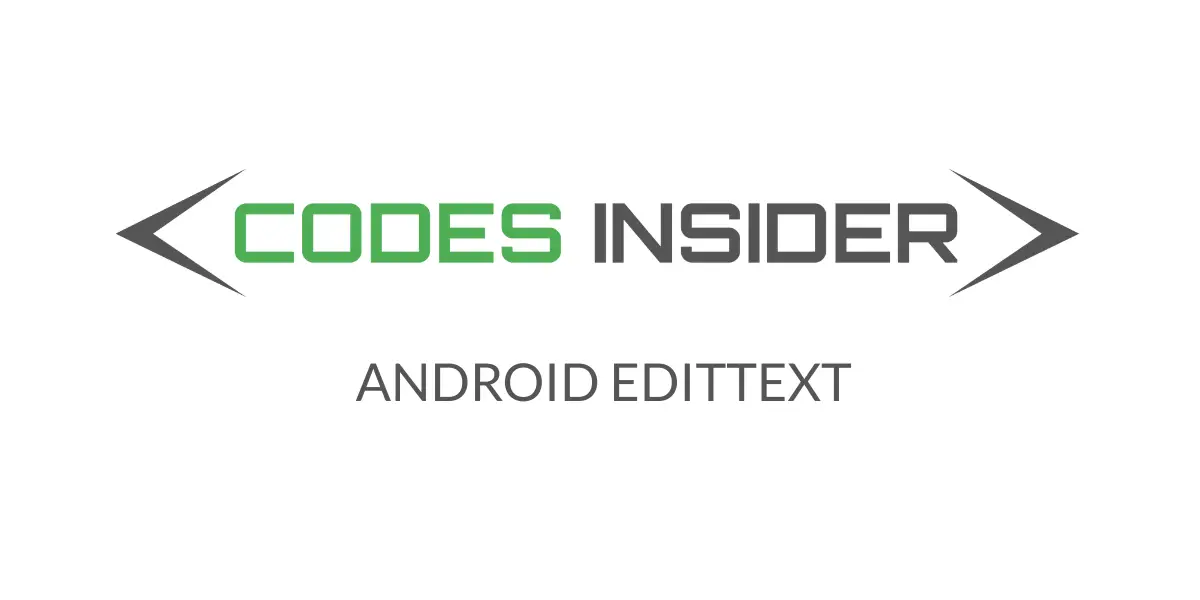
In this android EditText example tutorial you will learn what is an EditText, how to create it and different types of attributes for EditText with examples in android studio.
Android EditText
In android EditText is a user interface control which is used to accept input from the user or to edit the text.EditText is nothing but a text field in HTML which accepts input.EditText is a sub class of TextView that configures itself to be editable.
Check this android UI controls tutorial to know more about UI controls.
EditText is subclass of View class which is base class for all the UI controls in android.Since it is a sub class of View class we can use it inside a View Group or as a root element in android layout file.
Defining EditText in Android
Defining EditText in android can be done in two ways, one is by declaring it inside a layout(XML) file and the second one is by creating an object of it in JAVA.
Defining EditText in XML(layout)
Example code snippet for defining EditText in XML.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@color/white"
android:id="@+id/textlaout"
android:padding="20dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
Defining EditText in JAVA(programatically)
Example code snippet for defining EditText in JAVA.
package com.codesinsider.androidui;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.LinearLayout;
import android.widget.TextView;
public class LayoutExample extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.linear_laout);
EditText email = new EditText(this);
//setting width and height of textview
email.setLayoutParams(new LinearLayout.LayoutParams(50, 50));
LinearLayout layout = findViewById(R.id.linear);
layout.addView(email);
}
}
Basic Android EditText
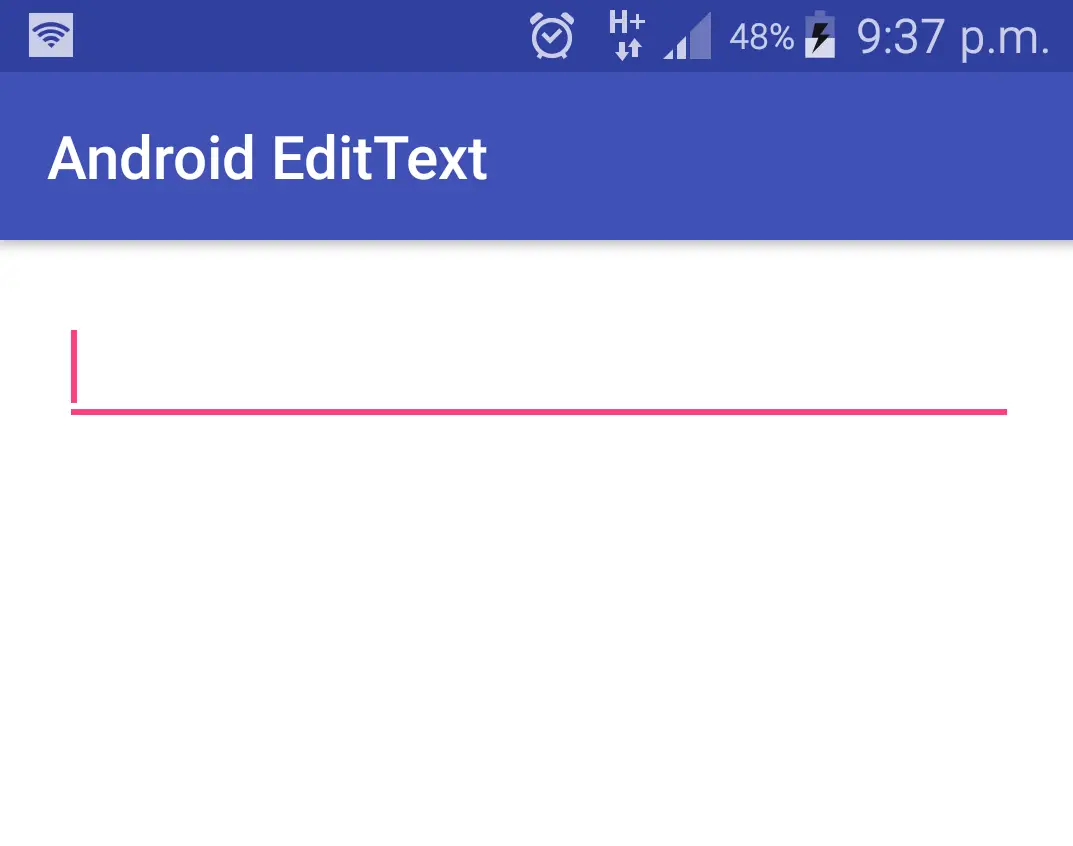
Android EditText Attributes
Like all the UI controls in android EditText also has some attributes, so lets see the most commonly used attributes of EditText in detail.
id : The id is the most common attribute used to identify the View or ViewGroup created in xml file in java.
Example code snippet for setting an id to an EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
gravity : gravity attribute is used to position the text of the EditText to right, left, top, bottom, centre_vertical, center_horizontal etc.By default the gravity is start(left).
Example code snippet for setting the gravity of EditText to center in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Codes insider"
android:gravity="center"
/>
Example code snippet for setting gravity of EditText in JAVA.
EditText email = findViewById(R.id.email);
email.setGravity(Gravity.CENTER);
Output of the above code snippets.
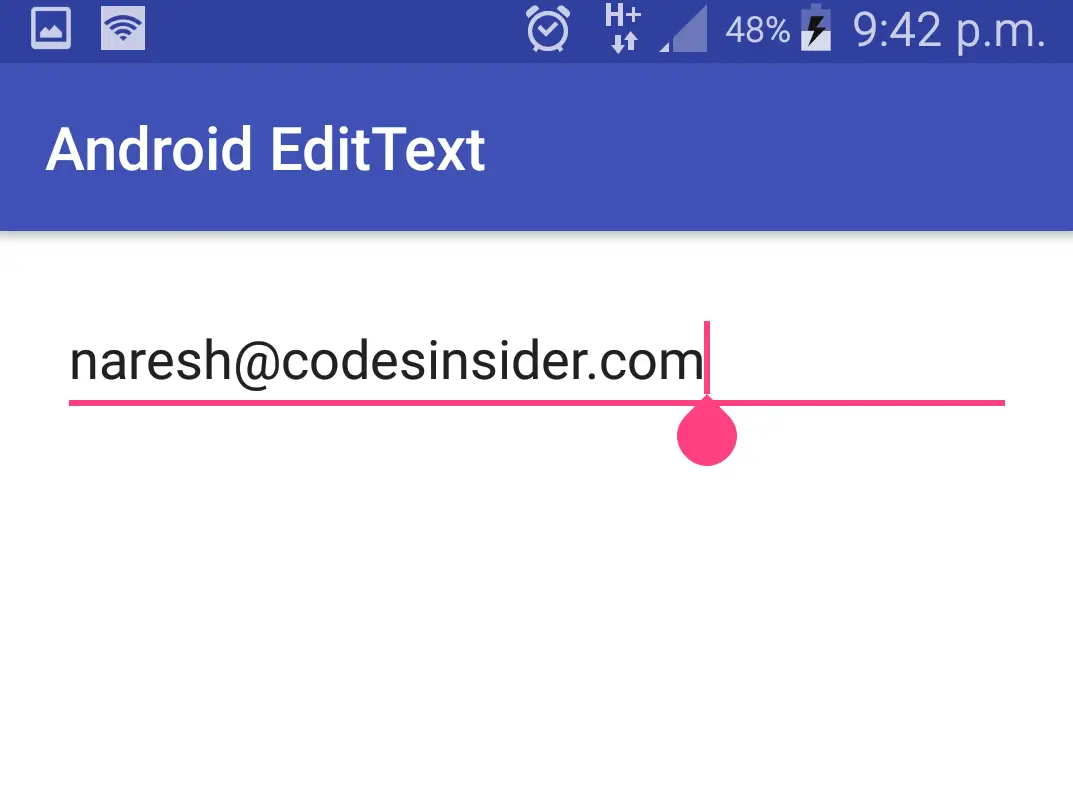
layout_gravity : layout gravity is similar to gravity but positions the entire EditText layout instead of text.By default the layout gravity is start(left).
Example code snippet for EditText with layout_gravity as center_horizontal in XML.
<EditText
android:id="@+id/email"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="Codes insider"
android:layout_gravity="center_horizontal"
android:gravity="start"
/>
Below is the output of the above code snippet.If we observe the code the layout gravity is center_horizontal which is why the layout is positioned center of screen and the gravity is set to start, for which the text is at the starting of the EditText.
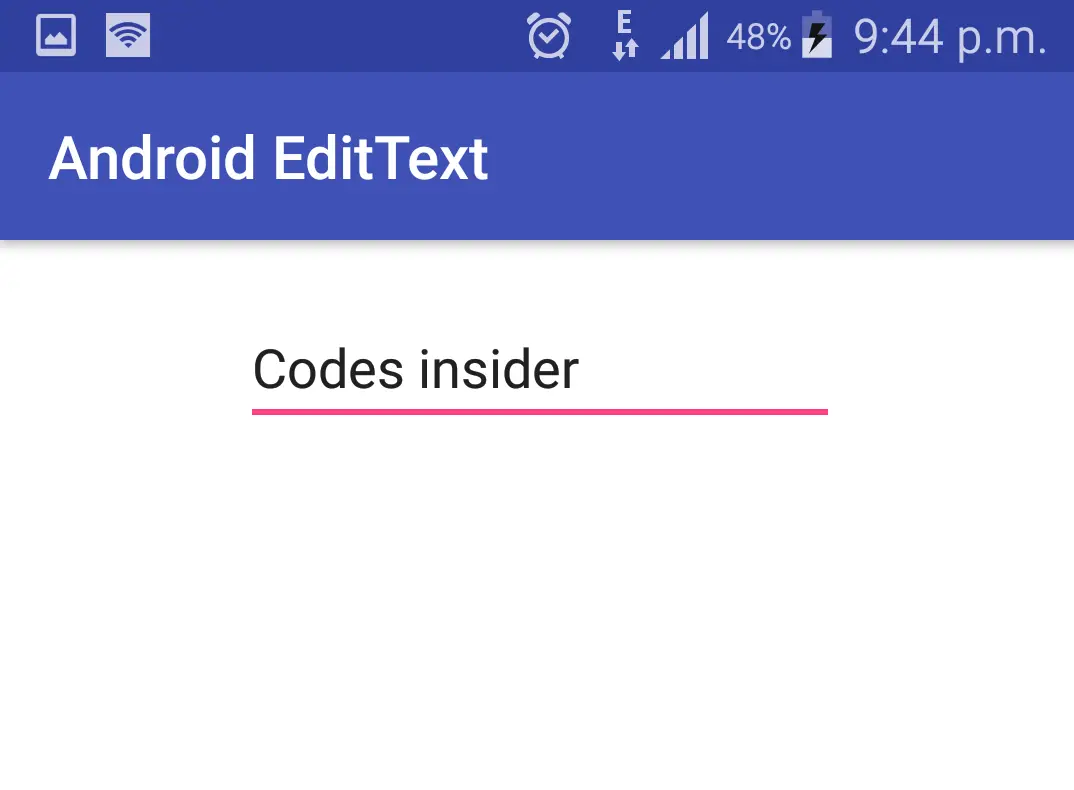
text : Text attribute is used to set the text to EditText.
Example code snippet for setting text to a EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Codes insider"
/>
Example code snippet for setting text to EditText in JAVA.
EditText email = findViewById(R.id.email);
email.setText("Email address");
Output of the above code snippets.
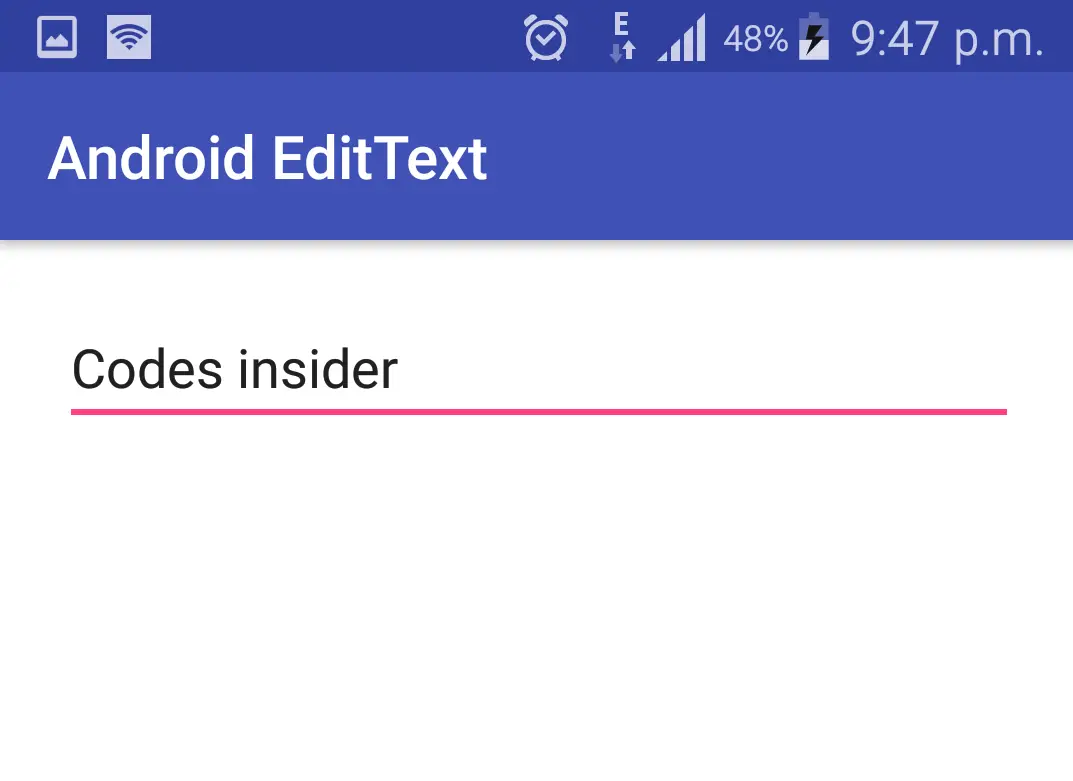
Note: The standard way of using strings, colors and dimentions in android was explained clearly in using strings, colors, dimens in UI controls section of this Android UI Controls tutorial.
hint : hint in android is similar to placeholder in html which is used to inform the user what type of data should be entered in the EditText.
Example code Snippet for setting hint to EditText in XML
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
/>
Example code snippet for setting hint to EdtText in JAVA.
EditText email = findViewById(R.id.email);
email.setHint("Email"); //setting hint
Output of the above code snippets.
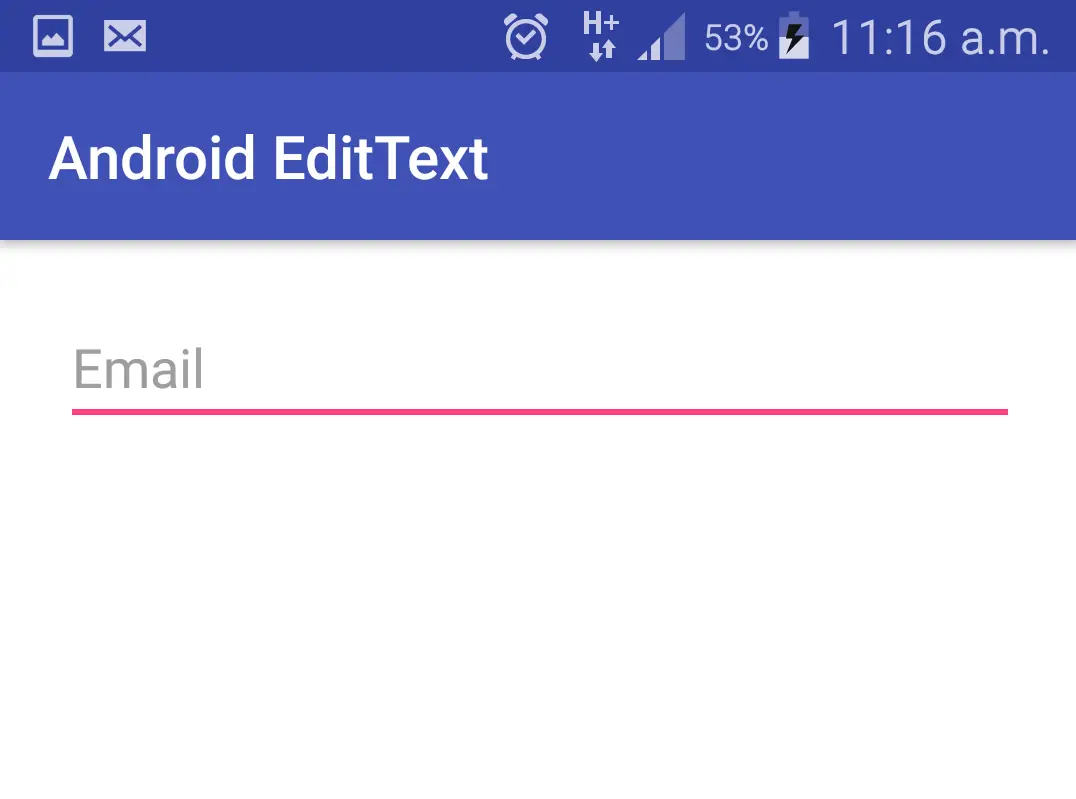
textColorHint : textColorHint is used to set the color to hint text.
Example code snippet for setting textColorHint to EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:textColorHint="@color/green"
/>
Example code snippet for setting textColorHint to EditText in JAVA.
EditText email = findViewById(R.id.email);
email.setHint("Email");
email.setHintTextColor(getResources().getColor(R.color.green)); //setting hint color
Output of the above code snippets.
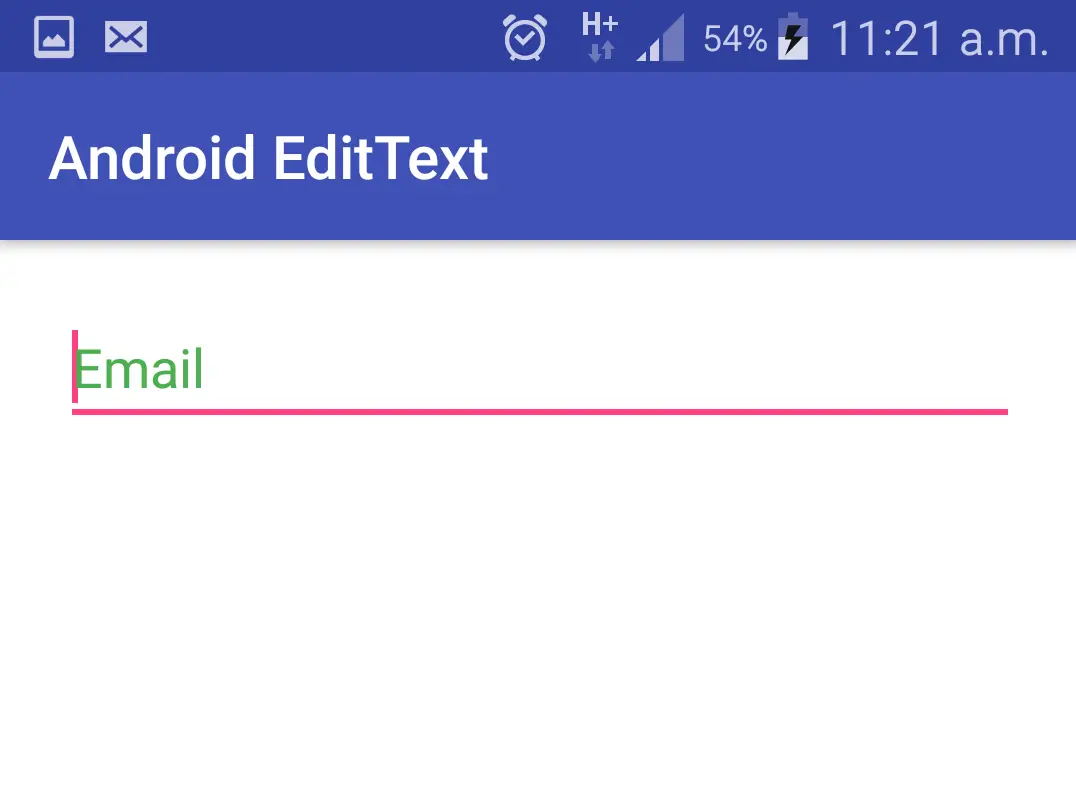
inputType : Every EditText expects a certain type of text input, such as an email address, phone number, or just plain text. So it’s important that you specify the input type for each EditText in your app so the system displays the appropriate soft input method like if we set inputType to phone the android system displays number keypad when we click on that EditText.
Though there are man input types available i’m just listing a few most commonly used input types below
- date
- phone
- number
- text
- textEmailAddress
- textPassword
Example code snippet for setting inputType for EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:text="Codes Insider"
android:inputType="phone"
android:textColorHint="@color/green"
/>
Output of the above code snippets.
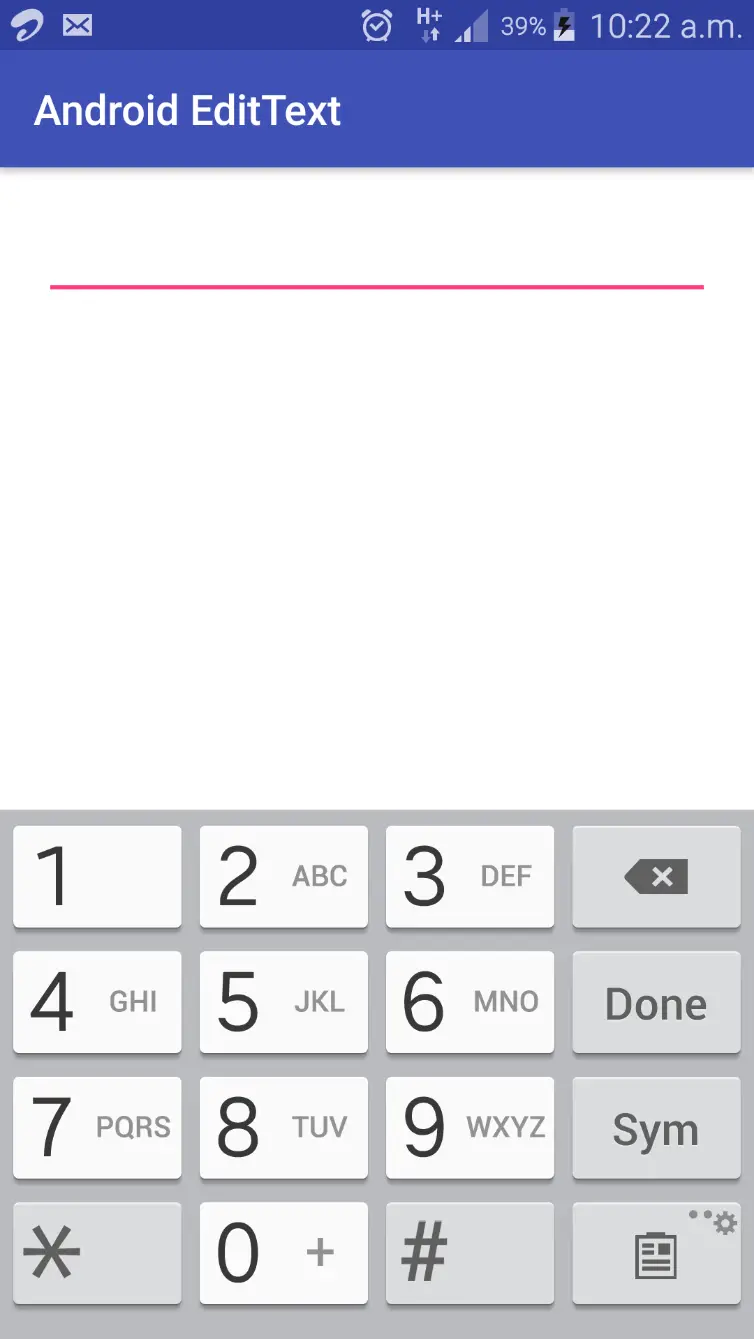
textColor : textColor attribute is used to set the text color.The value for textColor can be “#argb”, “#rgb”, “#rrggbb”, or “#aarrggbb”.
Example code snippet for setting text color of an EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:text="Codes insider"
android:textColor="@color/green"
/>
Example code snippet for setting text color of an EditText in java
EditText email = findViewById(R.id.email);
email.setHint("Email");
email.setTextColor(getResources().getColor(R.color.green)); //setting text color
Output of the above code snippets.
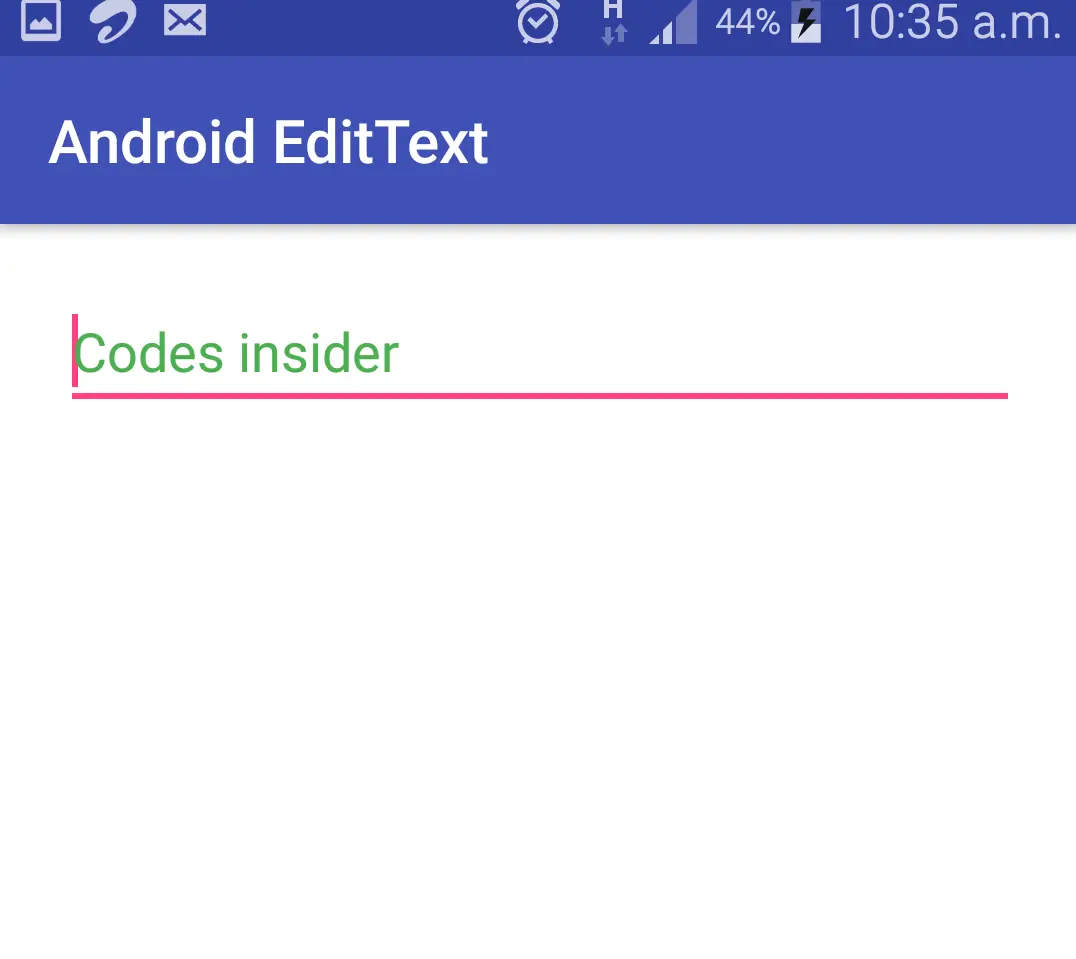
textSize : textSize attribute is used to set the text size of a EditText whose value can be either in sp(scale-independent pixels) or dp(density independent pixels) like for example 20sp or 20dp
Example code snippet for setting textSize of an EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:text="Codes insider"
android:textColor="@color/black"
android:textSize="20sp"
/>
Example code snippet for setting textSize of an EditText in JAVA.
EditText email = findViewById(R.id.email);
email.setText("Codes insider");
email.setTextSize(20);
Output of the above code snippets.
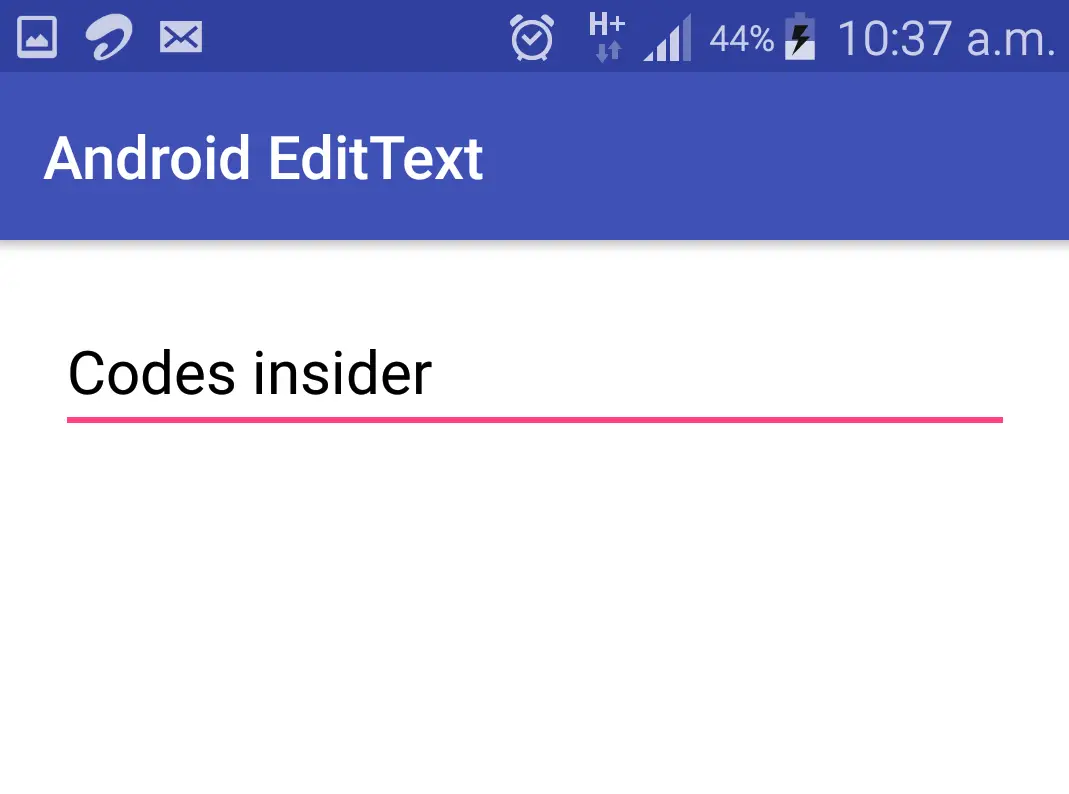
textStyle : textStyle attribute is used to style the text of the EditText.There are three values for a textStyle attribute like normal, bold, italic.By default it is normal.If we want to use more than one value at a time we can use “|” operator. like bold|italic.
Example code snippet for setting text style of a EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:text="Codes insider"
android:textColorHint="@color/green"
android:textColor="@color/black"
android:textSize="20sp"
android:textStyle="bold|italic"
/>
Output of the above code snippets.
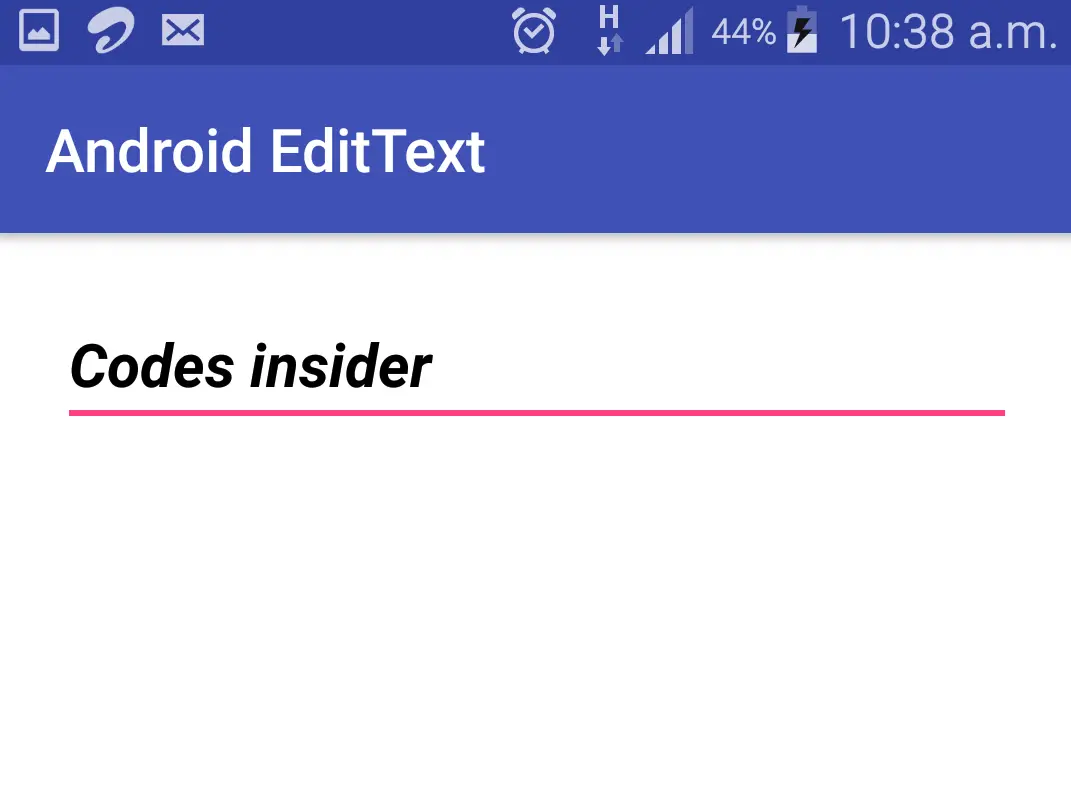
fontFamily : fontFamily attribute is used to set the font family like roboto, open sans etc to the EditText.
Example code snippet for setting fontFamily of EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:text="Codes insider"
android:textColor="@color/black"
android:textSize="20sp"
android:fontFamily="monospace"
/>
Output of the above code snippets.
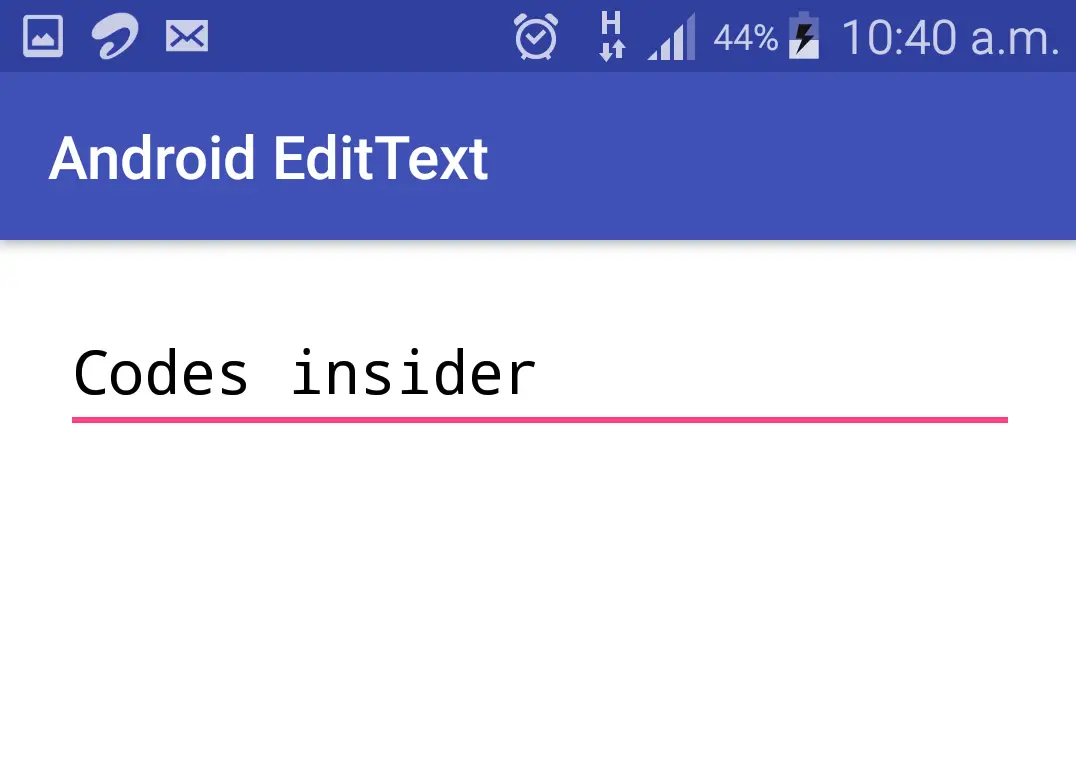
background : background attribute is common for all the layouts and UI controls which is used to set background.Background can either be a color, image or a drawable resource file.
Example code snippet for setting background to EditText in XML.
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Codes insider"
android:textColor="@color/black"
android:textSize="20sp"
android:background="@color/green"
/>
Example code snippet for setting background of EditText in JAVA.
EditText email = findViewById(R.id.email);
email.setText("Codes insider");
email.setBackgroundColor(getResources().getColor(R.color.green));
email.setBackgroundResource(R.color.green);
Output of the above code snippets.
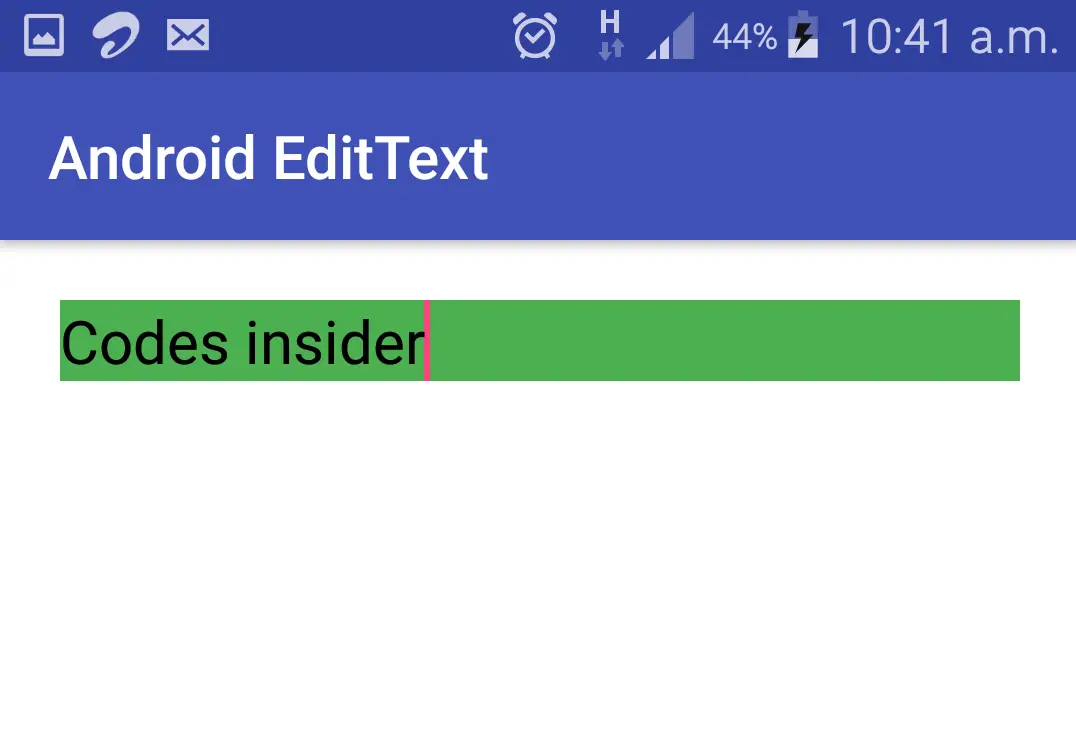
We can also use a drawable resource for EditText as background.A drawable resource is nothing but an image or a drawable resource file(drawable type xml file).All the images that we use locally in android app should be saved at app>res>drawable, and to access the files in drawable directory we need to define like android:background=”@drawable/filename“.Lets see the example code snippets below.
Example code snippet for setting drawable as background to EditText in XML
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="80dp"
android:text="Codes insider"
android:textColor="@color/white"
android:textSize="20sp"
android:background="@drawable/back"
/>
Example code snippet for setting drawable as background to EditText in JAVA
EditText email = findViewById(R.id.email);
email.setText("Codes insider");
email.setBackgroundResource(R.drawable.back);
Output of the above code snippets.
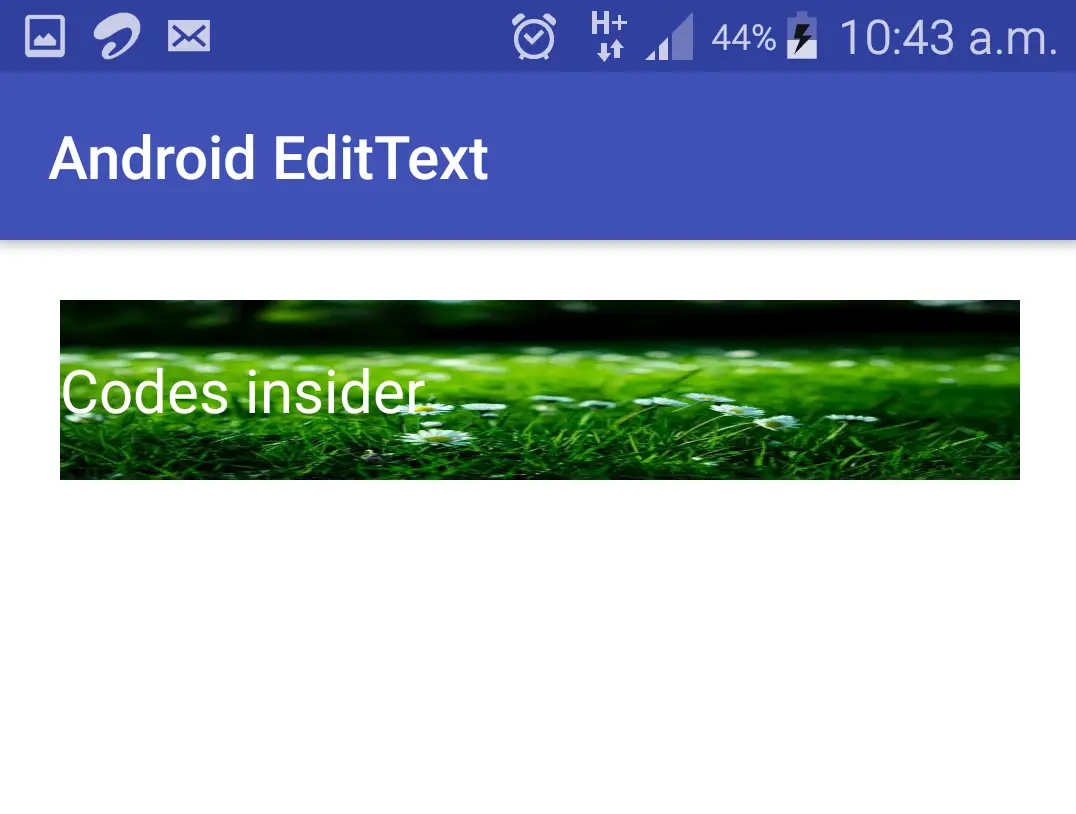
typeface :typeface attribute is used to set the typeface of the EditText among the four built in typefaces monospace, serif, sans, normal.
.To use custom font as typeface create a folder named assets in src>main and download .ttf file of your desired font like for example roboto.ttf and save it in the assets folder we created above.Your project will look like below
You can find .ttf files at Fontsquerrel, Google Fonts etc.
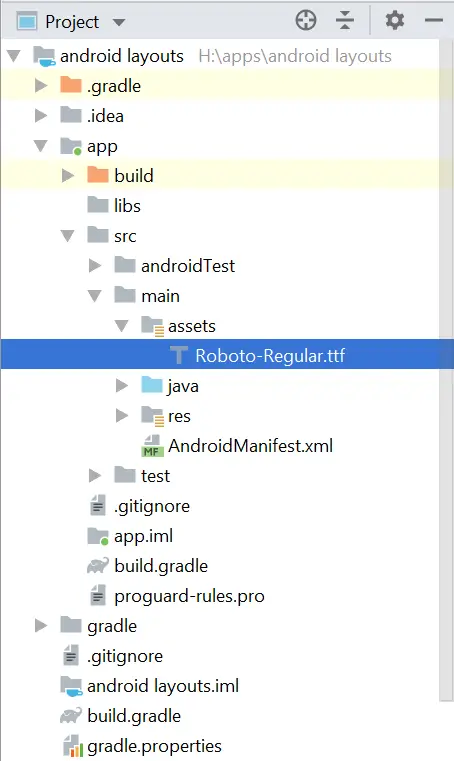
Example code snippet for setting typeface for EditText in XML
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="80dp"
android:text="Codes insider"
android:textColor="@color/white"
android:textSize="20sp"
android:background="@drawable/back"
android:typeface="monospace"
/>
Example code snippet for setting custom font as typeface for EditText in JAVA
EditText email = findViewById(R.id.email);
email.setText("Codes insider");
email.setTypeface(Typeface.createFromAsset(getAssets(),"Calibri Bold.TTF"));
Output of the above code snippets.
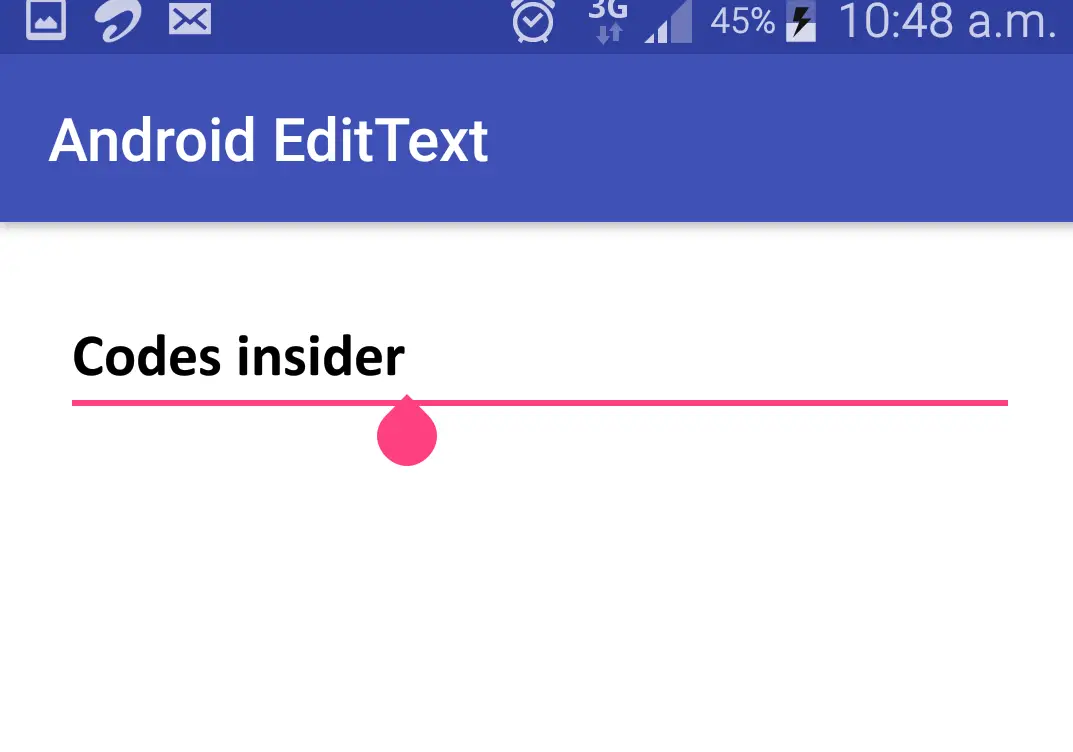
drawable : drawable attribute is used to set icons/images to any of the four sides of the text like left, right, top, bottom.It has four types like
drawable_Start : sets the icon/image to left of the text.
drawable_End : sets the icon/image to right of the text.
drawable_Top : sets the icon/image to the top of the text.
drawable_Bottom : sets the icon/image to bottom of the text.
There is also another attribute called drawable_padding which is used to set padding for the drawable icon/image.Eg drawable_padding=”10dp”.This is used to give space between the drawable icon and the text of the EditText.
Example code snippet for setting drawable for EditText in XML
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="username"
android:textColor="@color/black"
android:textSize="20sp"
android:drawableLeft="@drawable/user"
android:drawablePadding="10dp"
/>
Output of the above code snippets.
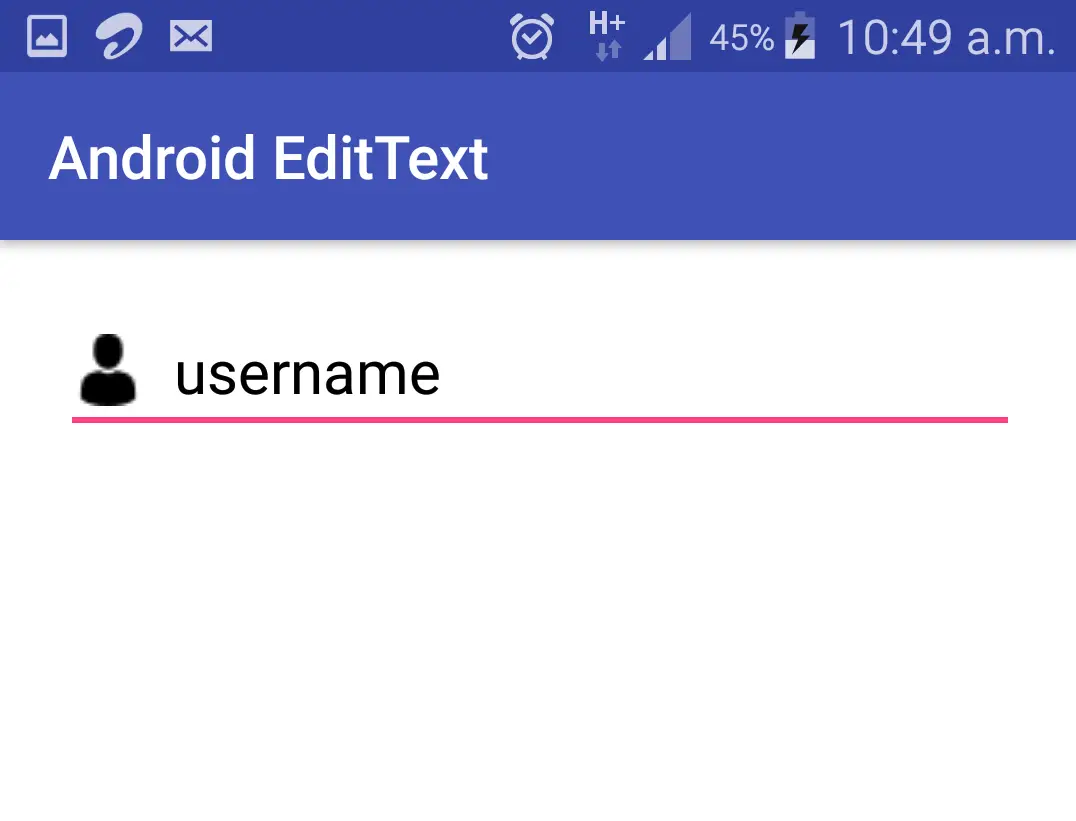
That’s all about the most commonly used attributes of EditText.To know the attributes that are common for all the elements refer the layout attributes section of this post about android layouts.
Displaing HTML in EditText
We can display HTML code in android by using fromHtml() method in Html class which is predefined in android libraries.Below is the code snippet to display HTML in EditText in android programatically (JAVA).
EditText email = findViewById(R.id.email);
email.setText(Html.fromHtml(
"<p><b>CodesInsider.com</b> <br>Android Tutorials</p>"));
Output of above code snippet.
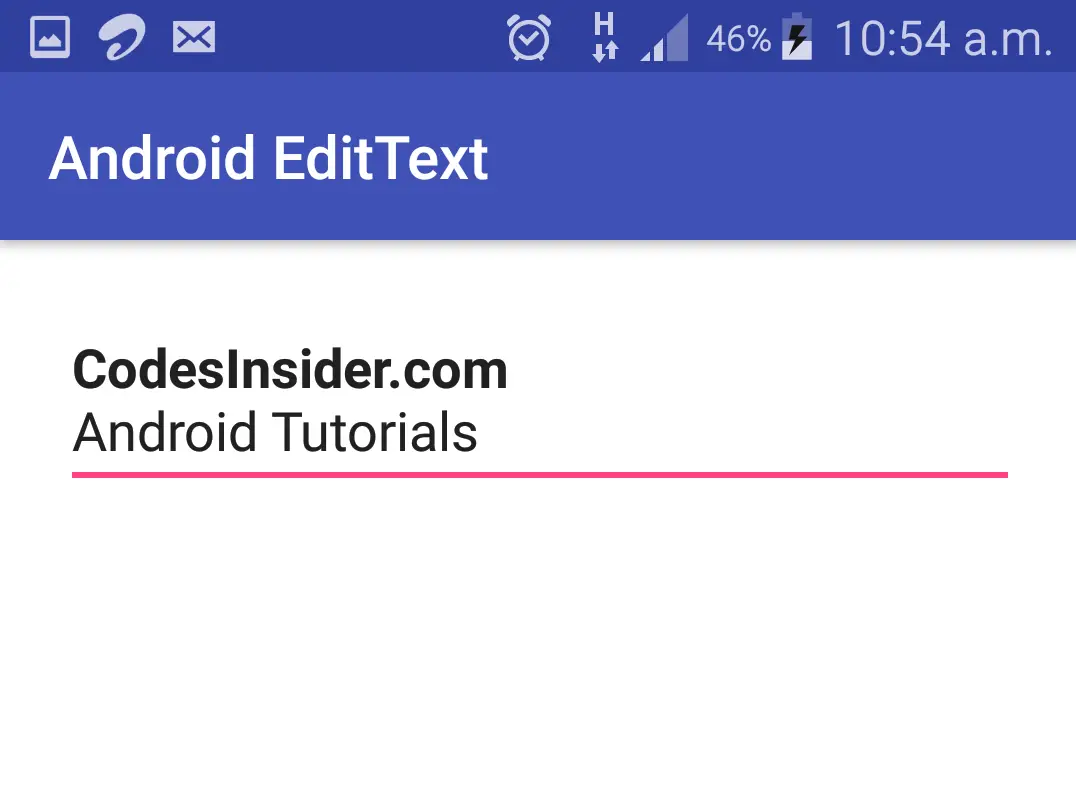
Click Listener for EditText
We can perform some action when the EditText is clicked by the user.This can be done in two ways.
One way is setting a method to onClick attribute in xml and the other is to invoke the onClickListener method on EditText object.
Example code snippet for setting click listener to EditText in XML
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="username"
android:textColor="@color/black"
android:textSize="20sp"
android:drawableLeft="@drawable/user"
android:drawablePadding="10dp"
android:onClick="testMethod"
/>
JAVA Code snippet to be included in Activity.java to trigger click listener using the above XML code.
public void testMethod(){
//Do what action you wanna perform here when the editText is clicked
}
Code snippet for triggering click event only in java without using XML.
EditText email = findViewById(R.id.email);
email.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Do what ever ou wanna do here when edit text is clicked
}
});
Getting Text From EditText
We can get text from EditText when an click or any other event is triggered by using getText() method.Lets see an example where we get text from EditText and setting that text to TextView on click of a button.
Code snippet to be included in XML file.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@color/white"
android:id="@+id/textlaout"
android:padding="20dp">
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:text="Codes Insider"
android:textColorHint="@color/green"
/>
<TextView
android:id="@+id/text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:textColor="@color/black"
android:textSize="18sp"/>
<Button
android:id="@+id/button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="get Text"
android:background="@color/green"
android:textColor="@color/white"
android:layout_marginTop="20dp"/>
</LinearLayout>
Code snippet to be included in JAVA
package androidlaouts.codesinsider.com.androidlayouts;
import android.graphics.Color;
import android.graphics.Typeface;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Html;
import android.view.Gravity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.TextView;
public class LayoutExample extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.android_ui);
final EditText email = findViewById(R.id.email);
final TextView text = findViewById(R.id.text);
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String emailtext = email.getText().toString(); //getting text of edittext
text.setText(emailtext); //setting text from edit text to textview
}
});
}
}
Output of the above code example before button click.
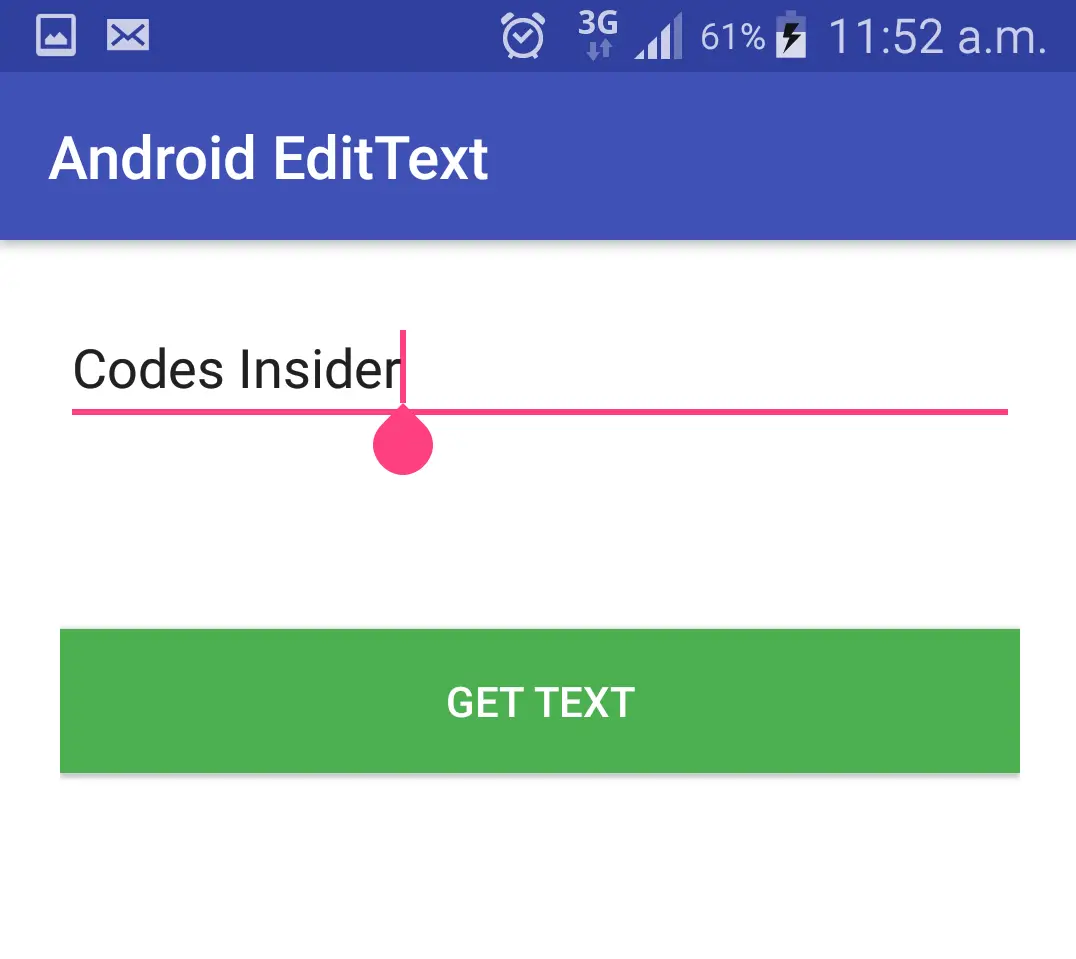
Output after button click.
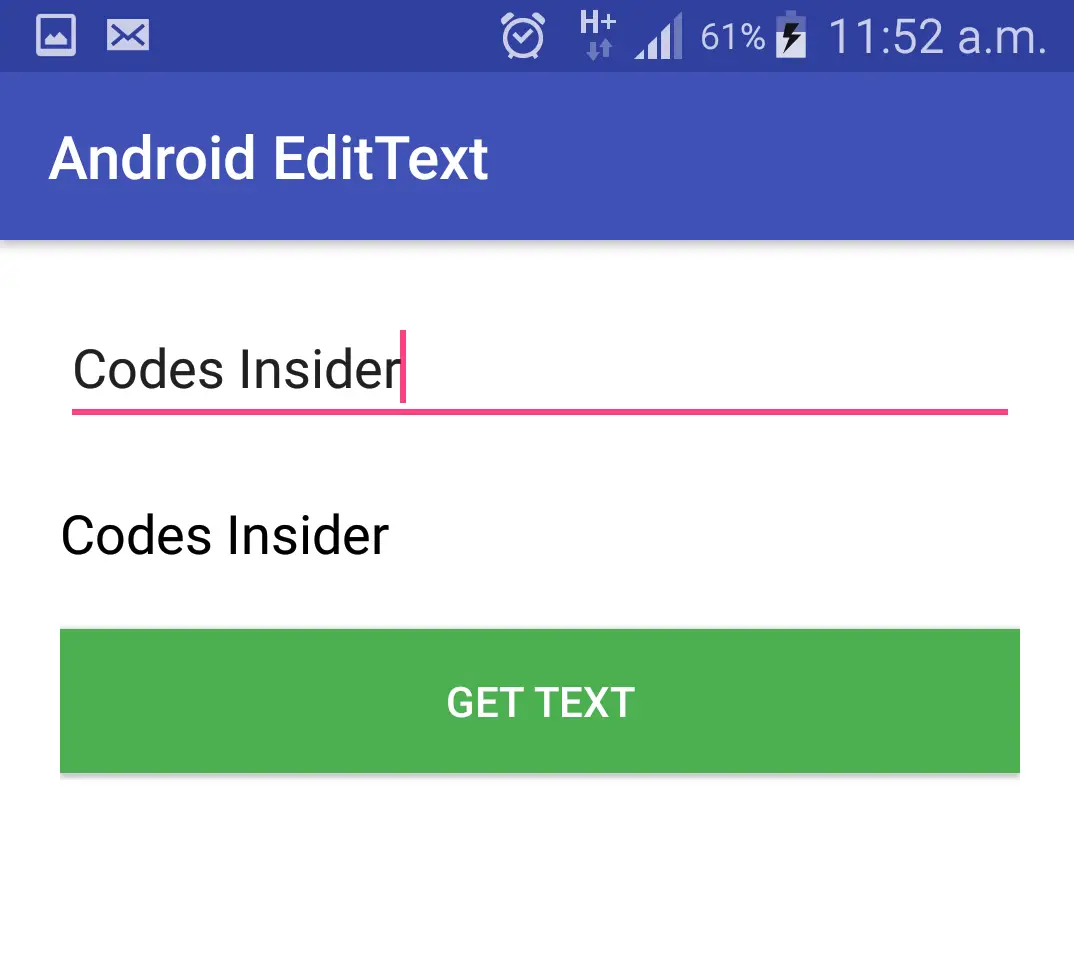
This comes to an end of android EditText example tutorial with all the attributes explained in detail with examples.We will discuss about other UI controls in next posts.
Do like and share if you find this post helpful.Thank you!!

Leave a Reply