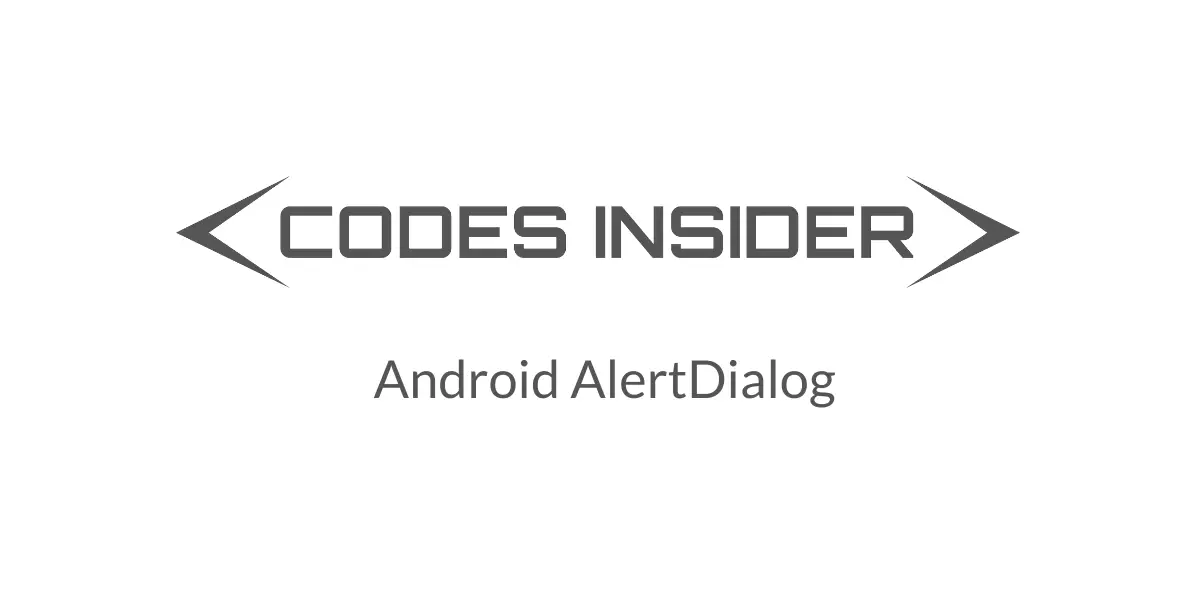
Android alertDialog is used in cases like asking for confirmation / making a decision when the user submits a form in android application or when the user want to delete something in the application.It’s just a confirmation from the user to proceed further.In this tutorial we will discuss how to display alertDialog in android studio with example.
Android AlertDialog
Android alertDialog is a small pop up window used to prompt some information to the user.Its nothing but a confirmation alert where the user approves or cancels the action.AlertDialog is a subclass of Dialog where we can display upto 3 buttons depending on the event.
AlertDialog mainly contains three components the title, content and action buttons.
Declaring an alertDialog
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this);
AlertDialog preview
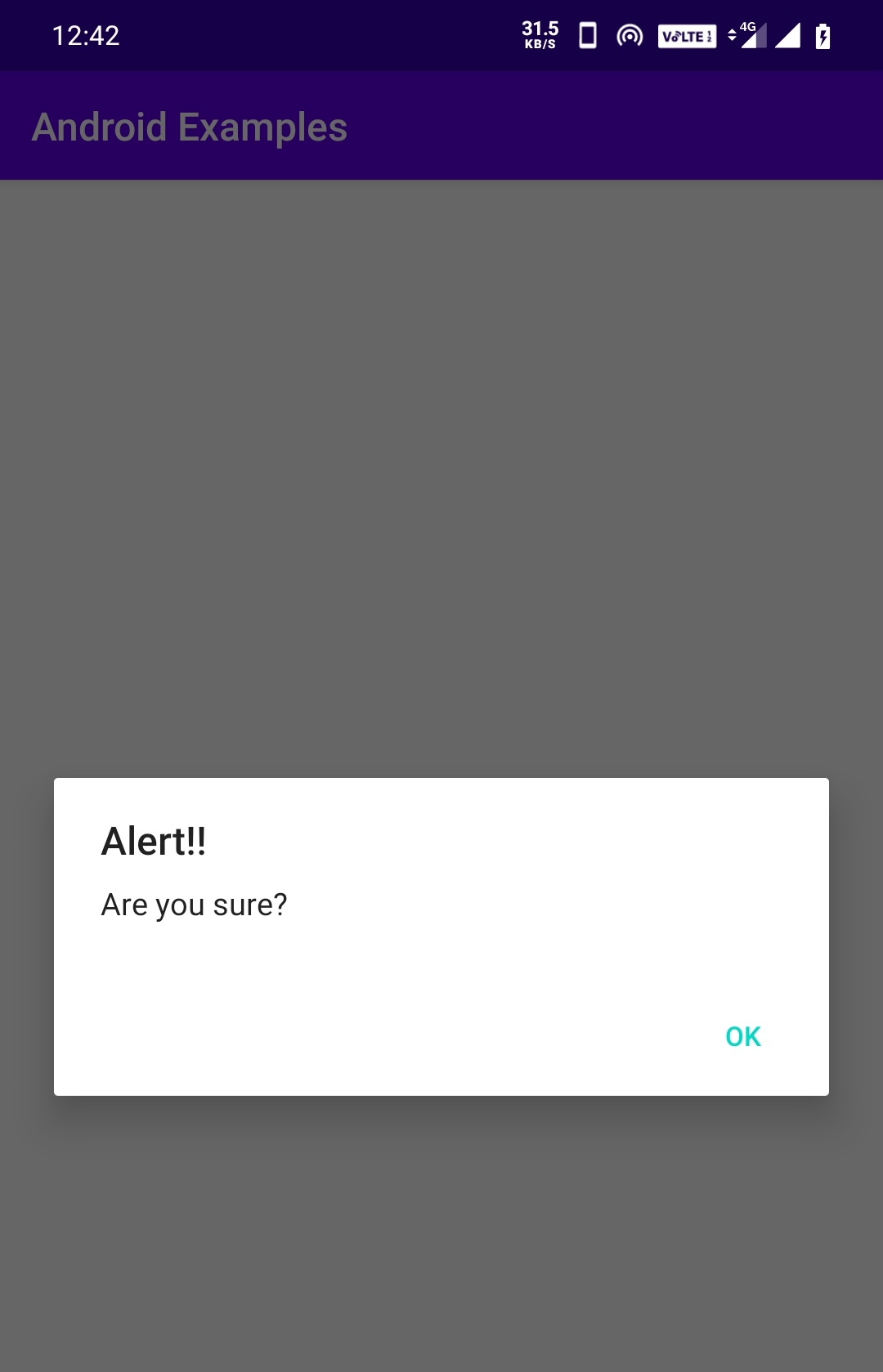
Check this android UI controls tutorial to know about UI controls in android.
Android AlertDialog Methods
Android alertDialog offers some methods to customize the dialog lets see them.
- setTitle(): This method is used to set title to the alertDialog(optional)
- setIcon(): This method is used to set icon to alertDialog which appears before the title.The icon is a drawable file.
- setMessage(): This method is used to set the message we want to display to the user in the alertDialog.
- setCancellable(): This method is used to set whether the user can cancel the alertDialog by clicking outside the dialog anywhere on the screen by setting true/false.
- setPositiveButton(): This method is used to set a positive button for alertDialog.A click event can be implemented for this button.
- setNegetiveButton(): This method is used to set a negative button for alertDialog.A click event can be implemented for this button.
- setNeutralButton(): This method is used to set a neutral button for alertDialog.A click event can be implemented for this button.
Android AlertDialog Example With Single Button
The following code will create an alertDialog with single button
package com.codesinsider;
import android.content.DialogInterface;
import android.os.Bundle;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this);
alertDialogBuilder.setTitle("Alert!!");
alertDialogBuilder.setMessage("AlertDialog with single button");
alertDialogBuilder.setPositiveButton("OK", new DialogInterface.OnClickListener()
{
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(MainActivity.this, "You clicked ok", Toast.LENGTH_SHORT).show();
}
});
alertDialogBuilder.show();
}
}
Output:
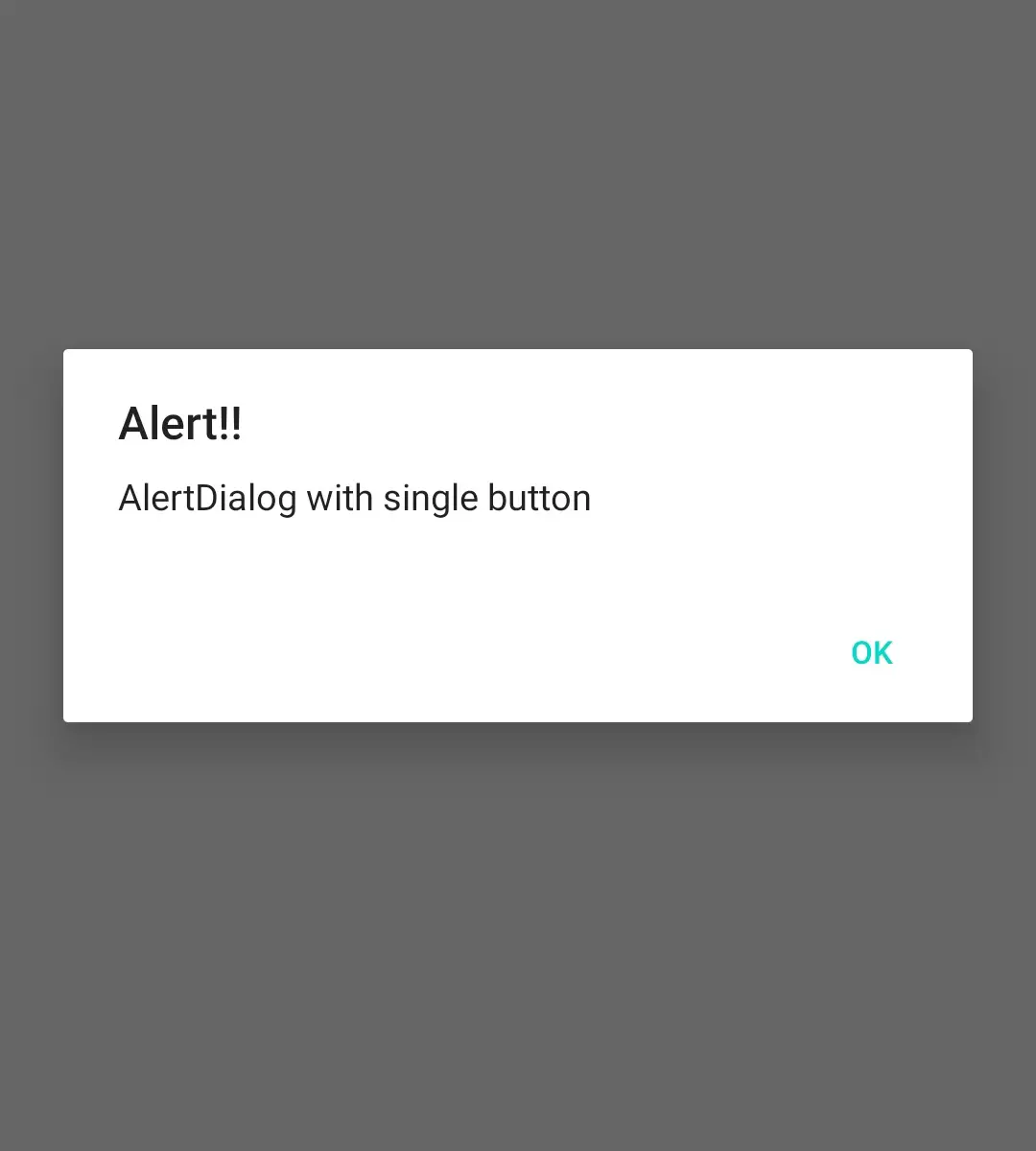
Android AlertDialog Example With Two Buttons
The following code will display an alertDialog with two buttons.
package com.codesinsider;
import android.content.DialogInterface;
import android.os.Bundle;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this);
alertDialogBuilder.setTitle("Alert!!");
alertDialogBuilder.setMessage("AlertDialog with two buttons");
alertDialogBuilder.setPositiveButton("YES", new DialogInterface.OnClickListener()
{
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(MainActivity.this, "You clicked yes", Toast.LENGTH_SHORT).show();
}
});
alertDialogBuilder.setNegativeButton("NO", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(MainActivity.this, "You clicked no", Toast.LENGTH_SHORT).show();
}
});
alertDialogBuilder.show();
}
}
Output:
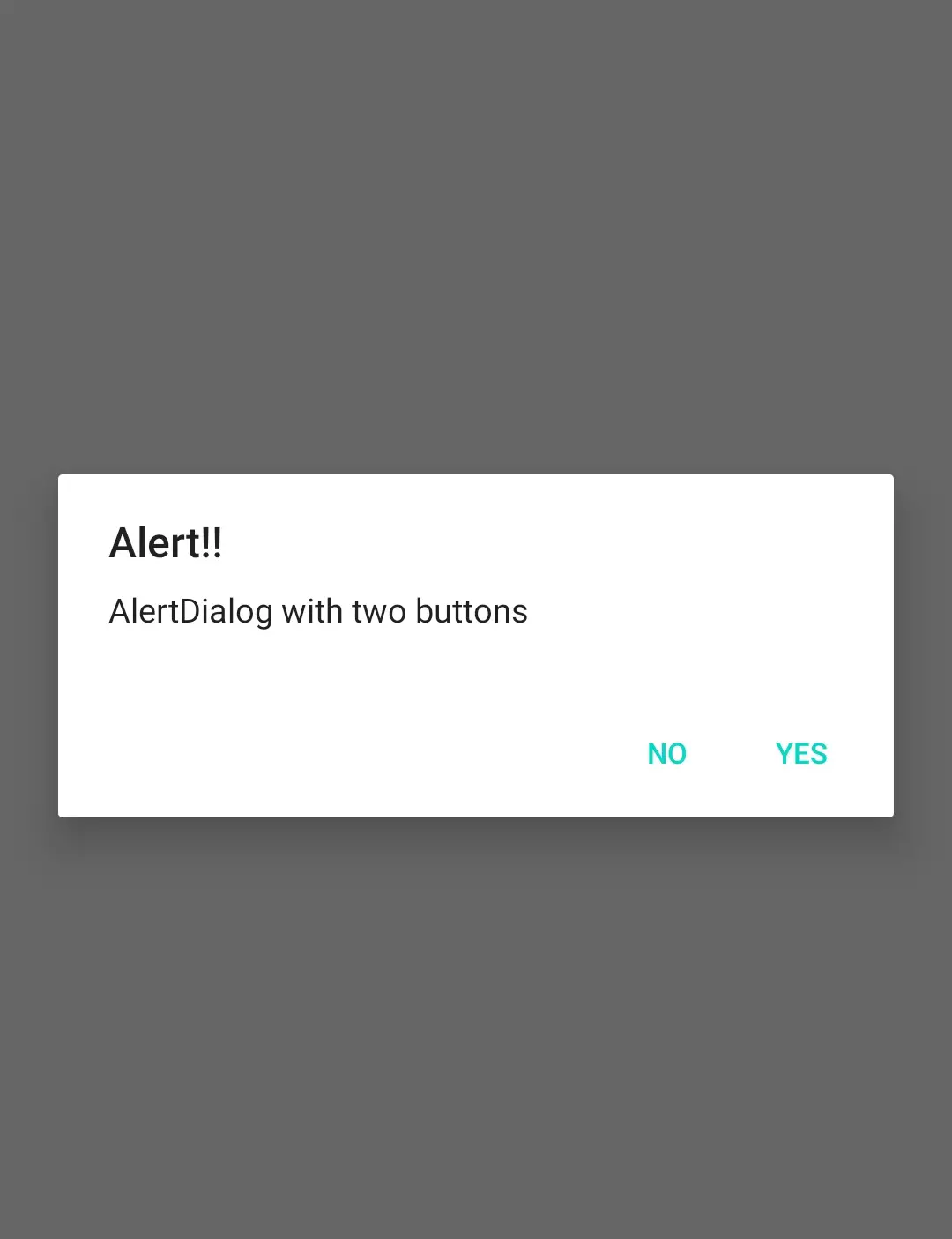
Android AlertDialog Example With Three Buttons
The following code creates an alertDialog with three buttons.
package com.codesinsider;
import android.content.DialogInterface;
import android.os.Bundle;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this);
alertDialogBuilder.setTitle("Alert!!");
alertDialogBuilder.setMessage("AlertDialog with three buttons");
alertDialogBuilder.setPositiveButton("YES", new DialogInterface.OnClickListener()
{
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(MainActivity.this, "You clicked yes", Toast.LENGTH_SHORT).show();
}
});
alertDialogBuilder.setNegativeButton("NO", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(MainActivity.this, "You clicked no", Toast.LENGTH_SHORT).show();
}
});
alertDialogBuilder.setNeutralButton("CANCEL", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(MainActivity.this, "You clicked cancel", Toast.LENGTH_SHORT).show();
}
});
alertDialogBuilder.show();
}
}
Output:
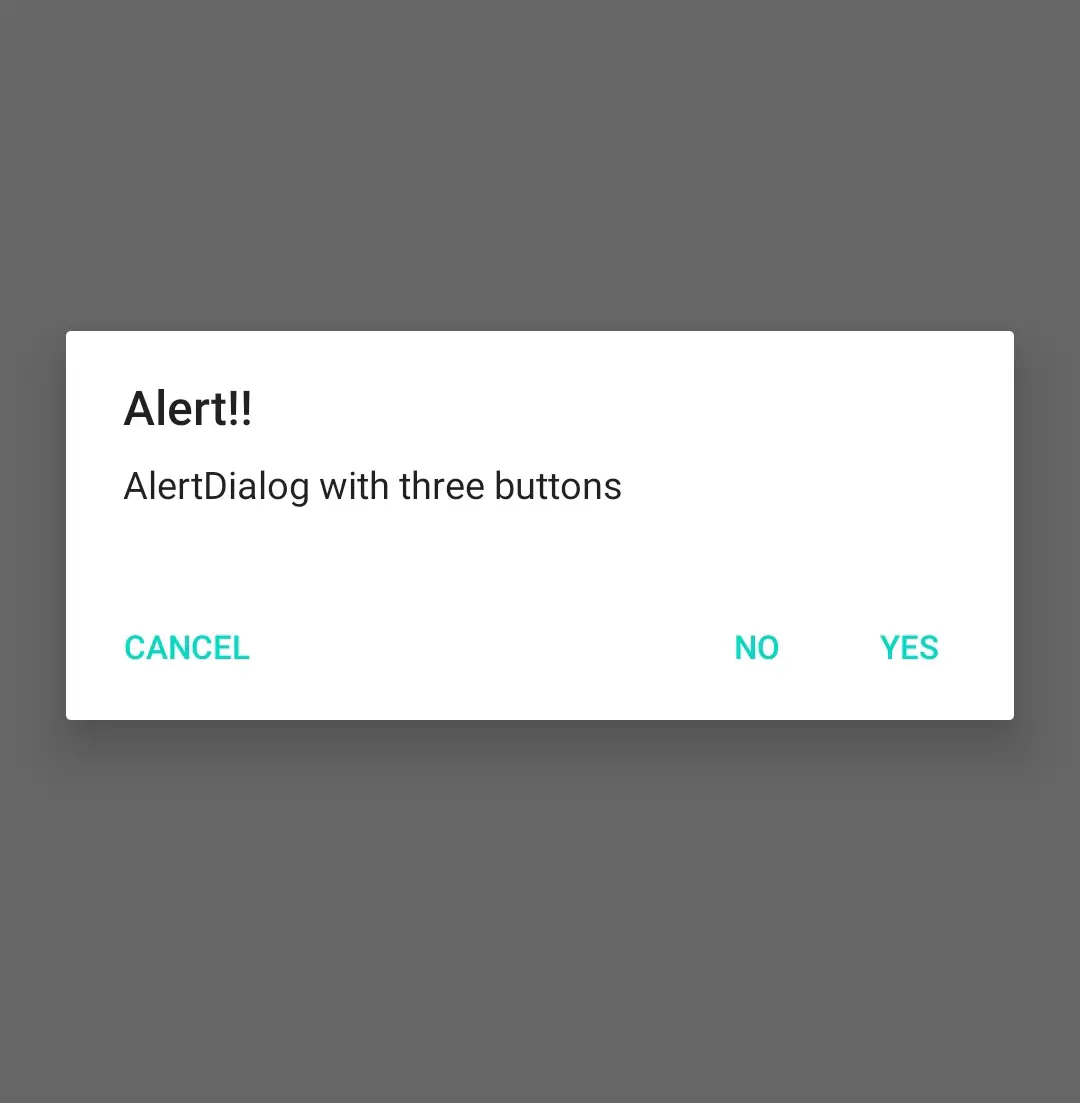
That’s all about android alertDialog example in detail with all the components and methods with example.Lets see other android concepts in next tutorials.
Do like and share if you find this post helpful.Thank you!!
Reference: Android official documentation

Leave a Reply