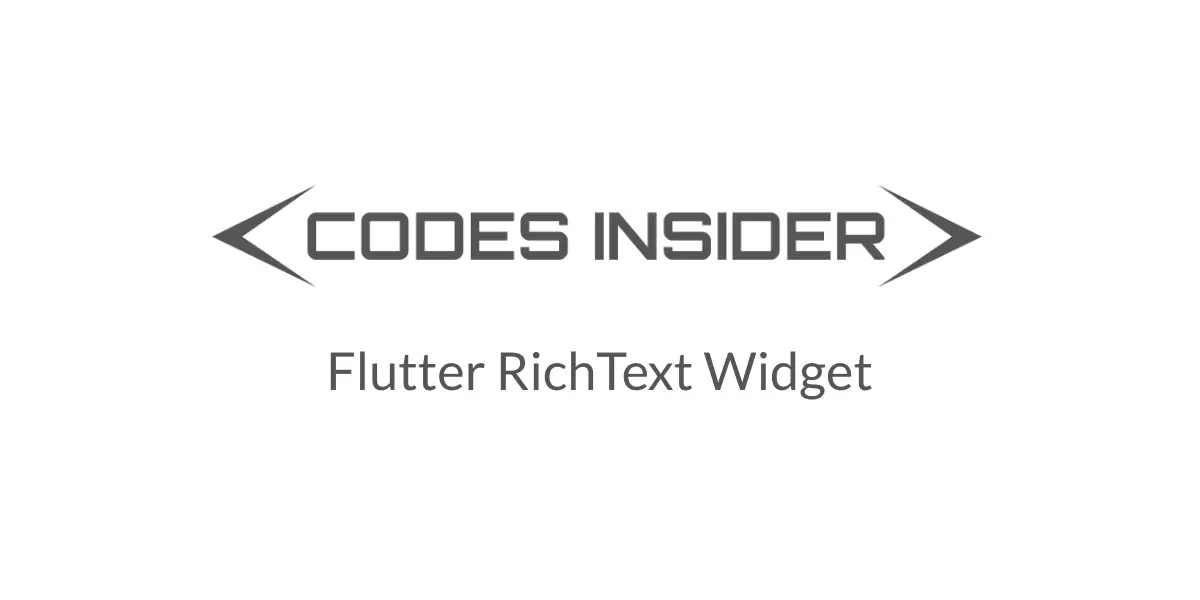
Flutter RichText Widget
The RichText widget in flutter is useful to display text in multiple styles. If we apply a style to a text widget, it will reflect the entire text. To apply different styles to the same text, we will use RichText widget. We can also add hyperlinks using rich text. The text is displayed using a set of TextSpan widgets. We can apply different style to each TextSpan widget.
In this tutorial, you will learn how to create and use a text widget in flutter with example. We will also customize the widget with different properties.
Basic implementation of richtext widget.
RichText(
text:TextSpan( //outer span
style: TextStyle(color: Colors.black54, fontSize: 30),
text:"This is ",
children: <TextSpan>[
TextSpan(text: 'Codesinsider ', style: TextStyle(color: Colors.deepOrange)), //inner span 1
TextSpan(text: 'dot '),
//inner span 2
TextSpan(text: 'com', style: TextStyle(decoration: TextDecoration.underline)) //inner span 3
],
),
)
Output:
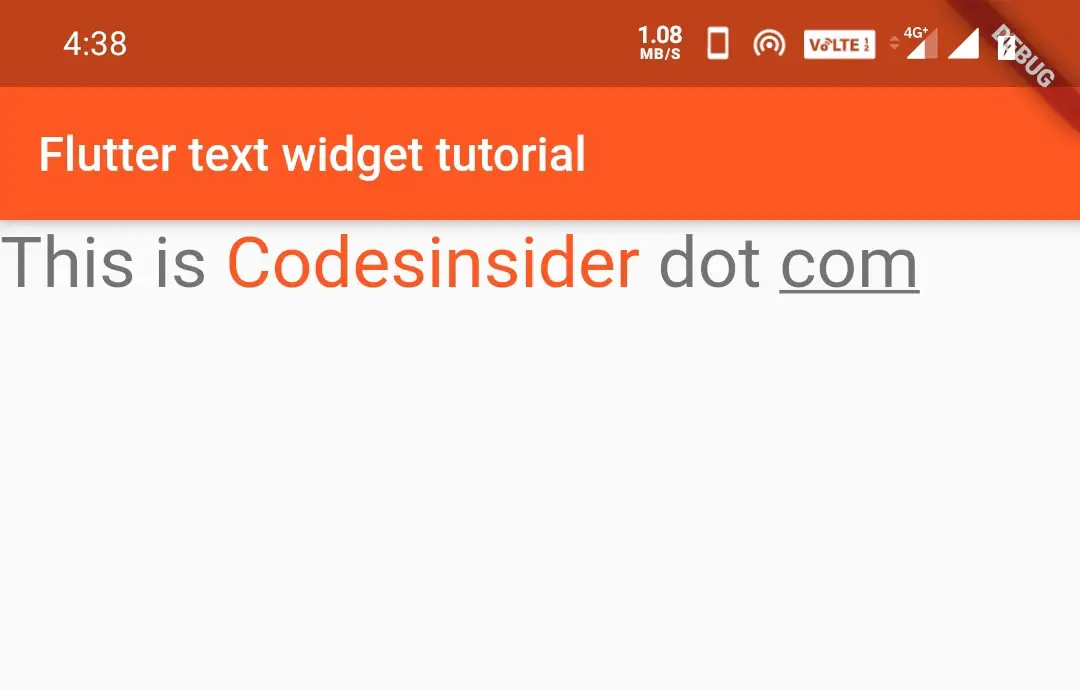
In the above example, we can observe RichText has an outer TextSpan, style, and children of TextSpans. If we apply style to a child, it will reflect only to that child. If we don’t apply a style to a child it will take the style of the outer span.
How To Create RichText In Flutter ?
We can create a richtext widget in flutter by calling its constructor and providing the required properties. If we observe the constructor the text property is a required property. We have to provide some value to that in order to use the widget.
Flutter RichText Constructor:
RichText(
{Key? key,
required InlineSpan text,
TextAlign textAlign,
TextDirection? textDirection,
bool softWrap,
TextOverflow overflow,
double textScaleFactor,
int? maxLines,
Locale? locale,
StrutStyle? strutStyle,
TextWidthBasis textWidthBasis,
TextHeightBehavior? textHeightBehavior}
)
Adding hyperlink to a text in flutter
By using richtext, we can create hyperlinks to text. To create a hyperlink we have to use recognizer parameter of TextSpan class. The recognizer accepts TapGestureRecognizer() as value.
Using url_launcher dependency for hyperlinks in flutter
To create hyperlinks in flutter, we need a dependency called url_launcher. It works with flutter skd version 1.20.2, so you have to download the latest release. Ignore if you already have the latest version.
You can find more info about url_launcher here.
To use url_launcher in flutter, we have to update the pubspec.yaml file with the following code.
dependencies:
flutter:
sdk: flutter
url_launcher: ^5.5.1
After adding the dependency we have to use recognizer parameter of TextSpan class.Lets see an example.
RichText(
text:TextSpan(
style: TextStyle(color: Colors.black54, fontSize: 30),
text:"This is ",
children: <TextSpan>[
TextSpan(text: 'Codesinsider ', style: TextStyle(color: Colors.deepOrange)),
TextSpan(text: 'dot '),
TextSpan(text: 'com', style: TextStyle(decoration: TextDecoration.underline),
recognizer: new TapGestureRecognizer()..onTap = (){
launch("https://codesinsider.com");
}),
],
),
)
So we have successfully created a hyperlink.To add underline to hyperlink, we have used underline constant of TextDecoration class.
Styling RichText Widget
We can apply all styles that are applicable for a text widget to a RichText. Both Text and RichText widgets use TextStyle class for styling.
To learn styling properties for a text widget in detail, you can refer this post about Flutter text widget tutorial.
That brings an end to flutter richtext widget tutorial with examples. Let’s catch up with some other cool flutter tutorial in the next post. Have a great day!!
Do like and share if you find this post helpful.
Thank You!!
Reference: Flutter Official Documentation.

Leave a Reply