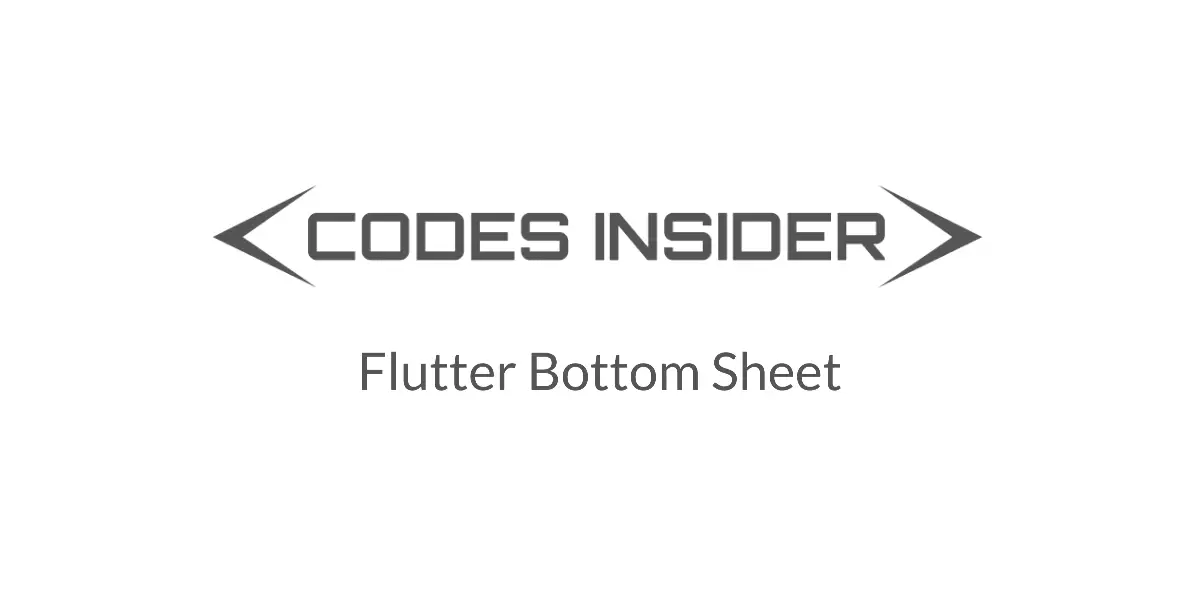
A tutorial on how to use bottom sheet in flutter and types of bottom sheets like modal bottom sheet and persistent bottom sheet.
Flutter Bottom Sheet
Bottom sheet is a material widget in flutter which appears above the contents of the current screen. The bottom sheet comes in handy if we want to display additional details or information in the available screen space. While the bottom sheet appears the user can’t interact with other components outside the bottom sheet. Generally, we will use the bottom sheet in instances like sharing content to other applications or while entering card details, etc.
To use bottom sheet we have to create one by calling its constructor.
Constructor :
BottomSheet(
{Key? key,
AnimationController? animationController,
bool enableDrag,
BottomSheetDragStartHandler? onDragStart,
BottomSheetDragEndHandler? onDragEnd,
Color? backgroundColor,
double? elevation,
ShapeBorder? shape,
Clip? clipBehavior,
required VoidCallback onClosing,
required WidgetBuilder builder}
)
How To Create Bottom Sheet In Flutter ?
To create a bottom sheet there is a widget called BottomSheet provided by flutter. There are two required properties onClosing and builder. The onClosing is a callback function which gets invoked when the bottom sheet is closed. If we want to do something immediately after closing the bottom sheet we can do that inside this callback function. The builder is nothing but a function that should return a widget, usually the UI of the bottom sheet.
Basic implementation of bottom sheet
BottomSheet(
onClosing: () {
// Do what you wanna do when the bottom sheet closes.
},
builder: (context) {
// should return a widget
},
);
How To Display Bottom Sheet In Flutter ?
To display a general bottom sheet we have to use bottomSheet property of scaffold widget.
return Scaffold(
appBar: AppBar(
title: Text("Flutter Bottom Sheet"),
),
body:Container(),
bottomSheet: BottomSheet(),
);
If we provide BottomSheet widget to that property like in the above code it will display the bottom sheet continuously and we cannot dismiss it. So let’s create a boolean variable _show which holds the state ( displaying / closed ) of bottom sheet. Initially this value will be false.
bool _show = false;
Now we will create a function _showBottomSheet() whose return type is a widget. Inside the function we will have a condition which will return BottomSheet if the _show is true and null when it is false. We will update the _show variable when the user clicks the show Bottom sheet button and also when the user clicks the close bottom sheet button inside the bottom sheet.
_showBottomSheet()
Widget _showBottomSheet()
{
if(_show)
{
return BottomSheet(
onClosing: () {
},
builder: (context) {
return Container(
height: 200,
width: double.infinity,
color: Colors.grey.shade200,
alignment: Alignment.center,
child: ElevatedButton(
child: Text("Close Bottom Sheet"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.green,
),
onPressed: () {
_show = false;
setState(() {
});
},
),
);
},
);
}
else{
return null;
}
}
Flutter Bottom Sheet Example Complete Code
Below is an example code where we display a bottom sheet. Let’s create a basic UI with a button which displays the bottom sheet. Inside onPressed callback we will call _showBottomSheet function that shows the bottom sheet.
import 'dart:io';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'widgets/add_entry_dialog.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.green,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
bool _show = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter Bottom Sheet"),
),
body:Center(
child:Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () {
_show = true;
setState(() {
});
},
child: Text("Show Bottom Sheet"),
)
],
)
),
bottomSheet: _showBottomSheet()
);
}
Widget _showBottomSheet()
{
if(_show)
{
return BottomSheet(
onClosing: () {
},
builder: (context) {
return Container(
height: 200,
width: double.infinity,
color: Colors.grey.shade200,
alignment: Alignment.center,
child: ElevatedButton(
child: Text("Close Bottom Sheet"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.green,
),
onPressed: () {
_show = false;
setState(() {
});
},
),
);
},
);
}
else{
return null;
}
}
}
Output :
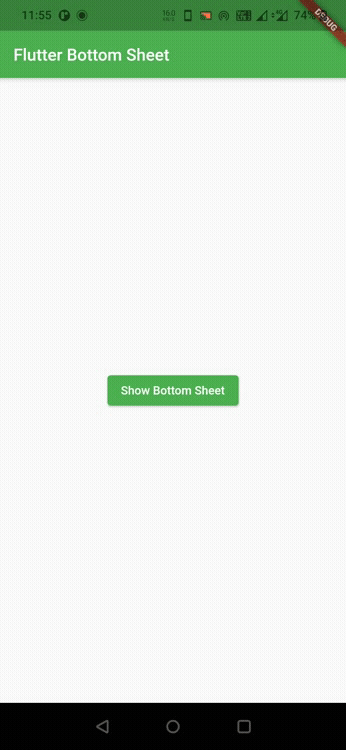
Types Of Bottom Sheets
There are two types of bottom sheets in flutter other than regular bottom sheet widget.
- Modal Bottom Sheet
- Persistent Bottom Sheet
Flutter Modal Bottom Sheet
Modal Bottom sheet is a material widget in flutter which can be used as an alternative to widgets like dialog. It provides additional space for other widgets by showing another layer over the current screen. To be able to interact with UI below the bottom sheet, we have to dismiss it.
To create a modal bottom sheet in flutter we have to use showModalBottomSheet() function provided by flutter. Let’s have a look of the function below.
showModalBottomSheet function :
showModalBottomSheet<T>(
{required BuildContext context,
required WidgetBuilder builder,
Color? backgroundColor,
double? elevation,
ShapeBorder? shape,
Clip? clipBehavior,
Color? barrierColor,
bool isScrollControlled = false,
bool useRootNavigator = false,
bool isDismissible = true,
bool enableDrag = true,
RouteSettings? routeSettings,
AnimationController? transitionAnimationController}
)
Creating And Displaying Modal Bottom sheet
To create and display modal bottom sheet, flutter provides a function showModalBottomsheet(). There are two required properties in this function context and builder. The builder should return a widget which will be the UI of the bottom sheet.
Basic implementation of ShowModalBottomSheet()
showModalBottomSheet(
context: context,
builder: (context) {
},
);
Flutter Modal Bottom Sheet Complete Code
In the below example we will create and display a modal bottom sheet. The basic UI will have an elevated button which on click will call _showModalBottomSheet function. Inside this function we will call showModalBottomSheet function provided by flutter. This function will display the bottom sheet.
import 'dart:io';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.green,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter Bottom Sheet"),
),
body:Center(
child:Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () {
_showModalBottomSheet();
},
child: Text("Show Bottom Sheet"),
)
],
)
),
);
}
_showModalBottomSheet()
{
showModalBottomSheet(
context: context,
builder: (context) {
return Container(
height: 200,
width: double.infinity,
color: Colors.grey.shade200,
alignment: Alignment.center,
child: ElevatedButton(
child: Text("Close Bottom Sheet"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.green,
),
onPressed: () {
Navigator.of(context).pop();
},
),
);
},
);
}
}
Output :
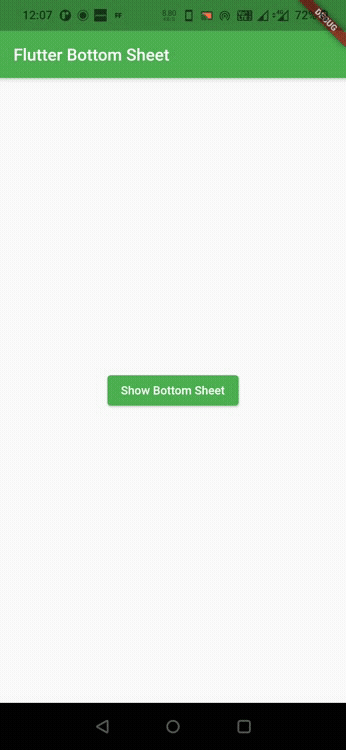
Flutter Persistent Bottom Sheet
Persistent bottom sheet is also a material widget in flutter. It stays on the screen even if the user interacts with other parts of the screen. We can dismiss it by swiping down or by pressing back button.
We can create a persistent bottom sheet in flutter by using showBottomSheet() function provided by flutter. Let’s see the function below.
showBottomSheet function :
showBottomSheet<T>(
{WidgetBuilder builder,
Color? backgroundColor,
double? elevation,
ShapeBorder? shape,
Clip? clipBehavior,
AnimationController? transitionAnimationController}
)
Creating And Displaying Persistent Bottom Sheet
Let’s create and display a persistent bottom sheet using showBottomSheet() function. We will have to create a global key of scaffoldState. Without this key we cannot display a persistent bottom sheet.
final _gKey = new GlobalKey<ScaffoldState>();
Add the above key to scaffold widget’s key property. like below.
return Scaffold(
key: _gKey,
);
Basic implementation of showBottomSheet()
showBottomSheet(
)
Flutter Persistent Bottom Sheet Complete code
Let’s make an example where we create and display a persistent bottom sheet. We will have an elevated button which on click will call displayBottomSheet function. Inside this function we will call showBottomSheet function provided by flutter. This function will display the bottom sheet.
import 'dart:io';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'widgets/add_entry_dialog.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Learning',
theme: ThemeData(
primarySwatch: Colors.green,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
@override
bool _show = false;
final _gKey = new GlobalKey<ScaffoldState>();
Widget build(BuildContext context) {
return Scaffold(
key: _gKey,
appBar: AppBar(
title: Text("Flutter Bottom Sheet"),
),
body:Center(
child:Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () {
displayBottomSheet();
},
child: Text("Show Bottom Sheet"),
)
],
)
),
);
}
void displayBottomSheet()
{
_gKey.currentState.showBottomSheet((context)
{
return Container(
height: 200,
width: double.infinity,
color: Colors.grey.shade200,
alignment: Alignment.center,
child: ElevatedButton(
child: Text("Close Bottom Sheet"),
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.green,
),
onPressed: () {
Navigator.of(context).pop();
},
),
);
}
);
}
}
Output :
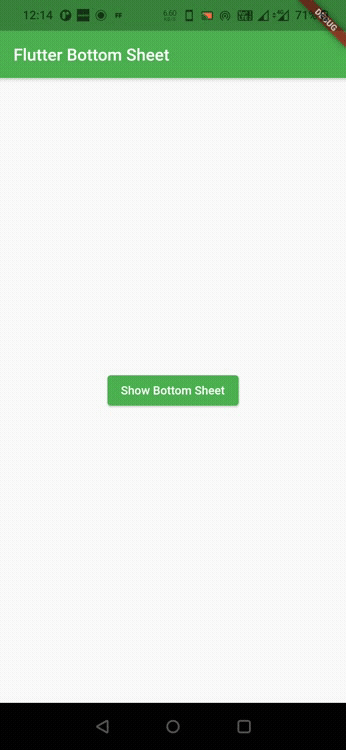
That brings an end to the tutorial on how to use different bottom sheets in flutter with example. I hope you understand how to create and display or show modal bottom sheet and persistent bottom sheet in flutter. Let’s catch up with some other widget in the next post. Have a great day!!
Do share, subscribe and like my facebook page if you find this post helpful. Thank you!!
Reference : Flutter Official Documentation

how to return value from bottomsheet to main page in flutter ???
Hey payel, you can return the value using async & await