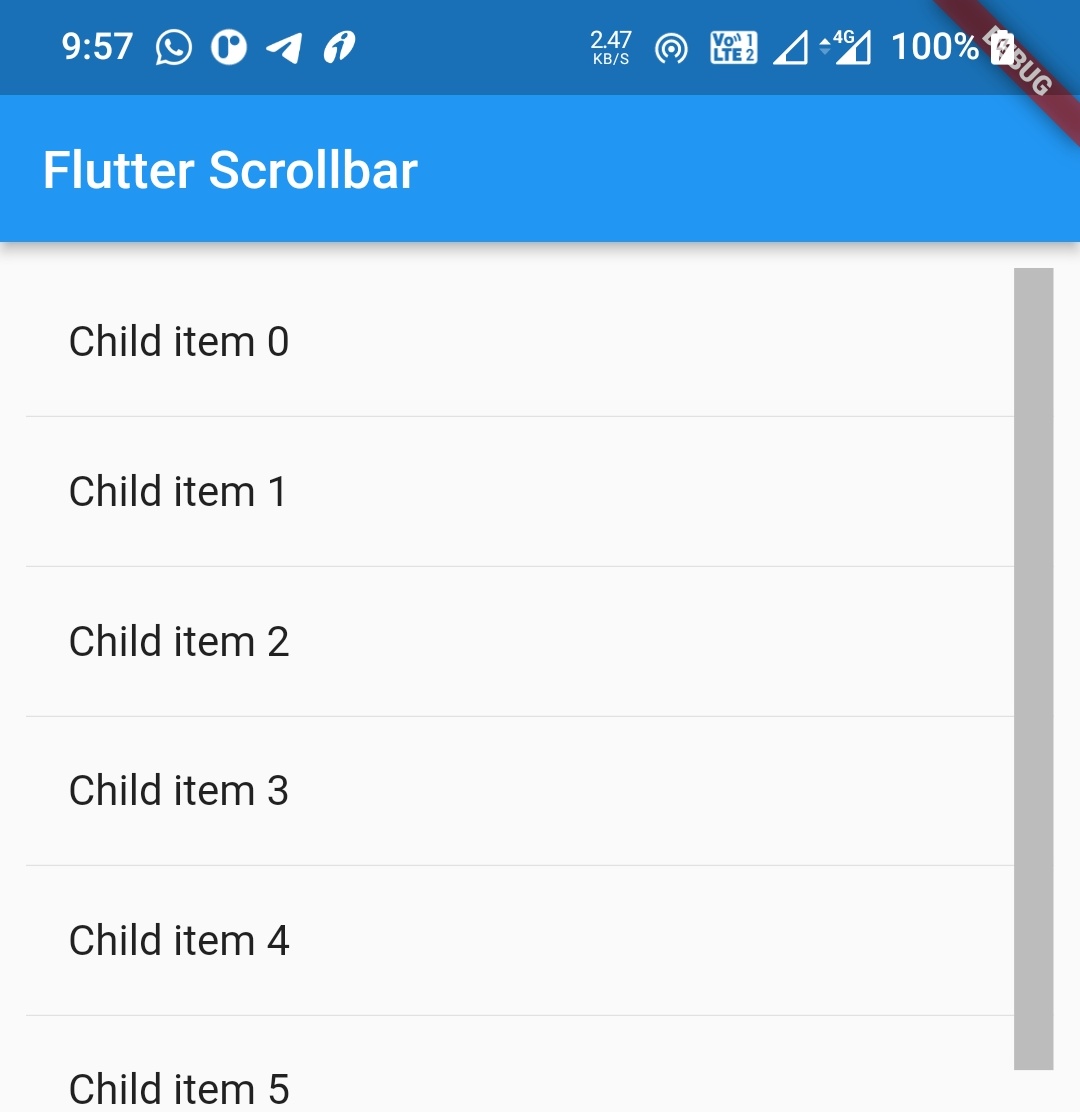
radius
To apply rounded corners to the scrollbar thumb we will use this property. It takes the Radius class constant as value. By default, the radius is 8.0 pixels.
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
isAlwaysShown: true,
showTrackOnHover: true,
thickness: 15,
radius: Radius.circular(20),
)
Output:
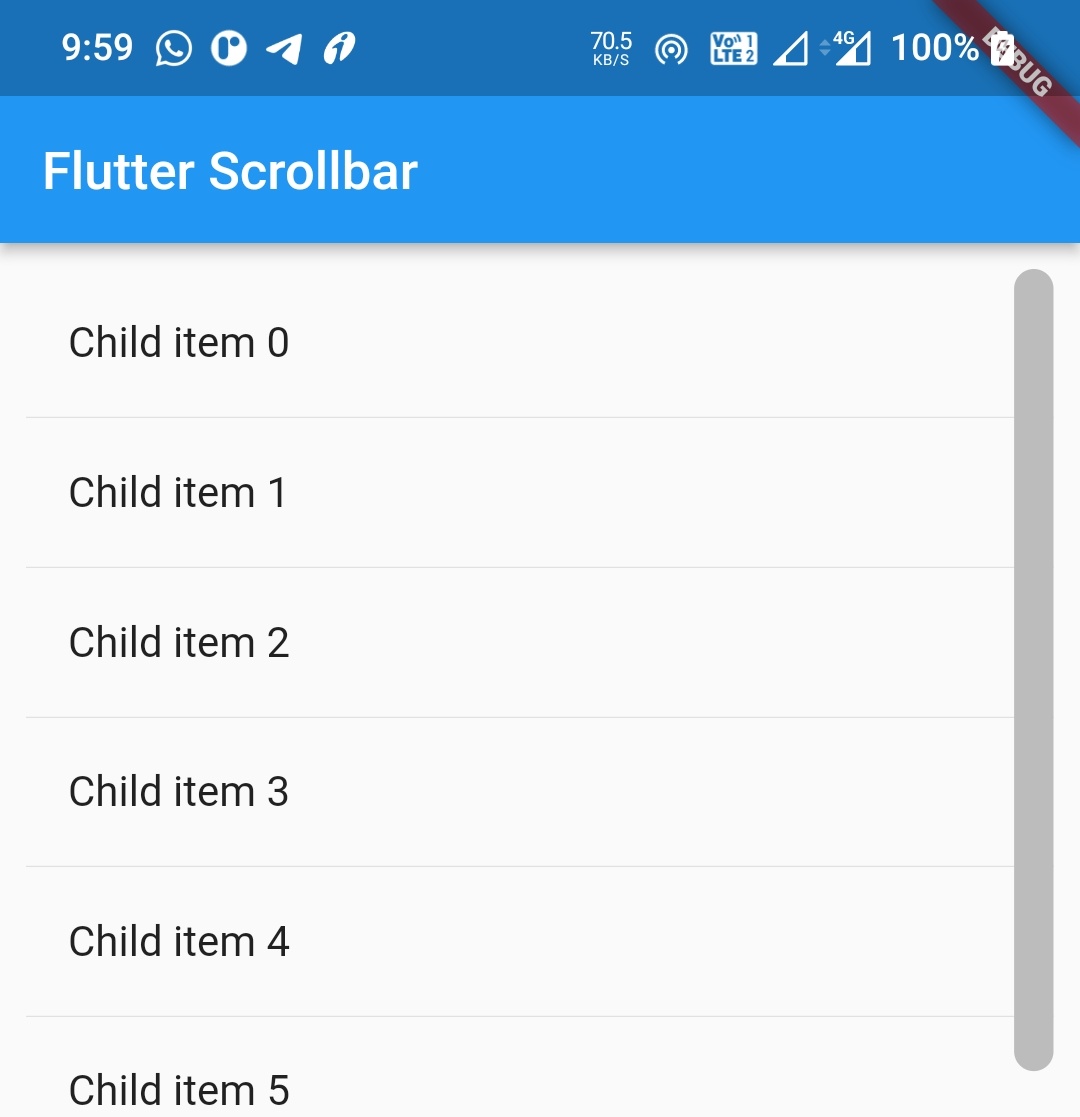
interactive
We will use this property to control whether we can drag the thumb to scroll. It takes boolean as value. Setting this property to true will allow us to drag the thumb to scroll. By default, the value is false.
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
isAlwaysShown: true,
showTrackOnHover: true,
thickness: 15,
radius: Radius.circular(20),
interactive: true,
)
scrollbarOrientation
We can change the orientation of the scrollbar using this property. It takes ScrollbarOrientation constant as value. It has constants left, right, bottom, and top. By default, the value is ScrollbarOrientation.right.
Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
ListTile(
title: Text(items[index]),
),
Divider(
height: 1,
)
],
)
);
},
itemCount: items.length,
),
isAlwaysShown: true,
showTrackOnHover: true,
thickness: 15,
radius: Radius.circular(20),
interactive: true,
scrollbarOrientation: ScrollbarOrientation.left,
)
Output:
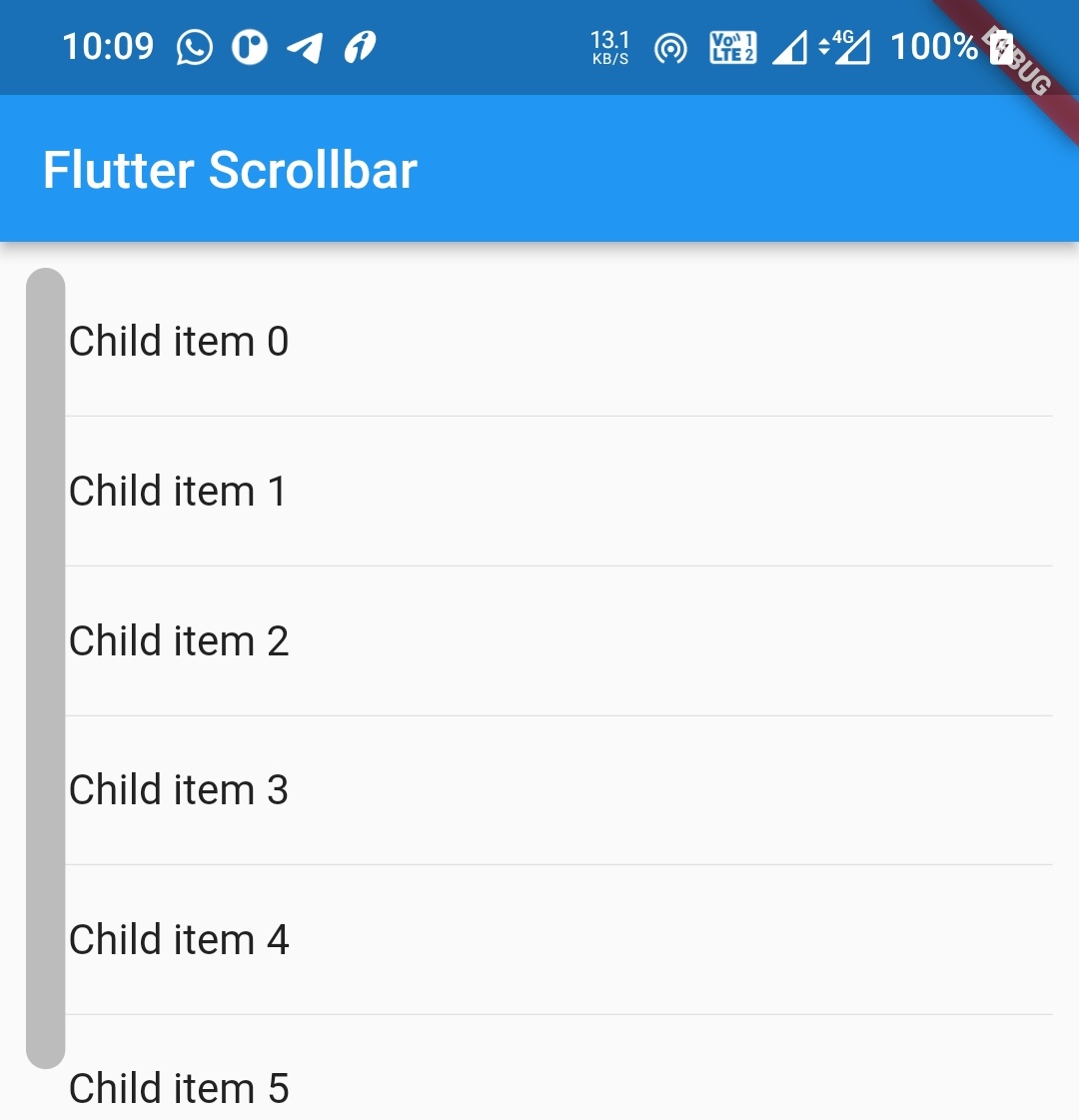
Flutter Scrollbar Example
Let’s create an example where we will implement scrollbar in flutter using Scrollbar widget. In this example, we will display a listView with 40 items as a child to the scrollbar. We will display the children using the card widget.
First, let’s create a list (items) and add data to it using List.generate method. We will use the generated data as an item to the listview.
List items = List.generate(40, (index) => "Child item $index ");
Now let’s add the scrollbar to the body of the Scaffold widget. We will provide a list view as a child to the Scrollbar widget. Inside the builder of the listview, we will return the card widget. The card widget contains a ListTile as a child. The listtile will have a yellow background color. The title for the listtile is set using the list (items) created above based on the index. The item count of the listview will be the length of the items.
Container(
margin: EdgeInsets.all(10),
child: Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Card(
child: Material(
child: ListTile(
title: Text(items[index]),
tileColor: Colors.yellow,
),
),
);
},
itemCount: items.length,
),
isAlwaysShown: true,
thickness: 10,
)
),
Complete Code
Let’s complete the scrollbar example in flutter by combining the code snippets above. Create a new flutter project and replace the code in main.dart file with the code below.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
List items = List.generate(30, (index) => "Child item $index ");
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: Text("Flutter Scrollbar"),
),
body:Container(
margin: EdgeInsets.all(10),
child: Scrollbar(
child: ListView.builder(
itemBuilder:(context, index) {
return Card(
child: Material(
child: ListTile(
title: Text(items[index]),
tileColor: Colors.yellow,
),
),
);
},
itemCount: items.length,
),
isAlwaysShown: true,
thickness: 10,
)
),
);
}
}
Output:
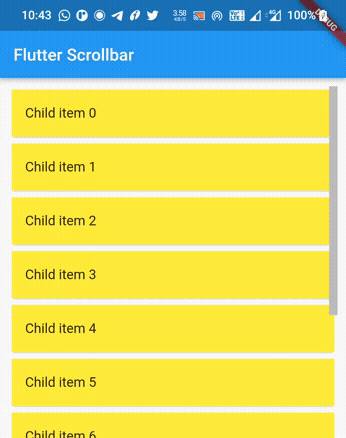
That’s all about the tutorial on how to create & use Scrollbar in flutter. We have also seen an example where we have used the Scrollbar widget and customized its style. Let’s catch up with some other widget in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official Documentation.

Leave a Reply