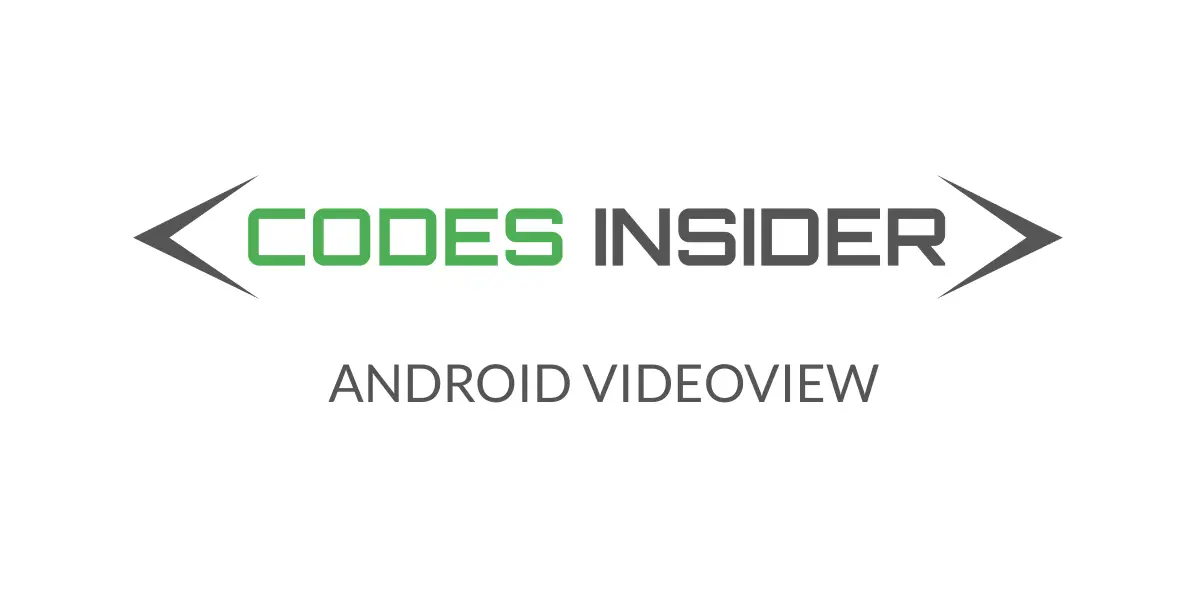
In this android videoview example tutorial you will learn how to implement a videoview in android studio and different methods available in detail.
Android VideoView
Android videoview is a ui control used to display and play video files in android applications.Using videoview we can play 3gp, mp4 (H.263, H.264, H.264 codecs) formats.The source for videoview can be resource files, files in local storage or web Urls.
Note: Videoview does’nt retain its full state while going into background.One have to take care of saving and restoring the playback position and play state.
Android videoview code in XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<VideoView
android:id="@+id/video"
android:layout_width="match_parent"
android:layout_height="300dp"
/>
</LinearLayout>
Android VideoView code in JAVA
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import android.net.Uri;
import android.os.Bundle;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity {
VideoView video;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
video = findViewById(R.id.video);
video.setVideoURI(Uri.parse("android.resource://" + getPackageName() + "/" + R.raw.bunny));
video.start();
}
}
Methods Used on VideoView
Lets have a look of various methods of android videoview that help us in controlling the video playback like playing, pausing etc.
setVideoUri(Uri uri) : This method is used to set the path of the video file that we want to play in our application.This method takes uri object as argument.
Setting video saved in application itself
Lest see how to save and play a video in the application itself.
Create a folder named raw under res directory like res>raw.Now copy the video file and paste it inside the raw folder.As we mentioned above it should be 3gp or MP4.Use below code to load the video file into videoview.
VideoView video = findViewById(R.id.video);
video.setVideoURI(Uri.parse("android.resource://" + getPackageName() + "/" + R.raw.bunny));
video.start();
Setting video from web url(online)
To access videos from online in our application we need to add internet permission in our manifest file so lets do that b adding below line of code above the <application > tag in manifest file.
<uses-permission android:name="android.permission.INTERNET"/>
Your manifest file should look like this after adding permission
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.codesinsider">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
now run below code
VideoView video = findViewById(R.id.video);
video.setVideoURI(Uri.parse("your 3gp or mp4 video url"));
video.start();
Android videoview project structure
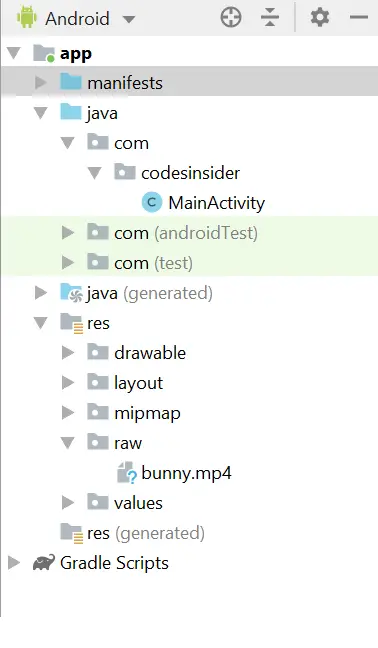
start(): This method is used to start the playback of video file in videoview.
Code snippet to start video in videoview
VideoView video = findViewById(R.id.video);
video.start();//plays the video
pause(): This method is used to pause the current playback of videoview.
Code snippet to pause video in videoview
VideoView video = findViewById(R.id.video);
video.pause();//pauses the video
canPause(): This method will tell whether videoview can pause the video playback.If a video can be paused then it returns true else it returns false.
VideoView video = findViewById(R.id.video);
boolean canPause = video.canPause(); //returns the boolean value(true/false)
canSeekForward(): This method will tell whether we can seek the video forward.If a video can seek forward then it returns true otherwise it returns false.
VideoView video = findViewById(R.id.video);
boolean canSeek = video.canSeekForward(); //returns boolean value(true/false)
canSeekBackward(): This method will tell whether we can seek the video backward.This method returns true if seeking is possible backward and false if not possible.
VideoView video = findViewById(R.id.video);
boolean canSeek = video.canSeekBackward(); //returns boolean value(true/false)
getDuration(): This method returns the total duration of the video which is an integer value.
VideoView video = findViewById(R.id.video);
int duration = video.getDuration();
getCurrentPosition(): This method returns the current position of playback which is also an integer value.
VideoView video = findViewById(R.id.video);
int position = video.getCurrentPosition();
isPlaying(): This method tells whether a video is currently playing or not.It returns true if video is playing and false if not playing.
VideoView video = findViewById(R.id.video);
boolean plaing = video.isPlaying();
stopPlayback(): This method is used to stop the video playback of a videoview.
VideoView video = findViewById(R.id.video);
video.stopPlayback();
setOnPreparedListener(MediaPlayer.OnPreparedListener): This is a listener which triggers a callback method when the video is ready to play.
VideoView video = findViewById(R.id.video);
video.setOnPreparedListener(new MediaPlayer.OnPreparedListener() {
@Override
public void onPrepared(MediaPlayer mediaPlayer) {
//do whatever you want here
}
});
setOnErrorListener(MediaPlayer.OnErrorListener): This is a listener that triggers a callback method when an error occurs during the video playback.
VideoView video = findViewById(R.id.video);
video.setOnErrorListener(new MediaPlayer.OnErrorListener() {
@Override
public boolean onError(MediaPlayer mediaPlayer, int i, int i1) {
//handle the error
return false;
}
});
setOnCompletionListener(MediaPlayer.OnCompletionListener): This is a listener which triggers a callback method when the video playback reaches to end.
video.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mediaPlayer) {
}
});
setMediaController(MediaController controller): This method is used to set the media controller for the controls of video playback to videoview.If media controller is not set then we cannot control the video playback and the video will continue to play without having the user to pause or stop.
MediaController code in XML
<MediaController
android:id="@+id/controller"
android:layout_width="match_parent"
android:layout_height="80dp"/>
MediaController code in JAVA
VideoView video = findViewById(R.id.video);
video.setVideoURI(Uri.parse("android.resource://" + getPackageName() + "/" + R.raw.bunny));
MediaController controller = new MediaController(this);
video.setMediaController(controller);
video.start();
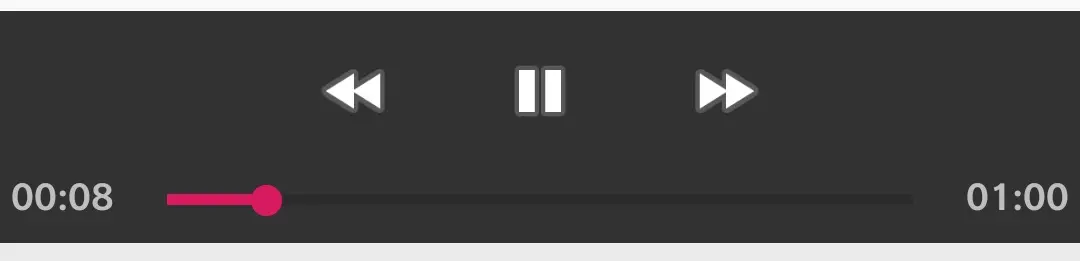
Methods of MediaController
Lets see a few important methods of media controller
setAnchorView(View view): setAnchorView is used to define the view to which the controller is to be anchored.
VideoView video = findViewById(R.id.video);
MediaController controller = new MediaController(this); // create an object
controller.setAnchorView(video);
show(): Used to show the controller on the screen.
MediaController mediaController = new MediaController(this);
mediaController.show();
show(int timeout): Used to set the time to show the media controller on the screen.
MediaController mediaController = new MediaController(this);
mediaController.show(1000);
hide(): Used to hide the media controller from the screen.
MediaController controller = new MediaController(this);
controller.hide();
Android VideoView Example
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<VideoView
android:id="@+id/video"
android:layout_width="match_parent"
android:layout_height="300dp"
android:layout_centerInParent="true"
/>
</RelativeLayout>
MainActivit.java
package com.codesinsider;
import androidx.appcompat.app.AppCompatActivity;
import android.media.MediaPlayer;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.widget.MediaController;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity {
VideoView video;
MediaController controller;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
video = findViewById(R.id.video);
controller = new MediaController(this);
video.setMediaController(controller);
video.setVideoURI(Uri.parse("android.resource://" + getPackageName() + "/" + R.raw.bunny));
video.start();
video.setOnPreparedListener(new MediaPlayer.OnPreparedListener() {
@Override
public void onPrepared(MediaPlayer mediaPlayer) {
mediaPlayer.setOnVideoSizeChangedListener(new MediaPlayer.OnVideoSizeChangedListener() {
@Override
public void onVideoSizeChanged(MediaPlayer mp, int width, int height) {
video.setMediaController(controller);
controller.setAnchorView(video);
}
});
}
});
video.setOnErrorListener(new MediaPlayer.OnErrorListener() {
@Override
public boolean onError(MediaPlayer mediaPlayer, int i, int i1) {
return false;
}
});
video.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mediaPlayer) {
mediaPlayer.release();
}
});
}
}
On completion of playback we must release the media player to avoid memory leaks.To release media player we’ve called mediaPlayer.release() in the above code.
If we observe the code when we call setAnchorView method outside the OnPreparedListener method, the MediaController is not inside the VideoView,it is positioned below the VideoView.Lets see the output.
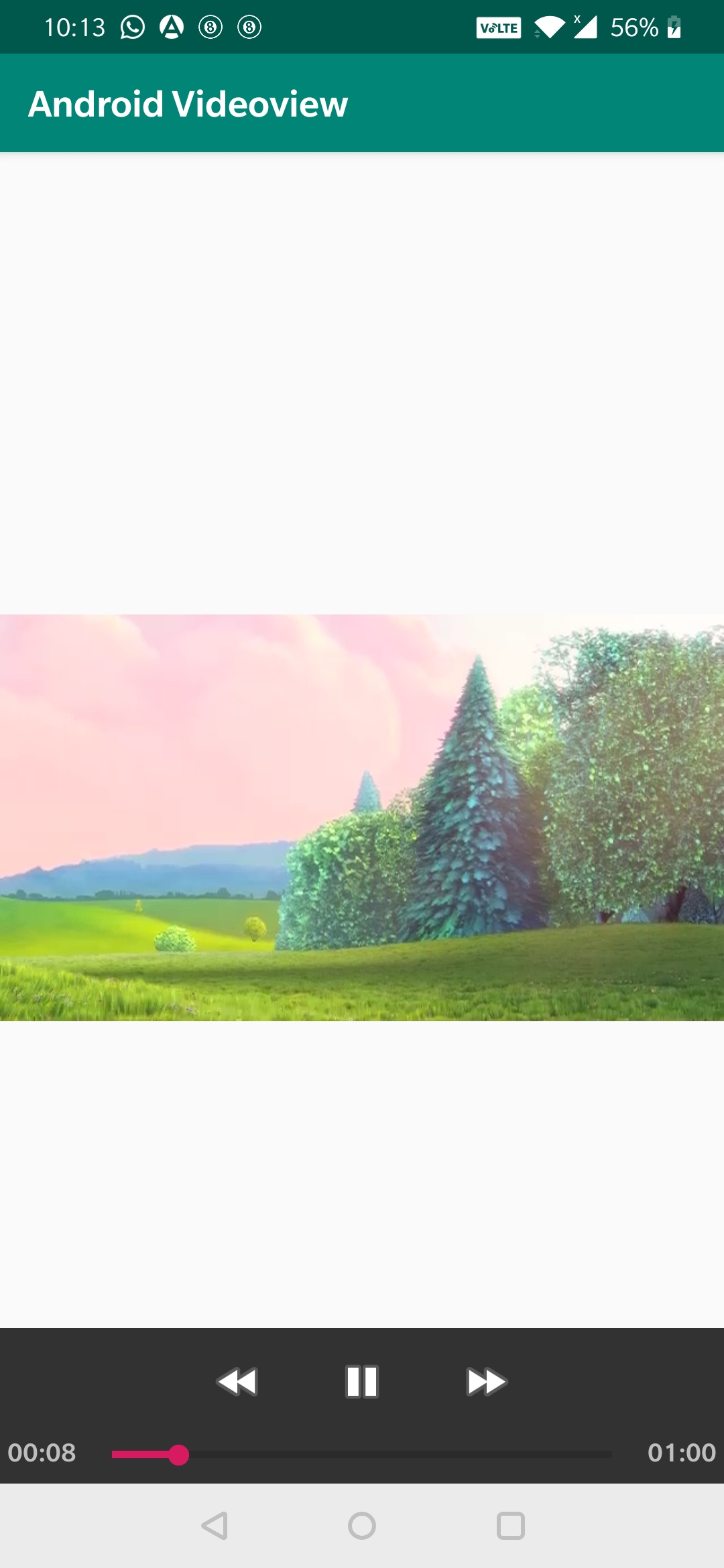
The reason for above scenario is the MediaController can’t get the dimensions of the VideoView until the video playback is started so the MediaController is positioned below the VideoView.For setting media controller inside the videoView, we need to set the anchorView inside OnPreparedListener.Now lets see the final output after code change.
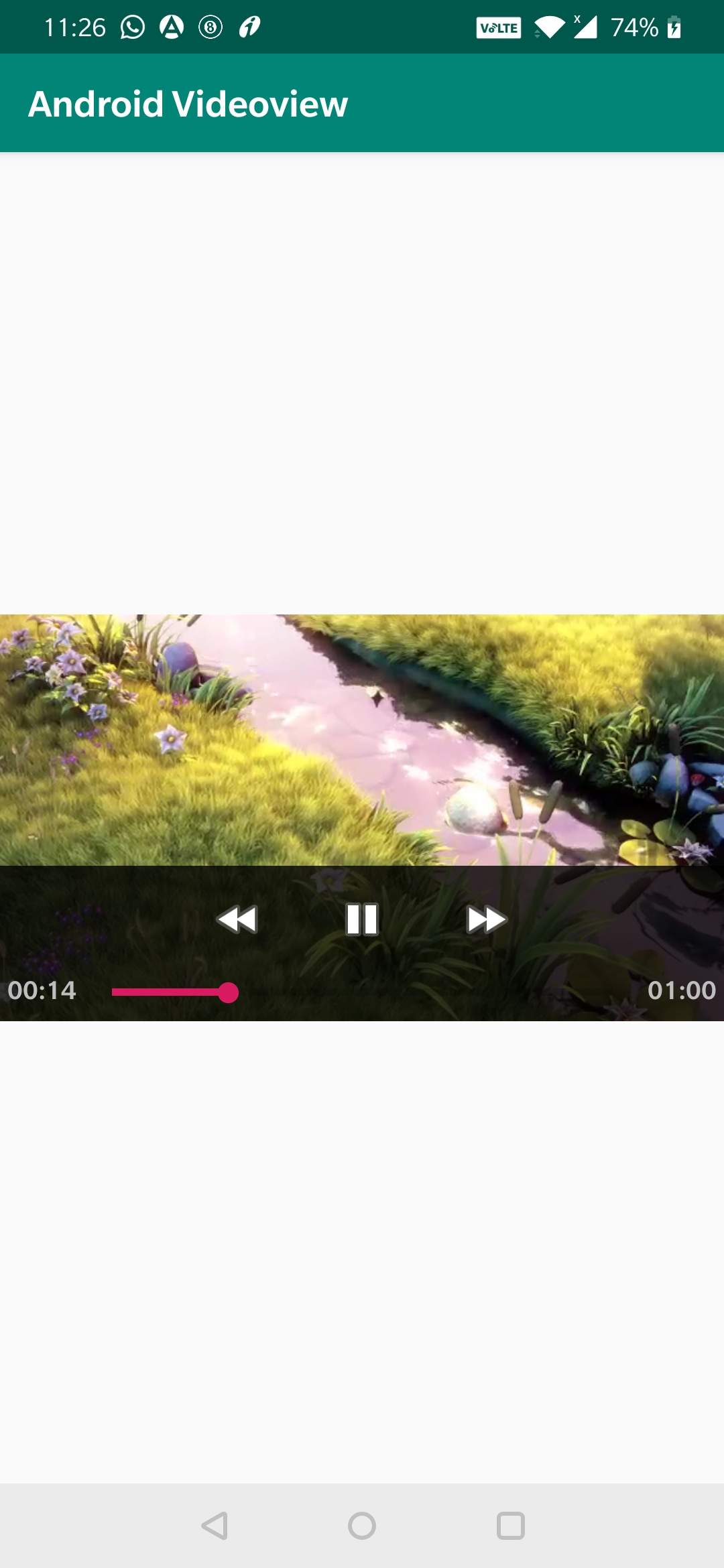
Thats all about android VideoView.In this example tutorial we’ve learnt what is VideoView and its methods.We will discuss other ui controls in next posts.
Do like and share if you find this post helpful.Thank you!!
Reference: Android Official Documentation

Leave a Reply