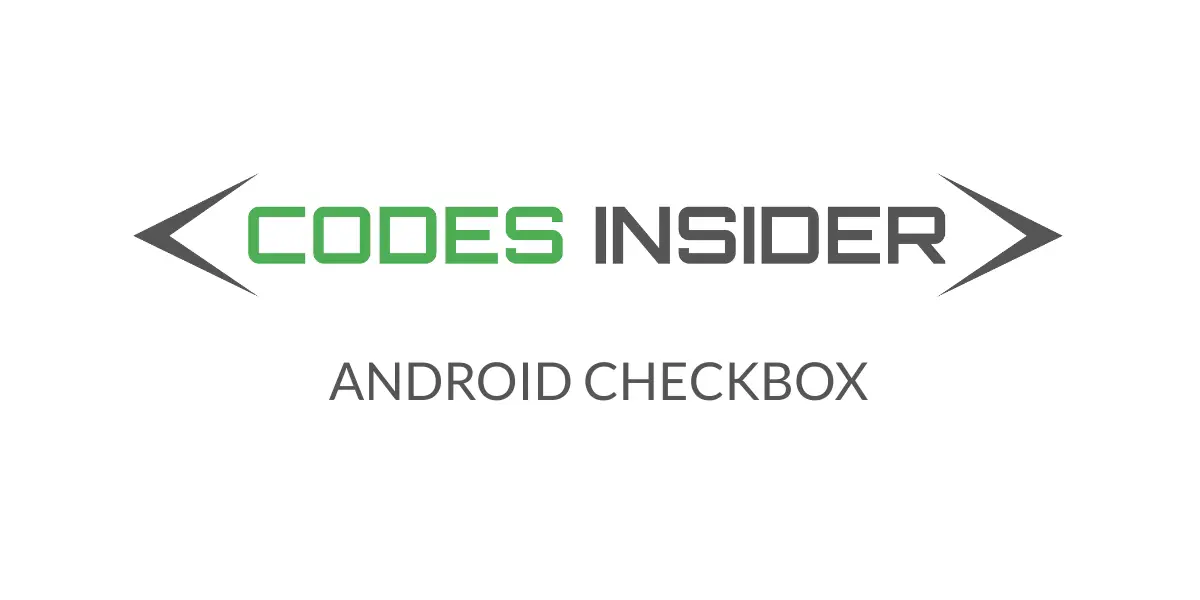
Android CheckBox is used to display group of options to user so that the user can select desired options from the given group of items.In this example tutorial you will learn how to implement a checkbox and different attributes with example.
Android CheckBox
Android CheckBox is a ui control which is nothing but a two state button that can be checked and unchecked based on the user preference.The checked state is nothing but ON and Unchecked is OFF.It allows the user to toggle between checked and unchecked states.
Check this android UI controls tutorial to know more about UI controls.
Android checkbox code in XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_horizontal"
tools:context=".MainActivity">
<androidx.appcompat.widget.AppCompatCheckBox
android:layout_width="wrap_content"
android:layout_height="40dp"
android:text="Checkbox"
/>
</LinearLayout>
Android checkbox code in JAVA
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.CheckBox;
import android.widget.LinearLayout;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
CheckBox box = new CheckBox(this);
LinearLayout main = new LinearLayout(this);
main.addView(box);
}
}
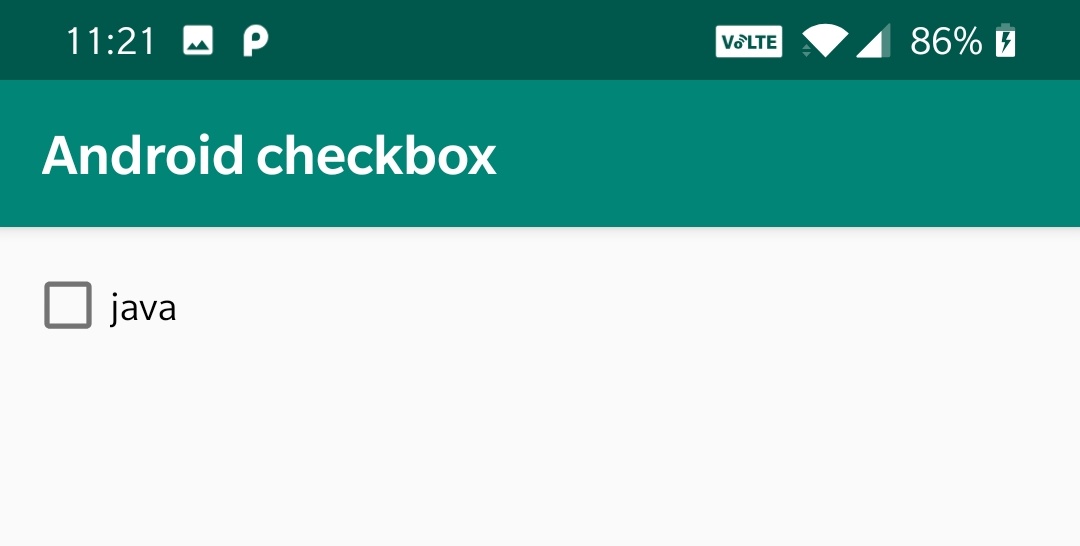
Android CheckBox Attributes
Lest see some important attributes of a checkbox that help in configuring and styling a checkbox.
checked : It is used to set the state of a checkbox.The value will be true for checked state and false for unchecked state of a checkbox.By default the checkbox’s state is unchecked which is false.
Code snippet for setting checked state in XML
<androidx.appcompat.widget.AppCompatCheckBox
android:layout_width="wrap_content"
android:layout_height="40dp"
android:text="Checkbox"
android:checked="true"
/>
Code snippet for setting checked state in JAVA
CheckBox box = findViewById(R.id.box);
box.setChecked(true);
gravity : The gravity attribute is used to align the checkbox text to left, right, center, top etc
Setting gravity in XML
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/box"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="Checkbox"
android:checked="true"
android:gravity="end|center_vertical"
/>
Setting gravity in JAVA
CheckBox box = findViewById(R.id.box);
box.setChecked(true);
box.setGravity(Gravity.END);
layoutGravity : Layout gravity is used to align the entire checkbox and its text(entire checkbox layout) to left, right, top, bottom etc.
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/box"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="Checkbox"
android:checked="true"
android:gravity="end|center_vertical"
android:layout_gravity="start"
/>
text : Text attribute is used to set text for a checkbox.
Setting text in XML
<androidx.appcompat.widget.AppCompatCheckBox
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="Checkbox"
android:checked="true"
/>
Setting text in JAVA
CheckBox box = findViewById(R.id.box);
box.setChecked(true);
box.setGravity(Gravity.END);
box.setText("checkbox");
textColor : TextColor attribute is used to set text color of checkbox.
Setting textColor in XML
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/box"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="Checkbox"
android:checked="true"
android:textColor="@color/colorAccent"
/>
Setting textColor in JAVA
CheckBox box = findViewById(R.id.box);
box.setText("checkbox");
box.setTextColor(Color.CYAN);
textSize : TextSize attribute is used to set the text size of the checkbox.
Setting textSize in XML
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/box"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="Checkbox"
android:textSize="16sp"
/>
Setting textSize in JAVA
CheckBox box = findViewById(R.id.box);
box.setText("checkbox");
box.setTextSize(18);
textStyle : TextStyle attribute is used to style the text of the checkbox.The value for text style can be normal, bold and italic.By default the value is normal.
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/box"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="Checkbox"
android:textStyle="bold"
/>
background : Background attribute is used to set background for checkbox.The background can be a color or a resource file.
Setting background in XML
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/box"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="Checkbox"
android:background="@color/colorAccent"
/>
Setting Background in JAVA
CheckBox box = findViewById(R.id.box);
box.setText("checkbox");
box.setBackgroundColor(Color.RED);
That’s all about the most commonly used attributes of Checkbox.To know the attributes that are common for all the elements refer the layout attributes section of this post about android layouts.
How to Find CheckBox is Checked or Unchecked
We can find whether a checkbox is checked or not in two ways
1. By using ischecked() method.
2. By using OnCheckedchangedListener method.
isChecked() : This method is used to find if a checkbox is checked or not.This method returns a boolean value which is true/false.It returns true if the checkbox is checked and false if unchecked.
CheckBox box = findViewById(R.id.box);
boolean checked = box.isChecked();
OnCheckedChangeListener : This OnCheckedChangeListener implements onCheckedChanged() method that has two parameters in which one parameter is object of compoundButton class and the other is a boolean value which is checked state of the checkbox.This method listens whenever the checkbox is checked or unchecked
CheckBox box = findViewById(R.id.box);
box.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
if(b)
{
Toast.makeText(MainActivity.this, "Checkbox is checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(MainActivity.this, "Checkbox is Unchecked", Toast.LENGTH_SHORT).show();
}
}
});
Android CheckBox Example
Lets see an example where we display 5 checkboxes with different properties like textcolor, textsize, background, textstyle and we will display a message whenever a checkbox is checked or unchecked
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_horizontal"
android:padding="10dp"
android:id="@+id/main"
tools:context=".MainActivity">
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/java"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="java"
/>
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/android"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="android"
android:textColor="@color/colorAccent"
/>
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/php"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="php"
android:textSize="20sp"
/>
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/angular"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="angular"
android:textStyle="bold"
/>
<androidx.appcompat.widget.AppCompatCheckBox
android:id="@+id/node"
android:layout_width="match_parent"
android:layout_height="40dp"
android:text="node"
android:background="#eee"
/>
</LinearLayout>
MainActivity.java
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Color;
import android.os.Bundle;
import android.view.Gravity;
import android.view.View;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.LinearLayout;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
CheckBox java, android, php, angular, node;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
java = findViewById(R.id.java);
android = findViewById(R.id.android);
php = findViewById(R.id.php);
angular = findViewById(R.id.angular);
node = findViewById(R.id.node);
java.setOnClickListener(this);
android.setOnClickListener(this);
php.setOnClickListener(this);
angular.setOnClickListener(this);
node.setOnClickListener(this);
}
@Override
public void onClick(View view) {
switch(view.getId())
{
case R.id.java:
if(java.isChecked()) {
Toast.makeText(this, "Java is checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(this, "Java is unchecked", Toast.LENGTH_SHORT).show();
}
break;
case R.id.android:
if(android.isChecked()) {
Toast.makeText(this, "Android is checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(this, "Android is unchecked", Toast.LENGTH_SHORT).show();
}
break;
case R.id.php:
if(php.isChecked()) {
Toast.makeText(this, "Php is checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(this, "Php is unchecked", Toast.LENGTH_SHORT).show();
}
break;
case R.id.angular:
if(angular.isChecked()) {
Toast.makeText(this, "Angular is checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(this, "Angular is unchecked", Toast.LENGTH_SHORT).show();
}
break;
case R.id.node:
if(node.isChecked()) {
Toast.makeText(this, "Node is checked", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(this, "Node is unchecked", Toast.LENGTH_SHORT).show();
}
break;
}
}
}
Output after checking
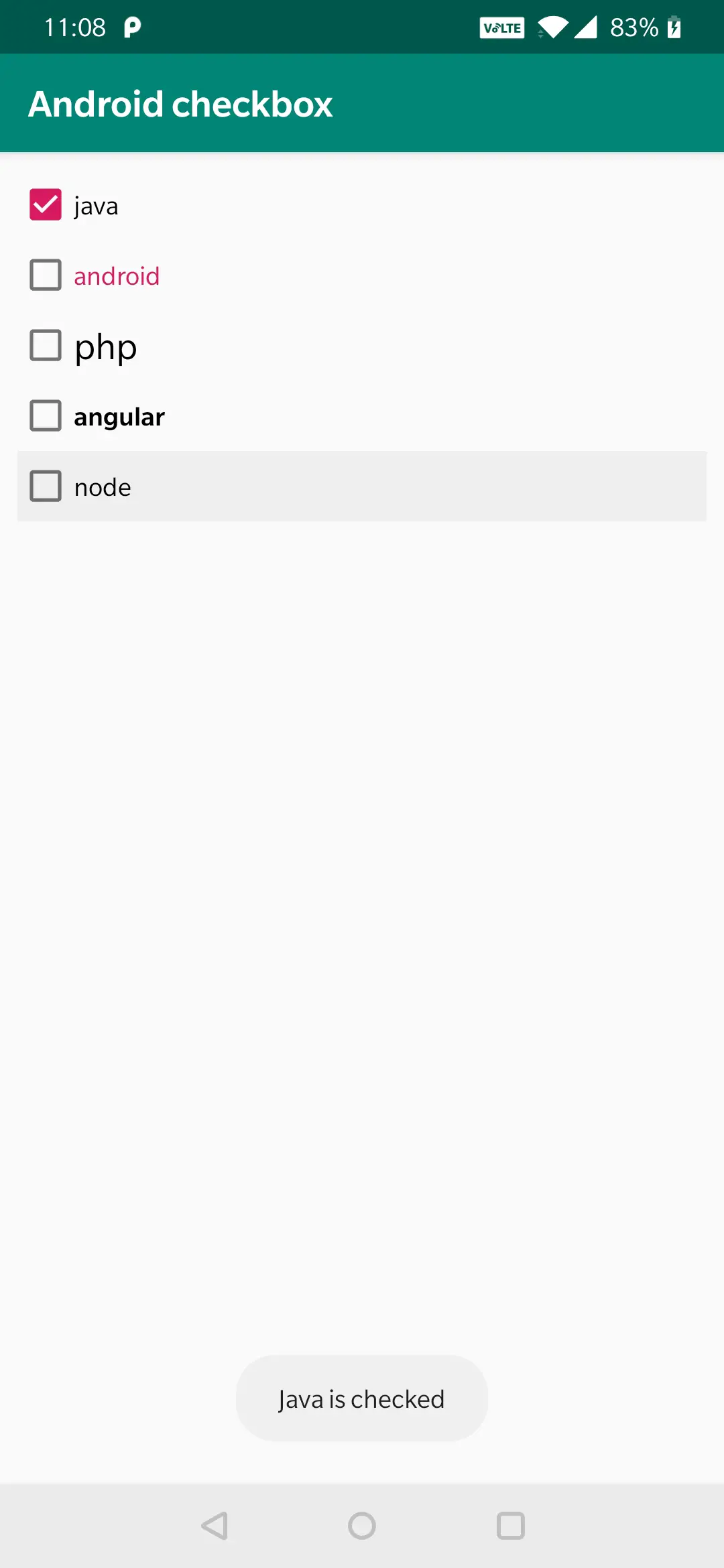
Output after unchecking
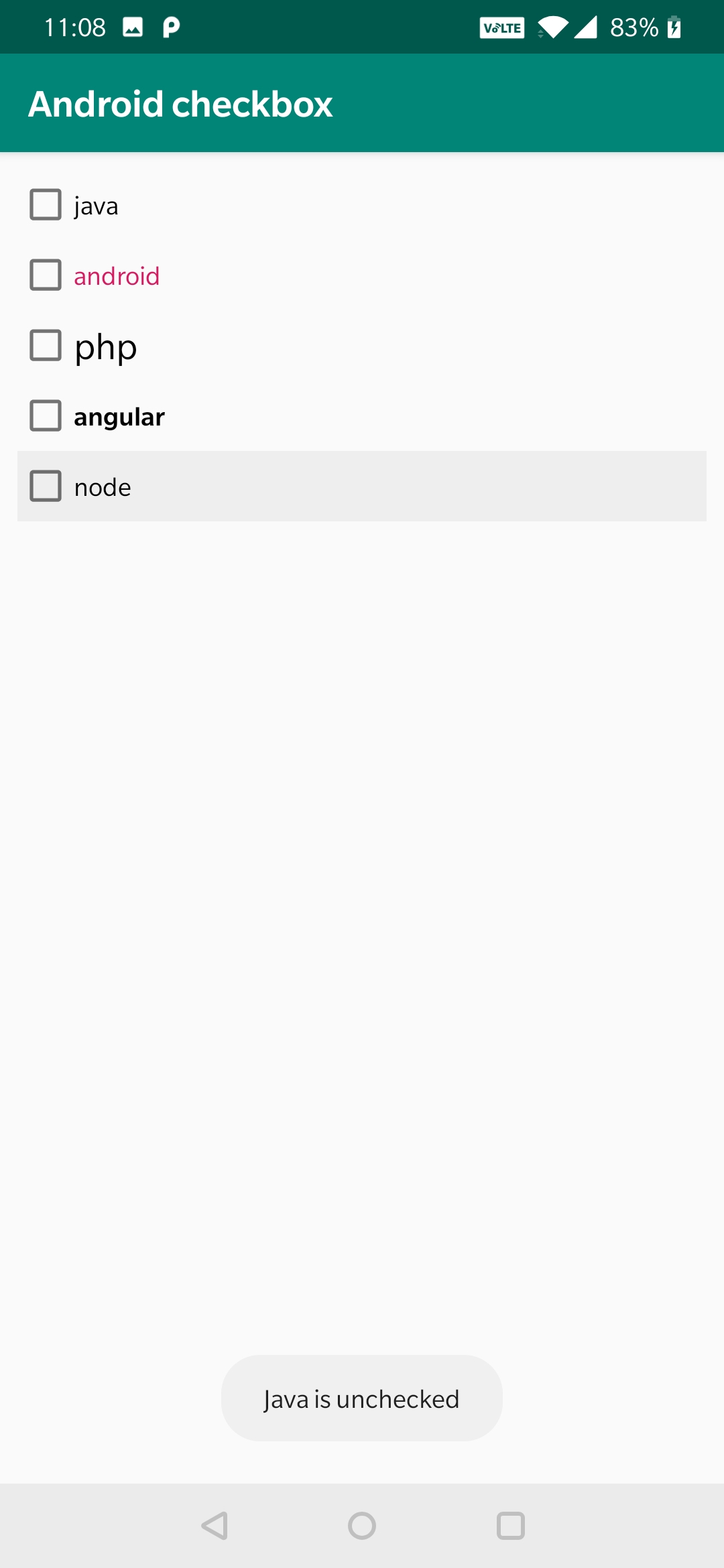
That’s all about android checkbox.In this example tutorial we have seen how to implement a checkbox and its attributes with example.We will discuss about other ui controls in next posts.
Do like and share if you find this post helpful.Thank you!!
Reference: Android Official Documentation.

Leave a Reply