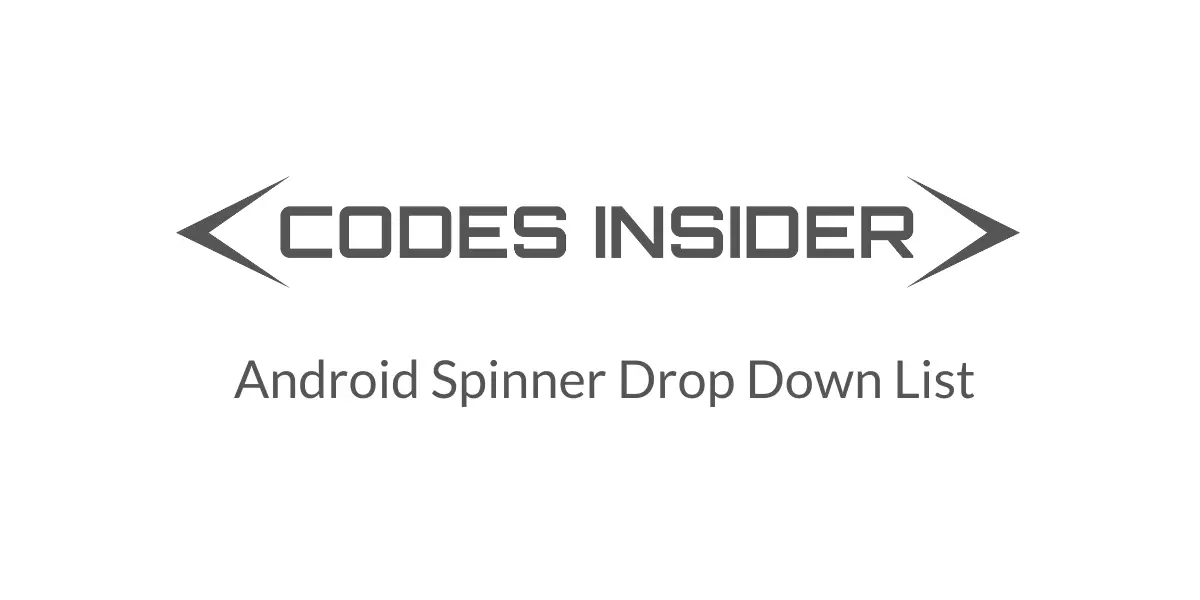
In this android spinner drop down list example tutorial we will see how to implement a spinner in android application development with example in android studio.
Android Spinner drop down list
Android spinner is nothing but a drop down list just like the drop down list we see in websites.Android spinner is used to select one value from list of values.By default the spinner displays selected value.When the user touches the spinner it displays list of available values.
Check this android UI controls tutorial to know more about UI controls.
Android Spinner code in XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:padding="20dp"
android:background="#ffffff"
android:id="@+id/main">
<androidx.appcompat.widget.AppCompatSpinner
android:layout_width="match_parent"
android:layout_height="40dp"
/>
</LinearLayout>
Android spinner code in JAVA
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.AppCompatSpinner;
import android.graphics.Color;
import android.os.Bundle;
import android.view.Gravity;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.LinearLayout;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Space;
import android.widget.Spinner;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Defining spinner programatically
AppCompatSpinner spinner = new AppCompatSpinner(this);
LinearLayout layout = findViewById(R.id.main);
layout.addView(spinner);
//Identifying spinner defined in xml layout
AppCompatSpinner spin = findViewById(R.id.spinner);
}
}
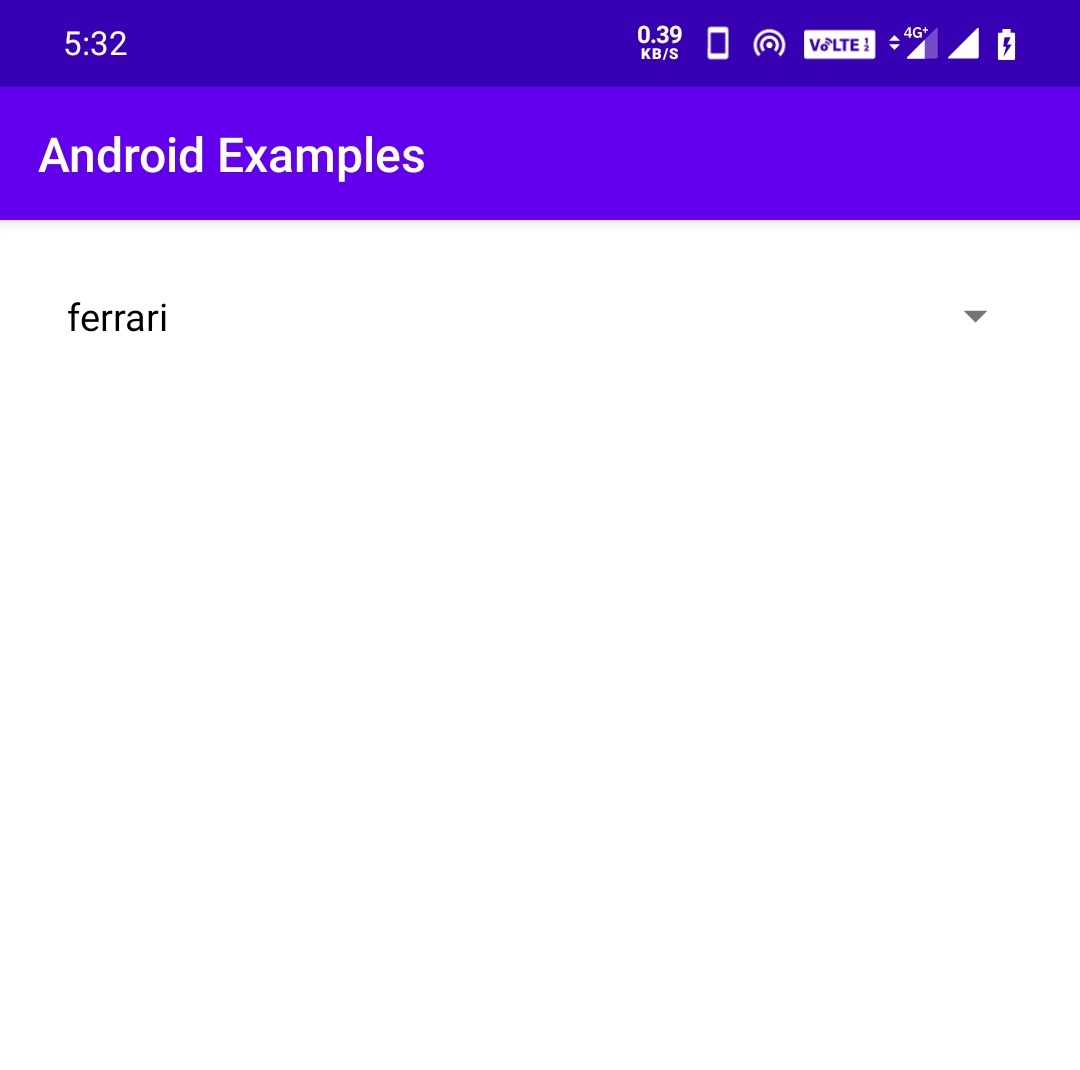
To display data in a spinner we need to set the spinner with an adapter.We need an arrayList to store the spinner data and an array adapter to set the values in arrayList to spinner as the spinner is linked with adapter view.
Lets dive into the code..
Adding data to display in spinner
Creating an arrayList and adding data into arrayList.Here we are creating an arrayList of string type and adding data to display in spinner.
ArrayList<String>list = new ArrayList<>();
list.add("ferrari");
list.add("lambo");
list.add("audi");
list.add("mercedes");
list.add("bmw");
Defining ArrayAdapter
The Array adapter require 3 arguments where first one is the context, second one is the layout for spinner item and third one is the array list with data to display in spinner.
ArrayAdapter adapter = new ArrayAdapter(this,R.layout.support_simple_spinner_dropdown_item,list);
Setting adapter to spinner
AppCompatSpinner spin = findViewById(R.id.spinner);
spin.setAdapter(adapter);
Android Spinner ItemClickListener / ItemSelectedListener
If we want to perform an action when an item from the spinner is selected, we are provided with two methods.
1.itemClickListener
2.itemSelectedListener
We can use any method as both the methods work in the same way
AppCompatSpinner spin = findViewById(R.id.spinner);
//Item click listener
spin.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
String value = adapterView.getItemAtPosition(i).toString();
Toast.makeText(MainActivity.this, value, Toast.LENGTH_SHORT).show();
}
});
//Item selected listener
spin.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
String value = adapterView.getItemAtPosition(i).toString();
Toast.makeText(MainActivity.this, value, Toast.LENGTH_SHORT).show();
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
}
});
In the above code we are displaying the selected item text in a toast when the user selects the item from the spinner dropdown.
Now Lets see the complete code
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:padding="20dp"
android:background="#ffffff"
android:id="@+id/main">
<androidx.appcompat.widget.AppCompatSpinner
android:id="@+id/spinner"
android:layout_width="match_parent"
android:layout_height="40dp"
/>
</LinearLayout>
ActivityMain.java
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.AppCompatSpinner;
import android.graphics.Color;
import android.os.Bundle;
import android.view.Gravity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.LinearLayout;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Space;
import android.widget.Spinner;
import android.widget.Toast;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Identifying spinner defined in xml layout
AppCompatSpinner spin = findViewById(R.id.spinner);
ArrayList<String>list = new ArrayList<>();
list.add("ferrari");
list.add("lambo");
list.add("audi");
list.add("mercedes");
list.add("bmw");
final ArrayAdapter adapter = new ArrayAdapter(this,R.layout.support_simple_spinner_dropdown_item,list);
spin.setAdapter(adapter);
spin.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
String value = adapterView.getItemAtPosition(i).toString();
Toast.makeText(MainActivity.this, value, Toast.LENGTH_SHORT).show();
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
}
});
}
}
Output:
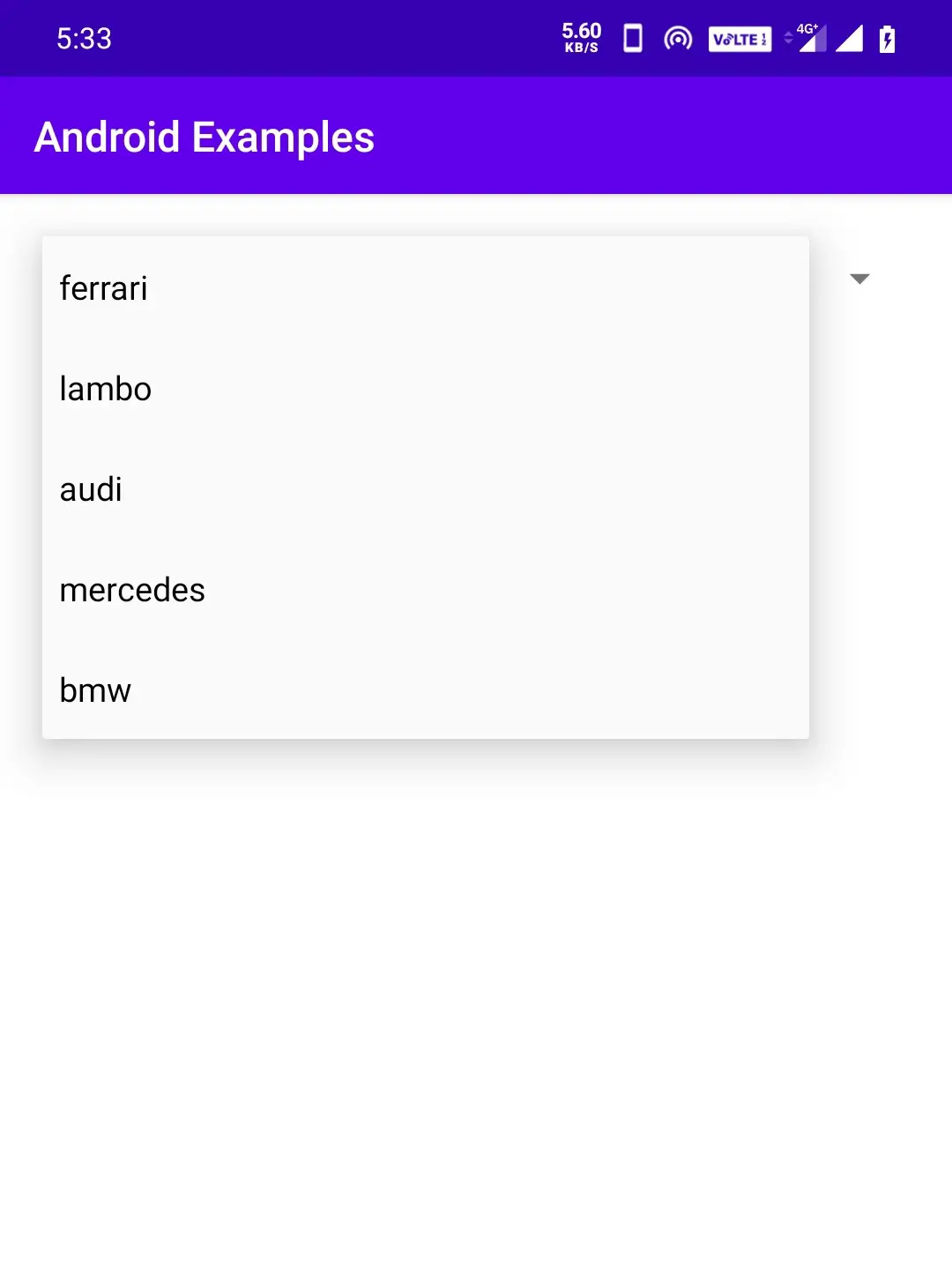
So lets discuss what we have done so far.In the above code we first defined a spinner and then we declared an arrayList and added the data(cars names) to be displayed in the spinner.After adding the data we defined an ArrayAdapter and set the ArrayAdapter to spinner.
We set ItemSelectedListener on spinner object and displayed a toast message with the selected item name when the user selects the item.
That’s all about android spinner drop down list example tutorial.Lets catch up with another cool android tutorial soon.
Happy Coding & have a great day!!
Do like and share if you find this post helpful.Thank you!!
Reference: Android Official Documentation.

Leave a Reply