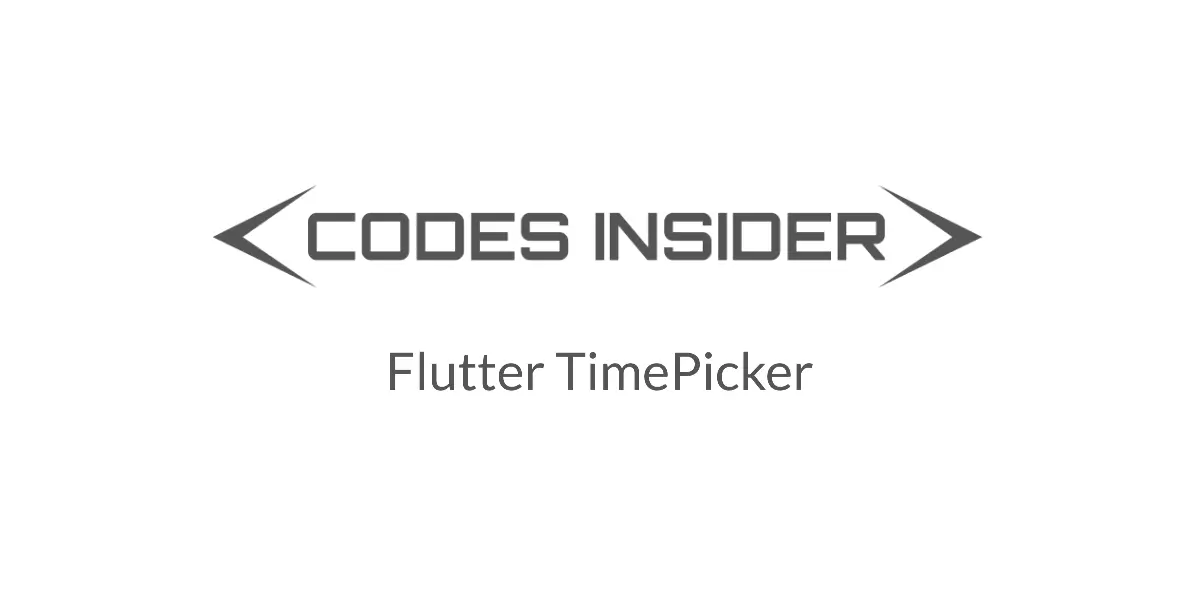
In this tutorial we will learn how to use a TimePicker widget in flutter with example.
Flutter TimePicker widget
TimePicker is a material widget in flutter that lets the user pick a time. Since there is no widget available for creating timepicker we will use showTimePicker() function. It will display a Material Design time picker in a dialog by calling flutter’s inbuilt function. We will use it in instances like scheduling meetings, setting alarms, etc.
To create a TimePicker we use showTimePicker() which is an asynchronous function. When we call the function it will display the dialog to the user to choose the time. It returns the selected time when the user confirms the dialog. If the user cancels the dialog, it will return null.
Constructor:
showTimePicker (
{
required BuildContext context,
required TimeOfDay initialTime,
TransitionBuilder? builder,
bool useRootNavigator: true,
TimePickerEntryMode initialEntryMode: TimePickerEntryMode.dial,
String? cancelText,
String? confirmText,
String? helpText,
RouteSettings? routeSettings
}
)
TimePicker has one required property initialTime without which we cannot use the Time Picker. When the time picker is first displayed, it will show the initialTime selected by default.
Creating TimePicker
To create a Timepicker in flutter we have to initialize a variable selectedTime of type TimeOfDay which will hold the selected time. But initially, we will assign today’s time to it.
TimeOfDay selectedTime = TimeOfDay.now();
Now let’s design the UI where we will have an ElevatedButton widget to display the TimePicker dialog and a text widget to display the selected time.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter TimePicker"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
_selectTime(context);
},
child: Text("Choose Time"),
),
Text("${selectedTime.hour}:${selectedTime.minute}"),
],
),
),
);
}
As the UI is ready now let’s create the method to display TimePicker. We will be using the TimeOfDay variable ‘selectedTime‘ created above for initialDate. We are assigning the user selected time to the variable in setState() to update the UI.
_selectTime(BuildContext context) async {
final TimeOfDay timeOfDay = await showTimePicker(
context: context,
initialTime: selectedTime,
initialEntryMode: TimePickerEntryMode.dial,
);
if(timeOfDay != null && timeOfDay != selectedTime)
{
setState(() {
selectedTime = timeOfDay;
});
}
}
Flutter Timepicker Example Complete Code
Below is the complete code example where we create a timepicker and display it using the showTimePicker function provided by flutter.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main()
{
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter TimePicker',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
TimeOfDay selectedTime = TimeOfDay.now();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter TimePicker"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
_selectTime(context);
},
child: Text("Choose Time"),
),
Text("${selectedTime.hour}:${selectedTime.minute}"),
],
),
),
);
}
_selectTime(BuildContext context) async {
final TimeOfDay timeOfDay = await showTimePicker(
context: context,
initialTime: selectedTime,
initialEntryMode: TimePickerEntryMode.dial,
);
if(timeOfDay != null && timeOfDay != selectedTime)
{
setState(() {
selectedTime = timeOfDay;
});
}
}
}
Output:
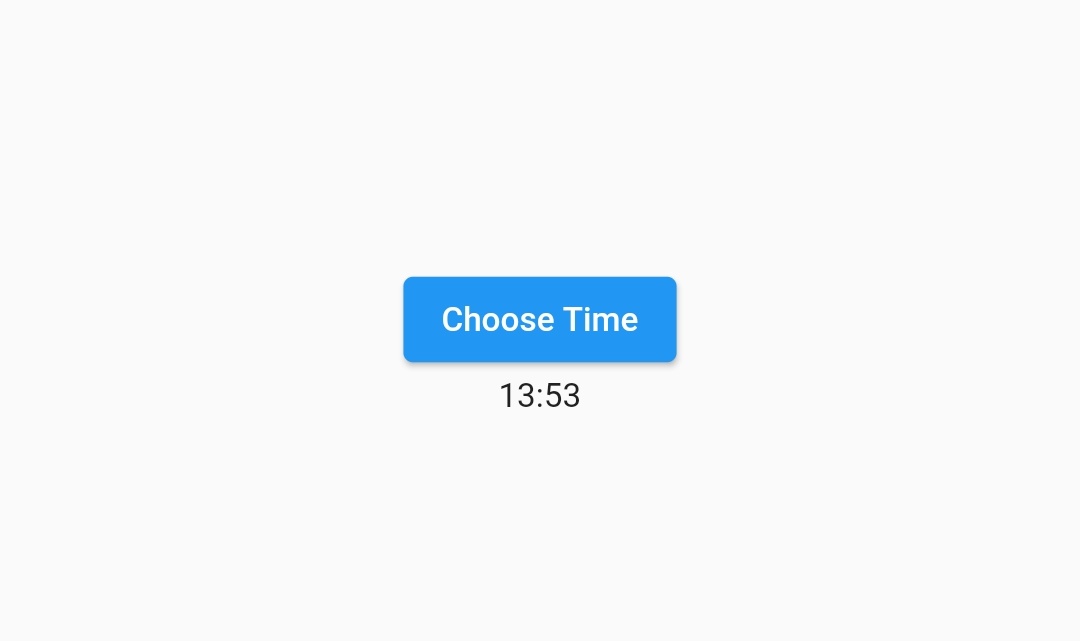
After clicking the button the time picker will be displayed.
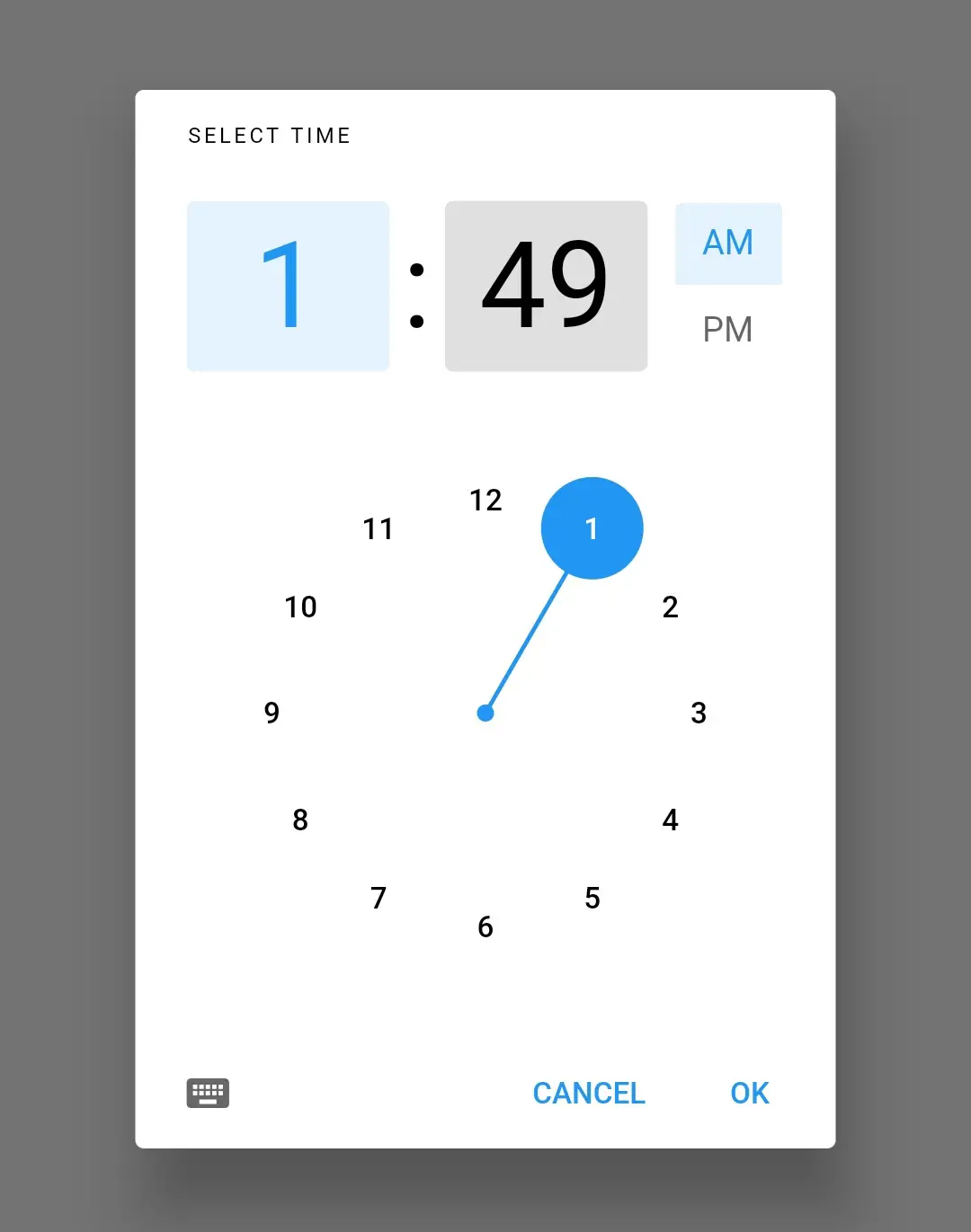
showTimePicker(
context: context,
initialTime: selectedTime,
initialEntryMode: TimePickerEntryMode.dial,
confirmText: "CONFIRM",
cancelText: "NOT NOW",
helpText: "BOOKING TIME"
);
Output:
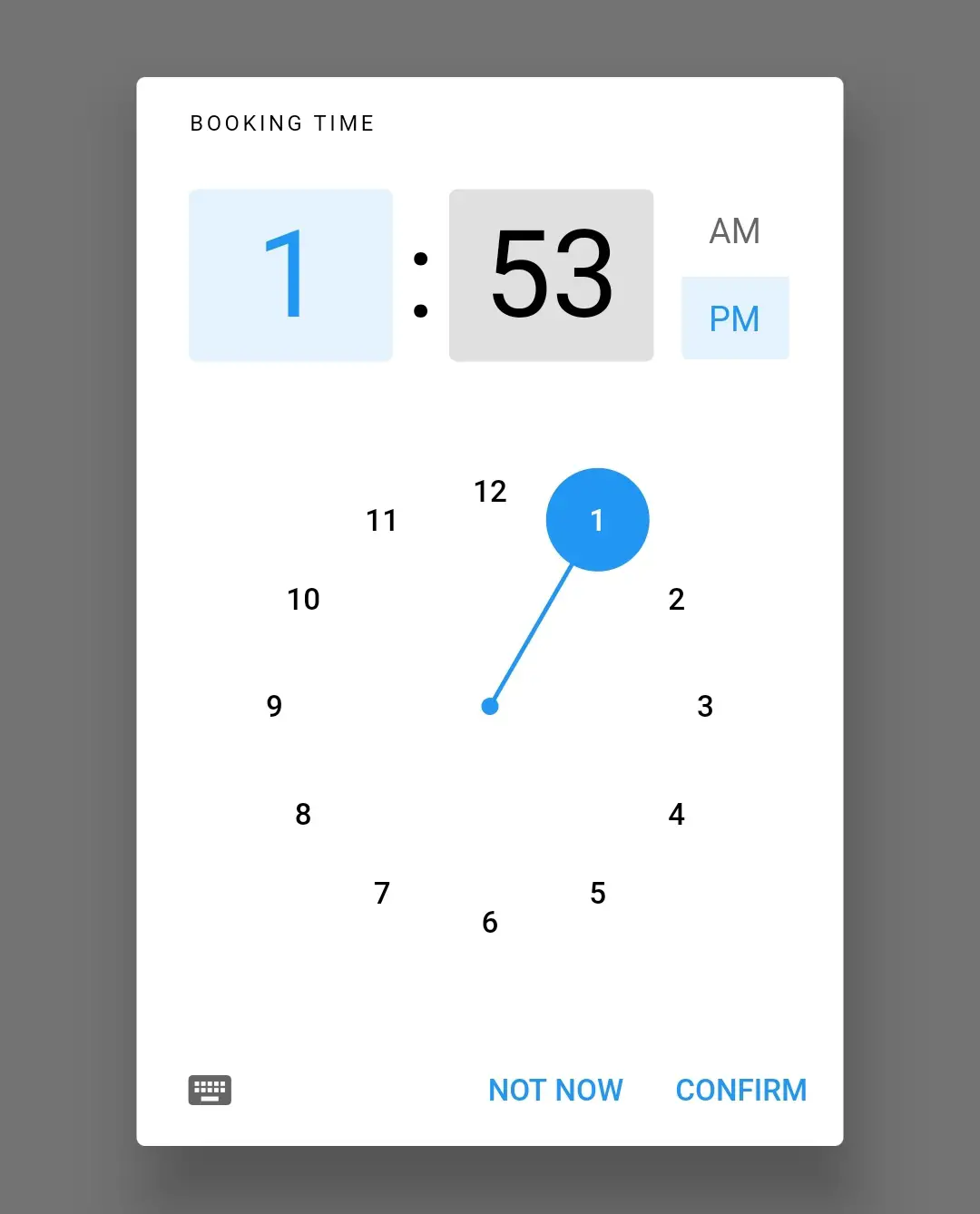
How to show a Text Input instead of dialer in timepicker
In flutter you can choose to display a text input instead of the dialer.
showTimePicker(
context: context,
initialTime: selectedTime,
initialEntryMode: TimePickerEntryMode.input,
confirmText: "CONFIRM",
cancelText: "NOT NOW",
helpText: "BOOKING TIME",
);
Output:
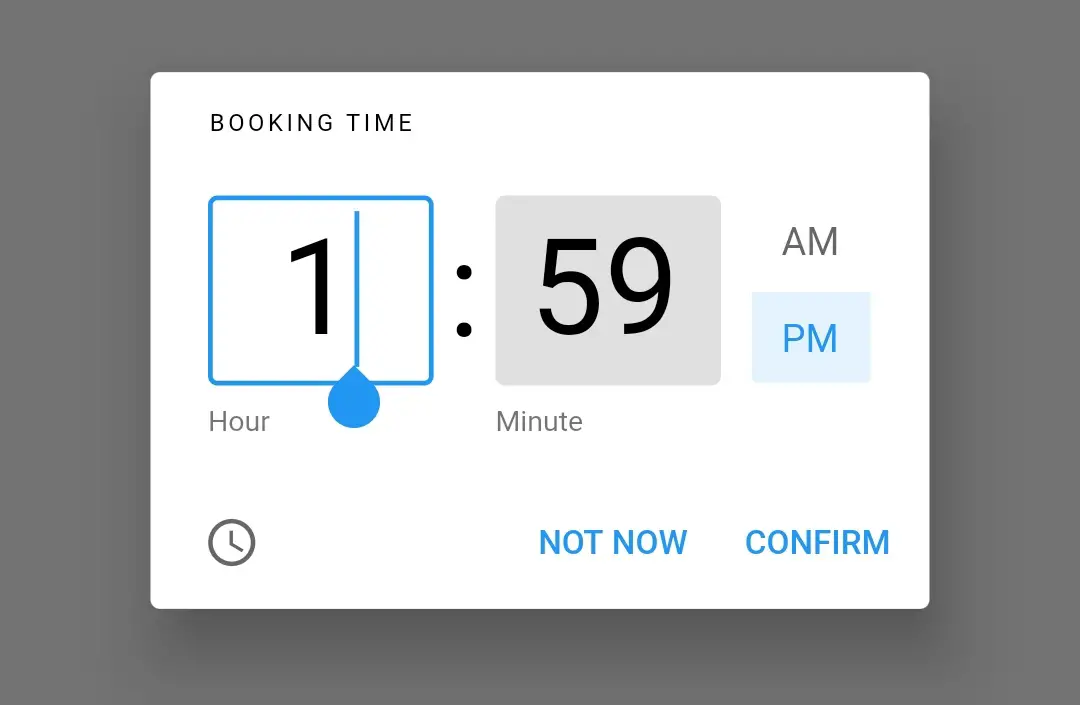
That’s all about how to use TimePicker widget in flutter with example. I hope you understand this tutorial. Let’s catch up with some other widget in the next post. Have a great day!!
Do share, subscribe, and like my Facebook page if you find this post helpful. Thank you!!
Reference: Flutter Official Documentation

Hello , Thank you for ur code , I have a question how can I save the date so even if I scrowl between pages the date won’t change ?
Welcome Naziha Nedri. Simply save the selected date in shared preferences.