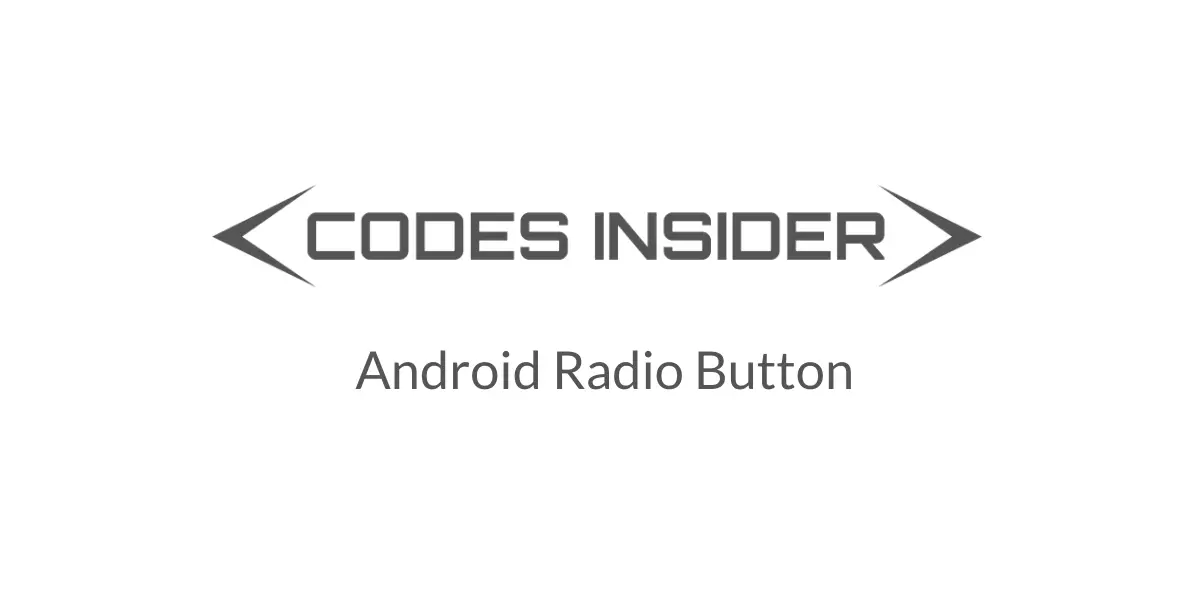
Android radio button is commonly used in applications especially while filling forms where a user have to select only one option from the list of options shown to the user(Eg: gender: male, female).
Android Radio Button
Android radio button is a two state button which is checked/unchecked. It is similar to checkbox but we cannot select multiple radio buttons like checkboxes.
A radio button once checked cannot be unchecked like a checkbox do. Generally radio buttons are used with radio group so that if we check one radio button in the group the others gets unchecked. To uncheck a radio button we have to check another radio button from the same radio group.
Check this android UI controls tutorial to know more about UI controls.
Android Radio Button Code in XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:padding="20dp"
android:background="#ffffff">
<RadioButton
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"/>
</LinearLayout>
Android Radio Button code in JAVA
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.LinearLayout;
import android.widget.RadioButton;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
RadioButton button = new RadioButton(this);
LinearLayout main = findViewById(R.id.main);
main.addView(button);
}
}
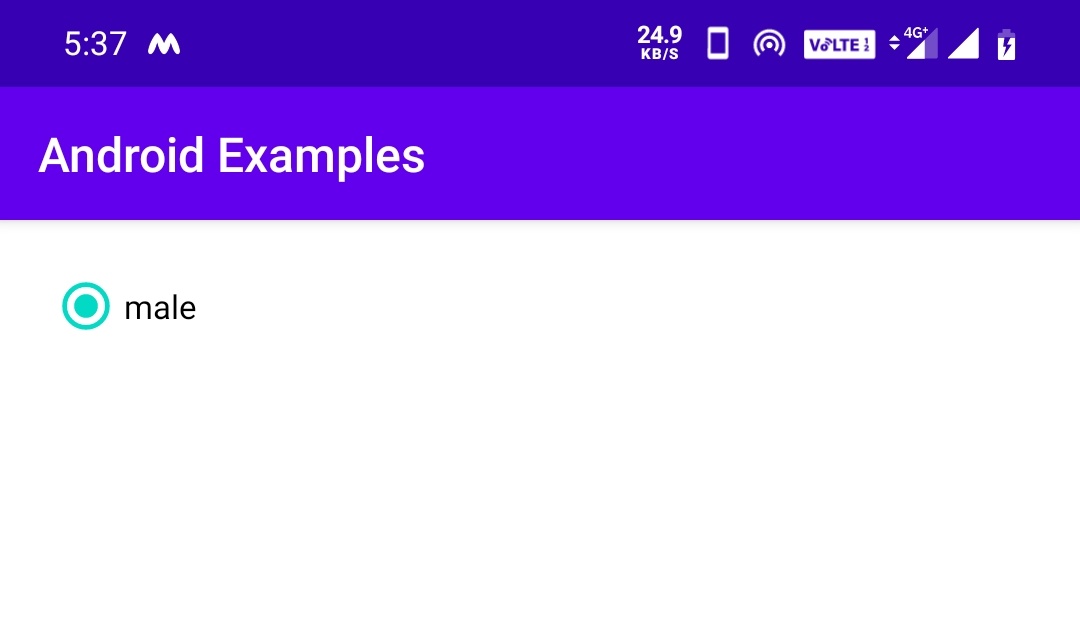
Android Radio Button Attributes
Lets see the attributes of a radio button
checked : It is used to set the state of a radio button. The value will be true for checked state and false for unchecked state of a checkbox. By default the Radio button’s state is unchecked which is false.
Code snippet for setting checked state in XML
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:checked="true"/>
Code snippet for setting checked state in JAVA
RadioButton radio = findViewById(R.id.radio);
radio.setChecked(true);
gravity : The gravity attribute is used to align the radio button text to left, right, center, top etc
Setting gravity in XML
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:checked="true"
android:gravity="end|center_vertical"/>
Setting gravity in JAVA
RadioButton radio = findViewById(R.id.radio);
radio.setChecked(true);
radio.setGravity(Gravity.END);
layoutGravity : Layout gravity is used to align the entire Radio button and its text(entire Radio button layout) to left, right, top, bottom etc.
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:checked="true"
android:layout_gravity="start"
/>
text : Text attribute is used to set text for a radio button.
Setting text in XML
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
/>
Setting text in JAVA
RadioButton radio = findViewById(R.id.radio);
radio.setChecked(true);
radio.setGravity(Gravity.END);
radio.setText("MALE");
textColor : TextColor attribute is used to set text color of radio button.
Setting text color in XML
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:textColor="#666666"
/>
Setting text color in JAVA
RadioButton radio = findViewById(R.id.radio);
radio.setText("MALE");
radio.setTextColor(Color.GRAY);
textSize : TextSize attribute is used to set the text size of the radio button.
Setting text size in XML
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:textSize="16sp"
/>
Setting text size in JAVA
RadioButton radio = findViewById(R.id.radio);
radio.setText("MALE");
radio.setTextSize(16);
textStyle : TextStyle attribute is used to style the text of the radio button. The value for text style can be normal, bold and italic. By default the value is normal.
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:textSize="16sp"
android:textStyle="bold"
/>
background : Background attribute is used to set background for radio button. The background can be a color or a resource file.
Setting background color in XML
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:textSize="16sp"
android:textStyle="bold"
android:background="@color/colorAccent"
/>
Setting background in JAVA
RadioButton radio = findViewById(R.id.radio);
radio.setText("MALE");
radio.setTextSize(16);
radio.setBackgroundColor(Color.RED);
That’s all about the most generally used attributes of radio button. To know the attributes that are common for all the elements refer the layout attributes section of this post about android layouts.
We can find whether a radio button is checked or not in two ways
1. By using ischecked() method.
2. By using OnCheckedchangedListener method.
isChecked() : This method is used to find if a radio button is checked or not. This method returns a boolean value which is true/false.It returns true if the radio button is checked and false if unchecked.
RadioButton radio = findViewById(R.id.radio);
boolean checked = radio.isChecked();
OnCheckedChangeListener : This OnCheckedChangeListener implements onCheckedChanged() method. It has two parameters in which one parameter is object of compoundButton class and the other is a boolean value. It is checked state of the checkbox. This method listens whenever the radiobutton is checked or unchecked.
RadioButton radio = findViewById(R.id.radio);
radio.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
if(b)
{
Toast.makeText(MainActivity.this, "checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(MainActivity.this, "unchecked", Toast.LENGTH_SHORT).show();
}
}
});
But this method is of no use if we call this on a single radio button as we cannot uncheck a radio button.
If we call the same method on a radio group it returns two parameters. One is RadioGroup object and the second one is the selected radio button id. By using this id we can get the info about the selected radio button. Lets see sample code of how it works.
RadioGroup rg = new RadioGroup(this);
rg.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup radioGroup, int i) {
RadioButton radio = radioGroup.findViewById(i);
Toast.makeText(MainActivity.this, radio.getText().toString(), Toast.LENGTH_SHORT).show();
}
});
Tip: We can also get checked radio button id by using
RadioButton rb = (RadioButton) radioGroup.findViewById(radioGroup.getCheckedRadioButtonId());
So in the above example we are displaying the text of selected radio button from the group of radio buttons.
Radio Button Example
Lets see an example where we display 2 radio buttons in a radio group and 2 radio buttons individually. Lets see how they work and also how to clear selection of a radio group.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:padding="20dp"
android:background="#ffffff"
android:orientation="vertical"
android:id="@+id/main">
<RadioButton
android:id="@+id/radio"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:textSize="16sp"
android:textStyle="bold"
/>
<RadioButton
android:id="@+id/radio2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="female"
android:textSize="16sp"
android:textStyle="bold"
android:layout_marginTop="20dp"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Radio Group"
android:textSize="20dp"
android:layout_marginTop="20dp"
android:textColor="#000000"
/>
<RadioGroup
android:id="@+id/rg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp">
<RadioButton
android:id="@+id/radio3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="male"
android:textSize="16sp"
android:textStyle="bold"
/>
<RadioButton
android:id="@+id/radio4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="female"
android:textSize="16sp"
android:textStyle="bold"
android:layout_marginTop="20dp"
/>
</RadioGroup>
<Button
android:id="@+id/clear"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorAccent"
android:text="clear"
android:textColor="#ffffff"
android:textSize="16sp"
android:textStyle="bold"
android:layout_marginTop="30dp"/>
</LinearLayout>
Now lets see the java code
MainActivity.java
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Color;
import android.os.Bundle;
import android.view.Gravity;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.LinearLayout;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
RadioGroup rg;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
rg = findViewById(R.id.rg);
RadioButton radio = findViewById(R.id.radio);
radio.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
if(b)
{
Toast.makeText(MainActivity.this, "male checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(MainActivity.this, "male unchecked", Toast.LENGTH_SHORT).show();
}
}
});
RadioButton radio2 = findViewById(R.id.radio2);
radio2.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
if(b)
{
Toast.makeText(MainActivity.this, "female checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(MainActivity.this, "female unchecked", Toast.LENGTH_SHORT).show();
}
}
});
rg.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup radioGroup, int i) {
RadioButton button = (RadioButton)radioGroup.findViewById(i);
if(button != null && i != -1)
{
Toast.makeText(MainActivity.this, button.getText()+"\t is selected", Toast.LENGTH_SHORT).show();
}
}
});
Button clear = findViewById(R.id.clear);
clear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
rg.clearCheck();
}
});
}
}
Output:
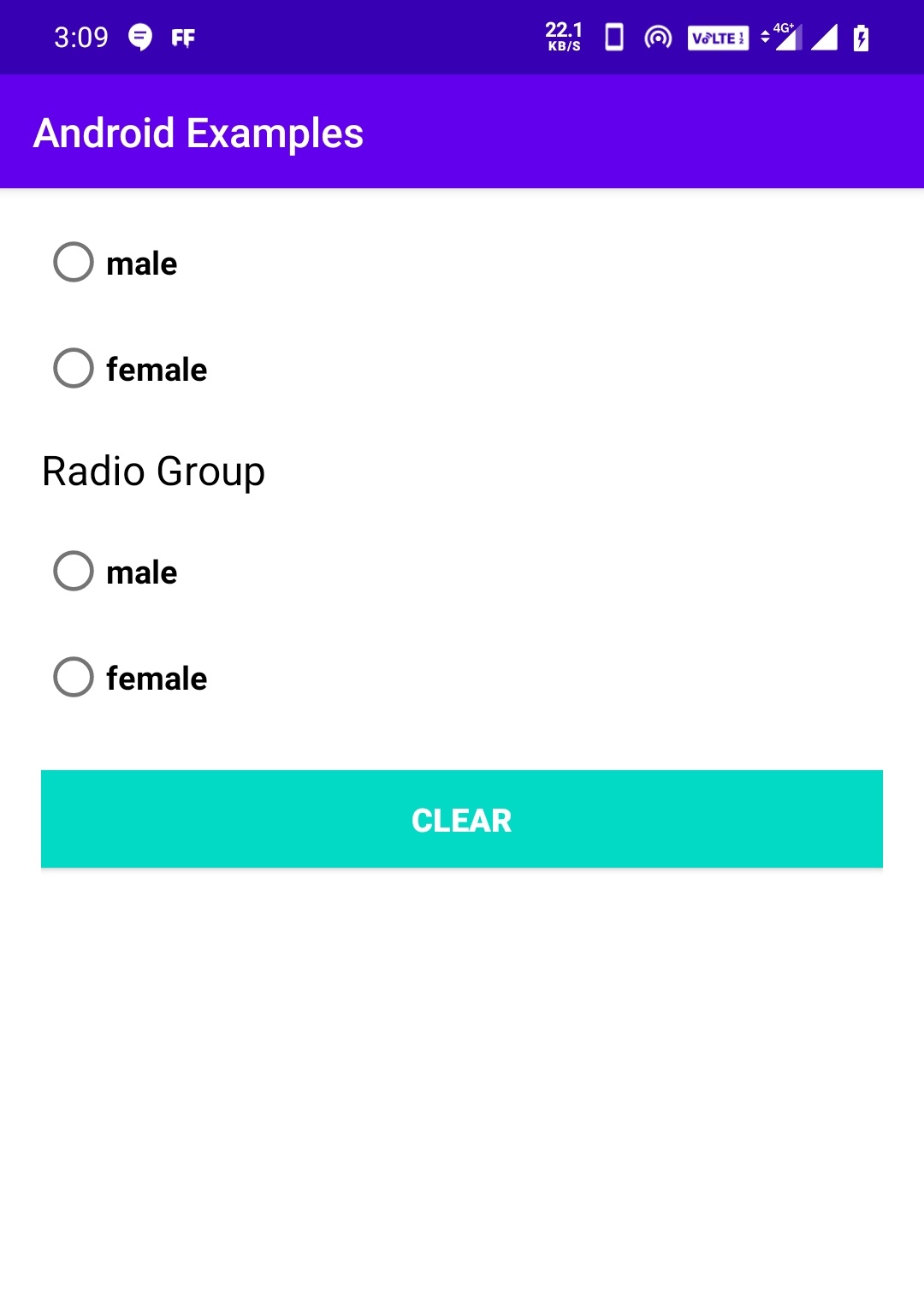
That’s all about android radio button.In this example tutorial. We have seen how to implement a radio button and its attributes with example. We will discuss about other android ui controls in next posts.
Do like and share if you find this post helpful.Thank you!!
Reference: Android Official Documentation.

Leave a Reply