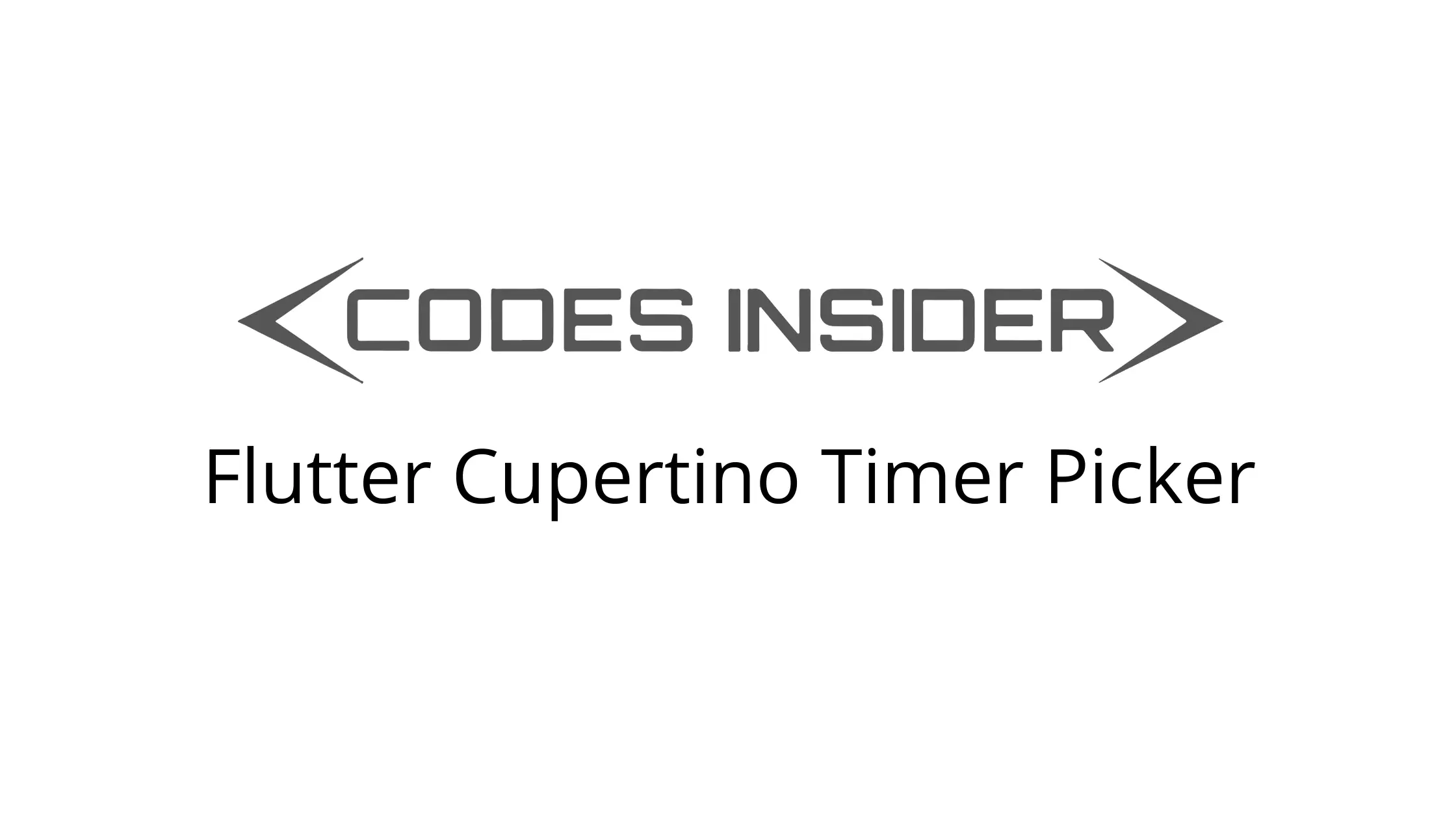
Flutter Cupertino Timer Picker
Cupertino timer picker in flutter is an ios style countdown timer picker. Using the CupertinoTimerPicker widget we can add an ios style countdown timer picker to our application. A timer picker lets us set the time for a countdown timer. We can also use it as a time picker for picking time instead of using it as a picker for a countdown timer. Generally, we will use a countdown timer to display the time left in an online examination or before launching an event, etc. It will display the countdown duration in hours, minutes, and seconds. The maximum duration we can set is 23 hours 59 minutes & 59 seconds.
Don’t know what Cupertino is? It is nothing but a set of flutter widgets that follow the ios design pattern. These widgets are designed to implement ios features in flutter apps built for the ios platform.
In this tutorial, we will learn how to use cupertino timer picker in flutter with example. We will also learn how to customize the style of CupertinoTimerPicker widget using different properties.
Also read: Flutter Cupertino DatePicker.
How To Create Cupertino Timer Picker In flutter ?
To create a cupertino timer picker in fluttter we have to use CupertinoTimerPicker class. Calling the constructor and providing the required properties will do the work. It has one required property onTimerDurationChanged. It takes a callback function as a value.
We don’t have to display a timer picker continuously like other widgets. We have to display it when an event triggers. For example, on click of a button, or any other widget. Generally, we will display a timer picker using showCupertinoModalPopup or showModalBottomSheet.
Flutter CupertinoTimerPicker constructor:
CupertinoTimerPicker(
{Key? key,
CupertinoTimerPickerMode mode,
Duration initialTimerDuration,
int minuteInterval,
int secondInterval,
AlignmentGeometry alignment,
Color? backgroundColor,
required ValueChanged<Duration> onTimerDurationChanged}
)
Flutter Cupertino Timer Picker Properties
The proeprties of a cupertino timer picker are:
- mode
- initialTimerDuration
- minuteInterval
- secondInterval
- alignment
- backgroundColor
- onTimerDurationChanged
mode
We will use this property to change the mode of the timer picker. It takes the CupertinotimerPickerMode constant as value. It has three constants hm, ms, and hms. By default, it is hms (hours minutes seconds).
hms (hours minutes seconds)
CupertinoTimerPicker(
mode: CupertinoTimerPickerMode.hms,
onTimerDurationChanged: (value) {
},
Output:
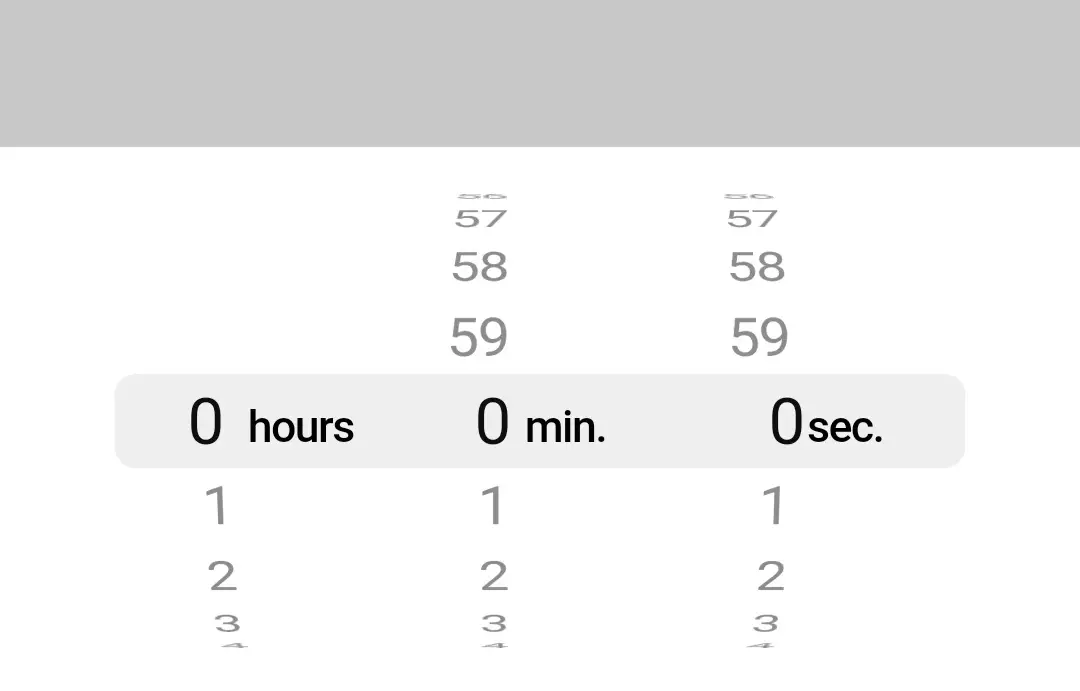
hm (hours minutes)
CupertinoTimerPicker(
mode: CupertinoTimerPickerMode.hm,
onTimerDurationChanged: (value) {
},
Output:
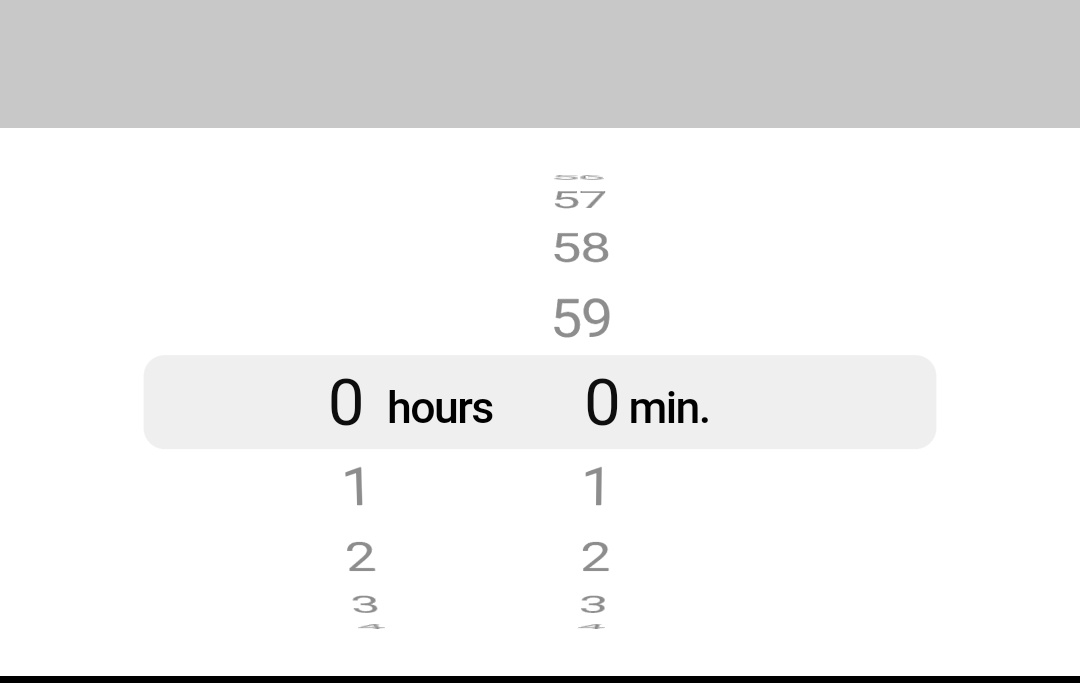
ms (minutes seconds)
CupertinoTimerPicker(
mode: CupertinoTimerPickerMode.ms,
onTimerDurationChanged: (value) {
},
)
Output:
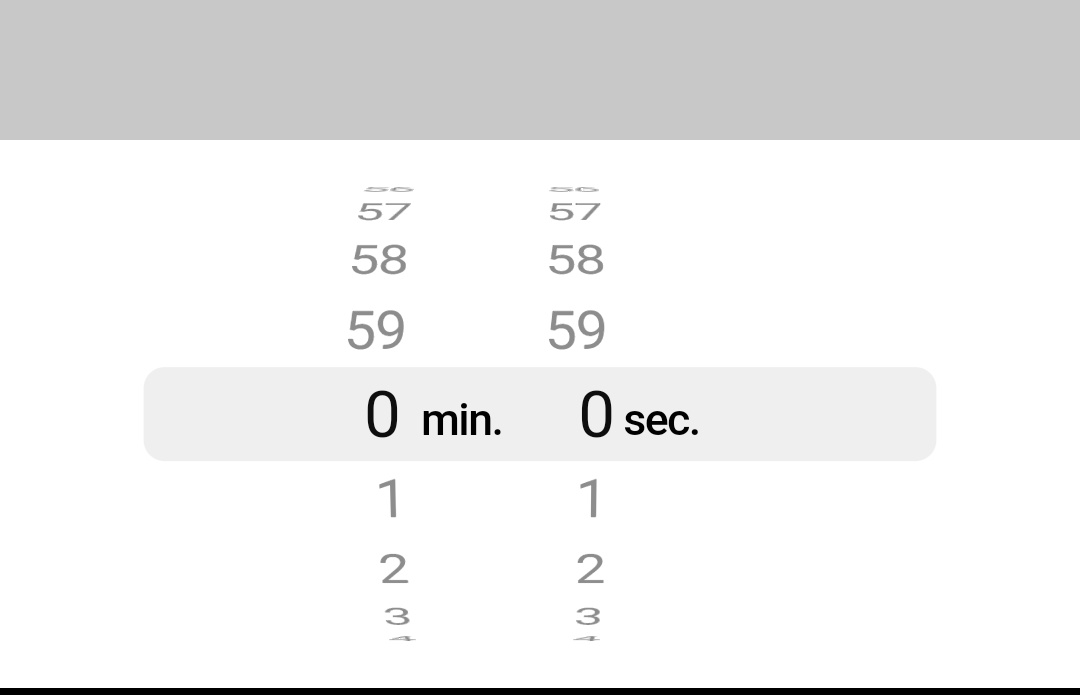
initialTimerDuration
We will use this property to change the initial time that appears on the timer. It takes duration as value.
CupertinoTimerPicker(
initialTimerDuration: Duration(hours: 1, minutes:1, seconds: 1),
onTimerDurationChanged: (value) {
},
)
Output:
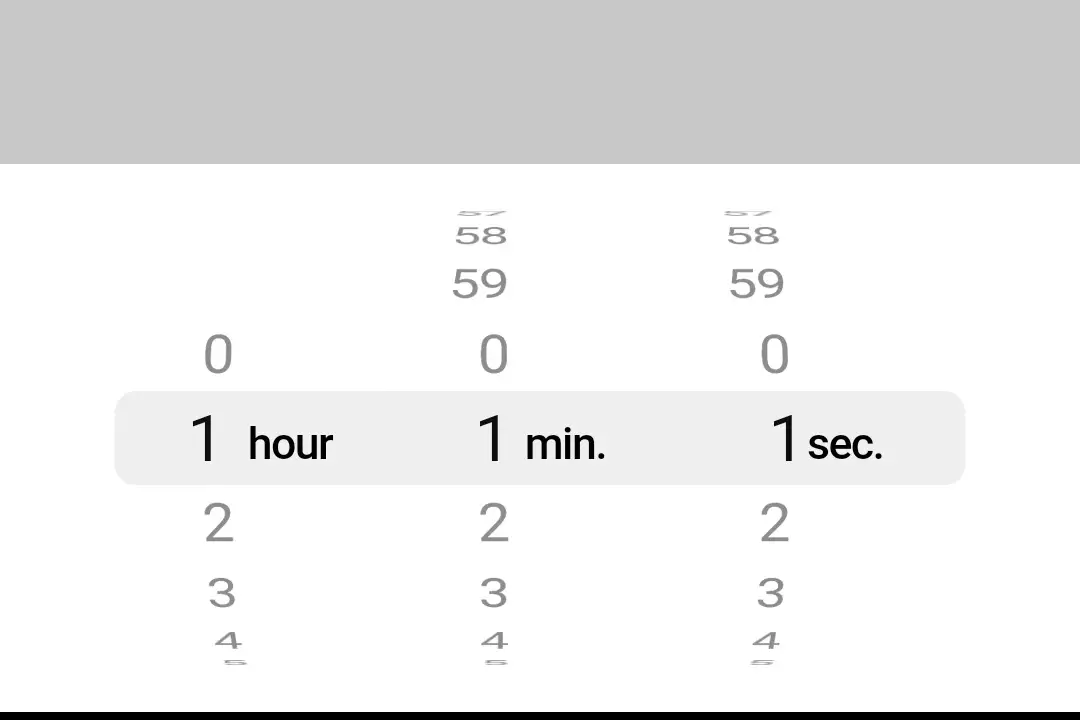
minuteInterval
To change the interval between the minutes of the timer picker we will use this property. It takes double as value. By default, it is 1.
CupertinoTimerPicker(
minuteInterval: 2,
onTimerDurationChanged: (value) {
},
)
Output:
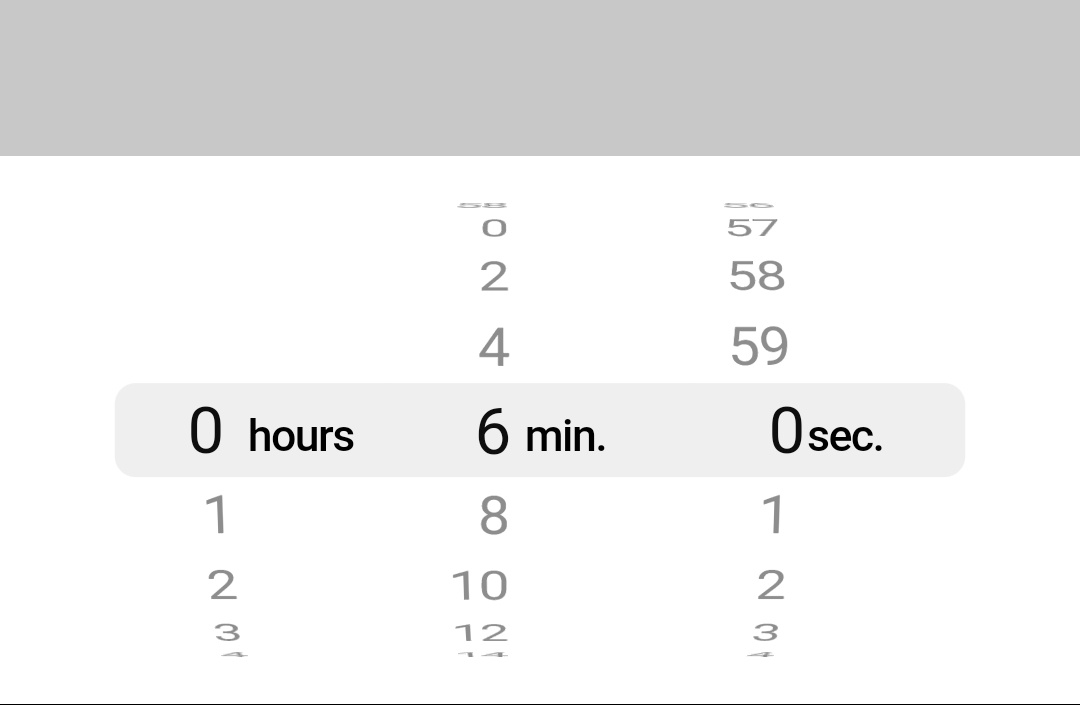
secondInterval
To change the interval of the seconds of the timer picker we will use this property. It also takes double as a value which is 1 by default.
CupertinoTimerPicker(
minuteInterval: 2,
secondInterval: 3,
onTimerDurationChanged: (value) {
},
)
Output:
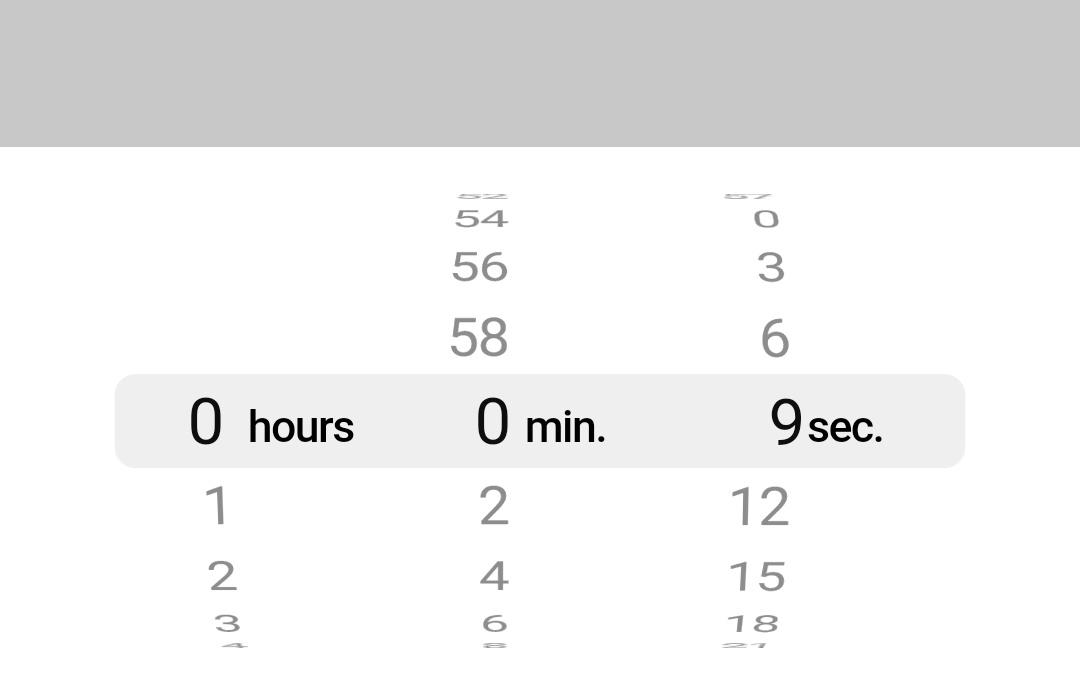
alignment
Use this property to align the picker if not used with showCupertinoModalPopup or showModalBottomSheet. It takes Alignment constant as value.
CupertinoTimerPicker(
minuteInterval: 2,
secondInterval: 3,
alignment: Alignment.topCenter,
onTimerDurationChanged: (value) {
},
)
backgroundColor
To change the background color of the picker we will use this property. It takes CupertinoColors or Colors class constant as value.
CupertinoTimerPicker(
backgroundColor: CupertinoColors.activeGreen,
onTimerDurationChanged: (value) {
},
)
Output:
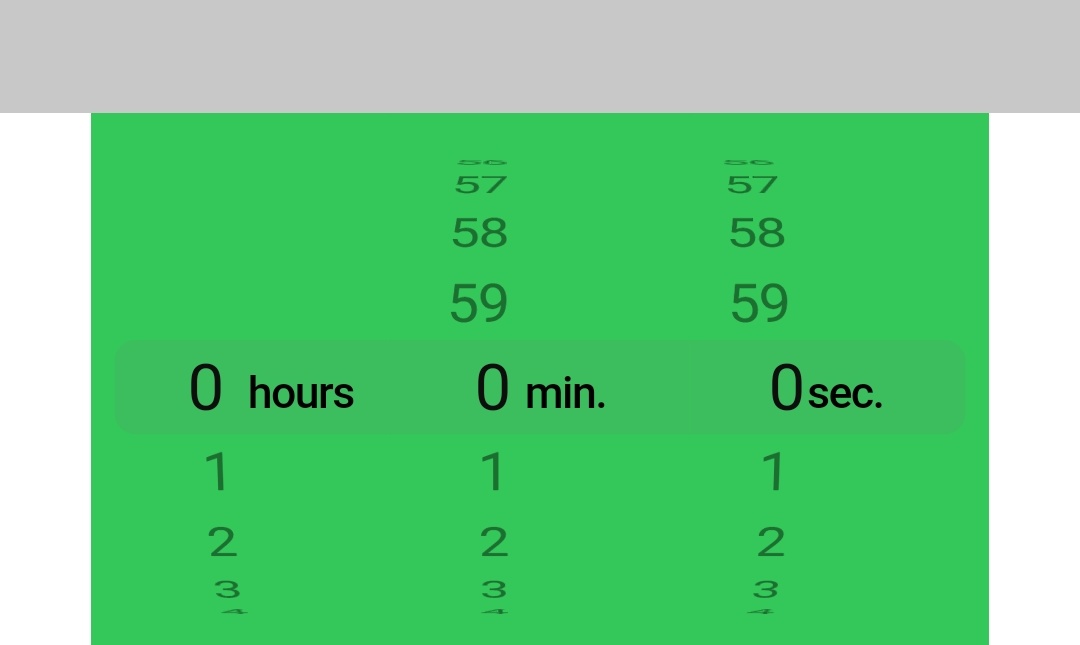
onTimerDurationChanged
It takes a callback function as value. This function will invoke every time the user changes the time in the picker. Inside this function, we will get the updated time. We will update the UI with this value by calling setState() function.
CupertinoTimerPicker(
backgroundColor: CupertinoColors.activeGreen,
onTimerDurationChanged: (value) {
selectedValue = value;
setState(() {
});
},
)
Flutter Cupertino Timer Picker Example
Let’s create an example where we will implement a cupertino timer picker using CupertinoTimerPicker widget in flutter. We will display a button which will display the picker. when the user picks a time we will display the time in a Text widget.
First, declare a variable for holding the time selected by the user.
Duration selectedValue = Duration(hours:0, minutes: 0, seconds: 0);
Now let’s implement the code to display a cupertino button and a Text widget. The button will trigger the cupertino timer picker and the text widget will display the selected time.
child:Container(
margin: EdgeInsets.all(40),
width: double.infinity,
height: MediaQuery.of(context).size.height*0.50,
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
CupertinoButton(
child: Text("Show Picker"),
onPressed: (){
showTimerPicker();
},
),
Material(
child: Text(
"$selectedValue",
style: TextStyle(
fontSize: 18
),
),
),
]
)
),
Now implement the code for displaying the picker inside a function showTimerPicker().
void showTimerPicker() {
showCupertinoModalPopup(
context: context,
builder: (BuildContext builder) {
return Container(
height: MediaQuery
.of(context)
.copyWith()
.size
.height * 0.25,
width: double.infinity,
color: Colors.white,
child: CupertinoTimerPicker(
initialTimerDuration: Duration(hours:0, minutes: 0, seconds: 0);
onTimerDurationChanged: (value) {
selectedValue = value;
setState(() {
});
},
)
);
}
);
}
Call the above function inside the onPressed callback of the cupertino button implemented in the beginning.
CupertinoButton(
child: Text("Show DatePicker"),
onPressed: (){
showTimerPicker();
},
),
Complete Code
Let’s complete our cupertino timer picker example in flutter by combining all the above code snippets together. Create a new flutter project and replace the code in main.dart file with the code below.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home:MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
Duration selectedValue;
@override
Widget build(BuildContext context) {
return new CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text("Flutter Cupertino Tabbar"),
),
child:Container(
margin: EdgeInsets.all(40),
width: double.infinity,
height: MediaQuery.of(context).size.height*0.50,
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
CupertinoButton(
child: Text("Show Picker"),
onPressed: (){
showTimerPicker();
},
),
Material(
child: Text(
"$selectedValue",
style: TextStyle(
fontSize: 18
),
),
),
]
)
),
);
}
void showTimerPicker() {
showCupertinoModalPopup(
context: context,
builder: (BuildContext builder) {
return Container(
height: MediaQuery
.of(context)
.copyWith()
.size
.height * 0.25,
width: double.infinity,
color: Colors.white,
child: CupertinoTimerPicker(
initialTimerDuration: Duration(hours:0, minutes: 0, seconds: 0);
onTimerDurationChanged: (value) {
selectedValue = value;
setState(() {
});
},
)
);
}
);
}
}
Output:
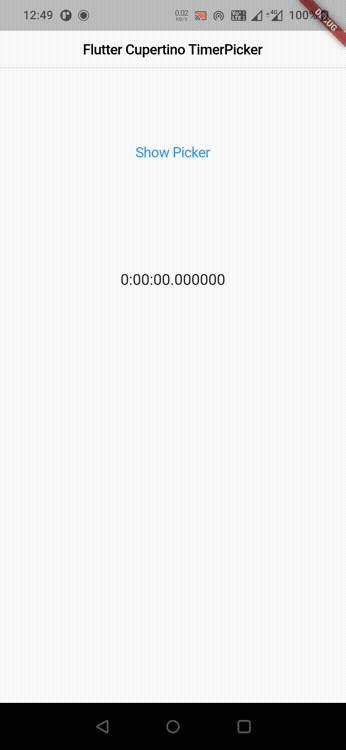
That’s all about the tutorial on how to create & use cupertino Timer Picker in flutter. We have also seen an example where we have used the CupertinoTimerPicker widget and customized its style. Let’s catch up with some other widgets in the next post. Have a great day !!
Do like & share my Facebook page. Subscribe to the newsletter if you find this post helpful. Thank you !!
Reference: Flutter Official Documentation.

Leave a Reply